一、自定义注解类
import java.lang.annotation.*;
@Documented
@Retention(RetentionPolicy.RUNTIME)
@Target({ElementType.METHOD})
public @interface TestAnnotation {
String value() default "";
}
二、自定义拦截(springaop)
import com.example.springbootdemo.anno.TestAnnotation; import lombok.extern.slf4j.Slf4j; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.Signature; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; import org.aspectj.lang.reflect.MethodSignature; import org.springframework.stereotype.Component; import java.lang.reflect.Method; @Aspect @Component @Slf4j public class MyInterceptor { @Pointcut("@annotation( com.example.springbootdemo.anno.TestAnnotation)") public void myPointCut() { } /** * 方法拦截 * * @param joinPoint 拦截点 * @return 执行结果 * @throws Throwable 异常 */
@Around("myPointCut()") public Object doInterceptor(ProceedingJoinPoint joinPoint) { Signature signature = joinPoint.getSignature(); MethodSignature methodSignature = (MethodSignature)signature; Method method = methodSignature.getMethod(); TestAnnotation annotation = method.getAnnotation(TestAnnotation.class); String key = annotation.value(); System.out.println("====Interceptor===="); System.out.println("通知之开始打印key值"+key); Object retmsg = null; try { retmsg = joinPoint.proceed(); System.err.println("++++++++ 最后执行,执行完后结束 -->" + retmsg); } catch (Throwable e) { e.printStackTrace(); } System.out.println("通知之结束 +retmsg -->" + retmsg); return retmsg; } }
三、执行结果
四、同理springboot+redission+自定义注解实现分布式锁(直接上代码)
import java.lang.annotation.*; @Documented @Retention(RetentionPolicy.RUNTIME) @Target({ElementType.METHOD}) public @interface RedisLockAnnotation { String lockKey(); }
五、自定义redis锁
import com.alibaba.fastjson.JSONObject; import com.example.springbootdemo.anno.RedisLockAnnotation; import lombok.extern.slf4j.Slf4j; import org.aspectj.lang.ProceedingJoinPoint; import org.aspectj.lang.Signature; import org.aspectj.lang.annotation.Around; import org.aspectj.lang.annotation.Aspect; import org.aspectj.lang.annotation.Pointcut; import org.aspectj.lang.reflect.MethodSignature; import org.redisson.api.RLock; import org.redisson.api.RedissonClient; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; import java.lang.reflect.Method; @Aspect @Component @Slf4j public class RedisLockAspect { @Autowired private RedissonClient redissonClient; /** * @annotation 中的路径表示拦截特定注解 */ @Pointcut("@annotation(com.example.springbootdemo.anno.RedisLockAnnotation)") public void redisLockPc() { } @Around(value = "redisLockPc()") public Object around(ProceedingJoinPoint joinPoint){ Signature signature = joinPoint.getSignature(); MethodSignature methodSignature = (MethodSignature)signature; Method method = methodSignature.getMethod(); RedisLockAnnotation annotation = method.getAnnotation(RedisLockAnnotation.class); String key = annotation.lockKey(); //加上方法名好区分 String finalKey = method.getName()+":"+key; log.info("finalkey="+finalKey+" 开始上锁,参数为+"+ JSONObject.toJSONString(key)); RLock lock = redissonClient.getLock(finalKey); Object result =null; try { boolean tryLock = lock.tryLock(); if(tryLock){ System.out.println("抢锁成功"); //获取分布式锁成功之后,正式运行方法 result = joinPoint.proceed(); }else { System.out.println("抢锁失败"); throw new Exception("抢锁失败"); } } catch (Throwable throwable) { throwable.printStackTrace(); } finally { //最后不要忘记手动释放锁,不要等着到expire time,不然会阻塞后面的方法运行 if(lock.isLocked()&&lock.isHeldByCurrentThread()){ lock.unlock(); } } return result; } }
六、测试
import com.example.springbootdemo.anno.RedisLockAnnotation; import lombok.extern.slf4j.Slf4j; import org.springframework.scheduling.annotation.Scheduled; import org.springframework.stereotype.Component; @Slf4j @Component public class TestJob { @Scheduled(cron = "0/3 * * * * ?") @RedisLockAnnotation(lockKey = "syncStatuteOfLimitationsTob") public void syncStatuteOfLimitationsTob() throws InterruptedException { log.info("----------1定时任务开始-----------"); log.info("----------1定时任务结束-----------"); } @Scheduled(cron = "0/3 * * * * ?") @RedisLockAnnotation(lockKey = "syncStatuteOfLimitationsTob") public void syncStatuteOfLimitationsTob1() throws InterruptedException { log.info("----------2定时任务开始-----------"); log.info("----------2定时任务结束-----------"); } }
七、测试结果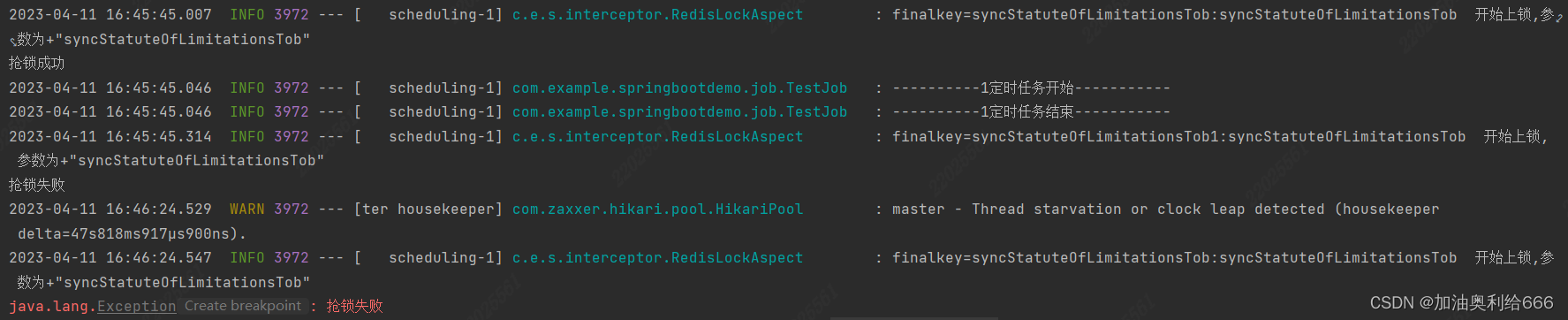
结果生效