1:Sansnn-uQRCode 导入插件(生成二维码)(插件市场)(源码和结果如下)
2:弹窗展示海报内容
3:createCanvasImage绘制canvas海报
4:canvasToTempFilePath (canvas转成图片文件)
<template>
<view class="popup" v-if="isPaperCode" @click="handlePaperCodeClose">
<view class="popup-content" @click.stop="()=>{}">
<view class="code">
<u-qrcode ref="qrCode" canvas-id="qrCode" :value="text" :size="size" :options="codeOptions"
@complete="complete"></u-qrcode>
</view>
<view class="username">
{{realName}}
</view>
</view>
<button class="popup-btn" @click.stop="saveShareImg">保存到相册</button>
<canvas canvas-id="myCanvas" style="width: 703rpx;height:989rpx; position: fixed;top: -10000rpx"></canvas>
</view>
</template>
<script>
import AES from "./../utils/AES.js"
import debounce from "./../utils/debounce.js"
export default {
props: {
isPaperCode: {
type: Boolean,
default: false
},
userName: {
type: String,
default: ''
},
idCardNumber: {
type: String,
default: ''
}
},
data() {
return {
codeUrl: '',
realName: '',
downLoadTime: '',
canvasToTempFilePath: '',
text: '',
size: 200,
codeOptions: {
errorCorrectLevel: 'L',
},
codeFinshed: false,
}
},
watch: {
isPaperCode(newVal) {
if (newVal) {
this.codeFinshed = false;
this.size = uni.upx2px(405)
const userinfo = JSON.parse(uni.getStorageSync('userinfo') || {});
const obj = {
cardNo: this.idCardNumber || userinfo.idCardNumber,
time: new Date().getTime() + 1800000
};
this.text = AES.encrypt(JSON.stringify(obj));
let myDate = new Date()
let year = myDate.getFullYear();
let mounths = ((myDate.getMonth() + 1) < 10 ? '0' : '') + (myDate.getMonth() + 1);
let days = (myDate.getDate() < 10 ? '0' : '') + myDate.getDate()
this.downLoadTime = `${year}.${mounths}.${days} 下载`
this.realName = this.userName || userinfo.name
}
}
},
methods: {
complete(e) {
if (e.success) {
this.$refs['qrCode'].toTempFilePath({
success: res => {
this.codeUrl = res.tempFilePath;
this.codeFinshed = true;
}
})
}
},
handlePaperCodeClose() {
if (!this.codeFinshed) return;
this.$emit("update:isPaperCode", false);
},
saveImageToPhotosAlbum() {
uni.saveImageToPhotosAlbum({
filePath: this.canvasToTempFilePath,
success: () => {
uni.showToast({
icon: 'success',
title: '保存成功',
duration: 2000
});
},
fail: () => {
uni.getSetting({
success(res) {
if (res.authSetting['scope.writePhotosAlbum'] ==
false) {
uni.showModal({
title: '提示',
content: `图片保存失败,请前往设置页面允许保存相册`,
success: function(res) {
if (res.confirm) {
uni.openSetting({
success(res) {
console.log('用户打开设置页面')
}
})
}
}
})
}
}
})
}
})
},
saveShareImg: debounce(function() {
if (this.canvasToTempFilePath) {
this.saveImageToPhotosAlbum()
} else {
this.createCanvasImage()
}
}, 500),
createCanvasImage() {
uni.showLoading({
title: '生成中...'
});
const ctx = uni.createCanvasContext('myCanvas', this);
ctx.drawImage('../static/convas-bg.png', 0, 0, uni.upx2px(703), uni.upx2px(989));
ctx.drawImage(this.codeUrl, uni.upx2px(150), uni.upx2px(241), uni.upx2px(405), uni.upx2px(
405));
ctx.font = 'normal bold 18px Source Han Sans CN';
ctx.setFillStyle('#666666');
ctx.fillText(this.realName, uni.upx2px(300), uni.upx2px(690))
ctx.font = 'normal bold 10px Source Han Sans CN';
ctx.setFillStyle('#CCCCCC');
ctx.fillText(this.downLoadTime, uni.upx2px(268), uni.upx2px(942));
ctx.draw(true, () => {
setTimeout(() => {
uni.canvasToTempFilePath({
canvasId: 'myCanvas',
width: 703,
height: 989,
destWidth: 703,
destHeight: 989,
success: res => {
this.canvasToTempFilePath = res.tempFilePath
this.saveImageToPhotosAlbum()
},
complete: () => {
uni.hideLoading();
}
}, this)
}, 200)
})
}
}
}
</script>
<style>
.popup {
position: fixed;
top: 0;
left: 0;
bottom: 0;
right: 0;
width: 750rpx;
height: 100%;
background: rgba(11, 32, 80, 0.7);
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
z-index: 9;
}
.popup-content {
margin-top: 40rpx;
width: 703rpx;
height: 989rpx;
background-size: 100% 100%;
border-radius: 6rpx;
display: flex;
flex-direction: column;
align-items: center;
}
.code {
margin-top: 240rpx;
width: 405rpx;
height: 405rpx;
}
.username {
font-size: 36rpx;
font-weight: bold;
color: #666666;
line-height: 76rpx;
}
.footer {
margin-top: 180rpx;
font-size: 20rpx;
font-family: Source Han Sans CN;
font-weight: 500;
color: #CCCCCC;
line-height: 76rpx;
}
.popup-btn {
margin-top: 35rpx;
width: 700rpx;
height: 91rpx;
background: #2E90F5;
border-radius: 6rpx;
font-size: 36rpx;
font-weight: 500;
color: #FFFFFF;
}
</style>
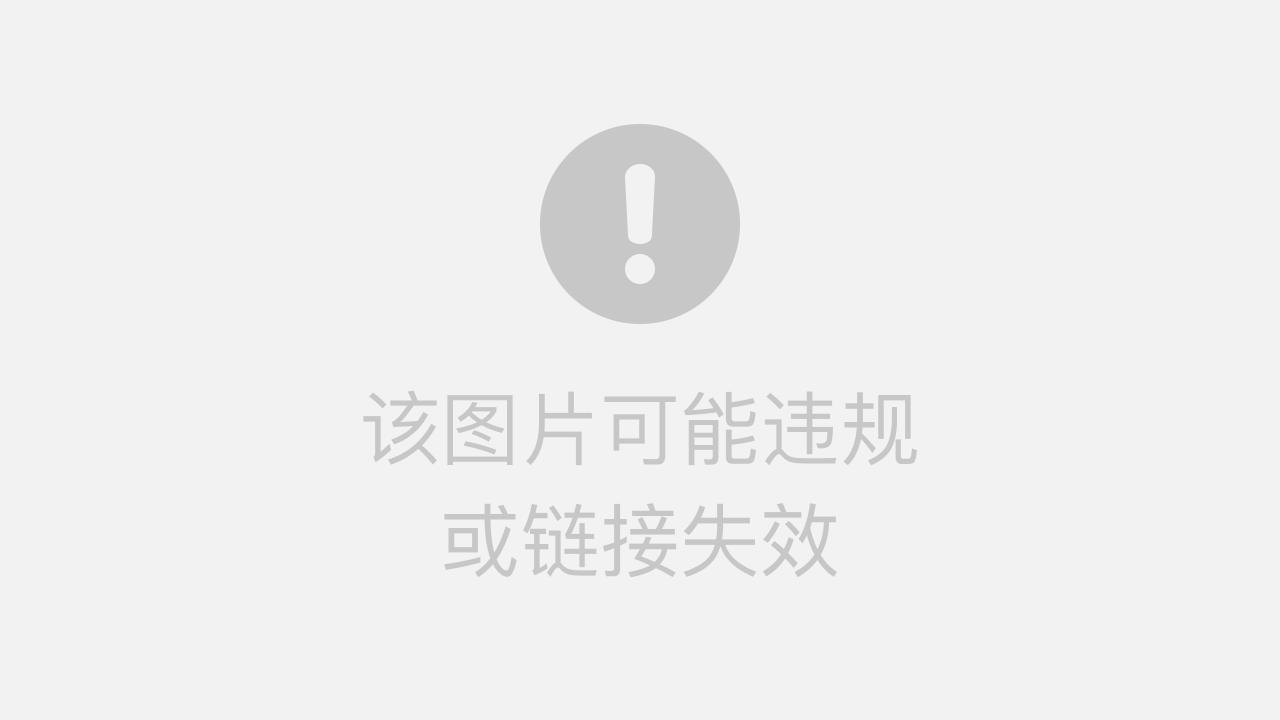