BaseExpandableListAdapter:支持动态加载
layout-item_child:
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="50dp"
android:orientation="vertical"
android:gravity="center_vertical"
android:paddingLeft="50dp">
<TextView
android:id="@+id/child"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
layout-item_group:
<LinearLayout
xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="50dp"
android:gravity="center_vertical"
android:paddingLeft="30dp">左边偏移30,显示三角形
<TextView
android:id="@+id/group"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
</LinearLayout>
SecondActivity:
public class SecondActivity extends Activity {
private ExpandableListView expandableListView;
private MyDemoBaseExpandableListAdapter adapter;
String groupStringArr[]={"腾讯","百度","阿里"};
String childStringArrs[][]={
{"QQ","微信","QQ浏览器"},
{"百度一下","百度地图","百度外卖"},
{"淘宝","支付宝"}
};
@Override
protected void onCreate( Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
expandableListView=(ExpandableListView)findViewById(R.id.my_elv);
adapter=new MyDemoBaseExpandableListAdapter();
expandableListView.setAdapter(adapter);
}
class MyDemoBaseExpandableListAdapter extends BaseExpandableListAdapter{//重点
@Override
public int getGroupCount() {
return groupStringArr.length;
}
@Override
public int getChildrenCount(int i) {
return childStringArrs[i].length;
}
@Override
public Object getGroup(int i) {
return groupStringArr[i];
}
@Override
public Object getChild(int i, int i1) {
return childStringArrs[i][i1];
}
@Override
public long getGroupId(int i) {
return i*100;
}
@Override
public long getChildId(int i, int i1) {
return i*100+i1;
}
@Override
public boolean hasStableIds() {
return false;
}
@Override
public View getGroupView(int i, boolean b, View view, ViewGroup viewGroup) {//动态加载
View view1= LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.item_group,null);
TextView textView=(TextView)view1.findViewById(R.id.group);
Object data=getGroup(i);
textView.setText((String)data);
if(i%2==1){
textView.setTextColor(Color.RED);//颜色
}
else{
textView.setTextColor(Color.BLUE);
}
return view1;
}
@Override
public View getChildView(int i, int i1, boolean b, View view, ViewGroup viewGroup) {
View view1= LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.item_child,null);
TextView textView=(TextView)view1.findViewById(R.id.child);
Object data=getChild(i,i1);
textView.setText((String)data);
if(i1>1){
textView.setTextSize(TypedValue.COMPLEX_UNIT_DIP,50);//字体大小
}
else{
textView.setTextSize(TypedValue.COMPLEX_UNIT_DIP,20);
}
return view1;
}
@Override
public boolean isChildSelectable(int i, int i1) {
return false;
}
}
}
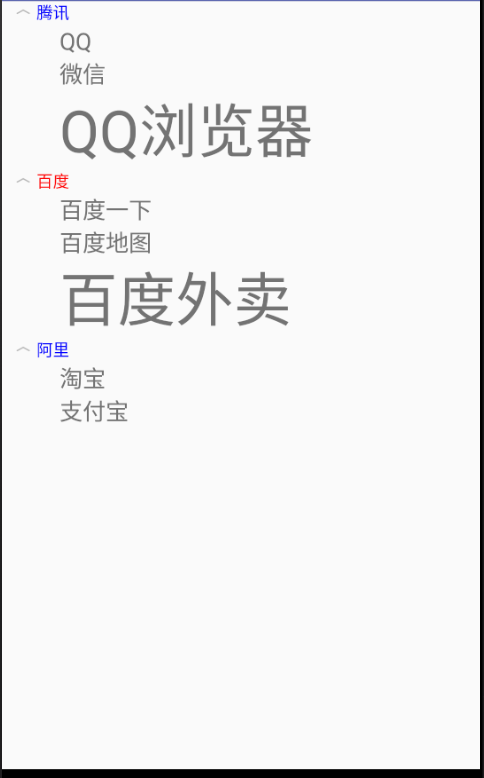