如果自下而上在类的继承层次结构中上移,位于上层的类更具有通用性,甚至可能更加抽象。
从某种角度看,族先类更加通用,人们只将它作为派生其他类的基类,而不作为想使用的特定的实例类。
例如,考虑一下对Employee类层次的扩展。一名雇员是人,一名学生也是一个人。
下面将类Person和类Student添加到类的层次结构中。图1是这三个类之间的关系层次图。
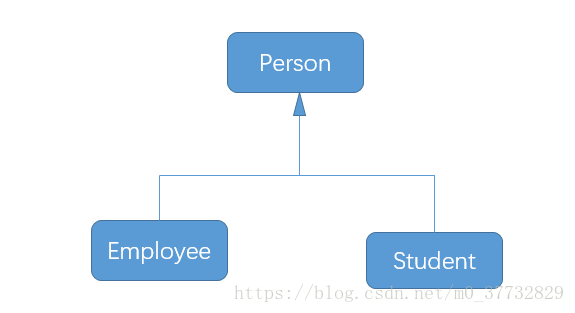
图1
abstract class Person
{
public abstract String getDescription();
}
抽象方法充当着占位的角色,它们的具体实现在子类当中。扩展抽象类可以有两种选择。一种是在抽象类中定义部分抽象方法或不定义抽象方法,这样就必须将子类标记为抽象类;另一种是定义全部的抽象方法,这样一来,子类就不是抽象的了。
抽象类不能被实例化。也就是说,如果将一个类声明为abstract,就不能创建这个类的对象。例如,表达式
new Person("Vince");
是错误的,但可以创建一个具体子类的对象。需要注意,可以定义一个抽象类的对象变量,但它只能引用非抽象子类的对象。例如,
Person p = new Student("Vince", "Economics");
这里的p是一个抽象类Person的变量,Person引用了一个非抽象子类Student的实例。
在下面程序中定义了一个抽象超类Person和两个具体子类Employee和Student。源代码如下:
public class PersonTest
{
public static void main(String[] args)
{
Person[] people = new Person[2];
people[0] = new Employee("Harry Hacker", 50000, 1989, 10, 1);
people[1] = new Student("Maria Morris", "computer science");
for(Person p:people)
{
System.out.println(p.getName() + ", " + p.getDescription());
}
}
}
public abstract class Person
{
public abstract String getDescription();
private String name;
public Person(String n)
{
name = n;
}
public String getName()
{
return name;
}
}
import java.util.Date;
import java.util.GregorianCalendar;
public class Employee extends Person
{
private double salary;
private Date hireDay;
public Employee(String n, double s, int years, int month, int day)
{
super(n);
salary = s;
GregorianCalendar calendar = new GregorianCalendar(years, month - 1, day);
hireDay = calendar.getTime();
}
public double getSalary()
{
return salary;
}
public Date getHireDay()
{
return hireDay;
}
public String getDescription()
{
return String.format("an employee with a salary of $%.2f", salary);
}
public void raiseSalary(double byPercent)
{
double raise = salary * byPercent / 100;
salary += raise;
}
}
public class Student extends Person
{
private String major;
public Student(String n, String m)
{
super(n);
major = m;
}
public String getDescription()
{
return "a student majoring in " + major;
}
}
测试抽象类,编译上面四个文件,运行 java PersonTest,执行结果见图2。
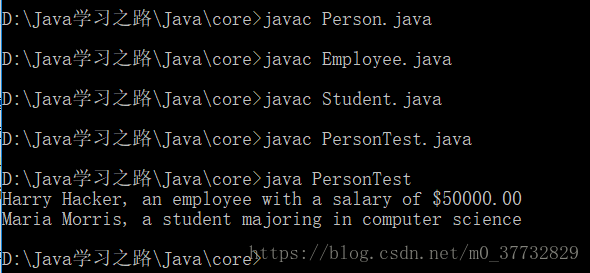
图2