Rose N. Blatt is designing an embedded neural network to place inside a cell phone. When trained by the phone's owner, the neural network will enable the user to dictate text messages in a hands-free way. The key idea in Rose's design is the use of complicated polynomial response functions in each of the nodes of the network (rather than the more traditional thresholding functions used in many other neural nets). Figure 1 shows a portion of such a neural network (the polynomials are not accurately graphed).
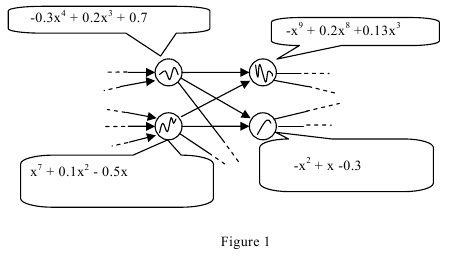
When Rose was ready to select her polynomials, she discovered a problem. Due to the limited amount of memory available, she did not have enough space to store all of the coefficients of the polynomials in her network. She has decided to use an approximation to each polynomial in the form of a continuous polygonal curve with two segments, y = a1x + a0 and y = b1x + b0 . The segments meet at a point whose x -coordinate, c , is between -1 and +1. Rose wants the approximation to be the best in the sense that the distance between p and the approximation function g is minimal. We define the distance between p and g as the integral of the square of their difference:

For instance, if the polynomial is x2 - 0.2 , then the best polygonal approximation, with lines meeting at c= 0 , is shown in Figure 2 (the dotted line shows the graph of the polygonal approximation).

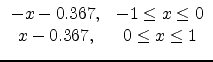
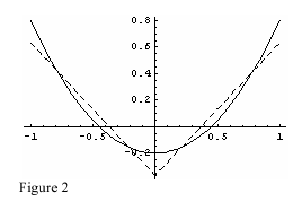
In the few bytes that are available for each node, Rose can store the values of a1 , a0 , b1 , b0 , and c as signed numbers. Fortunately Rose has a program that supplies her with a good guess for the value of c . Given this value, you are asked to help Rose find the optimal values for a1 , a0 , b1 , and b0 in the approximations to her polynomials.
Input
The input file contains multiple test cases. Each test case consists of three lines. The first line contains a positive integer n , 1n
10 , representing the degree of the polynomial p(x) . This is followed by a line containing n + 1 numbers between -1 and 1 inclusive, which are the coefficients of p(x) from highest order term down to the constant term, expressed with at most three places after the decimal point. The last line for each test case contains the value for c , -1 < c < 1 , expressed with at most three places after the decimal point.
A line containing the integer zero follows the last test case.
Output
For each test case, print the case number (beginning with 1) and the four optimal values, displaying each with exactly three places after the decimal point. The first and second values are the parameters a1 and a0of the line segment y = a1x + a0 defining g in the range -1x
c . The third and fourth values are the parameters b1 and b0 of the line segment y = b1x + b0 defining g in the range c
x
1 . The distance d (p,g) between p and g (as defined earlier) should be the minimum over all such choices for a1 , a0 , b1 , andb0 .
Sample Input
2 1.0 0.0 -0.2 0.0 3 1 0 -1 0 0.707 0
Sample Output
Case 1: -1.000 -0.367 1.000 -0.367 Case 2: -0.499 -0.036 1.198 -1.236
#include<stdio.h>
#include<stdlib.h>
#include<string.h>
#include <iostream>
#include <iomanip>
using namespace std;
double a[11],c,u;
int n,i,t;
double f(double k)
{
double b[22],r[11],s,x,y;
int i,j;
memcpy(r,a,sizeof(r));
r[1]-=k;
r[0]-=-k*c+u;
memset(b,0,sizeof(b));
for(i=0;i<11;i++)
for(j=0;j<11;j++)
b[i+j]+=r[i]*r[j];
for(i=21;i;i--)
b[i]=b[i-1]/i;
s=0;
x=1;
y=1;
for(i=1;i<22;i++)
{
x*=c;
s+=b[i]*x;
y=-y;
s-=b[i]*y;
}
return s;
}
double g(double k)
{
double b[22],r[11],s,x;
int i,j;
memcpy(r,a,sizeof(r));
r[1]-=k;
r[0]-=-k*c+u;
memset(b,0,sizeof(b));
for(i=0;i<11;i++)
for(j=0;j<11;j++)
b[i+j]+=r[i]*r[j];
for(i=21;i;i--)
b[i]=b[i-1]/i;
s=0;
x=1;
for(i=1;i<22;i++)
{
x*=c;
s+=b[i]*(1-x);
}
return s;
}
double h(double v)
{
double p0,p1,p2,v0,v1,mf,mg;
u=v;
p0=f(-0.5);
p1=f(0.5);
p2=f(1.5);
v0=p1-p0;
v1=p2-p1;
mf=f(v0/(v0-v1));
p0=g(-0.5);
p1=g(0.5);
p2=g(1.5);
v0=p1-p0;
v1=p2-p1;
mg=g(v0/(v0-v1));
return mf+mg;
}
void work()
{
double p0,p1,p2,v0,v1,bk;
printf("Case %d: ",++t);
p0=h(-0.5);
p1=h(0.5);
p2=h(1.5);
v0=p1-p0;
v1=p2-p1;
u=v0/(v0-v1);
p0=f(-0.5);
p1=f(0.5);
p2=f(1.5);
v0=p1-p0;
v1=p2-p1;
bk=v0/(v0-v1);
printf("%.3lf %.3lf",bk,-bk*c+u);
p0=g(-0.5);
p1=g(0.5);
p2=g(1.5);
v0=p1-p0;
v1=p2-p1;
bk=v0/(v0-v1);
printf(" %.3lf %.3lf\n",bk,-bk*c+u);
}
int main()
{
while(scanf("%d",&n)&&n)
{
memset(a,0,sizeof(a));
for(i=n;i>=0;i--)
scanf("%lf",&a[i]);
scanf("%lf",&c);
work();
}
return 0;
}