安卓开发--页面左右滑动
本次博客演示三个页面之间的滑动切换,利用ViewPager2实现,最终的文件目录结构如下:
app/
├── src/
│ ├── main/
│ │ ├── java/
│ │ │ └── com/
│ │ │ └── example/
│ │ │ └── myapp/
│ │ │ ├── HomeActivity.java
│ │ │ └── fragments/
│ │ │ ├── ViewPagerAdapter.java
│ │ │ ├── WebViewFragment.java
│ │ │ ├── LayoutFragment.java
│ │ │ └── OtherFragment.java
│ │ ├── res/
│ │ │ ├── layout/
│ │ │ │ ├── activity_home.xml
│ │ │ │ ├── fragment_webview.xml
│ │ │ │ ├── fragment_layout.xml
│ │ │ │ └── fragment_other.xml
│ │ │ └── ...
│ │ └── AndroidManifest.xml
├── ...
1.在布局文件中定义ViewPager2
这是一个主布局文件res/layout/activity_home.xml
,我们布局一个viewpager2
,代码如下:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<androidx.viewpager2.widget.ViewPager2
android:id="@+id/view_pager"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</LinearLayout>
2.三个子切换页面的布局代码以及效果
文件一 res/layout/fragment_layout.xml
,代码如下,显示的是一个折线图:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<WebView
android:id="@+id/webview"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
</FrameLayout>
文件二 res/layout/fragment_layout.xml
,代码如下,显示的是一个文本框:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<!-- 其他布局内容 -->
<TextView
android:layout_width="match_parent"
android:layout_height="match_parent"
android:text="这是一个其他布局"
android:textSize="50dp"/>
</FrameLayout>
文件三res/layout/fragment_other.xml
,代码如下,显示的是一个GIF动图:
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal">
<ImageView
android:id="@+id/gif_image_view"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:scaleType="centerCrop"/>
</FrameLayout>
其各自效果如图:
文件1 | 文件2 | 文件3 |
---|---|---|
![]() |
![]() |
![]() |
3.三个切换页面的活动文件代码
文件一 java/com/example/ecgphone/fragments/WebViewFragment.java
,代码如下,显示的是一个折线图:
package com.example.ecgphone.fragments;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.webkit.WebSettings;
import android.webkit.WebView;
import android.webkit.WebViewClient;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import com.example.ecgphone.R;
import org.json.JSONArray;
import java.util.Arrays;
public class WebViewFragment extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_webview, container, false);
WebView webview = view.findViewById(R.id.webview);
webview.getSettings().setJavaScriptEnabled(true);
webview.getSettings().setAllowFileAccess(true);
// 确保WebView内容完全加载后再执行JS
webview.setWebViewClient(new WebViewClient() {
// setWebViewClient用于确保在页面加载完成之后再调用JavaScript函数
// WebViewClient类有几个常用的方法,其中最常用的包括:
// onPageStarted: 在页面开始加载时调用。
// onPageFinished: 在页面加载完成时调用
// shouldOverrideUrlLoading: 当WebView需要处理某个URL时调用。
@Override
public void onPageFinished(WebView view, String url) {
super.onPageFinished(view, url);
// // 将字符串数组转换为JSONArray
// String[] value = {"12:00", "13:00", "14:00", "15:00", "16:00", "17:00", "18:00", "19:00", "20:00", "21:00", "22:00", "23:00", "00:00", "01:00", "02:00", "03:00", "04:00", "05:00", "06:00", "07:00", "08:00", "09:00", "10:00", "11:00", "12:00"};
// JSONArray xValue = new JSONArray(Arrays.asList(value));
// 将int数组转换为JSONArray
double[] yValue1 = { 0.08 , 0.085, 0.07 , 0.085, 0.09 , 0.105, 0.105, 0.09 , 0.09 ,0.105
,0.095, 0.1 , 0.09 , 0.08 , 0.085, 0.065, 0.1 , 0.07 , 0.06 ,0.09
,0.075, 0.06 , 0.095, 0.075, 0.075, 0.065, 0.075, 0.055, 0.055,0.07
,0.07 , 0.055, 0.06 , 0.045, 0.055, 0.05 , 0.09 , 0.145, 0.16 ,0.175
,0.225, 0.255, 0.275, 0.28 , 0.265, 0.255, 0.205, 0.145, 0.12 ,0.075
,0.06 , 0.07 , 0.03 , 0.01 ,-0.02 ,-0.01 , 0. , 0.02 , 0. ,0.
,0.01 , 0. , 0.01 ,-0.005, 0.015,-0.02 , 0.005, 0. , 0.01 ,0.005
,0. ,-0.01 , 0.005, 0.01 ,-0.01 ,-0.005, 0.005,-0.015, 0.005,0.
,0.005, 0.03 , 0.08 , 0.14 , 0.01 ,-0.265,-0.695,-1.325,-1.91 ,2.335
,2.555,-2.565,-2.47 ,-2.31 ,-2.18 ,-2.08 ,-1.94 ,-1.74 ,-1.555,1.44
,1.28 ,-1.13 ,-1.01 ,-0.955,-0.84 ,-0.74 ,-0.63 ,-0.545,-0.435,0.31
,0.175,-0.065, 0.055, 0.18 , 0.27 , 0.34 , 0.36 , 0.375, 0.41 ,0.435
,0.44 , 0.48 , 0.47 , 0.49 , 0.49 , 0.535, 0.52 , 0.545, 0.56 ,0.57
,0.59 , 0.615, 0.645, 0.67 , 0.69 , 0.725, 0.755, 0.77 , 0.8 ,0.81
,0.855, 0.88 , 0.885, 0.91 , 0.935, 0.97 , 0.97 , 0.955, 0.96 ,0.95
,0.94 , 0.89 , 0.845, 0.845, 0.8 , 0.765, 0.685, 0.655, 0.605,0.565
,0.49 , 0.43 , 0.375, 0.335, 0.28 , 0.265, 0.235, 0.19 , 0.19 ,0.18
,0.12 , 0.13 , 0.1 , 0.09 , 0.045, 0.09 , 0.075, 0.08 , 0.065,0.035
,0.02 , 0.05 , 0.035, 0.045, 0.045, 0.025, 0.035, 0.035, 0.04 ,0.005
,0.035, 0.015, 0.02 , 0.015, 0.045, 0.045, 0.055, 0.03 , 0.025,0.035};
JSONArray yValue1Array = new JSONArray();
for (double y : yValue1) {
yValue1Array.put(Double.valueOf(y));
}
// 调用JS函数,要注意需要确保在WebView内容完全加载之后再执行JavaScript代码
webview.loadUrl("javascript:doCreateChart('line'," + yValue1Array.toString() + ");");
}
});
webview.loadUrl("file:///android_asset/echarts.html");
return view;
}
}
文件二 java/com/example/ecgphone/fragments/LayoutFragment.java
,代码如下,显示的是一个文本框:
package com.example.ecgphone.fragments;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import com.example.ecgphone.R;
public class LayoutFragment extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
return inflater.inflate(R.layout.fragment_layout, container, false);
}
}
文件三java/com/example/ecgphone/fragments/OtherFragment.java
,代码如下,显示的是一个GIF动图:
package com.example.ecgphone.fragments;
import android.os.Bundle;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import androidx.fragment.app.Fragment;
import com.bumptech.glide.Glide;
import com.example.ecgphone.R;
public class OtherFragment extends Fragment {
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
View view = inflater.inflate(R.layout.fragment_other, container, false);
ImageView gifImageView = view.findViewById(R.id.gif_image_view);
// 使用Glide加载GIF图片
Glide.with(this)
.asGif()
.load(R.raw.video) // 这里放置你的GIF图片URL
.into(gifImageView);
return view;
}
}
4.设置ViewPager2的Adapter
首先,需要新建java/com/example/ecgphone/fragments/ViewPagerAdapter.java
文件,创建一个Adapter来管理这三个Fragments:
package com.example.ecgphone.fragments;
import androidx.annotation.NonNull;
import androidx.fragment.app.Fragment;
import androidx.fragment.app.FragmentActivity;
import androidx.viewpager2.adapter.FragmentStateAdapter;
public class ViewPagerAdapter extends FragmentStateAdapter {
public ViewPagerAdapter(@NonNull FragmentActivity fragmentActivity) {
super(fragmentActivity);
}
@NonNull
@Override
public Fragment createFragment(int position) {
switch (position) {
case 0:
return new WebViewFragment();
case 1:
return new LayoutFragment();
case 2:
return new OtherFragment();
default:
return new WebViewFragment();
}
}
@Override
public int getItemCount() {
return 3;
}
}
5.使用ViewPager2的Adapter
文件java/com/example/ecgphone/HomeActivity.java
package com.example.ecgphone;
import android.annotation.SuppressLint;
import android.content.pm.ActivityInfo;
import android.os.Bundle;
import android.view.WindowManager;
import androidx.appcompat.app.AppCompatActivity;
import com.example.ecgphone.fragments.ViewPagerAdapter;
import androidx.viewpager2.widget.ViewPager2;
public class HomeActivity extends AppCompatActivity {
@SuppressLint("SetJavaScriptEnabled")
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
// 强制横屏
setRequestedOrientation(ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE);
setContentView(R.layout.activity_home);
// 将顶部状态栏设置为透明,关闭顶部状态栏的高度
getWindow().addFlags(WindowManager.LayoutParams.FLAG_TRANSLUCENT_STATUS);
ViewPager2 viewPager = findViewById(R.id.view_pager);
ViewPagerAdapter adapter = new ViewPagerAdapter(this);
viewPager.setAdapter(adapter);
}
}
6.最终效果
可以看到左右滑动,页面可以直接切换。
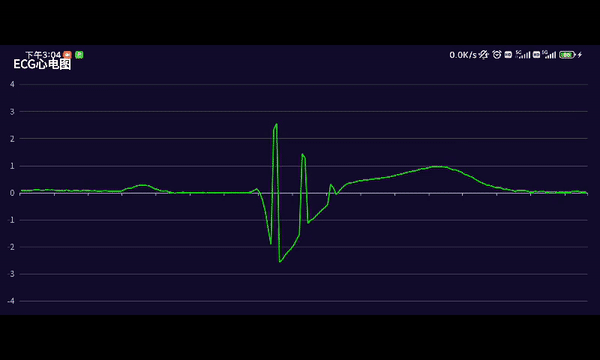