习题30:Else和If
people = 30
cars = 40
buses = 15
if people < cars:
print("We should take the cars.")
elif people > cars:
print("We should not take the cars.")
else:
print("We can't decide.")
if buses > cars:
print("That's too many buses.")
elif buses < cars:
print("Maybe we should take the buses.")
else:
print("We still can't decide.")
if people > buses:
print("Alright, let's just take the buses.")
else:
print("Fine, let's stay home then.")
1. 猜想一下 elif 和 else 的功能。
elif后面可以接判断条件,为真执行其包含的语句;为假,跳过。
else,在前面所有条件都为假的时候,执行。
2. 将 cars , people , 和 buses 的数量改掉,然后追溯每一个 if 语句。看看最后会打印出什么来。
3. 试着写一些复杂的布尔表达式,例如 cars > people and buses < cars 。
people = 30
buses = 10
cars = 5
if people < (cars*4):
print("People can go by cars.")
elif people < (buses*10):
print("People can take buses.")
else:
print("It's maybe hard for so many people go together.")
if people > cars and people > buses:
print("Too many people.")
else:
print("It's good!")
4. 在每一行的上面写注解,说明这一行的功用。
习题31:做出决定
print("You enter a dark room with two doors. Do you go through door #1 or door #2?")
door = input(">")
if door == "1":
print("There's a giant bear here eating a cheese cake. What do you do?")
print("1. Take the cake.")
print("2. Scream at the bear")
bear = input(">")
if bear == "1":
print("The bear eats your face off, Good job!")
elif bear == "2":
print("Well, doing %s is probably better. Bear runs away." % bear)
elif door == "2":
print("You stare into the endless abyss at Cthulhu's retina.")
print("1. Blueberries.")
print("2. Yellow jacket clothespins.")
print("3. Understanding revolvers yelling melodies.")
insanity = input(">")
if insanity == "1" or insanity == "2":
print("Your body survives powered by a mind of jello. Good job!")
else:
print("The insanity rots your eyes into a pool of muck. Good job!")
else:
print("You stumble around and fall on a knife and die. Good job!")
习题32:循环和列表
要开始学列表了
the_count = [1, 2, 3, 4, 5]
fruits = ['apples', 'oranges', 'peers', 'apricots']
change = [1, 'pennies', 2, 'dimes', 3, 'quarters']
for number in the_count:
print("This is count %d" % number)
for fruit in fruits:
print("A fruit of type: %s" % fruit)
for i in change:
print("I got %r" % i)
elements = []
for i in range(0, 6):
print("Adding %d to the list." % i)
elements.append(i)
for i in elements:
print("Element was: %d" % i)
1. 注意一下 range 的用法。查一下 range 函数并理解它。
range(start, stop[, step])
start默认为0;stop必填;step默认为1,必须为非零整数
range返回一个左闭右开[left, right)的序列数
2. 在第 22 行,你可以可以直接将 elements 赋值为 range(0,6),而无需使用 for 循环?
the_count = [1, 2, 3, 4, 5]
fruits = ['apples', 'oranges', 'peers', 'apricots']
change = [1, 'pennies', 2, 'dimes', 3, 'quarters']
for number in the_count:
print("This is count %d" % number)
for fruit in fruits:
print("A fruit of type: %s" % fruit)
for i in change:
print("I got %r" % i)
elements = range(0, 6)
for i in elements:
print("Element was: %d" % i)
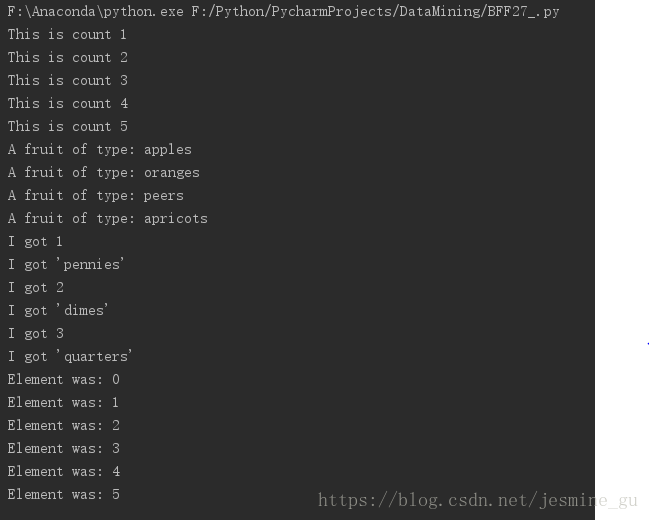
3. 在 Python 文档中找到关于列表的内容,仔细阅读以下,除了 append 以外列表还支持哪些操作?
Python基础教程——列表list: http://www.runoob.com/python/python-lists.html
习题33:while循环
i = 0
numbers = []
while i < 6:
print("At the top i is %d" % i)
numbers.append(i)
i = i + 1
print("Number now:", numbers) # 注意逗号
print("At the bottom i is %d" % i)
print("-------------------------------")
print("The numbers:")
for num in numbers:
print(num)
1. 将这个 while 循环改成一个函数,将测试条件( i < 6 )中的 6 换成一个变量。
2. 使用这个函数重写你的脚本,并用不同的数字进行测试。
3. 为函数添加另外一个参数,这个参数用来定义第 8 行的加值 + 1 ,这样你就可以让它任意加值了。
4. 再使用该函数重写一遍这个脚本。看看效果如何。
i = 0
numbers = []
n = int(input(">")) #自行输入
while i < n:
print("At the top i is %d" % i)
numbers.append(i)
i = i + 1
print("Number now:", numbers) # 注意逗号
print("At the bottom i is %d" % i)
print("-------------------------------")
print("The numbers:")
for num in numbers:
print(num)
5. 接下来使用 for-loop 和 range 把这个脚本再写一遍。你还需要中间的加值操作吗?如果你不去掉它,会有什么样的结果?
不需要了。不去掉程序就不会停止。
numbers = []
for i in range(0, 6):
print("At the top i is %d" % i)
numbers.append(i)
print("Number now:", numbers) # 注意逗号
print("At the bottom i is %d" % i)
print("The numbers:")
for num in numbers:
print(num)
很有可能你会碰到程序跑着停不下来了,这时你只要按着 CTRL 再敲 c (CTRL-c),这样程序就会中断下来了。
今天差不多了,东西有点多。主要是elif、if-else、for、range、while、list