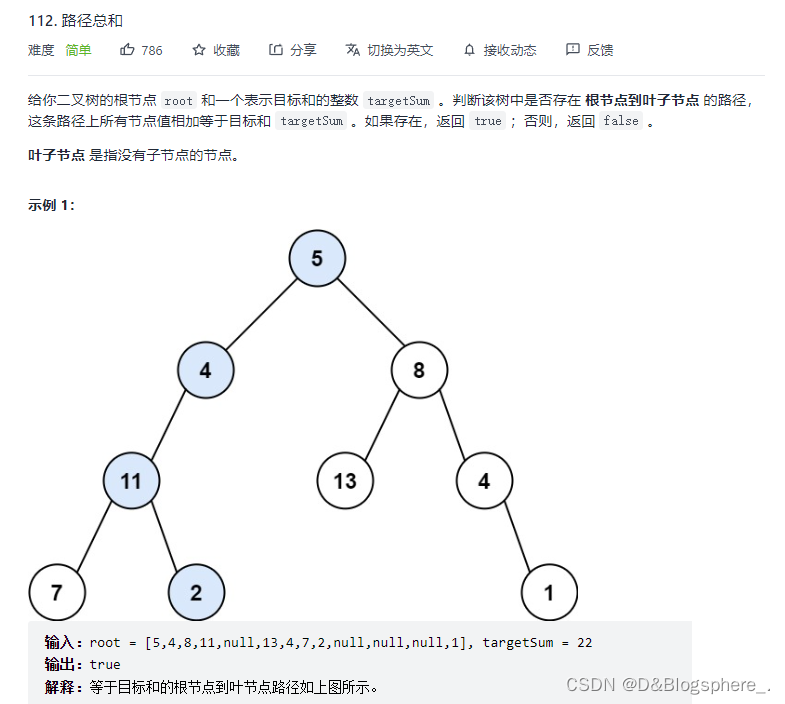
package com.tree.java;
import java.util.ArrayList;
import java.util.List;
import java.util.Stack;
public class seven {
public boolean hasPathSum(TreeNode root, int targetSum) {
if(root == null) {
return false;
}
targetSum -= root.val;
if(root.left == null && root.right == null) {
return targetSum == 0;
}
if(root.left != null) {
boolean left = hasPathSum(root.left, targetSum);
if(left) {
return true;
}
}
if(root.right != null) {
boolean right = hasPathSum(root.right, targetSum);
if(right) {
return true;
}
}
return false;
}
public boolean hasPathSum1(TreeNode root, int targetSum) {
if(root == null) {
return false;
}
if(root.left == null && root.right == null) {
return root.val == targetSum;
}
return hasPathSum1(root.left, targetSum - root.val) || hasPathSum1(root.right, targetSum - root.val);
}
public boolean hasPathSum2(TreeNode root, int targetSum) {
if(root == null) {
return false;
}
Stack<TreeNode> stack1 = new Stack<>();
Stack<Integer> stack2 = new Stack<>();
stack1.push(root);
stack2.push(root.val);
while(!stack1.isEmpty()) {
int size = stack1.size();
for(int i = 0; i < size; i++) {
TreeNode node = stack1.pop();
int sum = stack2.pop();
if(node.left == null && node.right == null && sum == targetSum) {
return true;
}
if(node.right != null) {
stack1.push(node.right);
stack2.push(sum+node.right.val);
}
if(node.left != null) {
stack1.push(node.left);
stack2.push(sum+node.left.val);
}
}
}
return false;
}
}
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode() {}
TreeNode(int val) { this.val = val; }
TreeNode(int val, TreeNode left, TreeNode right) {
this.val = val;
this.left = left;
this.right = right;
}
}
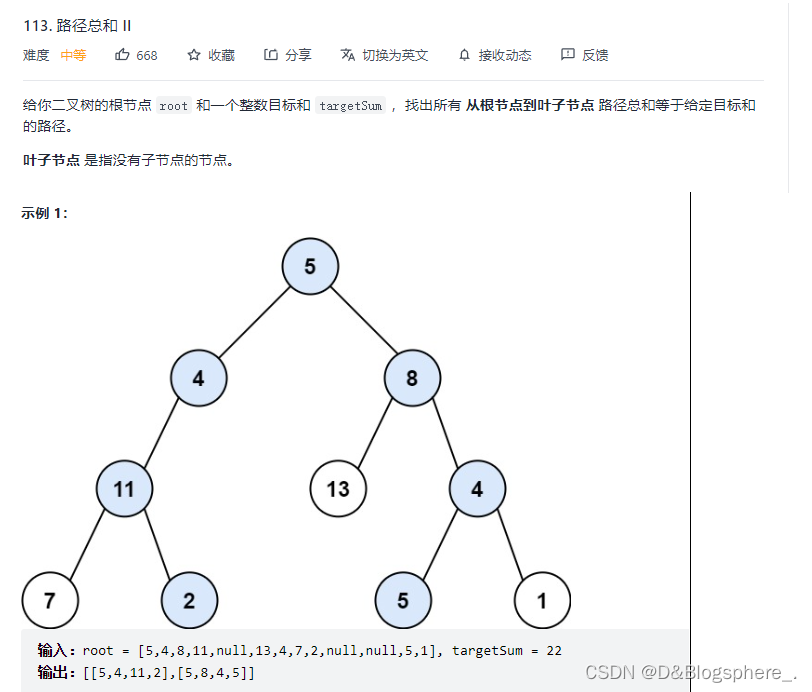
package com.tree.java;
import java.util.ArrayList;
import java.util.LinkedList;
import java.util.List;
public class seven {
public List<List<Integer>> pathSum(TreeNode root, int targetSum) {
List<List<Integer>> res = new ArrayList<>();
if(root == null) {
return res;
}
List<Integer> path = new LinkedList<>();
preorderdfs(root, targetSum, res, path);
return res;
}
private void preorderdfs(TreeNode root, int targetSum, List<List<Integer>> res, List<Integer> path) {
path.add(root.val);
if(root.left == null && root.right == null) {
if(targetSum - root.val == 0) {
res.add(new ArrayList<>(path));
}
return;
}
if(root.left != null) {
preorderdfs(root.left, targetSum-root.val, res, path);
path.remove(path.size() - 1);
}
if(root.right != null) {
preorderdfs(root.right, targetSum - root.val, res, path);
path.remove(path.size() - 1);
}
}
List<List<Integer>> result;
LinkedList<Integer> path;
public List<List<Integer>> pathSum1(TreeNode root, int targetSum) {
result = new LinkedList<>();
path = new LinkedList<>();
travesal(root, targetSum);
return result;
}
private void travesal(TreeNode root, int count) {
if(root == null) {
return;
}
path.offer(root.val);
count -= root.val;
if(root.left == null && root.right == null && count == 0) {
result.add(new LinkedList<>(path));
}
travesal(root.left, count);
travesal(root.right, count);
path.removeLast();
}
}
class TreeNode {
int val;
TreeNode left;
TreeNode right;
TreeNode() {}
TreeNode(int val) { this.val = val; }
TreeNode(int val, TreeNode left, TreeNode right) {
this.val = val;
this.left = left;
this.right = right;
}
}