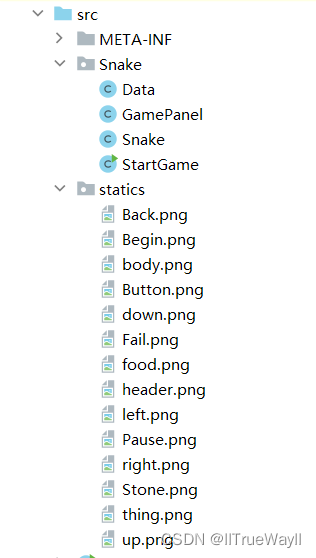
Data
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by FernFlower decompiler)
//
package Snake;
import java.net.URL;
import javax.swing.ImageIcon;
public class Data {
public static URL headerURL = Data.class.getResource("/statics/header.png");
public static ImageIcon header;
public static URL upURL;
public static URL downURL;
public static URL leftURL;
public static URL rightURL;
public static ImageIcon up;
public static ImageIcon down;
public static ImageIcon left;
public static ImageIcon right;
public static URL bodyURL;
public static ImageIcon body;
public static URL FoodURL;
public static ImageIcon Food;
public static ImageIcon thing;
public static URL thingURL;
public static ImageIcon Stone;
public static URL StoneURL;
public static ImageIcon Back;
public static URL BackURL;
public static ImageIcon Button;
public static URL ButtonURL;
public static ImageIcon Fail;
public static URL FailURL;
public static ImageIcon Begin;
public static URL BeginURL;
public static ImageIcon Pause;
public static URL PauseURL;
public Data() {
}
static {
header = new ImageIcon(headerURL);
upURL = Data.class.getResource("/statics/up.png");
downURL = Data.class.getResource("/statics/down.png");
leftURL = Data.class.getResource("/statics/left.png");
rightURL = Data.class.getResource("/statics/right.png");
up = new ImageIcon(upURL);
down = new ImageIcon(downURL);
left = new ImageIcon(leftURL);
right = new ImageIcon(rightURL);
bodyURL = Data.class.getResource("/statics/body.png");
body = new ImageIcon(bodyURL);
FoodURL = Data.class.getResource("/statics/Food.png");
Food = new ImageIcon(FoodURL);
thingURL = Data.class.getResource("/statics/thing.png");
thing = new ImageIcon(thingURL);
StoneURL = Data.class.getResource("/statics/Stone.png");
Stone = new ImageIcon(StoneURL);
BackURL = Data.class.getResource("/statics/Back.png");
Back = new ImageIcon(BackURL);
ButtonURL = Data.class.getResource("/statics/Button.png");
Button = new ImageIcon(ButtonURL);
FailURL = Data.class.getResource("/statics/Fail.png");
Fail = new ImageIcon(FailURL);
BeginURL = Data.class.getResource("/statics/Begin.png");
Begin = new ImageIcon(BeginURL);
PauseURL = Data.class.getResource("/statics/Pause.png");
Pause = new ImageIcon(PauseURL);
}
}
GamePanel
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by FernFlower decompiler)
//
package Snake;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.Random;
import javax.swing.*;
public class GamePanel extends JPanel implements KeyListener, ActionListener {
boolean isStart = false;
boolean isFail = false;
boolean isPause= false;
int score = 0;
int snake_Speed = 200;
Snake snake = new Snake();
Timer timer = new Timer(snake_Speed, this);
int Foodx;
int Foody;
int Thingx;
int Thingy;
int Stoney;
int Stonex;
int n = 0;
int level = 0;
Random random = new Random();
public GamePanel() {
this.setFocusable(true);
this.addKeyListener(this);
this.timer.start();
this.createFood();
this.createThing();
this.createStone();
}
protected void paintComponent(Graphics g) {
super.paintComponent(g);
this.setBackground(Color.cyan);
g.setColor(Color.darkGray);
Data.Button.paintIcon(this, g, 0, 0);
Data.header.paintIcon(this, g, 25, 11);
g.fillRect(25, 75, 850, 600);
Data.Back.paintIcon(this, g, 25, 75);
Data.Food.paintIcon(this, g, this.Foodx, this.Foody);
Data.thing.paintIcon(this, g, this.Thingx, this.Thingy);
Data.Stone.paintIcon(this, g, this.Stonex, this.Stoney);
g.setColor(Color.BLACK);
g.setFont(new Font("楷体", 1, 25));
g.drawString("贪吃蛇小游戏", 300, 45);
g.setFont(new Font("隶书", 1, 18));
g.drawString("长度" + this.snake.length, 750, 35);
g.drawString("分数" + this.score, 750, 55);
g.drawString("难度:" + level, 550, 55);
g.drawString("速度(MAX 100):" + (100 - snake_Speed / 2), 550, 35);
switch (this.snake.fx) {
case "W":
Data.up.paintIcon(this, g, this.snake.snakex[0], this.snake.snakey[0]);
break;
case "A":
Data.left.paintIcon(this, g, this.snake.snakex[0], this.snake.snakey[0]);
break;
case "S":
Data.down.paintIcon(this, g, this.snake.snakex[0], this.snake.snakey[0]);
break;
case "D":
Data.right.paintIcon(this, g, this.snake.snakex[0], this.snake.snakey[0]);
}
for (int i = 1; i < this.snake.length; ++i) {
Data.body.paintIcon(this, g, this.snake.snakex[i], this.snake.snakey[i]);
}
if (!this.isStart) {
if(this.isPause){
Data.Pause.paintIcon(this,g,237,240);
g.setColor(Color.WHITE);
g.setFont(new Font("楷体", 1, 40));
g.drawString("暂停游戏,点击空格继续", 260, 320);
}
if(!this.isPause) {
Data.Begin.paintIcon(this,g,0,0);
g.setColor(Color.WHITE);
g.setFont(new Font("楷体", 1, 60));
g.drawString("贪吃蛇小游戏", 220, 300);
g.drawString("点击空格开始游戏", 170, 500);
}
}
if (this.isFail) {
Data.Fail.paintIcon(this,g,25,200);
g.setColor(Color.black);
g.setFont(new Font("隶书", 1, 60));
g.drawString("你失败了 ", 340, 465);
}
}
public void keyTyped(KeyEvent e) {
}
public void keyPressed(KeyEvent e) {
int keyCode = e.getKeyCode();
if (keyCode == 32 ) {
if (this.isFail) {
this.isFail = false;
this.snake.init();
this.createFood();
this.createThing();
this.createStone();
this.score = 0;
this.level = 0;
this.snake_Speed = 200;
this.n = 0;
}
if (!this.isPause) {
this.isPause = !this.isPause;
}
else{
this.isStart = !this.isStart;
}
this.repaint();
}
switch (keyCode) {
case 65:
this.snake.fx = "A";
break;
case 68:
this.snake.fx = "D";
break;
case 83:
this.snake.fx = "S";
break;
case 87:
this.snake.fx = "W";
}
if (keyCode == 67){
}
}
public void keyReleased(KeyEvent e) {
}
public void actionPerformed(ActionEvent e) {
if (this.isStart && !this.isFail && this.isPause ){
int i;
for (i = this.snake.length - 1; i > 0; --i) {
this.snake.snakex[i] = this.snake.snakex[i - 1];
this.snake.snakey[i] = this.snake.snakey[i - 1];
}
switch (this.snake.fx) {
case "D":
this.snake.snakex[0] += 25;
if (this.snake.snakex[0] > 850) {
this.snake.snakex[0] = 25;
}
break;
case "A":
this.snake.snakex[0] -= 25;
if (this.snake.snakex[0] < 25) {
this.snake.snakex[0] = 850;
}
break;
case "W":
this.snake.snakey[0] -= 25;
if (this.snake.snakey[0] < 75) {
this.snake.snakey[0] = 650;
}
break;
case "S":
this.snake.snakey[0] += 25;
if (this.snake.snakey[0] > 650) {
this.snake.snakey[0] = 75;
}
}
for (i = 1; i < this.snake.length; ++i) {
if (this.snake.snakex[0] == this.snake.snakex[i] && this.snake.snakey[0] == this.snake.snakey[i]) {
this.isFail = true;
}
}
if (this.snake.snakex[0] == this.Foodx && this.snake.snakey[0] == this.Foody) {
++this.snake.length;
this.score = this.addCarry(this.score, 10);
this.createFood();
this.createThing();
this.createStone();
}
if (this.snake.snakex[0] == this.Thingx && this.snake.snakey[0] == this.Thingy
|| this.snake.snakex[0] == this.Stonex && this.snake.snakey[0] == this.Stoney) {
this.isFail = true;
}
if (snake.length >= (1 + 10 * n)) {
snake_Speed = snake_Speed - 20;
level++;
n++;
}
this.repaint();
}
this.timer.start();
}
public void createFood() {
int foodx ;
int foody ;
foodx= 25 + 25 * this.random.nextInt(34);
foody= 75 + 25 * this.random.nextInt(23);
this.Foodx = foodx;
this.Foody = foody;
}
public void createThing() {
int thingx ;
int thingy ;
thingx= 25 + 25 * this.random.nextInt(34);
thingy= 75 + 25 * this.random.nextInt(23);
this.Thingx = thingx;
this.Thingy = thingy;
}
public void createStone() {
int stonex ;
int stoney ;
stonex= 25 + 25 * this.random.nextInt(34);
stoney= 75 + 25 * this.random.nextInt(23);
this.Stonex = stonex;
this.Stoney = stoney;
}
int addCarry(int a, int b) {
int sum = a ^ b;
int carry = (a & b) << 1;
int result;
if (carry == 0) {
result = sum;
} else {
result = this.addCarry(sum, carry);
}
return result;
}
}
Snake
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by FernFlower decompiler)
//
package Snake;
public class Snake {
int length;
int[] snakex = new int[600];
int[] snakey = new int[500];
String fx;
public void init() {
this.length = 1;
this.snakex[0] = 100;
this.snakey[0] = 100;
this.snakex[1] = 75;
this.snakey[1] = 100;
this.snakex[2] = 50;
this.snakey[2] = 100;
this.fx = "D";
}
public Snake() {
this.init();
}
}
StartGame
//
// Source code recreated from a .class file by IntelliJ IDEA
// (powered by FernFlower decompiler)
//
package Snake;
import javax.swing.*;
public class StartGame {
public StartGame() {
}
public static void main(String[] args) {
JFrame jFrame = new JFrame("贪吃蛇"); //界面标题
jFrame.setSize(915, 735);//设置宽高
jFrame.setResizable(false);
GamePanel gamePanel = new GamePanel();
jFrame.add(gamePanel);
jFrame.setVisible(true);
jFrame.setDefaultCloseOperation(3);//退出
jFrame.setLocationRelativeTo(null);//布局正中间
}
}