前言
在工作中遇到省市县三级联动的需求,传统的pc端大部分是用3个HTML <select>标签根据选中的一级地址去获取二级地址,然后根据二级地址去获取三级地址。实现省市县三级地址选择,需要2次服务端请求。这里使用mint--ui的Popup弹出框组件和picker选择器组件,无需请求服务端,通过遍历本地的json文件,实现本地输出三级地址,同时给每级地址绑定一个code,从而满足与后台交互时,传递code代码,这个需求非常常见。
vue.js官网:https://cn.vuejs.org/
mint-ui官网:http://mint-ui.github.io/#!/zh-cn
步骤一:使用vue-cli快速构建demo项目
vue-cli是vue提供的官方命令行工具,用于快速搭建大型单页应用。
- 新建一个空文件夹demo,安装node.js,安装方法请谷歌或者百度,非常简单。
- 用IDEA开发工具打开demo文件夹,打开Terminal终端,输入下面的命令行全局安装vue-cli
npm install vue-cli -g
- 安装成功后输入npm -v可以查看npm的版本
- 输入以下命令行回车,初始化项目配置
vue init webpack demo
- 输入Project name, Project description , Author, Install vue-router?输入Y, Use ESLint to lint your code?输入N, Set up unit tests 输入N, Setup e2e tests with Nightwatch?输入N,回车初始化安装,初始化成功后可看到以下界面
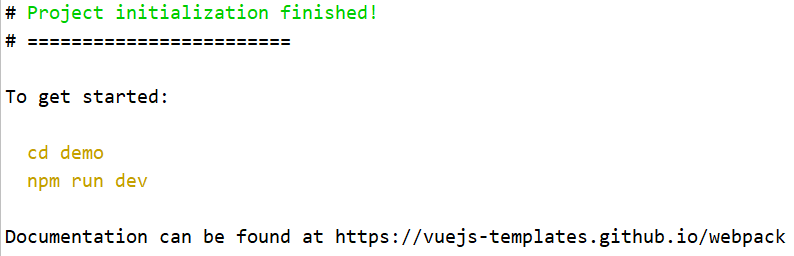
- 终端输入以下命令行,浏览器打开http://localhost:8080,demo项目构建完毕
cd demo
npm run dev
步骤二:新建一个ThreeLevelAddress.vue文件,并引入mint-ui
- 在demo/src/components路径下新建ThreeLevelAddress.vue文件
<template>
<div class="three-level-address">
hello
</div>
</template>
<script>
</script>
<style scoped>
</style>
- 在demo/src/router/index.js进行路由定义
import ThreeLevelAddress from '@/components/ThreeLevelAddress'
export default new Router({
routes: [
{
path:'/ThreeLevelAddress',
name:'ThreeLevelAddress',
component:ThreeLevelAddress
}
]
})
- 在浏览器内输入http://localhost:8080/#/ThreeLevelAddress可预览到下图
- 在终端输入以下命令行,引入mint-ui
npm i mint-ui -S
- 在demo/src/main.js路径下写入以下代码即可使用mint-ui
import MintUI from 'mint-ui'
import 'mint-ui/lib/style.css'
Vue.use(MintUI)
- 接下来测试一下是否引入成功,这里已mint-ui的button按钮组件为例。
<template>
<div class="three-level-address">
<mt-button type="default" size="large">default</mt-button>
<mt-button type="primary" size="large">primary</mt-button>
<mt-button type="danger" size="large">danger</mt-button>
</div>
</template>
<script>
import Vue from 'vue'
import { Button } from 'mint-ui';
Vue.component(Button.name, Button);
</script>
<style scoped>
</style>
效果图:
步骤三:引入Popup组件
import { Popup } from 'mint-ui';
Vue.component(Popup.name, Popup);
Popup的API
参数 | 说明 | 类型 | 可选值 | 默认值 |
---|---|---|---|---|
position | popup 的位置。省略则居中显示 |
String | 'top' 'right' 'bottom' 'left' |
|
pop-transition |