C语言取5个随机点放电荷e,求网格每点的电场力及方向、场强及方向
代码
# include <stdio.h>
# include <stdlib.h>
# include <time.h>
# include <math.h>
# define K 9.0e9
# define NE 1.6e-19
struct Point
{
int x;
int y;
void initX(int x0);
void initY(int y0);
};
void Point::initX(int x0)
{
x = x0;
}
void Point::initY(int y0)
{
y = y0;
}
struct Ele
{
Point loc;
double ne;
void init(int x1, int y1);
double getPower(int x1, int y1, double e1);
double getPowerX(int x1, int y1, double power);
double getPowerY(int x1, int y1, double power);
double getE(int x1, int y1);
double getEX(int x1, int y1, double E);
double getEY(int x1, int y1, double E);
bool isEmpty();
};
void Ele::init(int x1, int y1)
{
loc.initX(x1);
loc.initY(y1);
ne = NE;
}
double Ele::getPower(int x1, int y1, double e1)
{
double r = sqrt(pow(x1 - loc.x, 2) + pow(y1 - loc.y, 2));
return K * ne * e1 / pow(r, 2);
}
double Ele::getPowerX(int x1, int y1, double power)
{
if (y1 == loc.y)
{
if (x1 - loc.x > 0)
return power;
return -power;
}
double r = sqrt(pow(x1 - loc.x, 2) + pow(y1 - loc.y, 2));
return (x1 - loc.x / r) * power;
}
double Ele::getPowerY(int x1, int y1, double power)
{
if (x1 == loc.x)
{
if (y1 - loc.y > 0)
return power;
return -power;
}
double r = sqrt(pow(x1 - loc.x, 2) + pow(y1 - loc.y, 2));
return (y1 - loc.y / r) * power;
}
double Ele::getE(int x1, int y1)
{
double r = sqrt(pow(x1 - loc.x, 2) + pow(y1 - loc.y, 2));
return K * ne / pow(r, 2);
}
double Ele::getEX(int x1, int y1, double E)
{
if (y1 == loc.y)
{
if (x1 - loc.x > 0)
return E;
return -E;
}
double r = sqrt(pow(x1 - loc.x, 2) + pow(y1 - loc.y, 2));
return (x1 - loc.x / r) * E;
}
double Ele::getEY(int x1, int y1, double E)
{
if (x1 == loc.x)
{
if (y1 - loc.y > 0)
return E;
return -E;
}
double r = sqrt(pow(x1 - loc.x, 2) + pow(y1 - loc.y, 2));
return (y1 - loc.y / r) * E;
}
bool Ele::isEmpty()
{
if (ne > 0)
return false;
return true;
}
void main()
{
srand(unsigned(time(0)));
Point arr[21][21];
Ele randEle[5];
int i, j;
int tempx;
int tempy;
for (i = 0; i < 5; i++)
{
tempx = -10 + rand() % 21;
tempy = -10 + rand() % 21;
if (!randEle[i].isEmpty())
{
for (j = 0; j < 5; j++)
{
if (randEle[i].loc.x == tempx && randEle[i].loc.y == tempy)
{
i--;
continue;
}
randEle[i].init(tempx, tempy);
}
}
else
{
randEle[i].init(tempx, tempy);
}
}
printf("\n5个随机电荷的坐标:");
for (i = 0; i < 5; i++)
printf("(%3d,%3d) ", randEle[i].loc.x, randEle[i].loc.y);
printf("\n");
double power;
double E;
double directionF;
double directionE;
double powerX = 0;
double powerY = 0;
double EX = 0;
double EY = 0;
int inputX, inputY;
printf("\n请输入网格点(试探电荷)x轴坐标,y轴坐标【注:x轴坐标(-10 ~ 10)y轴坐标(-10 ~ 10)】\n");
printf("X轴坐标:");
scanf("%d", &inputX);
printf("y轴坐标:");
scanf("%d", &inputY);
if (inputX < -10 || inputX > 10 || inputY < -10 || inputY > 10)
{
printf("坐标(%3d,%3d)超界,请重启程序重新输入!!!\n\n", inputX, inputY);
exit(0);
}
for (i = 0; i < 5; i++)
{
if (randEle[i].loc.x == inputX && randEle[i].loc.y == inputY)
{
powerX += 0;
powerY += 0;
}
else
{
powerX += randEle[i].getPowerX(inputX, inputY, randEle[i].getPower(inputX, inputY, NE));
powerY += randEle[i].getPowerY(inputX, inputY, randEle[i].getPower(inputX, inputY, NE));
}
}
power = sqrt(pow(powerX, 2) + pow(powerY, 2));
directionF = powerY / power;
for (i = 0; i < 5; i++)
{
if (randEle[i].loc.x == inputX && randEle[i].loc.y == inputY)
{
EX += 0;
EY += 0;
}
else
{
EX += randEle[i].getEX(inputX, inputY, randEle[i].getE(inputX, inputY));
EY += randEle[i].getEY(inputX, inputY, randEle[i].getE(inputX, inputY));
}
}
E = sqrt(pow(EX, 2) + pow(EY, 2));
directionE = EY / E;
printf("\n****************************计算结果*******************************************\n");
printf("*******************************************************************************\n");
printf("(%d,%d)点电场力为:%.34lf 方向为:sinQ=%lf\n", inputX, inputY, power, directionF);
printf("*******************************************************************************\n");
printf("(%d,%d)点场强为:%.34lf 方向为:sinQ=%lf\n", inputX, inputY, E, directionE);
printf("*******************************************************************************\n\n");
}
运行截图
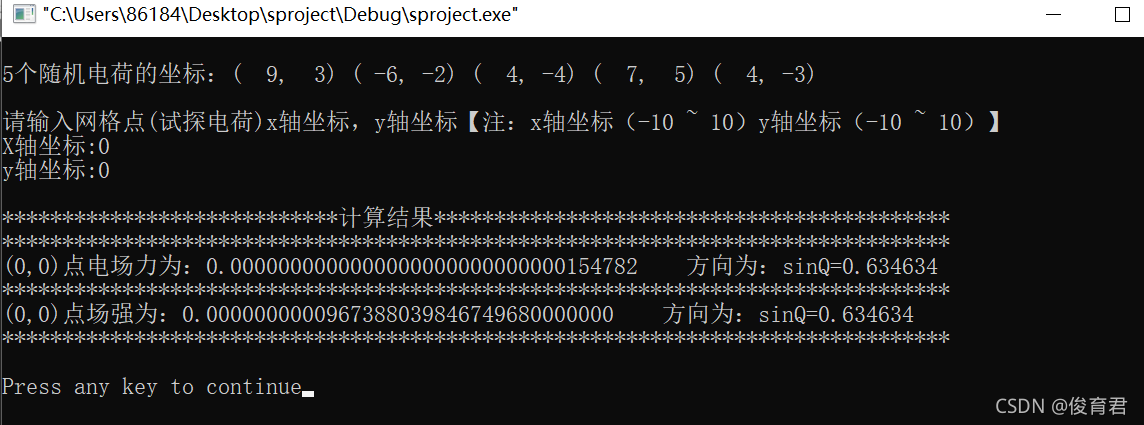