Touchable*系列组件封装了触摸点击的相关事件,比如:触摸、点击、长按、反馈等,官方提供Touchable*系列组件包括四种:
- TouchableHighlight
- TouchableNativeFeedback
- TouchableOpacity
- TouchableWithoutFeedback
其中,TouchableWithoutFeedback的触摸点击不带反馈效果,其他三个都有反馈效果。
TouchableWithoutFeedback
官方建议不使用该组件,因为任何可以响应事件的组件在触摸or点击的时候应该有视觉上的反应效果,但是这个组件没有。这个组件看起来像Web效果而不是原生(Native)效果。
**注意:**TouchableWithFeedback只支持一个子节点,如果你需要设置多个子视图组件,那么就需要使用View节点进行包装。
属性方法
- accessibilityComponentType --View.AccessibilityComponentType 设置可访问的组件类型
- accessibilityTraits -- View.AccessibilityTraits,[View.AccessibilityTraits] 设置访问特征
- accessible -- bool 设置当前组件是否可以访问
- delayLongPress -- number 设置延迟的时间,单位为毫秒。从onPressIn方法开始,到onLongPress被调用这一段时间
- delayPressIn -- number 设置延迟的时间,单位为毫秒,从用户触摸控件开始到onPressIn被调用这一段时间
- delayPressOut -- number 设置延迟的时间,单位为毫秒,从用户触摸事件释放开始到onPressOut被调用这一段时间
- onLayout -- function 当组件加载或者改组件的布局发生变化的时候调用。调用传入的参数为{nativeEvent:{layout:{x,y,width,height}}}
- onLongPress -- function 方法,当用户长时间按压组件(长按效果)的时候调用该方法
- onPress -- function 方法 当用户点击的时候调用(触摸结束)。 但是如果事件被取消了就不会调用。(例如:当前被滑动事件所替代)
- onPressIn -- function 用户开始触摸组件回调方法
- onPressOut -- function 用户完成触摸组件之后回调方法
- pressRetentionOffset {top:number,left:number,bottom:number,right:number} 该设置当视图滚动禁用的情况下,可以定义当手指距离组件的距离。当大于该距离该组件会失去响应。当少于该距离的时候,该组件会重新进行响应。
该组件我们一般不会直接进行使用,下面三种Touchable*系列组件对于该组件的属性方法都可以进行使用。具体会具体演示这些属性方法的使用实例。
TouchableHighlight触摸高亮
该组件进行封装视图触摸点击的属性。当手指点击按下的时候,该视图的不透明度会进行降低同时会看到相应的颜色(视图变暗或者变亮)。如果我们去查看该组件的源代码会发现,该底层实现是添加了一个新的视图。如果我们没有正确的使用,就可能不会出现正确的效果。例如:我们没有给该组件视图设置backgroudnColor的颜色值。
TouchableHighlight只支持一个子节点,如果你需要设置多个子视图组件,那么就需要使用View节点进行包装。
属性方法
- TouchableWithoutFeedback的 属性,这边TouchableHighlight组件全部可以进行使用
- activeOpacity -- number 该用来设置视图在进行触摸的时候,要要显示的不透明度(通常在0-1之间)
- onHideUnderlay -- function 方法 当底层被隐藏的时候调用
- onShowUnderlay -- function 方法 当底层显示的时候调用
- style -- 可以设置控件的风格演示,该风格演示可以参考View组件的style
- underlayColor -- 当触摸或者点击控件的时候显示出的颜色
TouchableWithoutFeedback、TouchableHighlight实例
'use strict';
import React, { Component } from 'react';
import {
StyleSheet,
Text,
View,
TouchableHighlight,
TouchableWithoutFeedback,
} from 'react-native';
export default class TouchableMazouri extends Component {
render() {
return (
<View style={styles.container}>
<TouchableHighlight
underlayColor="rgb(210, 230, 255)"
activeOpacity={0.5}
style={styles.touchable}
onHideUnderlay={() => console.log("onHideUnderlay")}
onShowUnderlay={() => console.log("onShowUnderlay")}
// accessible={false}
onPress={() => console.log("onPress TouchableHighlight")}
onLongPress={() => console.log("onLongPress TouchableHighlight")}
onPressIn={() => console.log("onPressIn TouchableHighlight")}
onPressOut={() => console.log("onPressOut TouchableHighlight")}
pressRetentionOffset={{top:200,left:50,bottom:0,right:50}}
delayPressIn={2000}
delayPressOut={2000}
delayLongPress={2000}
>
<Text style={{fontSize:16}}>我是TouchableHighlight,点我吧</Text>
</TouchableHighlight>
<TouchableWithoutFeedback
style={styles.touchable}
// accessible={false}
onPress={() => console.log("onPress TouchableWithoutFeedback")}
onLongPress={() => console.log("onLongPress TouchableWithoutFeedback")}
onPressIn={() => console.log("onPressIn TouchableWithoutFeedback")}
onPressOut={() => console.log("onPressOut TouchableWithoutFeedback")}
>
<View><Text style={{fontSize:16}}>我是TouchableWithoutFeedback,点我吧</Text></View>
</TouchableWithoutFeedback>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
touchable: {
borderWidth: 1,
borderRadius: 8,
borderColor: '#e0e0e0',
backgroundColor: '#e5eCee',
}
});
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
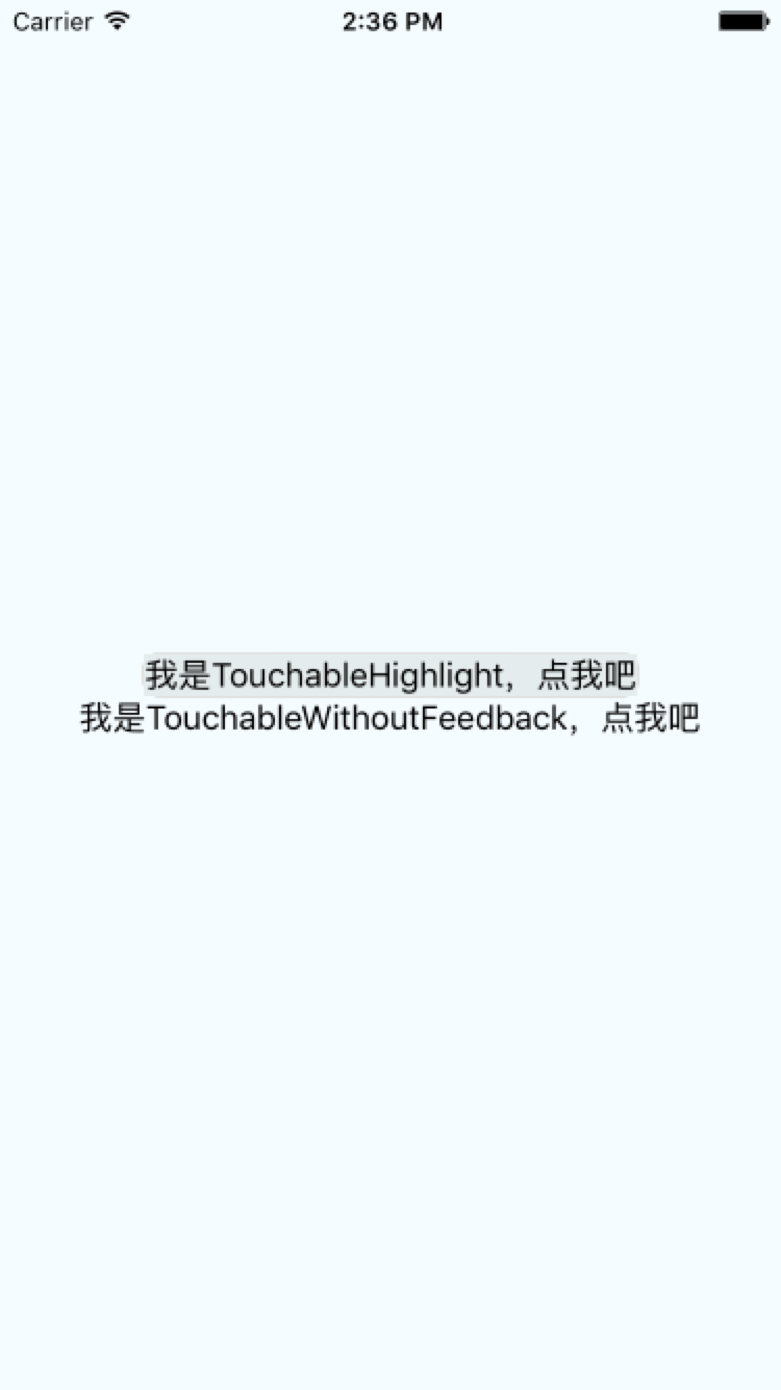
TouchableOpacity透明度变化
该组件封装了响应触摸事件。当点击按下的时候,该组件的透明度会降低。该组件使用过程中并不会改变视图的层级关系,而且我们可以非常容易的添加到应用并且不会产生额外的异常错误。
属性方法
- TouchableWithoutFeedback的属性,这边TouchableOpacity组件全部可以进行使用
- activeOpacity -- number 设置当用户触摸的时候,组件的透明度
TouchableNativeFeedback(Android专用)
该封装了可以进行响应触摸事件的组件(仅限Android平台)。在android平台上面该该组件可以使用原生平台的状态资源来显示触摸状态变化。【特别注意】现如今该组件只是支持仅有一个View的子视图实例(作为子节点)。在底层实现上面实际上面是创建一个新的RCTView的节点来进行替换当前的View节点视图,并且可以携带一些附加的属性。
该组件触摸反馈的背景图资源可以通过background属性进行自定义设置
下面一个很简单的使用实例方法如下:
'use strict';
import React, { Component } from 'react';
import {
StyleSheet,
Text,
View,
TouchableHighlight,
TouchableWithoutFeedback,
TouchableOpacity,
TouchableNativeFeedback,
} from 'react-native';
export default class TouchableMazouri extends Component {
render() {
return (
<View style={styles.container}>
<TouchableHighlight
underlayColor="rgb(210, 230, 255)"
activeOpacity={0.5}
style={styles.touchable}
onHideUnderlay={() => console.log("onHideUnderlay")}
onShowUnderlay={() => console.log("onShowUnderlay")}
// accessible={false}
onPress={() => console.log("onPress TouchableHighlight")}
onLongPress={() => console.log("onLongPress TouchableHighlight")}
onPressIn={() => console.log("onPressIn TouchableHighlight")}
onPressOut={() => console.log("onPressOut TouchableHighlight")}
pressRetentionOffset={{top:200,left:50,bottom:0,right:50}}
delayPressIn={2000}
delayPressOut={2000}
delayLongPress={2000}
>
<Text style={{fontSize:16}}>我是TouchableHighlight,点我吧</Text>
</TouchableHighlight>
<TouchableWithoutFeedback
style={styles.touchable}
// accessible={false}
onPress={() => console.log("onPress TouchableWithoutFeedback")}
onLongPress={() => console.log("onLongPress TouchableWithoutFeedback")}
onPressIn={() => console.log("onPressIn TouchableWithoutFeedback")}
onPressOut={() => console.log("onPressOut TouchableWithoutFeedback")}
>
<View><Text style={{fontSize:16}}>我是TouchableWithoutFeedback,点我吧</Text></View>
</TouchableWithoutFeedback>
<TouchableOpacity
activeOpacity={0}
style={styles.touchable}
onPress={() => console.log("onPress TouchableOpacity")}
onLongPress={() => console.log("onLongPress TouchableOpacity")}
onPressIn={() => console.log("onPressIn TouchableOpacity")}
onPressOut={() => console.log("onPressOut TouchableOpacity")}
>
<Text style={{fontSize:16}}>我是TouchableOpacity,点我就隐藏</Text>
</TouchableOpacity>
<TouchableNativeFeedback
style={styles.touchable}
>
<View style={{backgroundColor: 'blue'}}>
<Text style={{fontSize:16}}>我是TouchableNativeFeedback,点我吧</Text>
</View>
</TouchableNativeFeedback>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
touchable: {
borderWidth: 1,
borderRadius: 8,
borderColor: '#e0e0e0',
backgroundColor: '#e5eCee',
marginTop: 20,
}
});
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
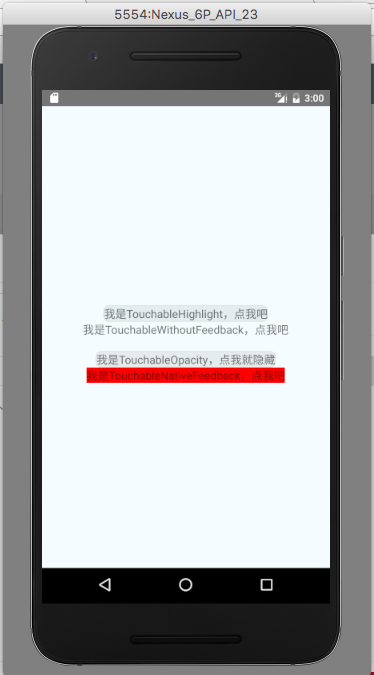
属性方法
- TouchableWithoutFeedback的 属性,这边TouchableNativeFeedback组件全部可以进行使用
- background -- backgroundPropType 该用来设置背景资源的类型,该属性会传入一个type属性和依赖的额外资源的data的对象。官方推荐采用如下的静态方法来进行生成该dictionary对象
生成该dictionary对象
- TouchableNativeFeedback.SelectableBackground() -- 该会创建使用android默认主题背景(?android:attr/selectableItemBackground)
- TouchableNativeFeedback.SelectableBackgroundBorderless() -- 该会创建使用android默认的无框的主题背景(?android:attr/selectableItemBackgroundBorderless)。不过该参数需要Android API 21+才可以使用
- TouchableNativeFeedback.Ripple(color,borderless) -- 该会创建当组件被按下的时候有一个水滴的效果.通过color参数指定颜色,如果borderless为true的时候,那么该水滴效果会渲染到该组件视图的外边。同样该背景类型参数也需要Android API 21+才可以使用
'use strict';
import React, { Component } from 'react';
import {
StyleSheet,
Text,
View,
TouchableHighlight,
TouchableWithoutFeedback,
TouchableOpacity,
TouchableNativeFeedback,
} from 'react-native';
export default class TouchableMazouri extends Component {
render() {
return (
<View style={styles.container}>
<TouchableHighlight
underlayColor="rgb(210, 230, 255)"
activeOpacity={0.5}
style={styles.touchable}
onHideUnderlay={() => console.log("onHideUnderlay")}
onShowUnderlay={() => console.log("onShowUnderlay")}
// accessible={false}
onPress={() => console.log("onPress TouchableHighlight")}
onLongPress={() => console.log("onLongPress TouchableHighlight")}
onPressIn={() => console.log("onPressIn TouchableHighlight")}
onPressOut={() => console.log("onPressOut TouchableHighlight")}
pressRetentionOffset={{top:200,left:50,bottom:0,right:50}}
delayPressIn={2000}
delayPressOut={2000}
delayLongPress={2000}
>
<Text style={{fontSize:16}}>我是TouchableHighlight,点我吧</Text>
</TouchableHighlight>
<TouchableWithoutFeedback
style={styles.touchable}
// accessible={false}
onPress={() => console.log("onPress TouchableWithoutFeedback")}
onLongPress={() => console.log("onLongPress TouchableWithoutFeedback")}
onPressIn={() => console.log("onPressIn TouchableWithoutFeedback")}
onPressOut={() => console.log("onPressOut TouchableWithoutFeedback")}
>
<View><Text style={{fontSize:16}}>我是TouchableWithoutFeedback,点我吧</Text></View>
</TouchableWithoutFeedback>
<TouchableOpacity
activeOpacity={0}
style={styles.touchable}
onPress={() => console.log("onPress TouchableOpacity")}
onLongPress={() => console.log("onLongPress TouchableOpacity")}
onPressIn={() => console.log("onPressIn TouchableOpacity")}
onPressOut={() => console.log("onPressOut TouchableOpacity")}
>
<Text style={{fontSize:16}}>我是TouchableOpacity,点我就隐藏</Text>
</TouchableOpacity>
<TouchableNativeFeedback
style={styles.touchable}
>
<View style={{backgroundColor: 'blue'}}>
<Text style={{fontSize:16}}>我是TouchableNativeFeedback,点我吧</Text>
</View>
</TouchableNativeFeedback>
<TouchableNativeFeedback
style={styles.touchable}
background={TouchableNativeFeedback.SelectableBackground()}
>
<View>
<Text style={{fontSize:16}}>selectableItemBackground,点我吧</Text>
</View>
</TouchableNativeFeedback>
<TouchableNativeFeedback
style={styles.touchable}
background={TouchableNativeFeedback.SelectableBackgroundBorderless()}
>
<View>
<Text style={{fontSize:16}}>selectableItemBackgroundBorderless,点我吧</Text>
</View>
</TouchableNativeFeedback>
<TouchableNativeFeedback
style={styles.touchable}
background={TouchableNativeFeedback.Ripple('#fff000', false)}
>
<View>
<Text style={{fontSize:16}}>selectableItemBackgroundBorderless false,点我吧</Text>
</View>
</TouchableNativeFeedback>
<TouchableNativeFeedback
style={styles.touchable}
background={TouchableNativeFeedback.Ripple('#000fff', true)}
>
<View>
<Text style={{fontSize:16}}>selectableItemBackgroundBorderless true,点我吧</Text>
</View>
</TouchableNativeFeedback>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
justifyContent: 'center',
alignItems: 'center',
backgroundColor: '#F5FCFF',
},
touchable: {
borderWidth: 1,
borderRadius: 8,
borderColor: '#e0e0e0',
backgroundColor: '#e5eCee',
marginTop: 20,
}
});
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
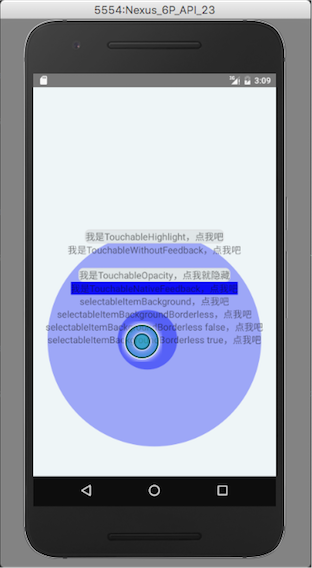
转载请标注地址:Geek马走日
Follow个人Github : mazouri
关于手势响应系统更多的学习资料: