多窗口排队系统
1、头文件1:WindowQueue.
struct Lnode
{
int data;
time_t nowTime;
Lnode *next;
};
struct Hnode
{
Lnode *front;
Lnode *rear;
};
struct Lqueue
{
Hnode *head;
int Len();
void Init();
bool IsEmpty();
void EnterQueue(int x);
bool OutQueue(int &e,time_t &t);
int GetHeadElem(int &e);
int SumTime();
};
int Lqueue::GetHeadElem(int &e)
{
Lnode *p;
if (IsEmpty()) return 0;
p=head->front;
e=p->data;
return e;
}
int Lqueue::SumTime()
{
Lnode *p;
int stime = 0;
p=head->front;
while (p)
{
stime += p->data;
p=p->next;
}
return stime;
}
int Lqueue::Len()
{
Lnode *p;
int leng=0;
p=head->front;
while (p)
{
leng++;
p=p->next;
}
return leng;
}
bool Lqueue::OutQueue(int &e,time_t &t)
{
Lnode *p;
if (IsEmpty()) false;
p=(Lnode *)malloc(sizeof(Lnode));
p=head->front;
if (p->next)
{
e=p->data;
t = (int)difftime(time(NULL),p->nowTime);
head->front=p->next;
free(p);
}
else
{
e=p->data;
t = (int)difftime(time(NULL),p->nowTime);
free(p);
head->front=NULL;
head->rear=NULL;
}
return true;
}
void Lqueue::EnterQueue(int x)
{
Lnode *p;
p=(Lnode *)malloc(sizeof(Lnode));
p->nowTime = time(NULL);
p->data=x;
p->next=NULL;
if (IsEmpty())
{
head->front=p;
head->rear=p;
}
else
{
head->rear->next=p;
head->rear=p;
}
}
bool Lqueue::IsEmpty()
{
if (head->front==NULL) return true;
return false;
}
void Lqueue::Init()
{
head=(Hnode *)malloc(sizeof(Hnode));
head->front=NULL;
head->rear=NULL;
}
2、头文件2:Function.h
int color(int c)
{
SetConsoleTextAttribute(GetStdHandle(STD_OUTPUT_HANDLE),c);
return 0;
}
void gotoxy(int x,int y)
{
COORD pos;
pos.X = x;
pos.Y = y;
SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE),pos);
}
void menu1(Lqueue L[])
{
gotoxy(20,5);
color(1);
printf("--------------------------------------------");
for (int i = 6; i <= 14; i++)
{
gotoxy(32,i);
printf("|");
gotoxy(50,i);
printf("|");
}
gotoxy(20,15);
printf("--------------------------------------------");
gotoxy(20,6);
color(7);
printf("(0号窗口)\t (1号窗口)\t (2号窗口)");
gotoxy(20,8);
color(1);
printf("--------------------------------------------");
color(5);
gotoxy(20,9);
printf("%d人在等待\t %d人在等待\t %d人在等待",L[0].Len(),L[1].Len(),L[2].Len());
color(1);
}
void menu2()
{
gotoxy(20,16);
printf("1、取号办理业务 2、查看当前窗口业务办理情况");
gotoxy(20,18);
printf("3、查看排队情况 4、结束办理");
gotoxy(20,20);
printf("5、退出 6、查看窗口办理效率");
}
void quhao(Lqueue L[])
{
int i,length;
while (1)
{
gotoxy(20,16);
color(2);
printf("请选择窗口(0,1,2)[ ]\b\b");
color(6);
scanf("%d",&i);
color(1);
if ( i == 0 || i == 1 || i == 2)
{
length = (L[i].Len()) + 1;
system("cls");
L[i].EnterQueue(length);
gotoxy(20,16);
printf("办理业务中,您的业务号是%d,您排在第%d个\n",L[i].Len(),L[i].Len());
break;
} else {
system("cls");
gotoxy(20,16);
color(12);
printf("输入有误!请重新输入\n");
color(1);
gotoxy(20,17);
system("pause");
system("cls");
menu1(L);
}
}
}
void chakan(Lqueue L[])
{
int i,e;
gotoxy(20,5);
color(1);
printf("--------------------------------------------");
for (i = 6; i <= 14; i++)
{
gotoxy(32,i);
printf("|");
gotoxy(50,i);
printf("|");
}
gotoxy(20,15);
printf("--------------------------------------------");
gotoxy(20,6);
color(7);
printf("(0号窗口) (1号窗口) (2号窗口)");
color(1);
gotoxy(20,8);
printf("--------------------------------------------");
gotoxy(20,9);
color(5);
printf("%d号顾客在办理 %d号顾客在办理 %d号顾客在办理",L[0].GetHeadElem(e),L[1].GetHeadElem(e),L[2].GetHeadElem(e));
gotoxy(20,16);
color(4);
printf("【注:0号即为该窗口处于空闲状态】");
color(1);
}
void endWork(Lqueue L[],Lqueue Lt[])
{
int e,e2,i;
time_t t;
while (1)
{
gotoxy(20,16);
color(2);
printf("请选择窗口(0,1,2)[ ]\b\b");
color(6);
scanf("%d",&i);
color(1);
if ( i == 0 || i == 1 || i == 2)
{
system("cls");
gotoxy(20,16);
if (L[i].IsEmpty())
{
} else {
printf("您的业务号是%d:\n",L[i].GetHeadElem(e));
L[i].OutQueue(e2,t);
gotoxy(20,17);
printf("已结束办理,办理业务消耗:%d秒,请迅速离开!",t);
Lt[i].EnterQueue(t);
gotoxy(20,18);
system("pause");
}
break;
} else {
system("cls");
gotoxy(20,16);
color(12);
printf("输入有误!请重新输入\n");
color(1);
gotoxy(20,17);
system("pause");
system("cls");
menu1(L);
}
}
}
void xiaolv(Lqueue Lt[])
{
int i;
gotoxy(20,5);
color(1);
printf("--------------------------------------------");
for (i = 6; i <= 14; i++)
{
gotoxy(32,i);
printf("|");
gotoxy(50,i);
printf("|");
}
gotoxy(20,15);
printf("--------------------------------------------");
gotoxy(20,6);
color(7);
printf("(0号窗口) (1号窗口) (2号窗口)");
color(1);
gotoxy(20,8);
printf("--------------------------------------------");
gotoxy(20,12);
color(5);
int L1 = Lt[0].Len();
int L2 = Lt[1].Len();
int L3 = Lt[2].Len();
float x1, x2, x3;
if (L1 == 0)
x1 = 0;
else
x1 = (Lt[0].SumTime() * 0.1) / L1;
if (L2 == 0)
x2 = 0;
else
x2 = (Lt[1].SumTime() * 0.1)/L2;
if (L3 == 0)
x3 = 0;
else
x3 = (Lt[2].SumTime() * 0.1)/L3;
printf("处理效率%0.2f 处理效率%0.2f 处理效率%0.2f",x1,x2,x3);
gotoxy(20,16);
color(4);
printf("【注:当窗口效率低于0.00时可认为该窗口空闲或该窗口业务处理效率高】");
printf("测试:窗口3的人数是:%d 窗口1人数是:%d\n",L3,L1);
color(1);
}
4、main函数:LineUp.c
# include <stdio.h>
# include <stdlib.h>
# include <windows.h>
# include <conio.h>
# include <time.h>
# define WINDOWSIZE 2
# include "WindowQueue.h"
# include "Function.h"
void main()
{
char str;
static Lqueue L[WINDOWSIZE];
static Lqueue Lt[WINDOWSIZE];
for (int j = 0; j <= WINDOWSIZE; j++)
{
L[j].Init();
Lt[j].Init();
}
while (1)
{
gotoxy(20,2);
color(1);
printf("--------------------------------------------");
gotoxy(35,3);
printf("多窗口排队系统");
menu1(L);
menu2();
gotoxy(20,22);
color(2);
printf("请输入序号[ ]\b\b");
color(6);
str = getchar();
color(1);
switch (str)
{
case '1':
system("cls");
menu1(L);
quhao(L);
gotoxy(20,17);
system("pause");
system("cls");
break;
case '2':
system("cls");
gotoxy(30,4);
printf("各窗口正在办理业务顾客情况");
chakan(L);
gotoxy(20,17);
system("pause");
system("cls");
break;
case '3':
system("cls");
gotoxy(35,4);
printf("各窗口排队情况");
menu1(L);
gotoxy(20,16);
system("pause");
system("cls");
break;
case '4':
system("cls");
menu1(L);
endWork(L,Lt);
system("puase");
system("cls");
break;
case '5':
gotoxy(20,20);
printf("欢迎你下次继续使用,再按一次退出!\t\t");
exit(0);
break;
case '6':
system("cls");
gotoxy(30,4);
printf("各窗口正在办理业务效率(秒/人)");
xiaolv(Lt);
gotoxy(20,17);
system("pause");
system("cls");
break;
default:;
break;
}
}
}
3、运行结果
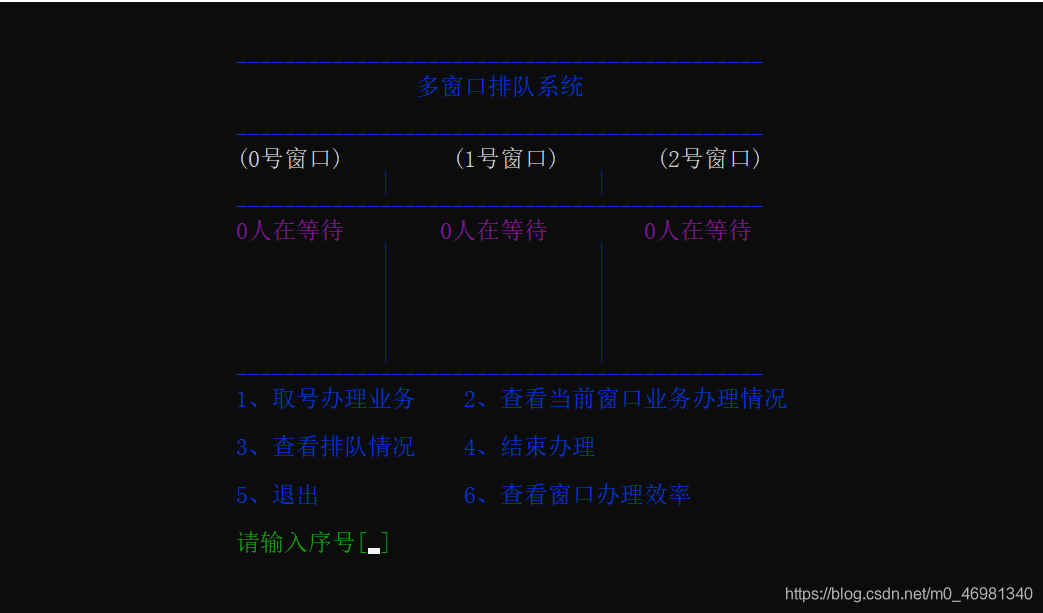