3、接口类 StudentController:
package com.example.demo.controller;
@RestController
public class StudentController {
@RequestMapping(name="/getStudent", method= RequestMethod.POST)
public User getStudent() {
Student student = new Student();
student.setName("小红");
student.setAge(12);
student .setScore(560);
return user;
}
}
@RestController 注解相当于 @ResponseBody + @Controller 合在一起的作用,如果 Web 层的类上使用了 @RestController 注解,就代表这个类中所有的方法都会以 JSON 的形式返回结果,也相当于 JSON 的一种快捷使用方式;
@RequestMapping(name=“/getStudent”, method= RequestMethod.POST),以 /getStudent 的方式去请求,method= RequestMethod.POST 是指只可以使用 Post 的方式去请求,如果使用 Get 的方式去请求的话,则会报 405 不允许访问的错误。
4、测试类:
在 test 包下新建 ControllerTest 测试类,对 getUser() 方法使用MockMvc进行测试。
package com.example.demo;
@RunWith(SpringRunner.class)
@SpringBootTest
@AutoConfigureMockMvc
public class ControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void getStudent() throws Exception{
String responseString = mockMvc.perform(MockMvcRequestBuilders.get("/getStudent"))
.andReturn().getResponse().getContentAsString();
System.out.println("result : "+responseString);
}
}
运行Test,得到结果:
result : {"name":"小红","age":12,"score":650}
说明 Spring Boot 自动将 Student对象转成了 JSON 进行返回。那么如果返回的是一个 list 呢?
@RequestMapping("/getStudents")
public List<Student> getStudents() {
List<Student> students = new ArrayList<>();
Student student1 = new Student();
student1.setName("王小宏");
student1.setAge(31);
student1.setScore(635);
students.add(student1);
Student student2 = new Student();
student2.setName("宋小专");
student2.setAge(27);
student2.setScore(522);
students.add(student2);
return students;
}
添加测试方法:
@Test
public void getStudents() throws Exception{
String responseString = mockMvc.perform(MockMvcRequestBuilders.get("/getStudents"))
.andReturn().getResponse().getContentAsString();
System.out.println("result : "+responseString);
}
运行测试结果,得到结果:
result : [{"name":"王小宏","age":31,"score":635},{"name":"宋小专","age":27,"score":522}]
==============================================================
前端浏览器和后端服务器正是依赖交互过程中的参数完成了诸多用户操作行为,因此参数的传递和接收是一个 Web 系统最基础的功能。SpringBoot对参数接收做了很好的支持,内置了很多种参数接收方式,提供一些注解来帮助限制请求的类型、接收不同格式的参数等。
Get与Post:
如果我们希望一个接口以Post方式访问,在方法上添加一个配置(method= RequestMethod.POST)即可:
@RequestMapping(name="/getStudent", method= RequestMethod.POST)
public User getStudent() {
...
}
如果以Get方法请求该接口,将得到【Request method ‘GET’ not supported】的报错。
同样,如果是GET 请求,method 设置为:method= RequestMethod.GET;如果不进行设置默认两种方式的请求都支持。
请求传参一般分为 URL 地址传参和表单传参两种方式,都以键值对的方式将参数传递到后端。作为后端程序不用关注前端采用的那种方式,只需要根据参数的键来获取值。
只要后端处理请求的方法中存在参数键相同名称的属性,在请求的过程中 Spring 会自动将参数值赋值到属性中,最后在方法中直接使用即可。
@RequestMapping(name = "/test", method = RequestMethod.GET)
public String test(String name) {
return name;
}
测试:
@RunWith(SpringRunner.class)
@SpringBootTest
@AutoConfigureMockMvc
public class ControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void getStudents() throws Exception {
String responseString = mockMvc.perform(MockMvcRequestBuilders.get("/test?name=xiaohong"))
.andReturn().getResponse().getContentAsString();
System.out.println("result : " + responseString);
}
}
结果:
result : xiaohong
另一种方式:
@RequestMapping(value="test/{name}", method=RequestMethod.GET)
public String get(@PathVariable String name) {
return name;
}
这样的写法,传参地址栏会更加美观一些。
测试:
@Test
public void getStudents() throws Exception {
String responseString = mockMvc.perform(MockMvcRequestBuilders.get("/test/xiaohong"))
.andReturn().getResponse().getContentAsString();
# 总结
面试前的“练手”还是很重要的,所以开始面试之前一定要准备好啊,不然也是耽搁面试官和自己的时间。
我自己是刷了不少面试题的,所以在面试过程中才能够做到心中有数,基本上会清楚面试过程中会问到哪些知识点,高频题又有哪些,所以刷题是面试前期准备过程中非常重要的一点。
# 面试题及解析总结
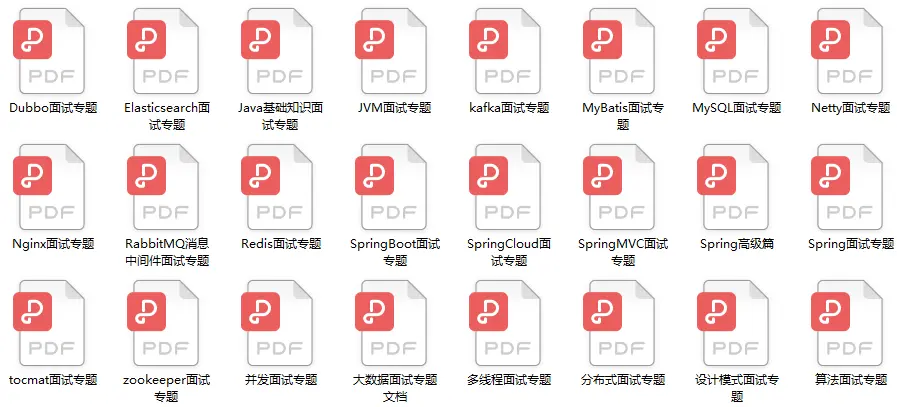
# 大厂面试场景
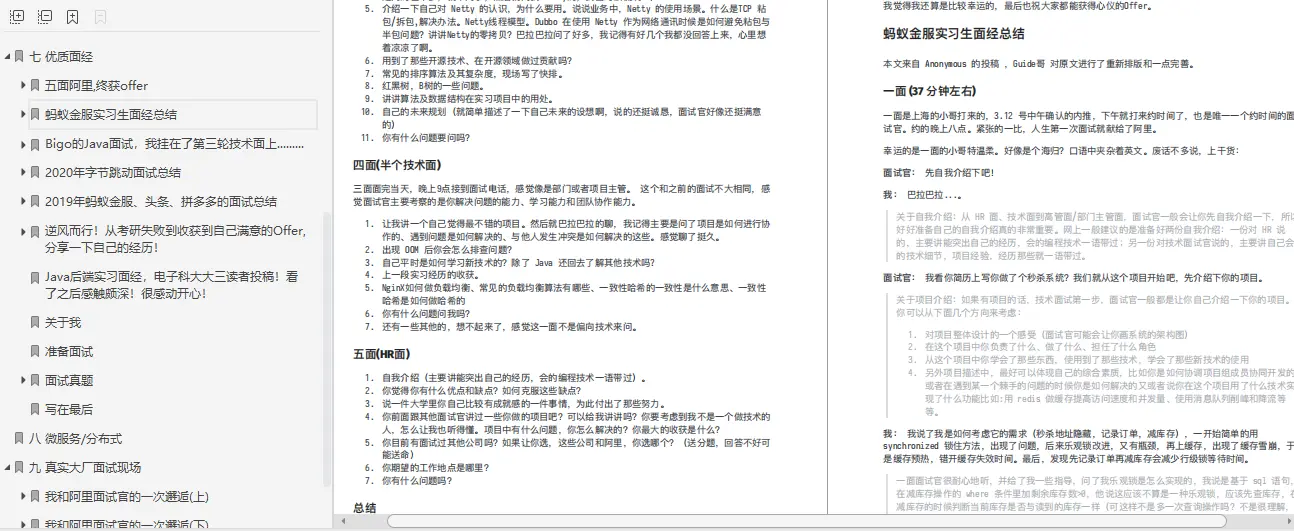
# 知识点总结
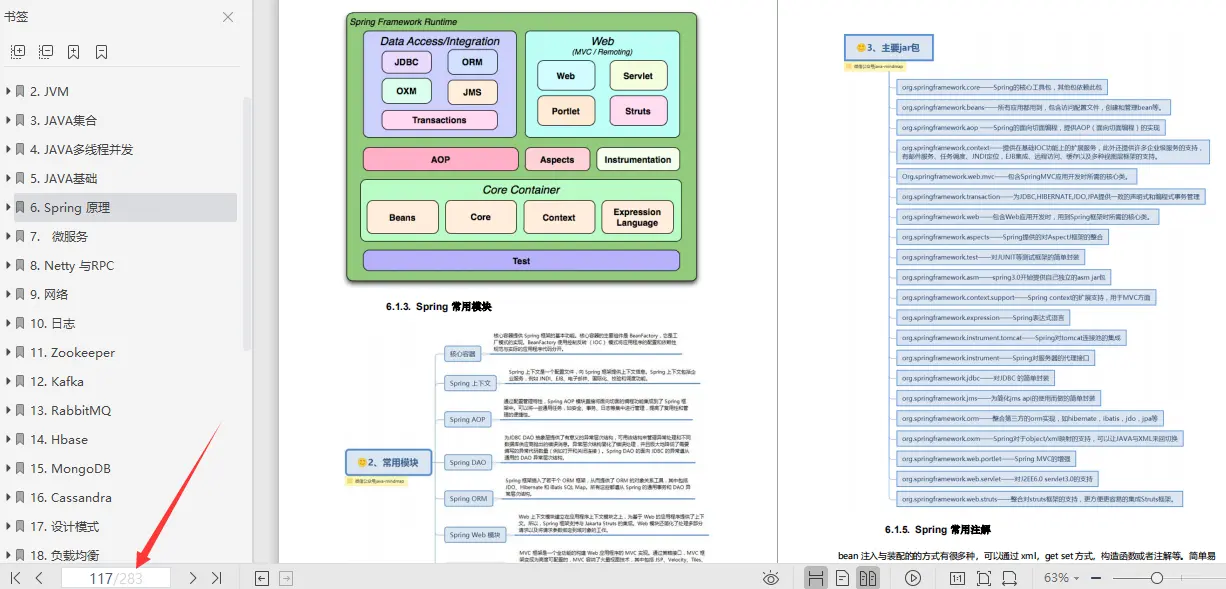
eturn().getResponse().getContentAsString();
# 总结
面试前的“练手”还是很重要的,所以开始面试之前一定要准备好啊,不然也是耽搁面试官和自己的时间。
我自己是刷了不少面试题的,所以在面试过程中才能够做到心中有数,基本上会清楚面试过程中会问到哪些知识点,高频题又有哪些,所以刷题是面试前期准备过程中非常重要的一点。
# 面试题及解析总结
[外链图片转存中...(img-A3zESprr-1714520695904)]
# 大厂面试场景
[外链图片转存中...(img-EmRsoOel-1714520695905)]
# 知识点总结
[外链图片转存中...(img-SGSnUAod-1714520695905)]
> **本文已被[CODING开源项目:【一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频+实战项目源码】](https://bbs.csdn.net/topics/618154847)收录**