先看效果
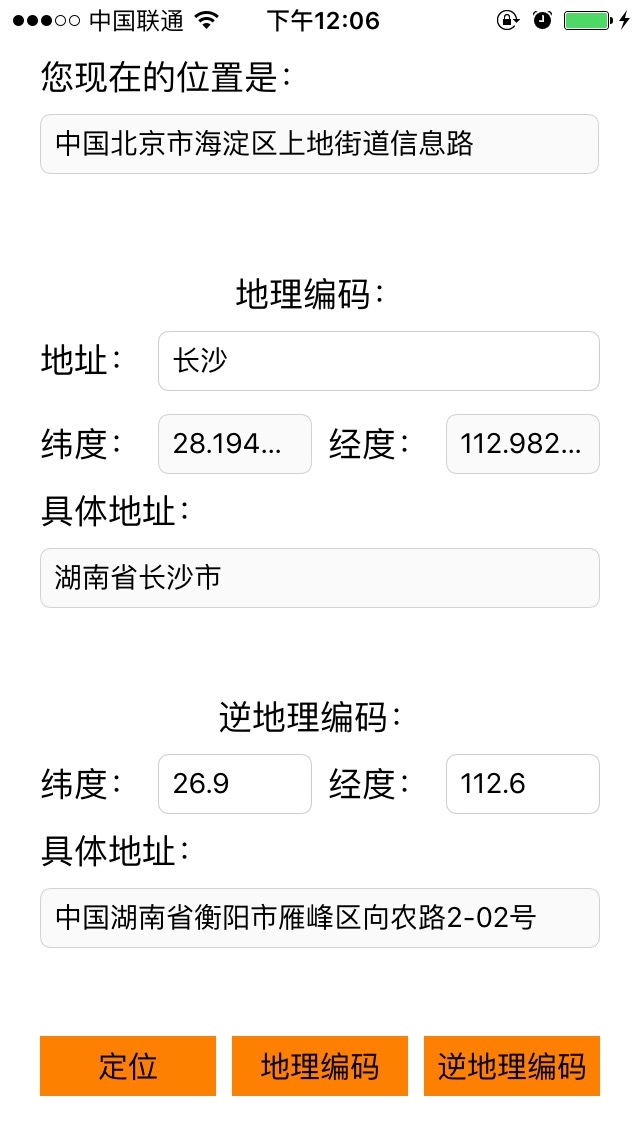
代码下载
代码下载请猛戳这里!
再看代码
- (CLLocationManager *)locationManager
{
NSLog(@"%i,%i",[CLLocationManager locationServicesEnabled],[CLLocationManager authorizationStatus]);
if ((![CLLocationManager locationServicesEnabled]) || [CLLocationManager authorizationStatus] == kCLAuthorizationStatusDenied) {
[self showAlertViewControllerWithMessage:@"请到\"设置—隐私—定位服务\"开启本软件的定位服务"];
return nil;
}
if (!_locationManager) {
_locationManager = [[CLLocationManager alloc] init];
_locationManager.delegate = self;
_locationManager.desiredAccuracy = kCLLocationAccuracyBest;
_locationManager.distanceFilter = 1000.0f;
if ([[UIDevice currentDevice].systemVersion floatValue] > 8.0) {
[_locationManager requestWhenInUseAuthorization];
}
}
return _locationManager;
}
#pragma mark - 控制器周期
- (void)dealloc
{
[[NSNotificationCenter defaultCenter] removeObserver:self];
}
- (void)viewDidLoad {
[super viewDidLoad];
[self.locTextField becomeFirstResponder];
NSLog(@"衡阳到长沙:%f米",[[[CLLocation alloc] initWithLatitude:28.194 longitude:112.982] distanceFromLocation:[[CLLocation alloc] initWithLatitude:29.6 longitude:112.6]]);
[[NSNotificationCenter defaultCenter] addObserver:self selector:@selector(keyBoardChanged:) name:UIKeyboardWillChangeFrameNotification object:nil];
}
- (void)viewDidAppear:(BOOL)animated
{
[super viewDidAppear:animated];
[self.locationManager startUpdatingLocation];
}
#pragma mark - 触摸点击方法
- (IBAction)locationAction:(UIButton *)sender {
[self.locationManager startUpdatingLocation];
}
- (IBAction)geocodingAction:(UIButton *)sender {
if (![self isBlinkStr:self.locTextField.text]) {
[self geoCodingLocationStr:self.locTextField.text];
}
}
- (IBAction)reGeocodingAction:(UIButton *)sender {
[self.view endEditing:YES];
if ((![self isBlinkStr:self.reLatitudeTextField.text] && (![self isBlinkStr:self.reLongitudeTextField.text]))) {
[self reGeoCodingLatitude:[self.reLatitudeTextField.text doubleValue] andLongitude:[self.reLongitudeTextField.text doubleValue]];
}
else
{
[self showAlertViewControllerWithMessage:@"请输入经纬度!"];
}
}
- (void)touchesEnded:(NSSet<UITouch *> *)touches withEvent:(UIEvent *)event
{
[self.view endEditing:YES];
}
- (void)keyBoardChanged:(NSNotification *)sender
{
UIView *view = [self findFirstResponder:self.view];
CGRect keyBF = [sender.userInfo[UIKeyboardFrameEndUserInfoKey] CGRectValue];
CGFloat distance = CGRectGetMaxY(view.frame) - keyBF.origin.y;
[UIView beginAnimations:nil context:nil];
[UIView setAnimationDuration:[sender.userInfo[UIKeyboardAnimationDurationUserInfoKey] doubleValue]];
[UIView setAnimationCurve:[sender.userInfo[UIKeyboardAnimationDurationUserInfoKey] integerValue]];
if (distance > 0) {
CGFloat spacing = 8;
self.view.transform = CGAffineTransformMakeTranslation(0, -distance - spacing);
}
else
{
self.view.transform = CGAffineTransformIdentity;
}
}
#pragma mark - 自定义方法
- (void)geoCodingLocationStr:(NSString *)str
{
CLGeocoder *geocoder = [[CLGeocoder alloc] init];
[geocoder geocodeAddressString:str completionHandler:^(NSArray<CLPlacemark *> * _Nullable placemarks, NSError * _Nullable error) {
if (error) {
[self showAlertViewControllerWithMessage:@"您的地址输入有误!"];
}
else
{
CLPlacemark *placeMark = [placemarks firstObject];
self.latitudeTextField.text = [NSString stringWithFormat:@"%f",placeMark.location.coordinate.latitude];
self.longitudeTextField.text = [NSString stringWithFormat:@"%f",placeMark.location.coordinate.longitude];
self.detailLocTextField.text = placeMark.name;
}
}];
}
- (void)reGeoCodingLatitude:(CLLocationDegrees)latitude andLongitude:(CLLocationDegrees)longitude
{
CLLocation *location = [[CLLocation alloc] initWithLatitude:latitude longitude:longitude];
CLGeocoder *geocoder = [[CLGeocoder alloc] init];
[geocoder reverseGeocodeLocation:location completionHandler:^(NSArray<CLPlacemark *> * _Nullable placemarks, NSError * _Nullable error) {
if (error) {
[self showAlertViewControllerWithMessage:@"没有找到此经纬度的地理信息!"];
}
else
{
CLPlacemark *pMark = [placemarks firstObject];
self.reDetailLocTextField.text = pMark.name;
}
}];
}
- (BOOL)isBlinkStr:(NSString *)str
{
if (str == nil || str == NULL) {
return YES;
}
else if ([str isKindOfClass:[NSNull class]])
{
return YES;
}
else if ([[str stringByTrimmingCharactersInSet:[NSCharacterSet whitespaceAndNewlineCharacterSet]] length] == 0)
{
return YES;
}
return NO;
}
- (void)showAlertViewControllerWithMessage:(NSString *)message
{
UIAlertController *alertController = [UIAlertController alertControllerWithTitle:@"提示" message:message preferredStyle:UIAlertControllerStyleAlert];
UIAlertAction *okAction = [UIAlertAction actionWithTitle:@"确定" style:UIAlertActionStyleCancel handler:nil];
[alertController addAction:okAction];
[self presentViewController:alertController animated:YES completion:nil];
}
- (UIView *)findFirstResponder:(UIView *)view
{
if ([view isFirstResponder]) {
return view;
}
for (UIView *theView in view.subviews) {
UIView *firstView = [self findFirstResponder:theView];
if (firstView) {
return firstView;
}
}
return nil;
}
#pragma mark - <CLLocationManagerDelegate>代理方法
- (void)locationManager:(CLLocationManager *)manager didUpdateLocations:(NSArray<CLLocation *> *)locations
{
CLLocation *location = [locations lastObject];
CLGeocoder *geocoder = [[CLGeocoder alloc] init];
[geocoder reverseGeocodeLocation:location completionHandler:^(NSArray<CLPlacemark *> * _Nullable placemarks, NSError * _Nullable error) {
if (error) {
NSLog(@"此地址错误");
}
else
{
CLPlacemark *pMark = [placemarks firstObject];
self.currentLocTextField.text = pMark.name;
NSLog(@"%f,%f,%@,%@,%@",location.coordinate.latitude,location.coordinate.longitude,pMark.name,pMark.locality,pMark.addressDictionary[@"City"]);
}
}];
[manager stopUpdatingLocation];
}
#pragma mark - <UITextFieldDelegate>代理方法
- (BOOL)textFieldShouldReturn:(UITextField *)textField
{
if (textField == self.locTextField && (![self isBlinkStr:self.locTextField.text])) {
[self geoCodingLocationStr:self.locTextField.text];
}
else if ((textField == self.reLatitudeTextField || textField == self.reLongitudeTextField) && (![self isBlinkStr:self.latitudeTextField.text]) && (![self isBlinkStr:self.longitudeTextField.text]))
{
[self reGeoCodingLatitude:[self.reLatitudeTextField.text doubleValue] andLongitude:[self.reLongitudeTextField.text doubleValue]];
}
[textField resignFirstResponder];
return YES;
}