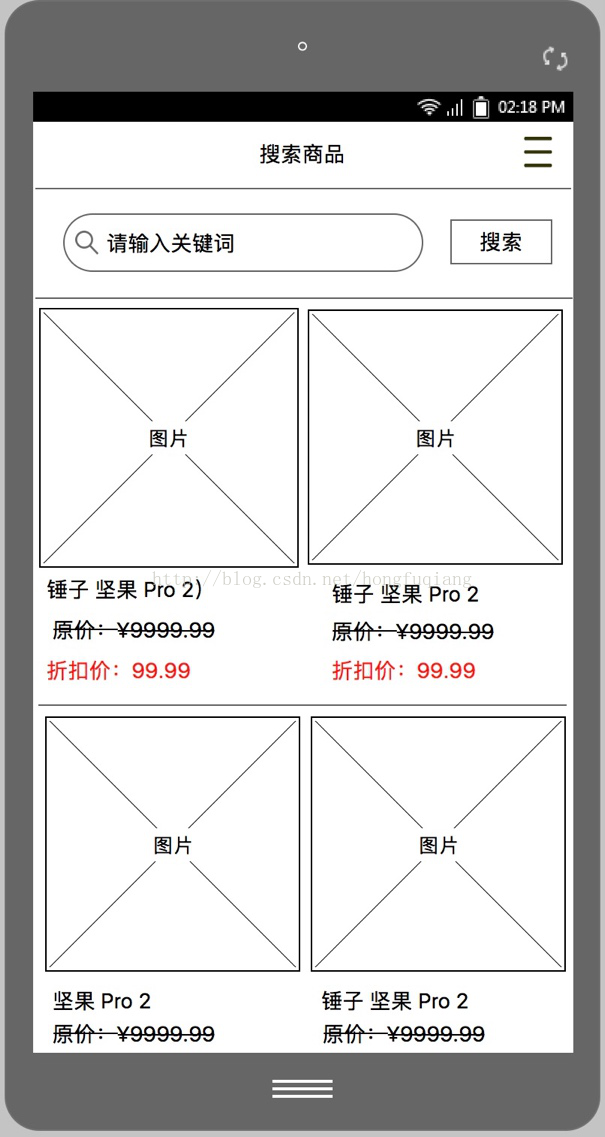
源代码如下:
首先要导入依赖
compile 'com.android.support:recyclerview-v7:25.3.1' compile 'com.github.bumptech.glide:glide:3.7.0' compile 'com.squareup.okhttp3:okhttp:3.9.0' compile 'com.jcodecraeer:xrecyclerview:1.3.2' compile 'com.google.code.gson:gson:2.8.2'再就是主布局
main布局
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:textSize="24dp" android:layout_marginTop="10dp" android:text="搜索商品"/> <Button android:id="@+id/btn_grid_lv" android:layout_width="42dp" android:layout_height="42dp" android:layout_alignParentEnd="true" android:layout_alignParentRight="true" android:layout_alignParentTop="true" android:background="@drawable/grid_icon" /> <TextView android:id="@+id/textView" android:layout_marginTop="5dp" android:layout_width="match_parent" android:layout_height="2dp" android:layout_below="@+id/btn_grid_lv" android:layout_centerHorizontal="true" android:background="#000" /> <LinearLayout android:id="@+id/ll" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal" android:layout_alignTop="@+id/textView" android:layout_alignParentStart="true"> <EditText android:id="@+id/et_seek" android:layout_weight="5" android:layout_width="0dp" android:layout_height="50dp" /> <Button android:id="@+id/btn_seek" android:layout_weight="1" android:layout_width="0dp" android:layout_height="wrap_content" android:text="搜索" /> </LinearLayout> <com.jcodecraeer.xrecyclerview.XRecyclerView android:id="@+id/xrv" android:layout_below="@+id/ll" android:layout_width="match_parent" android:layout_height="wrap_content"> </com.jcodecraeer.xrecyclerview.XRecyclerView> </RelativeLayout>第二个布局
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:id="@+id/list_img" android:layout_width="100dp" android:layout_height="100dp" android:src="@mipmap/ic_launcher" /> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical"> <TextView android:id="@+id/list_title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:layout_marginLeft="10dp" android:text="手机"/> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:layout_marginLeft="10dp" android:text="原价:9999"/> <TextView android:id="@+id/list_price" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="10dp" android:layout_marginLeft="50dp" android:text="折扣价:"/> </LinearLayout> </LinearLayout> </LinearLayout>第三个布局
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="horizontal" android:layout_width="match_parent" android:layout_height="match_parent"> <ImageView android:id="@+id/list_img" android:layout_width="200dp" android:layout_height="200dp" android:src="@mipmap/ic_launcher" /> <TextView android:id="@+id/list_title" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="10dp" android:text="手机" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="10dp" android:text="原价:$9999" /> <TextView android:id="@+id/list_price" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="10dp" android:text="折扣价:" /> </LinearLayout>
创建Adapter包和Adapter类
public class XAdapter extends RecyclerView.Adapter<XAdapter.ViewHolder> { private Context context; private List<Bean.DataBean> list; private int myResource; public XAdapter(Context context, List<Bean.DataBean> list, int myResource) { this.context = context; this.list = list; this.myResource = myResource; } @Override public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View view = View.inflate(context, myResource, null); ViewHolder holder = new ViewHolder(view); return holder; } @Override public void onBindViewHolder(ViewHolder holder, int position) { String[] split = list.get(position).getImages().split("\\|"); Log.e("--------",split[0]); Glide.with(context).load(split[0]).into(holder.listimg); holder.listprice.setText("折扣价:$"+list.get(position).getPrice()); holder.listtitle.setText(list.get(position).getTitle()); } @Override public int getItemCount() { return list.size(); } class ViewHolder extends RecyclerView.ViewHolder{ private ImageView listimg; private TextView listprice; private TextView listtitle; public ViewHolder(View itemView) { super(itemView); listimg =(ImageView)itemView.findViewById(R.id.list_img); listprice =(TextView)itemView.findViewById(R.id.list_price); listtitle = (TextView) itemView.findViewById(R.id.list_title); } } }创建Bean包
bean类
private String msg; private String code; private String page; private List<DataBean> data;创建persener类 p层
public class Perseners { private static final String TAG = "Perseners"; private Iview inv; private List<Bean.DataBean> list; public void attach(Iview inv){ this.inv=inv; } public void get(Map<String,String> map){ HttpUtils.getInstance().get("http://120.27.23.105/product/searchProducts", map, new CallBack() { @Override public void onSuccess(Object o) { Bean bean=(Bean) o; list = bean.getData(); //Log.e(TAG, "onSuccess: "+list.toString()); inv.success(list); } @Override public void onFailed(Exception e) { inv.failed(e); } },Bean.class); } //解决内存泄漏问题 public void deleteView(){ if(inv!=null){ inv=null; } } }
CallBack类 放两个接口
public interface CallBack { void onSuccess(Object o); void onFailed(Exception e); }HttpUtils类
public class HttpUtils { private static volatile HttpUtils instance; private static Handler handler = new Handler(); private HttpUtils(){ } public static HttpUtils getInstance() { if (instance == null) { synchronized (HttpUtils.class) { if (instance == null) { instance = new HttpUtils(); } } } return instance; } //get请求 public void get(String url, Map<String,String> map, final CallBack callBack, final Class c){ //对url和参数做拼接处理 StringBuffer stringBuffer = new StringBuffer(); stringBuffer.append(url); //判断是否存在? if中是存在 if(stringBuffer.indexOf("?")!=-1 ){ //判断?是否在最后一位 if中是不在最后一位 if(stringBuffer.indexOf("?")!=stringBuffer.length()-1){ stringBuffer.append("&"); } }else{ stringBuffer.append("?"); } for(Map.Entry<String,String> entry:map.entrySet()){ stringBuffer.append(entry.getKey()) .append("=") .append(entry.getValue()) .append("&"); } //判断是否存在& if中是存在 if(stringBuffer.indexOf("&")!=-1){ stringBuffer.deleteCharAt(stringBuffer.lastIndexOf("&")); } //1:创建OkHttpClient对象 OkHttpClient okHttpClient = new OkHttpClient.Builder() .addInterceptor(new Logger()) .build(); //2:创建Request对象 final Request request = new Request.Builder() .get() .url(stringBuffer.toString()) .build(); //3:创建Call对象 Call call = okHttpClient.newCall(request); //4:请求网络 call.enqueue(new Callback() { //请求失败 @Override public void onFailure(Call call, final IOException e) { handler.post(new Runnable() { @Override public void run() { callBack.onFailed(e); } }); } //请求成功 @Override public void onResponse(Call call, Response response) throws IOException { String result = response.body().string(); //拿到数据解析 final Object o = new Gson().fromJson(result, c); //当前是在子线程,回到主线程中 handler.post(new Runnable() { @Override public void run() { //回调 callBack.onSuccess(o); } }); } }); } //post请求 public void post(String url, Map<String,String> map, final CallBack callBack, final Class c){ //1:创建OkHttpClient对象 OkHttpClient okHttpClient = new OkHttpClient(); //2:提供post请求需要的body对象 FormBody.Builder builder = new FormBody.Builder(); for(Map.Entry<String,String> entry:map.entrySet()){ builder.add(entry.getKey(),entry.getValue()); } FormBody body = builder.build(); //3:创建Request对象 final Request request = new Request.Builder() .post(body) .url(url) .build(); //4:创建Call对象 Call call = okHttpClient.newCall(request); //5:请求网络 call.enqueue(new Callback() { //请求失败 @Override public void onFailure(Call call, final IOException e) { handler.post(new Runnable() { @Override public void run() { callBack.onFailed(e); } }); } //请求成功 @Override public void onResponse(Call call, Response response) throws IOException { String result = response.body().string(); //拿到数据解析 final Object o = new Gson().fromJson(result, c); //当前是在子线程,回到主线程中 handler.post(new Runnable() { @Override public void run() { //回调 callBack.onSuccess(o); } }); } }); } }
Iview类 也是放接口的
public interface Iview { void success(List<Bean.DataBean> list); void failed(Exception e); }
拦截器的代码
public class Logger implements Interceptor { @Override public Response intercept(Chain chain) throws IOException { Request original = chain.request(); HttpUrl url=original.url().newBuilder() .addQueryParameter("source","android") .build(); //添加请求头 Request request = original.newBuilder() .url(url) .build(); return chain.proceed(request); } }
mainActivity主页
public class MainActivity extends AppCompatActivity implements XRecyclerView.LoadingListener,Iview{ private XRecyclerView xrv; private TextView etseek; private Button btn; private TextView btnseek; private Perseners perseners; int flag=1; int page=1; private XAdapter xAdapter; private ArrayList<Bean.DataBean> list = new ArrayList<>(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); xrv =(XRecyclerView)findViewById(R.id.xrv); btn =(Button)findViewById(R.id.btn_grid_lv); etseek =(TextView)findViewById(R.id.et_seek); btnseek =(TextView) findViewById(R.id.btn_seek); //设置上下刷新 xrv.setLoadingListener(this); xrv.setPullRefreshEnabled(true); xrv.setLoadingMoreEnabled(true); xAdapter = new XAdapter(MainActivity.this,list,R.layout.list1); LinearLayoutManager linearLayoutManager=new LinearLayoutManager(this); xrv.setLayoutManager(linearLayoutManager); xrv.setAdapter(xAdapter); //p层对象 perseners = new Perseners(); perseners.attach(this); Map<String,String> map = new HashMap<>(); map.put("keywords","笔记本"); map.put("page","1"); perseners.get(map); btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { flag++; if (flag%2==0){ btn.setBackgroundResource(R.drawable.grid_icon); GridLayoutManager manager=new GridLayoutManager(MainActivity.this,2); xrv.setLayoutManager(manager); xAdapter = new XAdapter(MainActivity.this, list, R.layout.list1); xrv.setAdapter(xAdapter); }else{ btn.setBackgroundResource(R.drawable.lv_icon); LinearLayoutManager linearLayoutManager=new LinearLayoutManager(MainActivity.this); xrv.setLayoutManager(linearLayoutManager); xAdapter = new XAdapter( MainActivity.this, list,R.layout.list2); xrv.setAdapter(xAdapter); } } }); btnseek.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { String string = etseek.getText().toString(); list.clear(); Map<String,String> map = new HashMap<>(); map.put("keywords",string);//keywords=笔记本&page=1 map.put("page","1");//keywords=笔记本&page=1 perseners.get(map); } }); } @Override public void onRefresh() { page=1; list.clear(); Map<String,String> map = new HashMap<>(); map.put("keywords","笔记本"); map.put("page","1"); perseners.get(map); xrv.refreshComplete(); } @Override public void onLoadMore() { page++; Map<String,String> map = new HashMap<>(); map.put("keywords","笔记本");//keywords=笔记本&page=1 map.put("page",page+"");//keywords=笔记本&page=1 perseners.get(map); xrv.refreshComplete(); } @Override public void success(List<Bean.DataBean> list) { this.list.addAll(list); Log.e("嗝屁", "success: "+list.toString() ); xAdapter.notifyDataSetChanged(); } @Override public void failed(Exception e) { } //内存泄漏 @Override protected void onDestroy() { super.onDestroy(); if (perseners!=null){ perseners.deleteView(); } } }
文章最后进行网络请求
<uses-permission android:name="android.permission.INTERNET"></uses-permission> <uses-permission android:name="android.permission.MOUNT_UNMOUNT_FILESYSTEMS"></uses-permission> <uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"></uses-permission> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"></uses-permission>