You are a technician for the Air Conditioning Machinery company (ACM). Unfortunately, when you arrive at a customer site to install some air conditioning ducts, you discover that you are running low on supplies. You have only six duct segments, and they are all of the same kind, called an ``elbow.''
You must install a duct in a confined space: a rectangular prism whose sides are multiples of a unit length. Think of the confined space as consisting of an array of unit cubes. Each elbow occupies exactly four unit cubes, as shown in Figure 1 below. A unit cube can be occupied by at most one elbow. Each elbow has exactly two openings, as indicated by the gray squares in the elbow shown in Figure 1. You may assemble the elbows into longer ducts, but your duct must be completely contained inside the given space. One way to connect two elbows is shown in Figure 2. Your task is to connect an inflow to an outflow. The inflow and the outflow are located on the exterior surface of the confined space, aligned with the unit cubes, as shown in Figure 3. To keep expenses down, you must accomplish this task while using the minimum number of elbows. out
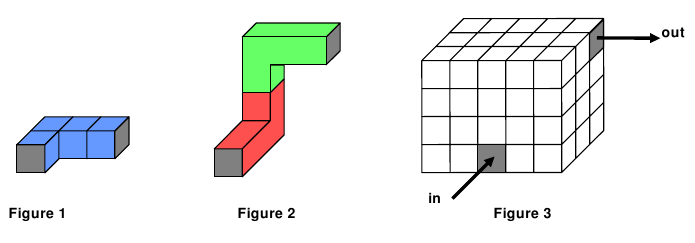
Input
The input consists of several test cases, each of which consists of a single line containing eleven input values separated by blanks. The input values for each test case are as follows.
The first three input values are integers (xmax , ymax , and zmax ) that indicate the size of the confined space in the x , y , and z dimensions, respectively. Each unit cube in the confined space can be identified by coordinates (x, y, z) where 1x
xmax , 1
y
ymax , and 1
z
zmax . xmax , ymax , and zmax are all positive and not greater than 20.
The next three input values are integers that indicate the location of the inflow by identifying the x , y , and z coordinates of the unit cube that connects to the inflow.
The next input value is a two-character string that indicates the direction of the inward flow, using one of the following codes: + x, - x, + y, - y, + z, - z . The inflow connection is on the face of the unit cube that receives this inward flow. For example, if the data specifies an inflow direction of + y , the inflow connection is on the face of the unit cube that faces in the negative y direction.
The next three input values are integers that indicate the location of the outflow by identifying the x , y, and z coordinates of the unit cube that connects to the outflow.
The last input value is a two-character string that indicates the direction of the outward flow, using the same codes described above. The outflow connection is on the face of the unit cube that generates this outward flow. For example, if the data specifies an outflow direction of + y , the outflow connection is on the face of the unit cube that faces in the positive y direction.
The last line of the input file consists of a single zero to indicate end of input.
Output
For each test case, print the case number (starting with 1) followed by the minimum number of elbows that are required to connect the inflow to the outflow without going outside the confined space. If the task cannot be accomplished with your supply of six elbow segments, print the word `Impossible' instead. Use the format in the sample data.
Sample Input
5 4 3 3 1 1 +z 5 4 3 +x 5 4 3 3 1 1 +z 1 2 3 -x 0
Sample Output
Case 1: 2 Case 2: Impossible
#include<stdio.h>
#include<string.h>
#include<stdlib.h>
const int Direction[6][3]={1,0,0, -1,0,0, 0,1,0, 0,-1,0, 0,0,1, 0,0,-1};
struct node
{
int x,y,z,face;
};
const int xMax=20;
const int yMax=20;
const int zMax=20;
bool find;
char ch_axis,ch_direction;
int Available[xMax+5][yMax+5][zMax+5];
int i,j,k;
int ans;
int cases;
node inflow,outflow,Max;
int add(node Try)
{
if(Try.x<1||Try.y<1||Try.z<1||Try.x>Max.x||Try.y>Max.y||Try.z>Max.z)
return 1;
Available[Try.x][Try.y][Try.z]++;
if(Available[Try.x][Try.y][Try.z]>1)
return 1;
else
return 0;
}
void del(node Try)
{
if(Try.x<1||Try.y<1||Try.z<1||Try.x>Max.x||Try.y>Max.y||Try.z>Max.z)
return;
Available[Try.x][Try.y][Try.z]--;
}
void search(node now,int deep)
{
int i;
node n1,n2,n3,n4;
if((find && deep==ans) || (!find && deep>ans))
return;
if(now.x==outflow.x && now.y==outflow.y &&now.z==outflow.z &&now.face==outflow.face)
{
find=true;
ans=deep;
return;
}
n1.x=now.x + Direction[now.face][0];n1.y=now.y + Direction[now.face][1];n1.z=now.z + Direction[now.face][2];
n2.x=n1.x + Direction[now.face][0];n2.y=n1.y + Direction[now.face][1];n2.z=n1.z + Direction[now.face][2];
for(i=0;i<6;i++)
if(i/2!=now.face/2)
{
n3.x=n2.x+Direction[i][0];n3.y=n2.y+Direction[i][1];n3.z=n2.z+Direction[i][2];
n4.x=n3.x+Direction[i][0];n4.y=n3.y+Direction[i][1];n4.z=n3.z+Direction[i][2];
n4.face=i;
if(add(n1)+add(n2)+add(n3)+add(n4)==0)
search(n4,deep+1);
del(n1);del(n2);del(n3);del(n4);
}
n1.x=now.x + Direction[now.face][0];n1.y=now.y + Direction[now.face][1];n1.z=now.z + Direction[now.face][2];
n2.x=n1.x + Direction[now.face][0];n2.y=n1.y + Direction[now.face][1];n2.z=n1.z + Direction[now.face][2];
n3.x=n2.x + Direction[now.face][0];n3.y=n2.y + Direction[now.face][1];n3.z=n2.z + Direction[now.face][2];
for(i=0;i<6;i++)
if(i/2!=now.face/2)
{
n4.x=n3.x+Direction[i][0];n4.y=n3.y+Direction[i][1];n4.z=n3.z+Direction[i][2];
n4.face=i;
if(add(n1)+add(n2)+add(n3)+add(n4)==0)
search(n4,deep+1);
del(n1);del(n2);del(n3);del(n4);
}
}
bool init()
{
scanf("%d",&Max.x);
if(Max.x==0)
return false;
scanf("%d %d",&Max.y,&Max.z);
scanf("%d %d %d ",&inflow.x,&inflow.y,&inflow.z);
scanf("%c%c",&ch_direction,&ch_axis);
if(ch_axis=='x')
inflow.face=0;
else if(ch_axis=='y')
inflow.face=2;
else
inflow.face=4;
if(ch_direction=='-')
inflow.face++;
scanf("%d %d %d ",&outflow.x,&outflow.y,&outflow.z);
scanf("%c%c",&ch_direction,&ch_axis);
if(ch_axis=='x')
outflow.face=0;
else if(ch_axis=='y')
outflow.face=2;
else
outflow.face=4;
if(ch_direction=='-')
outflow.face++;
inflow.x -= Direction[inflow.face][0];
inflow.y -= Direction[inflow.face][1];
inflow.z -= Direction[inflow.face][2];
return true;
}
int main()
{
cases=0;
while(init())
{
memset(Available,0,sizeof(Available));
ans=6;
find=false;
search(inflow,0);
printf("Case %d: ",++cases);
if(find)
printf("%d\n",ans);
else
printf("Impossible\n");
}
return 0;
}