相关函数API
- av_seek_frame();//跳转到指定帧
- av_rescale_q_rnd()//时间基转换函数
- av_read_frame()//读取输入流
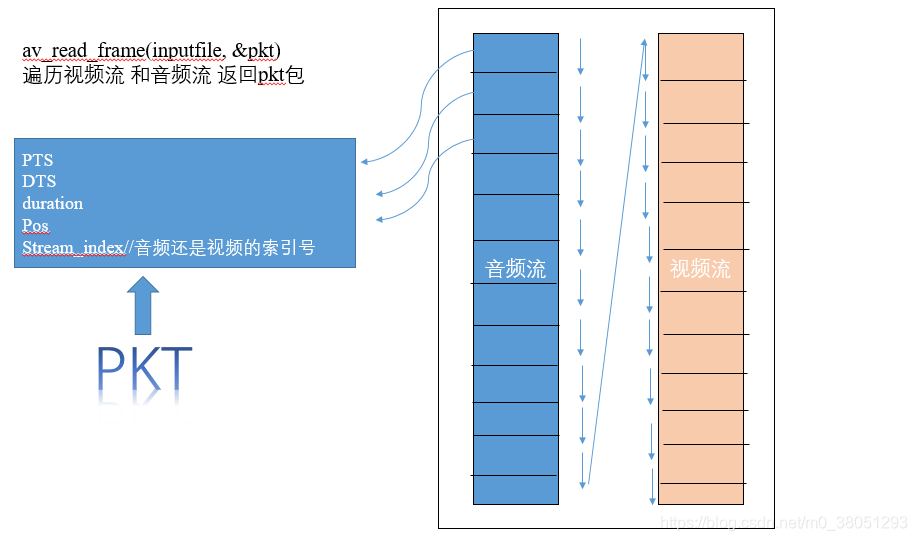
处理逻辑
- API注册
- 创建输入、输出上下文
- 初始化上下文
- 创建流及参数拷贝
- 打开输出文件,写入头信息
- 根据时间基函数判断裁剪位置写入数据
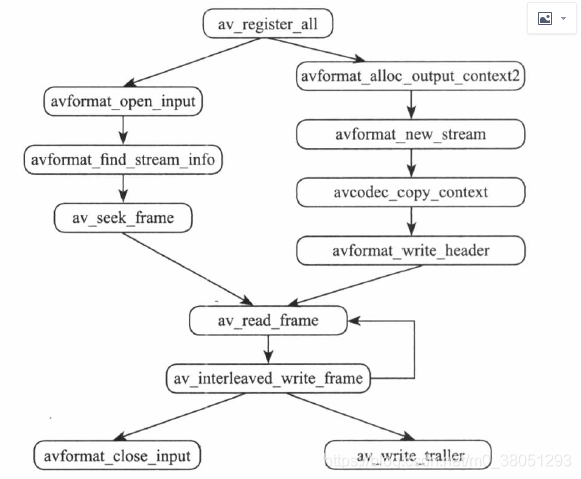
相关代码
#include<stdio.h>
#include <stdlib.h>
#include <iostream>
extern "C" {
#include "libavcodec/avcodec.h"
#include "libavformat/avformat.h"
#include "libavformat/avio.h"
#include <libavutil/log.h>
#include <libavutil/timestamp.h>
}
#define ERROR_STR_SIZE 1024
void cutVideo(double start_seconds, double end_seconds, const char *inputFileName, const char *outputFileName) {
av_register_all();
AVFormatContext * inputfile = NULL;
AVFormatContext * outputfile = NULL;
AVOutputFormat *ofmt = NULL;
AVPacket pkt;
int error_code;
int ret;
av_log_set_level(AV_LOG_DEBUG);
if (error_code = avformat_open_input(&inputfile, inputFileName, NULL, NULL) < 0) {
av_log(NULL, AV_LOG_ERROR, "Could not open src file, %s, %d(%s)\n", inputFileName);
avformat_close_input(&inputfile);
}
if (error_code = avformat_alloc_output_context2(&outputfile, NULL, NULL, outputFileName) < 0) {
av_log(NULL, AV_LOG_ERROR, "Could not open src file, %s, %d(%s)\n", inputFileName);
avformat_close_input(&inputfile);
}
for (int i = 0; i < inputfile->nb_streams; i++) {
AVStream * inputStream = inputfile->streams[i];
AVStream * outputStream = avformat_new_stream(outputfile, NULL);
if (!outputStream) {
av_log(NULL, AV_LOG_ERROR, "Could not create The Stream\n");
avformat_close_input(&inputfile);
}
avcodec_parameters_copy(outputStream->codecpar, inputStream->codecpar);
outputStream->codecpar->codec_tag = 0;
}
avio_open(&outputfile->pb, outputFileName, AVIO_FLAG_WRITE);
avformat_write_header(outputfile, NULL);
ret = av_seek_frame(inputfile, -1, start_seconds * AV_TIME_BASE, AVSEEK_FLAG_ANY);
if (ret < 0) {
fprintf(stderr, "Error seek\n");
avformat_close_input(&inputfile);
}
int64_t *dts_start_from = (int64_t*)malloc(sizeof(int64_t) * inputfile->nb_streams);
memset(dts_start_from, 0, sizeof(int64_t) * inputfile->nb_streams);
int64_t *pts_start_from = (int64_t*)malloc(sizeof(int64_t) * inputfile->nb_streams);
memset(pts_start_from, 0, sizeof(int64_t) * inputfile->nb_streams);
while (1) {
AVStream *in_stream, *out_stream;
ret = av_read_frame(inputfile, &pkt);
if (ret < 0)
break;
in_stream = inputfile->streams[pkt.stream_index];
out_stream = outputfile->streams[pkt.stream_index];
if (av_q2d(in_stream->time_base) * pkt.pts > end_seconds) {
av_free_packet(&pkt);
break;
}
if (dts_start_from[pkt.stream_index] == 0) {
dts_start_from[pkt.stream_index] = pkt.dts;
printf("dts_start_from:\n");
}
if (pts_start_from[pkt.stream_index] == 0) {
pts_start_from[pkt.stream_index] = pkt.pts;
printf("pts_start_from:\n");
}
pkt.pts = av_rescale_q_rnd(pkt.pts - pts_start_from[pkt.stream_index], in_stream->time_base, out_stream->time_base, (AVRounding)(AV_ROUND_NEAR_INF | AV_ROUND_PASS_MINMAX));
pkt.dts = av_rescale_q_rnd(pkt.dts - dts_start_from[pkt.stream_index], in_stream->time_base, out_stream->time_base, (AVRounding)(AV_ROUND_NEAR_INF | AV_ROUND_PASS_MINMAX));
if (pkt.pts < 0) {
pkt.pts = 0;
}
if (pkt.dts < 0) {
pkt.dts = 0;
}
pkt.duration = (int)av_rescale_q((int64_t)pkt.duration, in_stream->time_base, out_stream->time_base);
pkt.pos = -1;
printf("\n");
ret = av_interleaved_write_frame(outputfile, &pkt);
if (ret < 0) {
fprintf(stderr, "Error muxing packet\n");
break;
}
av_free_packet(&pkt);
}
free(dts_start_from);
free(pts_start_from);
av_write_trailer(outputfile);
}
int main(int argc, char const *argv[])
{
cutVideo(39.0, 100.0, "C:\\Users\\haizhengzheng\\Desktop\\mercury.mp4", "C:\\Users\\haizhengzheng\\Desktop\\mercut.mp4");
}