A constant is a symbol that has a never-changing value.
常量被视为类型定义的一部分(即常量被当作静态成员,而非实例成员)。定义常量导致元数据的创建。
常量的值必须在编译时确定。在编译时,编译器将常量的值嵌入到IL代码中(即常量在编译时被替换,有点像C语言中的宏),因此不能获取常量,也不能将常量通过引用的方式进行传递。
常量存在跨程序集版本控制控制的问题。
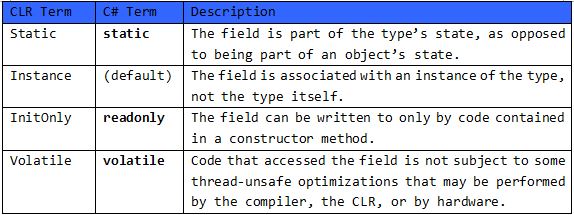
常量与字段的差别
常量被视为类型定义的一部分(即常量被当作静态成员,而非实例成员)。定义常量导致元数据的创建。
常量的值必须在编译时确定。在编译时,编译器将常量的值嵌入到IL代码中(即常量在编译时被替换,有点像C语言中的宏),因此不能获取常量,也不能将常量通过引用的方式进行传递。
常量存在跨程序集版本控制控制的问题。
A field is a data member that holds an instance of a value type or a reference to a reference type.
字段存储在动态内存中,因此它们的值在运行时才能获取。常量与字段的差别
1)常量的值必须在编译时确定,字段的值在运行时才能获取;
2)只能定义编译器识别的基元类型的常量,而字段没有限制;
3)常量存在跨程序集版本控制的问题。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace MyField
{
class SomeType
{
// SomeType is not a primitive type but C# does allow
// a constant variable of this type to be set to 'null'.
public const SomeType Empty = null;
// NOTE: C# doesn't allow you to specify static for constants
// because constants are always implicitly static.
public const Int32 MaxEntriesInList = 50;
// The static is required to associate the field with the type.
public static readonly Int32 MinEntriesInList = 5;
}
class TestType
{
// This is a static readonly field; its value is calculated and
// stored in memory when this class is initialized at run time.
public static readonly Random s_random = new Random();
// This is a static read/write field.
private static Int32 s_numberOfWrites = 0;
// This is an instance readonly field.
public readonly String Pathname = "Untitled";
// This is an instance read/write field.
private System.IO.FileStream m_fs;
public TestType(String pathname)
{
// This line changes a readonly field.
// This is OK because the code is in a constructor.
this.Pathname = pathname;
}
public String DoSomething()
{
// This line reads and writes to the static read/write field.
s_numberOfWrites = s_numberOfWrites + 1;
// This line reads the readonly instance field.
return Pathname;
}
}
public sealed class AType
{
// InvalidChars must always refer to the same array object
public static readonly Char[] InvalidChars = new Char[] { 'A', 'B', 'C' };
}
}
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace MyField
{
class Program
{
static void Main(string[] args)
{
// The lines below are legal, compile, and successfully
// change the characters in the InvalidChars array
AType.InvalidChars[0] = 'X';
AType.InvalidChars[1] = 'Y';
AType.InvalidChars[2] = 'Z';
// The line below is illegal and will not compile because
// what InvalidChars refers to cannot be changed
// AType.InvalidChars = new Char[] { 'X', 'Y', 'Z' };
Console.ReadKey();
}
}
}