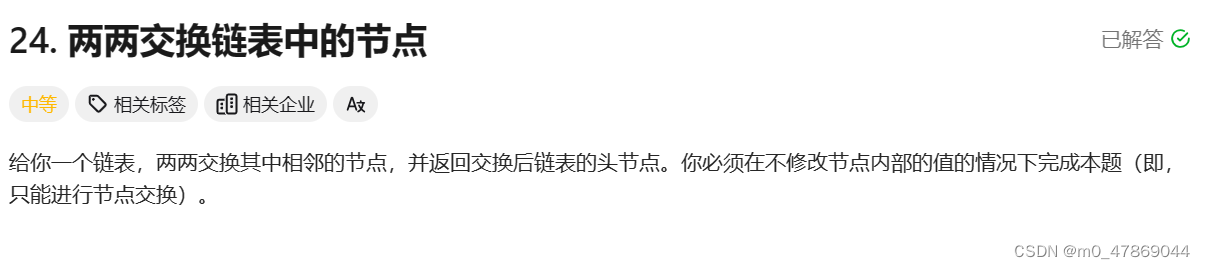
class Solution {
public ListNode swapPairs(ListNode head) {
if (head==null ||head.next ==null) return head;
ListNode dummy = new ListNode(-1);
dummy.next = head;
ListNode cur = dummy;
ListNode t1 = null;
ListNode t2 = null;
ListNode t3 = null;
while(cur.next!=null && cur.next.next!=null){
t1 = cur.next;
t2 = cur.next.next;
t3 = cur.next.next.next;
cur.next = t2;
t2.next = t1;
t1.next = t3;
cur = t1;
}
return dummy.next;
}
}
class Solution {
public ListNode swapPairs(ListNode head) {
if (head==null ||head.next ==null) return head;
ListNode pre = head;
ListNode cur = pre.next;
ListNode newnode = swapPairs(cur.next);
pre.next = newnode;
cur.next = pre;
return cur;
}
}
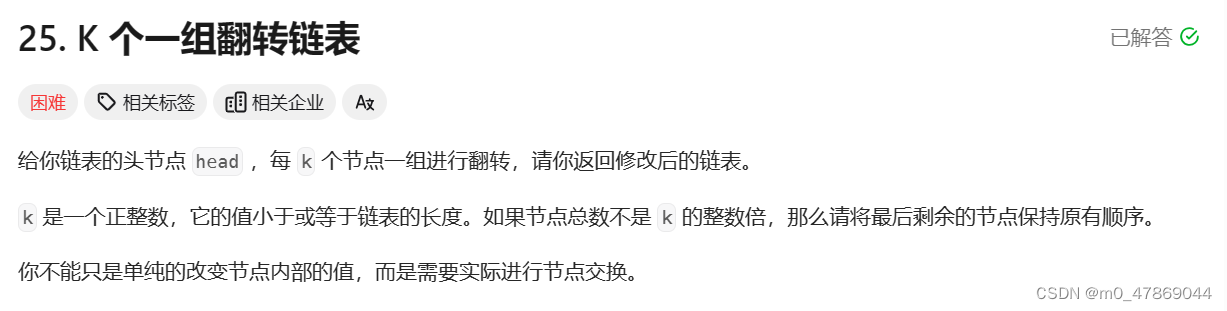
class Solution {
public ListNode reverseKGroup(ListNode head, int k) {
if (head==null || head.next==null) return head;
ListNode start = head;
ListNode end = head;
for (int i=0;i<k;i++){
if (end==null) return start;
end = end.next;
}
ListNode headNode = reverse(head,end);
start.next = reverseKGroup(end,k);
return headNode;
}
public ListNode reverse(ListNode start, ListNode end){
ListNode pre = null;
ListNode cur = start;
while(cur!=end){
ListNode temp = cur.next;
cur.next = pre;
pre = cur;
cur = temp;
}
return pre;
}
}
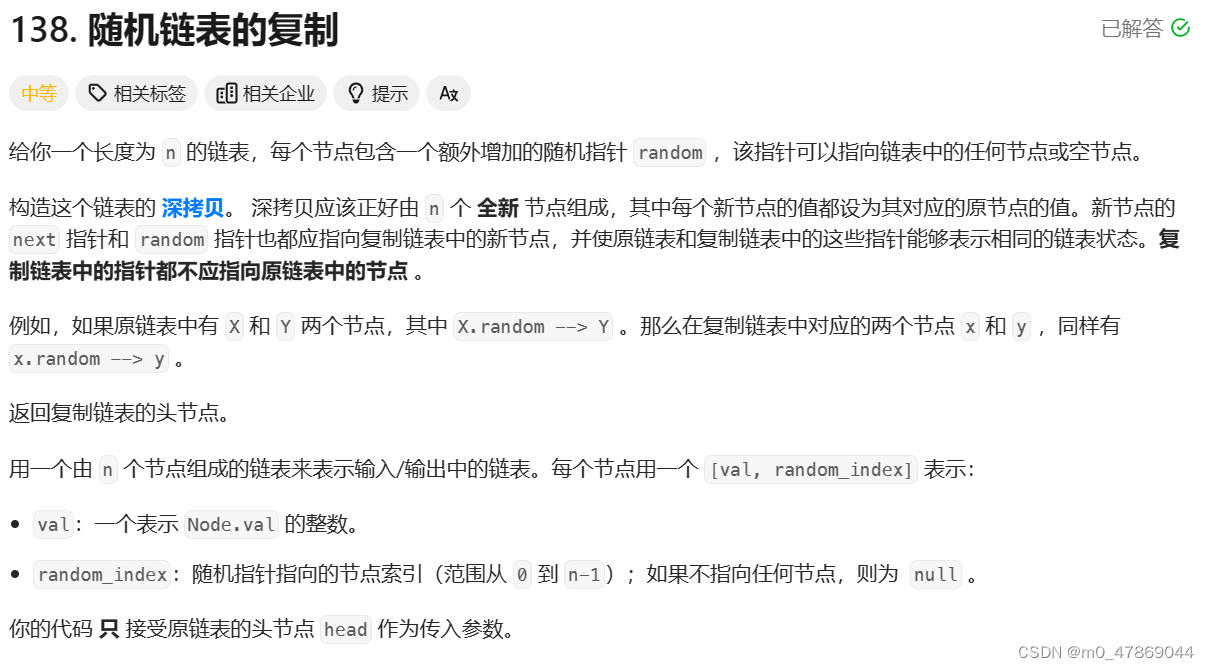
class Solution {
public Node copyRandomList(Node head) {
if (head==null) return null;
Node cur = head;
Map<Node,Node> map = new HashMap<>();
while(cur!=null){
map.put(cur,new Node(cur.val));
cur = cur.next;
}
cur = head;
while(cur!=null){
map.get(cur).next = map.get(cur.next);
map.get(cur).random = map.get(cur.random);
cur = cur.next;
}
return map.get(head);
}
}

class Solution {
public ListNode sortList(ListNode head) {
return sortList1(head, null);
}
public ListNode sortList1(ListNode head, ListNode tail){
if (head==null) return head;
if (head.next==tail) {
head.next = null;
return head;
}
ListNode slow = head, fast = head;
while( fast!=tail){
slow = slow.next;
fast = fast.next;
if (fast!=tail) fast = fast.next;
}
ListNode mid = slow;
ListNode l1 = sortList1(head,mid);
ListNode l2 = sortList1(mid,tail);
ListNode sorted = merge(l1,l2);
return sorted;
}
public ListNode merge(ListNode l1,ListNode l2){
ListNode dummy = new ListNode(0);
ListNode first = dummy, t1 = l1, t2 = l2;
while(t1!=null && t2!=null){
if (t1.val<=t2.val){
first.next = t1;
t1 = t1.next;
}else {
first.next = t2;
t2 = t2.next;
}
first = first.next;
}
if (t1!=null){
first.next = t1;
}else if (t2!=null){
first.next = t2;
}
return dummy.next;
}
}
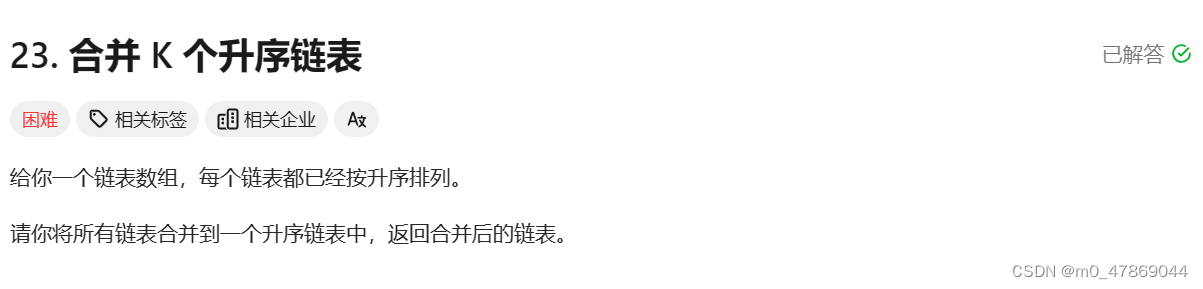
class Solution {
public ListNode mergeKLists(ListNode[] lists) {
ListNode result = null;
for (int i=0;i<lists.length;i++){
result = merge(result,lists[i]);
}
return result;
}
public ListNode merge(ListNode a, ListNode b){
if (a==null || b==null){
return a!=null ? a : b;
}
ListNode dummy = new ListNode(0);
ListNode temp = dummy, t1 = a, t2 = b;
while(t1!=null && t2!=null){
if (t1.val<=t2.val){
temp.next = t1;
t1 =t1.next;
}else{
temp.next = t2;
t2 = t2.next;
}
temp = temp.next;
}
temp.next = (t1!=null ? t1 : t2);
return dummy.next;
}
}
class Solution {
public ListNode mergeKLists(ListNode[] lists) {
PriorityQueue<ListNode> pq = new PriorityQueue<>((a,b)-> a.val-b.val);
for (ListNode list : lists){
if (list!=null){
pq.offer(list);
}
}
ListNode dummy = new ListNode();
ListNode cur = dummy;
while(!pq.isEmpty()){
ListNode node = pq.poll();
if (node.next!=null){
pq.offer(node.next);
}
cur.next = node;
cur = cur.next;
}
return dummy.next;
}
}
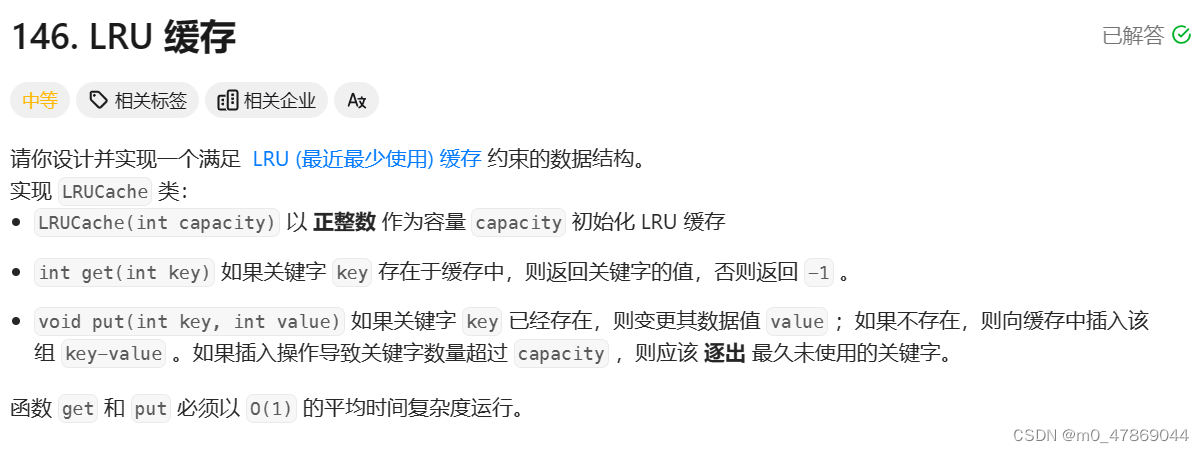
class LRUCache {
private static class Node{
int key,value;
Node prev, next;
Node(int key, int value){
this.key = key;
this.value = value;
}
}
private final int capacity;
private final Node dummy = new Node(0,0);
private final Map<Integer, Node> keyToNode = new HashMap<>();
// 初始化
public LRUCache(int capacity) {
this.capacity = capacity;
dummy.prev = dummy;
dummy.next = dummy;
}
public int get(int key) {
Node node = getNode(key);
return node != null ? node.value : -1;
}
public void put(int key, int value) {
Node node = getNode(key);
if (node!=null){
node.value = value;
return;
}
node = new Node(key,value);
keyToNode.put(key,node);
pushFront(node);
if (keyToNode.size()>capacity){
Node backNode = dummy.prev;
keyToNode.remove(backNode.key);
remove(backNode);
}
}
private Node getNode(int key){
if (!keyToNode.containsKey(key)){
return null;
}
Node node = keyToNode.get(key);
remove(node);
pushFront(node);
return node;
}
private void remove(Node x){
x.prev.next = x.next;
x.next.prev = x.prev;
}
private void pushFront(Node x){
x.prev = dummy;
x.next = dummy.next;
x.next.prev = x;
dummy.next = x;
}
}
/**
* Your LRUCache object will be instantiated and called as such:
* LRUCache obj = new LRUCache(capacity);
* int param_1 = obj.get(key);
* obj.put(key,value);
*/