import com.google.common.hash.Hashing;
import org.apache.commons.codec.digest.DigestUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.*;
import java.security.DigestInputStream;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class ShaUtils {
private static final Logger LOG = LoggerFactory.getLogger(ShaUtils.class);
/**
* 使用apache.commons计算SAH-256哈希值
* @param absolutePathFile 形如:/home/admin/0dd5cd96e0ac445d82b265c8f582cd0d.pdf
* @return
*/
public static String sha256_01(String absolutePathFile) {
LOG.info("使用apache.commons计算SAH-256哈希值,{}", absolutePathFile);
String result = null;
try {
result = DigestUtils.sha256Hex(new FileInputStream(absolutePathFile));
} catch (Exception e) {
LOG.error("使用apache.commons计算SAH-256异常", e);
}
return result;
}
/**
* 使用google.guava计算SAH-256哈希值
* @param absolutePathFile 形如:/home/admin/0dd5cd96e0ac445d82b265c8f582cd0d.pdf
* @return
*/
public static String sha256_02(String absolutePathFile) {
LOG.info("使用google.guava计算SAH-256哈希值,{}", absolutePathFile);
String result = null;
try {
result = Hashing.sha256().hashBytes(FileUtils.file2Byte(absolutePathFile)).toString();
} catch (Exception e) {
LOG.error("使用google.guava计算SAH-256异常", e);
}
return result;
}
/**
* 使用java.security计算SAH-256哈希值
* @param absolutePathFile 形如:/home/admin/0dd5cd96e0ac445d82b265c8f582cd0d.pdf
* @return
*/
public static String sha256_03(String absolutePathFile) {
LOG.info("使用java.security计算SAH-256哈希值,{}", absolutePathFile);
MessageDigest md;
try {
md = MessageDigest.getInstance("SHA-256");
} catch (NoSuchAlgorithmException e) {
throw new IllegalArgumentException(e);
}
try (InputStream is = new FileInputStream(absolutePathFile);
DigestInputStream dis = new DigestInputStream(is, md)) {
while (dis.read() != -1) ; //empty loop to clear the data
md = dis.getMessageDigest();
} catch (IOException e) {
throw new IllegalArgumentException(e);
}
return bytesToHex(md.digest());
}
/**
* 该方法很容易出错
*/
private static String bytesToHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
/**
* 使用Linux命令行的sha256sum命令计算SAH-256哈希值
* @param absolutePathFile
* @return
*/
public static String sha256_04(String absolutePathFile) {
LOG.info("使用Linux命令行的sha256sum命令哈希,{}", absolutePathFile);
String result = null;
InputStream in = null;
BufferedReader read = null;
try {
Process pro = Runtime.getRuntime().exec(new String[]{"/bin/sh", "-c", "sha256sum " + absolutePathFile});
pro.waitFor();
in = pro.getInputStream();
read = new BufferedReader(new InputStreamReader(in));
result = read.readLine();
if (result != null) {
String[] arr = result.split(" ");
if (arr != null && arr.length > 0) {
return arr[0];
}
}
} catch (Exception e) {
LOG.error("使用Linux命令行的sha256sum命令哈希异常", e);
} finally {
if (in != null) {
try {
in.close();
} catch (Exception e) {
}
}
if (read != null) {
try {
read.close();
} catch (Exception e) {
}
}
}
return result;
}
}
SHA-256算法的种种
最新推荐文章于 2024-08-15 11:57:21 发布
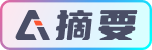