取消JDBC的自动提交机制
JDBC在提交事务的时候若遇到了异常,则会导致下方的代码无法正常运行下去,所以就有一种取消自动提交的方法,这里用一个例子来列举一下
package com.luo.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Scanner;
public class JDBCTest03 {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement ps= null;
try{
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/smbms","root","123456");
String sql = "update t_act set balance = ? where actno = ? ";
ps = conn.prepareStatement(sql);
ps.setDouble(1,20000);
ps.setInt(2,111);
int i = ps.executeUpdate();
System.out.println(i == 1?"修改111成功":"修改111失败");
String str = null;
str.toString();
ps.setDouble(1,10000);
ps.setInt(2,222);
System.out.println(ps.executeUpdate() == 1?"修改222成功":"修改222失败");
}catch (Exception e){
e.printStackTrace();
}finally {
try{
if(ps != null){
ps.close();
}
}catch (SQLException e){
e.printStackTrace();
}
try{
if(conn != null){
conn.close();
}
}catch (SQLException e){
e.printStackTrace();
}
}
}
}
这里我的smbms中有一个t_act的表!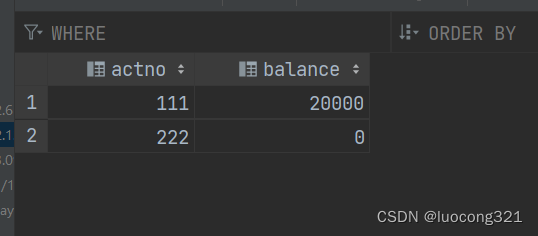
代码中,我用了两个sql语句去修改acton为111和222的两个balance,但是在修改222之前我加了一个异常,就是这个
String str = null;
str.toString();
显然,在正常情况下,程序会跳出运行,然后结束,但是前面的111的数据已经提交上去了,就会导致下面的222的数据丢失,我的理解是这是由于jdbc自动提交所导致的,所以需要修改为手动提交,既增加
conn.setAutocommit(false);
conn.commit();
conn.rollback();
增加的位置后改成的代码为:
package com.luo.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Scanner;
public class JDBCTest03 {
public static void main(String[] args) {
Connection conn = null;
PreparedStatement ps= null;
try{
Class.forName("com.mysql.cj.jdbc.Driver");
conn = DriverManager.getConnection("jdbc:mysql://localhost:3306/smbms","root","123456");
conn.setAutoCommit(false);
String sql = "update t_act set balance = ? where actno = ? ";
ps = conn.prepareStatement(sql);
ps.setDouble(1,20000);
ps.setInt(2,111);
int i = ps.executeUpdate();
System.out.println(i == 1?"修改111成功":"修改111失败");
String str = null;
str.toString();
ps.setDouble(1,10000);
ps.setInt(2,222);
System.out.println(ps.executeUpdate() == 1?"修改222成功":"修改222失败");
conn.commit();
}catch (Exception e){
if(conn != null){
try {
conn.rollback();
} catch (SQLException throwables) {
throwables.printStackTrace();
}
}
e.printStackTrace();
}finally {
try{
if(ps != null){
ps.close();
}
}catch (SQLException e){
e.printStackTrace();
}
try{
if(conn != null){
conn.close();
}
}catch (SQLException e){
e.printStackTrace();
}
}
}
}
增加之后,在str为空的前提下,程序就会跳出去,无法运行,前面的数据也不会被提交,只有当排除异常之后,111和222所修改的数据才会被一同提交上去
java小白,如果有错,欢饮大佬帮我解决我尚未理解之处,感谢感谢