一、背景
- 本篇文章是将以前Redis实战的系列文章进行汇总,针对Redis中常用的一些数据结构,进行实战模拟。
strings |
hashes |
lists |
sets |
sorted sets |
封锁一个IP地址 |
存储用户信息 |
模拟消息队列 |
自动排重 |
以某一个条件为权重,进行排序 |
1.1 开发环境
- JDK 1.8
- SpringBoot 2.2.5
- JPA
- Spring Security
- Mysql 8.0
- Redis Server 3.2.1
- Redis Desktop Manager
- Swagger2
1.2 项目配置
<!--pom.xl-->
<!--Redis-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<!-- application.yml-->
server:
port: 8000
spring:
freemarker:
check-template-location: false
profiles:
active: dev
jackson:
time-zone: GMT+8
data:
redis:
repositories:
enabled: false
jpa:
properties:
hibernate:
dialect: org.hibernate.dialect.MySQL5InnoDBDialect
open-in-view: true
redis:
database: 0
host: 127.0.0.1
port: 6379
password:
- 增加RedisConfig配置类
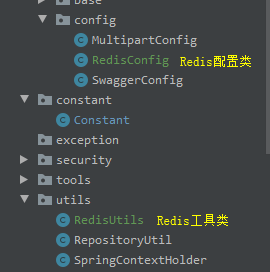
@Configuration
@EnableCaching
@ConditionalOnClass(RedisOperations.class)
@EnableConfigurationProperties(RedisProperties.class)
public class RedisConfig extends CachingConfigurerSupport {
@Bean
public RedisCacheConfiguration redisCacheConfiguration(){
FastJsonRedisSerializer<Object> fastJsonRedisSerializer = new FastJsonRedisSerializer<>(Object.class);
RedisCacheConfiguration configuration = RedisCacheConfiguration.defaultCacheConfig();
configuration = configuration.serializeValuesWith(RedisSerializationContext.SerializationPair.fromSerializer(fastJsonRedisSerializer)).entryTtl(Duration.ofHours(Constant.CACHE_TIMEOUT_HOUR));
return configuration;
}
@Bean
public RedisTemplate<Object, Object> redisTemplate(RedisConnectionFactory redisConnectionFactory) {
RedisTemplate<Object, Object> template = new RedisTemplate<>();
template.setConnectionFactory(redisConnectionFactory);
FastJsonRedisSerializer fastJsonRedisSerializer = new FastJsonRedisSerializer(Object.class);
template.setValueSerializer(fastJsonRedisSerializer);
template.setHashValueSerializer(fastJsonRedisSerializer);
ParserConfig.getGlobalInstance().setAutoTypeSupport(true);
template.setKeySerializer(new StringRedisSerializer());
template.setHashKeySerializer(new StringRedisSerializer());
template.afterPropertiesSet();
return template;
}
@Bean
@Override
public KeyGenerator keyGenerator() {
return (target, method, params) -> {
Map<String,Object> container = new HashMap<>(3);
Class<?> targetClassClass = target.getClass();
container.put("class",targetClassClass.toGenericString());
container.put("methodName",method.getName());
container.put("package",targetClassClass.getPackage());
for (int i = 0; i < params.length; i++) {
container.put(String.valueOf(i),params[i]);
}
String jsonString = JSON.toJSONString(container);
return DigestUtils.sha256Hex(jsonString);
};
}
- 增加RedisUtils工具类:实现对各种数据结构的封装
@Component
@AllArgsConstructor
public class RedisUtils {
private RedisTemplate<Object, Object> redisTemplate;
public Object hashGet(String key, String item) {
return redisTemplate.opsForHash().get(key, item);
}
public boolean hashSet(String key, String item, Object value) {
try {
redisTemplate.opsForHash().put(key, item, value);
return true