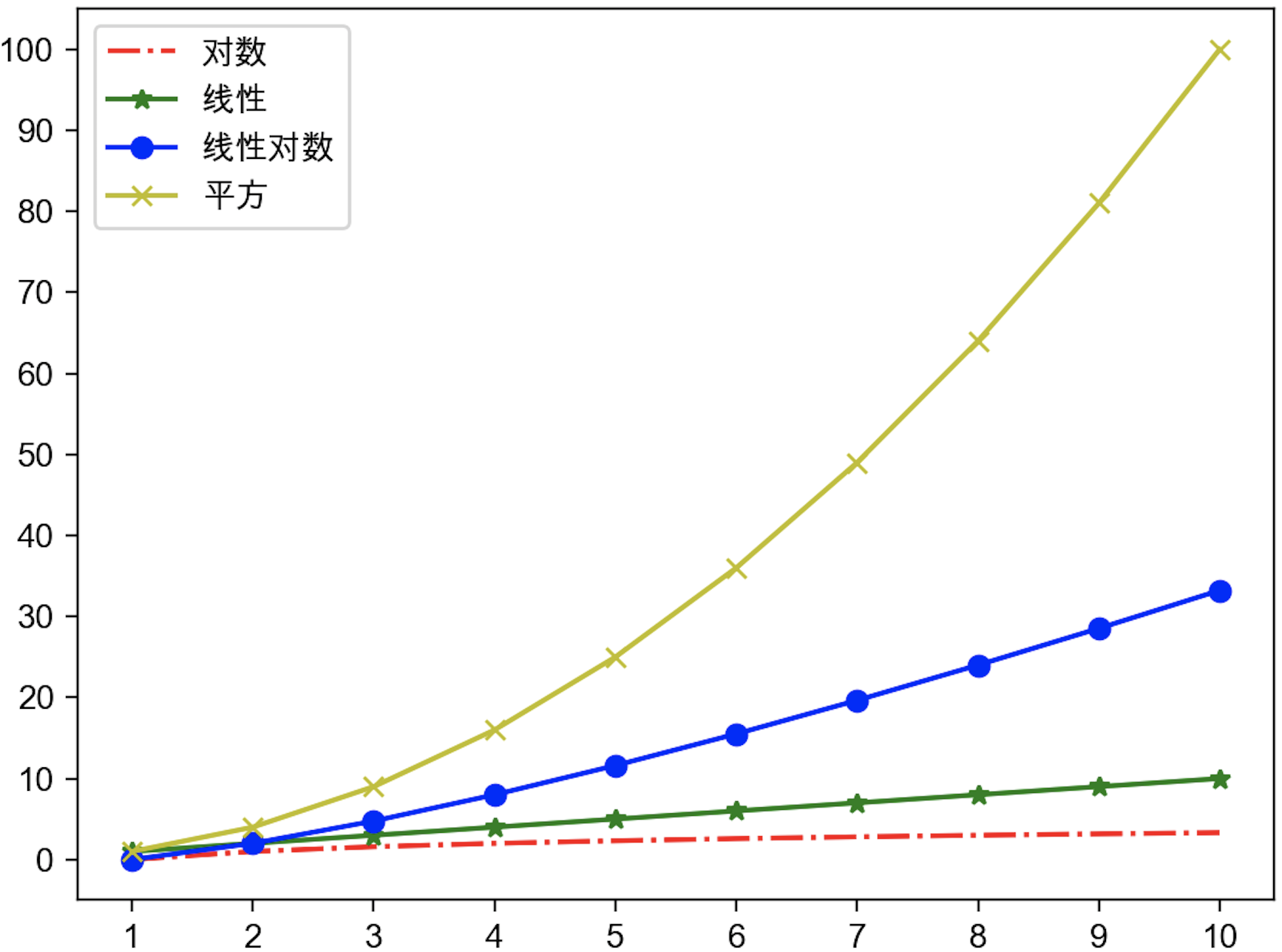
前言
- O ( c ) O(c) O(c):常量时间复杂度 - 哈希存储 / 布隆过滤器
- O ( l o g 2 n ) O(log_{2}n) O(log2n):对数时间复杂度 - 折半查找
- O ( n ) O(n) O(n):线性时间复杂度 - 顺序查找
- O ( n ∗ l o g 2 n ) O(n*log_{2}n) O(n∗log2n):对数线性时间复杂度 - 高级排序算法(归并排序、快速排序)
- O ( n 2 ) O(n^2) O(n2):平方时间复杂度 - 简单排序算法(冒泡排序、选择排序、插入排序)
- O ( n 3 ) O(n^3) O(n3):立方时间复杂度 - Floyd 算法 / 矩阵乘法运算
- O ( 2 n ) O(2^n) O(2n):几何级数时间复杂度 - 汉诺塔
- O ( 3 n ) O(3^{n}) O(3n):几何级数时间复杂度,也称为指数时间复杂度
- O ( n ! ) O(n!) O(n!):阶乘时间复杂度 - 旅行经销商问题 NP
一、希尔排序
希尔排序也称为缩小增量排序,选择步长是该算法重要的一步。
def shellsort(lists):
length = len(lists)
pace = length // 2
while pace >= 1:
for i in range(pace, length):
while i-pace >= 0:
if lists[i] < lists[i - pace]:
lists[i], lists[i - pace] = lists[i - pace], lists[i]
i = i - pace # 若换位一次说明前面还可以有可换数值,若不可换说明没有可换数值
pace = pace // 2
return lists
二、归并排序
采用分治策略,来降低算法的复杂度
import random
def bubble_sort(list, start, end):
length = end - start + 1
for i in range(0, length-1):
for j in range(start, end-i):
if list[j] < list[j+1]:
list[j], list[j+1] = list[j+1], list[j]
def combine_sort(list):
width = 2
w = width
time = 1
length = len(list)
while w*2 < length:
w *= 2
time += 1
for i in range(0, time):
remainder = length % width
w_time = length // width
for i in range(1, w_time+1):
bubble_sort(list, width*(i-1), width*i-1)
if remainder != 0:
bubble_sort(list, length-remainder, length-1)
width *= 2
bubble_sort(list, 0, length-1)
return list
三、堆排序
import random
def heapinit(heap):
length = len(heap)
for i in range(1, length//2, 1):
for j in range(length, i, -1):
if heap[j-1] > heap[j//2-1]:
heap[j-1], heap[j//2-1] = heap[j//2-1], heap[j-1]
printheap(heap)
return heap
def bubbleup(heap, end):
unfinish = 1
stone = 0
while unfinish:
if stone * 2 + 2 <= end:
if heap[stone*2+1] >= heap[stone*2+2] and heap[stone*2+1] > heap[stone]:
heap[stone*2+1], heap[stone] = heap[stone], heap[stone*2+1]
stone = stone*2+1
elif heap[stone*2+1] < heap[stone*2+2] and heap[stone*2+2] > heap[stone]:
heap[stone*2+2], heap[stone] = heap[stone], heap[stone*2+2]
stone = stone*2+2
else:
unfinish = 0
elif stone*2+1 <= end:
if heap[stone] < heap[stone*2+1]:
heap[stone], heap[stone*2+1] = heap[stone*2+1], heap[stone]
unfinish = 0
else:
unfinish = 0
def heapsort(heap):
heapinit(heap)
length = len(heap)
heap[length-1], heap[0] = heap[0], heap[length-1]
for end in range(length-2, 0, -1):
bubbleup(heap, end)
heap[end], heap[0] = heap[0], heap[end]
return heap
def printheap(heap):
length = len(heap)
i = 0
n = 1
print('heap')
while i <= length-1:
if i+n <= length-1:
print(heap[i: i+n])
i += n
n *= 2
else:
print(heap[i: length])
i = length
五、快速排序
def quicksort(list, left, right):
if left >= right:
return list
key = list[left]
# 将左边第一位定位基准数,以此数将序列分为两部分
low = left
high = right
while left != right:
# 从最右边开始查,查找比基准值小的数
while left < right and list[right] >= key:
right -= 1
list[left] = list[right]
# 从最左边开始查,查找比基准值大的数
while left < right and list[left] <= key:
left += 1
list[right] = list[left]
list[right] = key
# 分别对两部分数据再调用quick_sort函数
quick_sort(list,low,left-1)
quick_sort(list,left+1,high)
return list