既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,涵盖了95%以上大数据知识点,真正体系化!
由于文件比较多,这里只是将部分目录截图出来,全套包含大厂面经、学习笔记、源码讲义、实战项目、大纲路线、讲解视频,并且后续会持续更新
if not os.path.isfile(self.gift_json):
raise error.NotFileError()
if name == ‘__main__’:
user_path = os.path.join(os.getcwd(), "storage", "user.json")
gift_path = os.path.join(os.getcwd(), "storage", "gift.json")
print(user_path)
print(gift_path)
base = Base(user_json=user_path, gift_json=gift_path)
print(base)
### 🌟 common 模块 的 error.py 脚本的代码如下:
coding:utf-8
class NotPathError(Exception): # 文件路径错误
def __init__(self, message):
self.message = message
class FormatError(Exception): # 文件格式后缀错误
def __init__(self, message=“file need json format”):
self.message = message
class NotFileError(Exception): # 非文件错误
def __init__(self, message=“It’s not file”):
self.message = message
此时在 `base.py` 基础模块中我们发现, `__check_user_json()` 与 `__check_gift_json()` 函数的基本功能是一样的,都是检查文件。这个时候就可以将其封装为一个公共函数 `check_file` 用以调用,所以我们可以在 `utils.py` 模块定义一个 `check_file` 函数。
### 🌟 utils.py 模块 check\_file 函数示例如下
coding:utf-8
import os
from .error import NotPathError, NotFileError, FormatError
def check_file(path):
if not os.path.exists(path): # 判断文件地址路径是否存在
raise NotPathError("not found {} ".format(path))
if not path.endswith('.json'): # 判断文件是否是 json 格式
raise FormatError()
if not os.path.isfile(path): # 判断是否是文件
raise NotFileError()
那么此时我们的 `base.py` 基础模块优化后的脚本代码就如下:
coding:utf-8
“”"
1:导入 user.json ,文件检查
2:导入 gift.json ,文件检查
“”"
import os
from common.utils import check_file
class Base(object):
def __init__(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self):
check_file(self.user_json)
def \_\_check\_gift\_json(self):
check_file(self.gift_json)
if name == ‘__main__’:
user_path = os.path.join(os.getcwd(), "storage", "user.json")
gift_path = os.path.join(os.getcwd(), "storage", "gift.json")
print(user_path)
print(gift_path)
base = Base(user_json=user_path, gift_json=gift_path)
print(base)
执行结果如下:
---
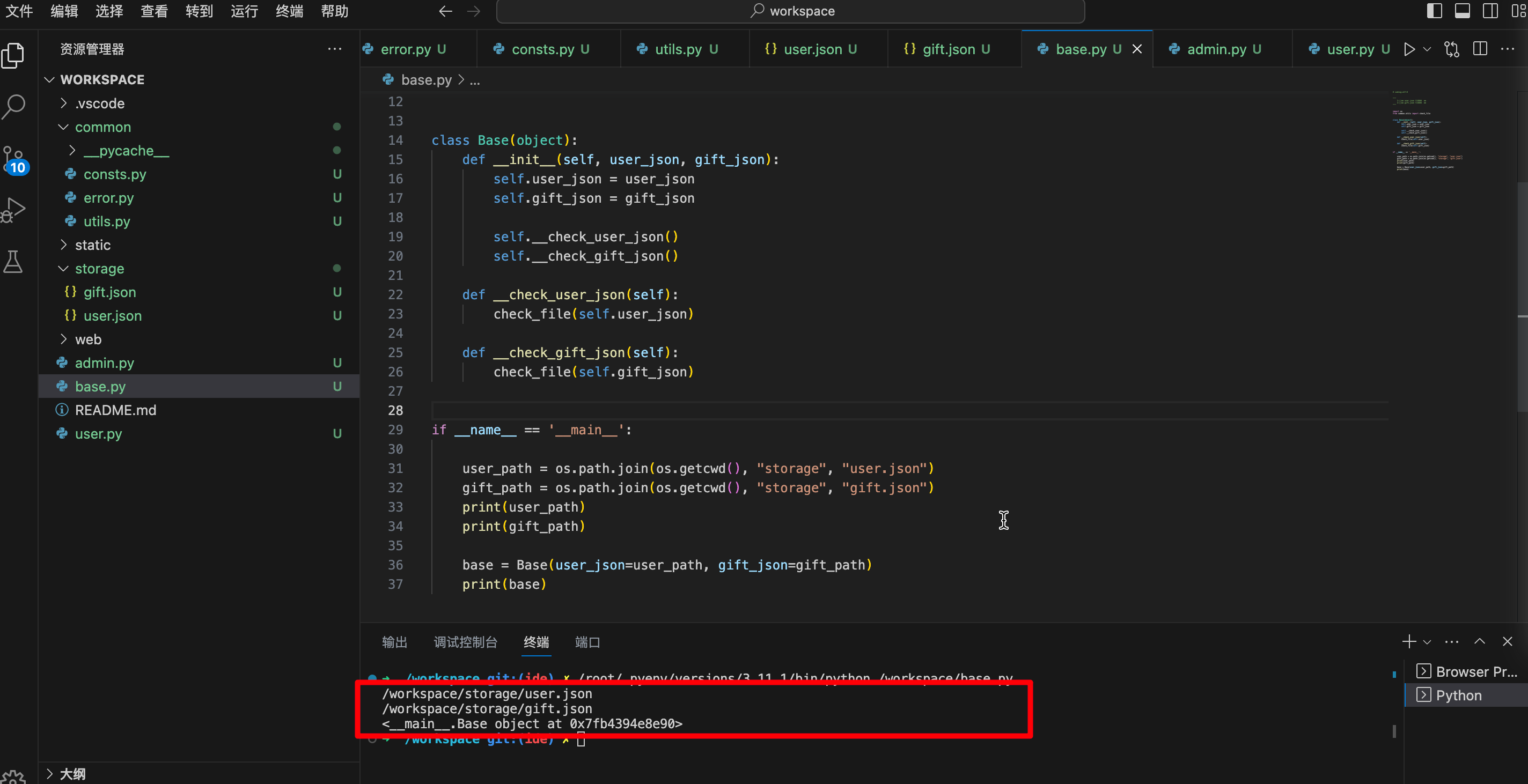
---
`尝试做几个文件异常的判断:`
---
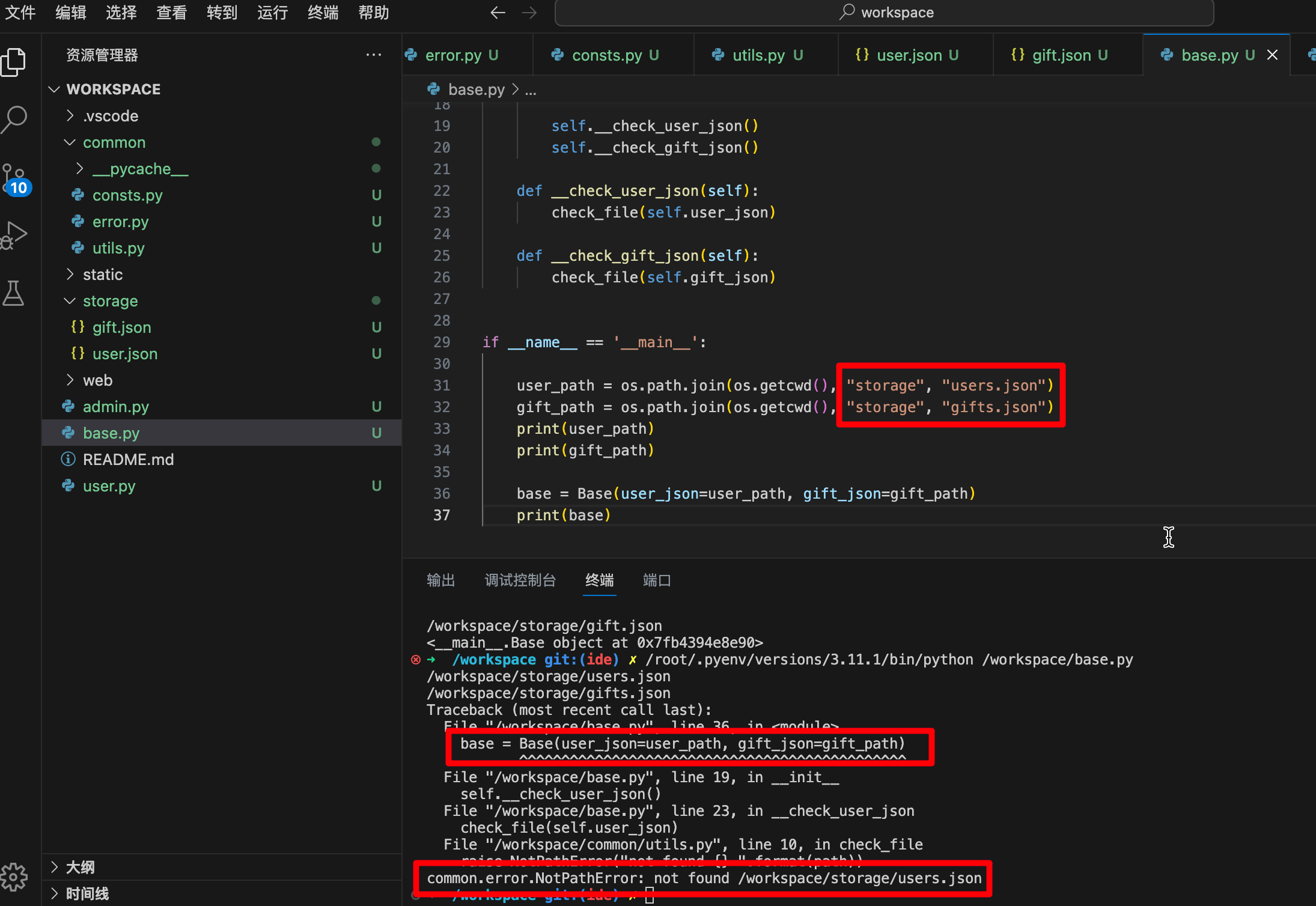
---
## ⭐️ base用户相关功能实现
在 `base.py` 模块基础上,增加 `__read_users()` 与 `__write_user()` 函数。
所以接下来,我们就实现一下前三个目标。
coding:utf-8
“”"
1:导入 user.json ,文件检查
2:导入 gift.json ,文件检查
*************************
3、确定用户表中每个用户的信息字段
4、读取 user.json
文件
5、写入 user.json
文件(检测该用户是否存在),存在则不可写入
“”"
import os
import json
import time
from common.utils import check_file
from common.error import UserExistsError
class Base(object):
def __init__(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 user.json 文件
check_file(self.user_json)
def \_\_check\_gift\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 gift.json 文件
check_file(self.gift_json)
def \_\_read\_user(self): # 读取 user.json 文件
with open(self.user_json) as f:
data = json.loads(f.read())
return data
def \_\_write\_user(self, \*\*user): # 写入用户信息(进行写入时的判断)
if "username" not in user:
raise ValueError("missing username") # 缺少 username 信息
if "role" not in user:
raise ValueError("missing role") # 缺少角色信息(缺少权限信息)
user['active'] = True # 初始化用户基础信息
user['create\_time'] = time.time()
user['update\_time'] = time.time()
user['gifts'] = []
users = self.__read_user() # 读取 user.json
print(users) # 打印输出 users 是为了调试
return # 打印输出调试 users ,return 是为了不在执行后面的代码
if user['username'] in users: # 判断用户信息是否存在,如果存在则抛出 'error.py' 模块自定义的 UserExistsError
raise UserExistsError('username {} had existe'.format(user['username']))
users.update(
{user['username']: user}
)
json_users = json.dumps(users)
with open(self.user_json, 'w') as f:
f.write(json_users)
if name == ‘__main__’:
user_path = os.path.join(os.getcwd(), "storage", "user.json")
gift_path = os.path.join(os.getcwd(), "storage", "gift.json")
print(user_path)
print(gift_path)
base = Base(user_json=user_path, gift_json=gift_path)
print(base)
base.write_user(username='Neo', role='admin')
### 🌟 将 时间戳 封装为 utils.py 模块的一个公共函数
coding:utf-8
import os
import time
from .error import NotPathError, NotFileError, FormatError
def timestamp_to_string(timestamp):
time_obj = time.localtime(timestamp) # 将传入的时间戳实例化成一个时间对象
time_str = time.strftime('%Y-%m-%d %H:%M:%S ', time_obj)
return time_str
def check_file(path):
if not os.path.exists(path): # 判断文件地址路径是否存在
raise NotPathError("not found %s " % path)
if not path.endswith('.json'): # 判断文件是否是 json 格式
raise FormatError()
if not os.path.isfile(path): # 判断是否是文件
raise NotFileError()
### 🌟 对 base.py 模块中的 \_\_read\_user() 进行修改
coding:utf-8
“”"
1:导入 user.json ,文件检查
2:导入 gift.json ,文件检查
*************************
3、确定用户表中每个用户的信息字段
4、读取 user.json
文件
5、写入 user.json
文件(检测该用户是否存在),存在则不可写入
******************************************************
“”"
import os
import json
import time
from common.utils import check_file, timestamp_to_string
from common.error import UserExistsError
class Base(object):
def __init__(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 user.json 文件
check_file(self.user_json)
def \_\_check\_gift\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 gift.json 文件
check_file(self.gift_json)
def \_\_read\_user(self, time_to_str=True): # 读取 user.json 文件;
# timestamp\_to\_string 修改为 True 时时间戳为可读的格式(调试)
with open(self.user_json, 'r') as f:
data = json.loads(f.read())
if time_to_str == True:
for username, t in data.items():
t['create\_time'] = timestamp_to_string(t['create\_time'])
# print(t['create\_time']) # 打印输出时间格式是否改为 可读的 "%Y-%m-%d %H:%M:%S" 格式
t['update\_time'] = timestamp_to_string(t['update\_time'])
data[username] = t
print(data) # 调试打印输出 \_\_read\_user() 的用户信息
return data
def write\_user(self, \*\*user): # 写入用户信息(进行写入时的判断)
if "username" not in user:
raise ValueError("missing username") # 缺少 username 信息
if "role" not in user:
raise ValueError("missing role") # 缺少角色信息(缺少权限信息)
user['active'] = True # 初始化用户基础信息
user['create\_time'] = time.time()
user['update\_time'] = time.time()
user['gifts'] = []
users = self.__read_user() # 读取 user.json
print(users) # 打印输出 users 是为了调试
return # 打印输出调试 users ,return 是为了不在执行后面的代码
if user['username'] in users: # 判断用户信息是否存在,如果存在则抛出 'error.py' 模块自定义的 UserExistsError
raise UserExistsError('username {} had existe'.format(user['username']))
users.update(
{user['username']: user}
)
json_users = json.dumps(users)
with open(self.user_json, 'w') as f:
f.write(json_users)
print(users) # 调试打印输出 users 的用户信息
if name == ‘__main__’:
user_path = os.path.join(os.getcwd(), "storage", "user.json")
gift_path = os.path.join(os.getcwd(), "storage", "gift.json")
print(user_path)
print(gift_path)
base = Base(user_json=user_path, gift_json=gift_path)
base.write_user(username='Neo', role='admin')
这里将代码中的如下两行取消注释,且将 `def __read_user(self, time_to_str=False)` 改为 `def __read_user(self, time_to_str=True)`可以看到调试的 读取文件时,时间戳变为 `"%Y-%m-%d %H:%M:%S" 的时间格式`
# print(users) # 打印输出 users 是为了调试
# return # 打印输出调试 users ,return 是为了不在执行后面的代码
#*******************************************************************************
print(users) # 打印输出 users 是为了调试
return # 打印输出调试 users ,return 是为了不在执行后面的代码
## ⭐️ base 模块 - 用户的修改与删除
接下来我们继续对 `base.py` 模块关于 `user.json` 文件进行相关的操作,同样是三个目标。
>
> 1、对于某个用户 `role` 权限的修改(或者说是身份的修改) `__change_role() 函数`
>
>
> 2、对于 acitve 用户的活跃度的修改
>
>
> 3、delete\_user 删除某个用户的修改
>
>
>
>
> ---
>
>
> `PS:以上三个函数,依然是` base `类的内置函数;大家可能会有个疑问,为什么现在使用的一些函数都是内置函数呢?原因是未来我们开发 "admin"、"user" 相关类的时候,会覆盖或包裹这些内置函数在它们的外层进行业务相关的开发`
>
>
>
>
> ---
>
>
> * 用户信息的字段:
> + username:姓名
> + role:角色(normal 或 admin)
> + active:活跃度(身份是否有效:True or False)
> + create\_time:创建时间(timestamp)
> + update\_time:更新时间(timestamp)
> + gifts:奖品列表(用以存储用户抽到了哪些奖品)
> * 用户信息存储格式:(字典)
> + username:{username, role, active}
>
>
>
先来编写 `__change_role() 函数`
coding:utf-8
“”"
1:导入 user.json ,文件检查 “__check_user_json() 函数”
2:导入 gift.json ,文件检查 “__check_gift_json() 函数”
*******************************************************
3、确定用户表中每个用户的信息字段
4、读取 user.json
文件 “__read_users() 函数”
5、写入 user.json
文件(检测该用户是否存在),存在则不可写入;“__write_user() 函数” ,这里方便调试,先使用 write_user()
***********************************************************************************************************
6、对于某个用户 role
权限的修改(或者说是身份的修改) \_\_change\_role() 函数
; 同样方便调试,先使用 change_role()
7、对于 acitve 用户的活跃度的修改; \_\_change\_acitve() 函数
;
8、delete_user 删除某个用户的修改
***********************************************************************************************************
“”"
import os
import json
import time
from common.consts import ROLES
from common.error import UserExistsError, RoleError, UserNameNotExist
from common.utils import check_file, timestamp_to_string
class Base(object):
def __init__(self, user_json, gift_json):
self.user_json = user_json
self.gift_json = gift_json
self.__check_user_json()
self.__check_gift_json()
def \_\_check\_user\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 user.json 文件
check_file(self.user_json)
def \_\_check\_gift\_json(self): # 调用 utils 模块的公共函数 check\_file 检查 gift.json 文件
check_file(self.gift_json)
def \_\_read\_users(self, time_to_str=False): # 读取 user.json 文件;
# timestamp\_to\_string 修改为 True 时时间戳为可读的格式(调试)
with open(self.user_json, 'r') as f:
data = json.loads(f.read())
if time_to_str == True:
for username, t in data.items():
t['create\_time'] = timestamp_to_string(t['create\_time'])
# print(t['create\_time']) # 打印输出时间格式是否改为 可读的 "%Y-%m-%d %H:%M:%S" 格式
t['update\_time'] = timestamp_to_string(t['update\_time'])
data[username] = t
print(data) # 调试打印输出 \_\_read\_user() 的用户信息
return data
def write\_user(self, \*\*user): # 写入用户信息(进行写入时的判断)
if "username" not in user:
raise ValueError("missing username") # 缺少 username 信息
if "role" not in user:
raise ValueError("missing role") # 缺少角色信息(缺少权限信息)
user['active'] = True # 初始化用户基础信息
user['create\_time'] = time.time()
user['update\_time'] = time.time()
user['gifts'] = []
users = self.__read_users() # 读取 user.json
# print(users) # 打印输出 users 是为了调试
# return # 打印输出调试 users ,return 是为了不在执行后面的代码
if user['username'] in users: # 判断用户信息是否存在,如果存在则抛出 'error.py' 模块自定义的 UserExistsError
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
打印输出 users 是为了调试
# return # 打印输出调试 users ,return 是为了不在执行后面的代码
if user['username'] in users: # 判断用户信息是否存在,如果存在则抛出 'error.py' 模块自定义的 UserExistsError
[外链图片转存中…(img-R1O3e50y-1715371548134)]
[外链图片转存中…(img-7gGLtaql-1715371548134)]
网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!