效果图
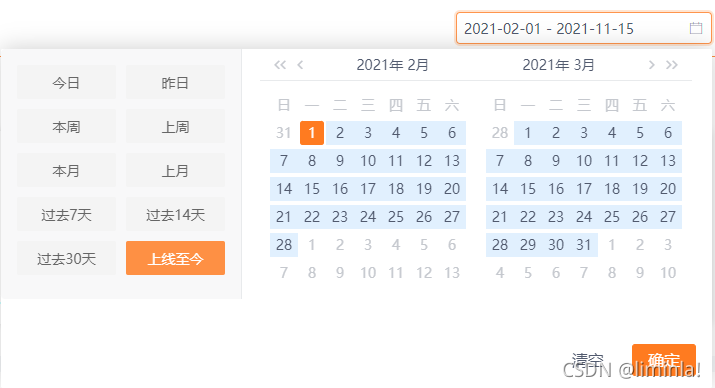
代码
<template>
<div class="dataPicker">
<DatePicker
type="daterange"
:options="options"
confirm
placement="bottom-end"
placeholder="请选择时间"
style="width: 256px"
>
</DatePicker>
</div>
</template>
<script>
export default {
data() {
return {
options: {
shortcuts: [
{
text: "今日",
value() {
const end = new Date();
const start = new Date();
return [start, end];
},
onClick: (picker) => {
this.changeStyle(picker, 0);
}
},
{
text: "昨日",
value() {
const end = new Date();
const start = new Date();
start.setTime(start.getTime() - 3600 * 1000 * 24);
return [start, end];
},
onClick: (picker) => {
this.changeStyle(picker, 1);
}
},
{
text: "本周",
value() {
const date = new Date();
const week = date.getDay();
const millisecond = 1000 * 60 * 60 * 24;
const minusDay = week != 0 ? week - 1 : 6;
const monday = new Date(date.getTime() - minusDay * millisecond);
const sunday = new Date(monday.getTime() + 6 * millisecond);
return [monday, sunday];
},
onClick: (picker) => {
this.changeStyle(picker, 2);
}
},
{
text: "上周",
value() {
const date = new Date();
var week = date.getDay();
var millisecond = 1000 * 60 * 60 * 24;
var minusDay = week != 0 ? week - 1 : 6;
var currentWeekDayOne = new Date(
date.getTime() - millisecond * minusDay
);
var priorWeekLastDay = new Date(
currentWeekDayOne.getTime() - millisecond
);
var priorWeekFirstDay = new Date(
priorWeekLastDay.getTime() - millisecond * 6
);
return [priorWeekFirstDay, priorWeekLastDay];
},
onClick: (picker) => {
this.changeStyle(picker, 3);
}
},
{
text: "本月",
value() {
const date = new Date();
let month = date.getMonth();
let year = date.getFullYear();
let firstDay = new Date(year, month, 1);
if (month == 11) {
year++;
month = 0;
} else {
month++;
}
var millisecond = 1000 * 60 * 60 * 24;
var nextMonthDayOne = new Date(year, month, 1);
var lastDay = new Date(nextMonthDayOne.getTime() - millisecond);
return [firstDay, lastDay];
},
onClick: (picker) => {
this.changeStyle(picker, 4);
}
},
{
text: "上月",
value() {
const date = new Date();
let month = date.getMonth();
let year = date.getFullYear();
let firstDay = new Date(year, month, 1);
var millisecond = 1000 * 60 * 60 * 24;
let prevLastDay = new Date(firstDay.getTime() - millisecond);
if (month == 0) {
year--;
month = 12;
} else {
month--;
}
var prevFirstDay = new Date(year, month, 1);
return [prevFirstDay, prevLastDay];
},
onClick: (picker) => {
this.changeStyle(picker, 5);
}
},
{
text: "过去7天",
value() {
const end = new Date();
const start = new Date();
start.setTime(start.getTime() - 3600 * 1000 * 24 * 7);
return [start, end];
},
onClick: (picker) => {
this.changeStyle(picker, 6);
}
},
{
text: "过去14天",
value() {
const end = new Date();
const start = new Date();
start.setTime(start.getTime() - 3600 * 1000 * 24 * 14);
return [start, end];
},
onClick: (picker) => {
this.changeStyle(picker, 7);
}
},
{
text: "过去30天",
value() {
const end = new Date();
const start = new Date();
start.setTime(start.getTime() - 3600 * 1000 * 24 * 30);
return [start, end];
},
onClick: (picker) => {
this.changeStyle(picker, 8);
}
},
{
text: "上线至今",
value() {
const end = new Date();
const start = new Date(2021, 1, 1);
return [start, end];
},
onClick: (picker) => {
this.changeStyle(picker, 9);
}
}
]
}
};
},
mounted() {},
methods: {
changeStyle(picker, index) {
let nodes = picker.$el.childNodes[0].childNodes;
this.$nextTick(() => {
for (let i = 0; i < nodes.length; i++) {
if (i === index) {
nodes[i].classList.add("active");
} else {
nodes[i].classList.remove("active");
}
}
});
}
}
};
</script>
<style lang="less" scoped>
.dataPicker {
/deep/ .ivu-select-dropdown {
left: 909px;
width: 711px;
height: 339px;
background: linear-gradient(270deg, #ffffff 0%, #ffffff 100%);
box-shadow: 0px 0px 24px 0px rgba(0, 0, 0, 0.2);
border-radius: 2px;
.ivu-picker-panel-sidebar {
width: 241px;
overflow: visible;
display: flex;
flex-wrap: wrap;
padding: 16px;
height: 250px;
}
.ivu-picker-panel-shortcut {
width: 99px;
height: 34px;
background: #f4f4f4;
border-radius: 2px;
margin-bottom: 10px;
display: flex;
color: #666666;
align-items: center;
justify-content: center;
&:hover {
background: #fe9044;
color: #ffffff;
}
&.active {
background: #fe9044;
color: #fff;
}
&:not(:nth-of-type(2n)) {
margin-right: 10px;
}
}
.ivu-picker-panel-body {
float: right;
margin-right: 20px;
}
.ivu-picker-confirm {
position: absolute;
bottom: 12px;
right: 16px;
border: none;
padding: 0;
.ivu-btn-small {
width: 64px;
height: 32px;
border-radius: 3px;
}
.ivu-btn-default {
border: none;
margin-right: 8px;
}
}
}
}
</style>