告诉大家该怎么学?
这是什么?
它怎么玩?
该如何去在我们平时运用?
组件:
窗口
弹窗
面板
文本框
列表框
按钮
图片
监听事件
鼠标
键盘事件
外挂
简介
Gui的核心技术:
1、Swing AWT, 界面不美观
需要jre环境
可以写出自己心中想要的一些小工具。
工作时候,也可能需要维护swing界面。
MVC的架构,了解监听。
AWT
2、1 Awt介绍(abstract window tools)
1、包含很多类和接口!Gui(图形用户界面编程)
Eeclipse
元素:窗口、按钮、文本框
java.awt包
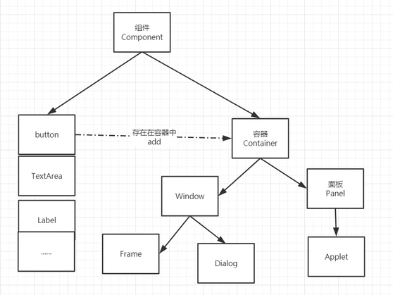
2、2 组件和容器
2、2、1Frame
package com.Zhang.lesson01;
import java.awt.*;
//GUI的第一个界面
public class TestFrame {
public static void main(String[] args) {
//Frame对象,jdk
Frame frame = new Frame("我的第一个Java图形界面窗口");
//需要设置可见性
frame.setVisible(true);
//设置窗口大小
frame.setSize(400,400);
//设置颜色 Color(没学过此类,看源码)
frame.setBackground(new Color(128, 128, 128));
//弹出的初始位置
frame.setLocation(200,200);//(0,0)在左上角
//设置大小固定
frame.setResizable(false);
}
}
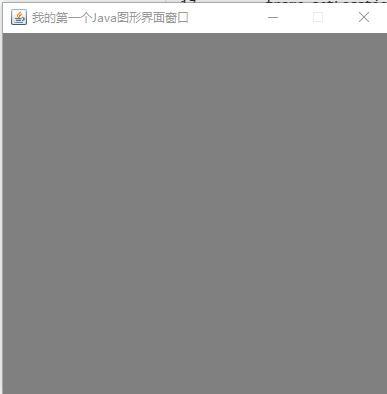
问题:发现窗口关闭不掉,停止运行即可。
尝试回顾封装:
package com.Zhang.lesson01;
import java.awt.*;
public class TestFrame2 {
public static void main(String[] args) {
//展示多个窗口
MyFrame myFrame = new MyFrame(100, 100, 200, 200, Color.blue);
MyFrame myFrame2 = new MyFrame(300, 100, 200, 200, Color.white);
MyFrame myFrame3 = new MyFrame(100, 300, 200, 200, Color.yellow);
MyFrame myFrame4 = new MyFrame(300, 300, 200, 200, Color.green);
}
}
class MyFrame extends Frame {
static int id=0;//可能存在多个窗口,需要一个计数器
public MyFrame(int x, int y,int w,int h,Color color){
super("MyFrame+"+(++id));
setVisible(true);
setBounds(x,y,w,h);
//设置长宽和坐标
//setSize(w,h);
//setLocation(x,y);
setBackground(color);
}
}
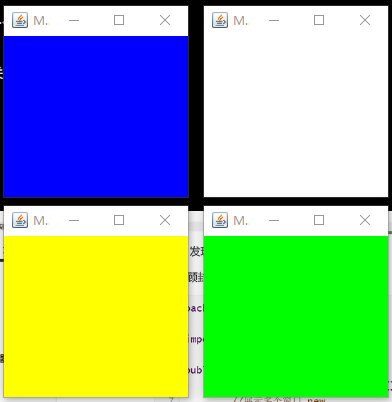
2、2、2面板Panel
解决了关闭事件!
package com.Zhang.lesson01;
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
//Panel,可以看成是一个空间,但不能单独存在,放置在窗口上//组件是放在面板上。
public class TestPanel {
public static void main(String[] args) {
Frame frame = new Frame();//总窗口
//布局的概念
Panel panel = new Panel();//面板
//设置布局
frame.setLayout(null);
//设置坐标
frame.setBounds(300,300,500,500);
frame.setBackground(new Color(13, 130, 116));
//panel设置坐标,相对于Frame;(相对坐标)
panel.setBounds(50,50,400,400);
panel.setBackground(new Color(197, 48, 197));
//frame.add(panel);//panel是个component
frame.add(panel);
frame.setVisible(true);
//监听事件,监听窗口关闭事件(退出) System.exit(0)
//适配器模式:
frame.addWindowListener(new WindowAdapter() {
//窗口点击关闭需要做的事情
@Override
public void windowClosing(WindowEvent e) {
//结束程序
System.exit(1);
//super.windowClosing(e);
}
});
}
}
点×可关闭该窗口。
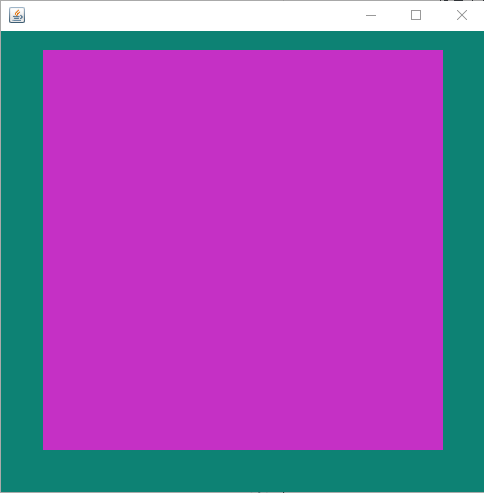
3、布局管理器
流式布局
package com.Zhang.lesson01;
import java.awt.*;
public class TestFlowLayout {
public static void main(String[] args) {
Frame frame = new Frame();
//组件-按钮
Button button1 = new Button("button1");
Button button2 = new Button("button1");
Button button3 = new Button("button1");
//设置为流式布局
//frame.setLayout(new FlowLayout());//默认为center,按钮在窗口的中间
frame.setLayout(new FlowLayout(FlowLayout.LEFT));//按钮在窗口的左边
frame.setSize(200,200);
//添加按钮
frame.add(button1);
frame.add(button2);
frame.add(button3);
frame.setVisible(true);
}
}
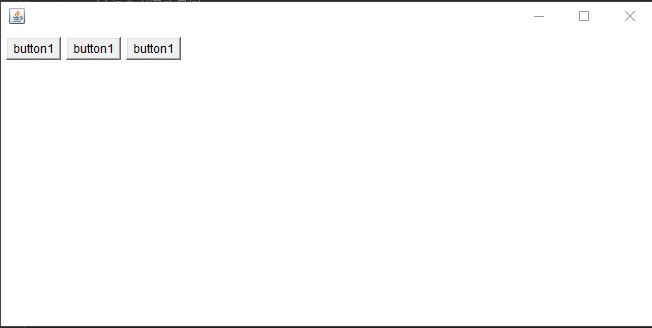
东西南北中(布局如下图)
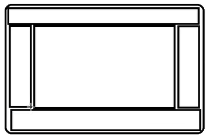
public static void main(String[] args) {
Frame frame = new Frame("TestBorderLayout");
Button east = new Button("East");
Button west = new Button("West");
Button south = new Button("South");
Button north = new Button("North");
Button center = new Button("Center");
frame.add(east,BorderLayout.EAST);
frame.add(west,BorderLayout.WEST);
frame.add(south,BorderLayout.SOUTH);
frame.add(north,BorderLayout.NORTH);
frame.add(center,BorderLayout.CENTER);
frame.setSize(200,200);
frame.setVisible(true);
}
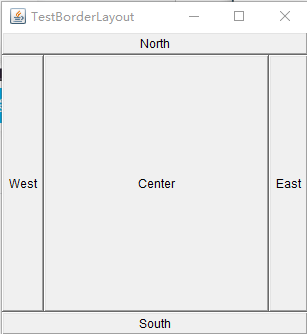
表格布局Grid
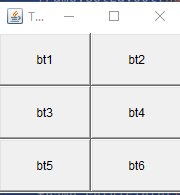
Frame frame = new Frame("TestGridLayout");
Button bt1 = new Button("bt1");
Button bt2 = new Button("bt2");
Button bt3 = new Button("bt3");
Button bt4 = new Button("bt4");
Button bt5 = new Button("bt5");
Button bt6 = new Button("bt6");
frame.setLayout(new GridLayout(3,2));
frame.add(bt1);
frame.add(bt2);
frame.add(bt3);
frame.add(bt4);
frame.add(bt5);
frame.add(bt6);
frame.pack();//java函数!
frame.setSize(200,200);
frame.setVisible(true);
练习
自己:
public static void main(String[] args) {
Frame frame = new Frame("TestExercise");
Panel panel1 = new Panel();//面板
Panel panel2= new Panel();//面板
Panel panel3 = new Panel();//面板
Panel panel4 = new Panel();//面板
frame.setLayout(new GridLayout(2,2));
frame.add(panel1);
frame.add(panel2);
//上边
Button east = new Button("East");//按钮
Button west = new Button("West");//按钮
panel1.add(east,BorderLayout.EAST);
panel1.add(panel3,BorderLayout.CENTER);
panel1.add(west,BorderLayout.WEST);
panel3.setLayout(new GridLayout(2,1));
Button bt1 = new Button("bt1");
Button bt2 = new Button("bt2");
panel3.add(bt1);
panel3.add(bt2);
//下边
Button east1 = new Button("East");//按钮
Button west1 = new Button("West");//按钮
panel2.add(east1,BorderLayout.EAST);
panel2.add(panel4,BorderLayout.CENTER);
panel2.add(west1,BorderLayout.WEST);
panel4.setLayout(new GridLayout(2,2));
Button btn1 = new Button("bt1");
Button btn2 = new Button("bt2");
Button btn3 = new Button("bt3");
Button btn4 = new Button("bt4");
panel4.add(btn1);
panel4.add(btn2);
panel4.add(btn3);
panel4.add(btn4);
frame.setSize(200,200);
frame.setVisible(true);
}
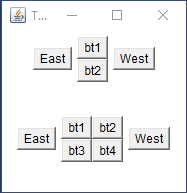
老师:
分析过程:
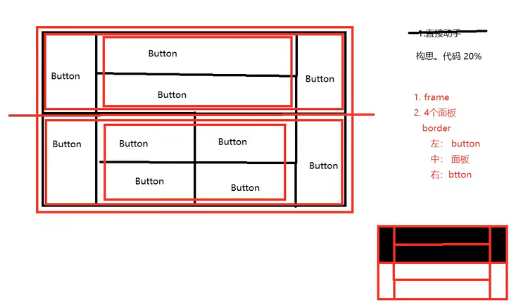
代码实现:
package com.Zhang.lesson01;
import java.awt.*;
public class ExDemo {
public static void main(String[] args) {
//总的Frame窗
Frame frame = new Frame();
frame.setSize(400,300);
frame.setLocation(300,400);
frame.setBackground(Color.BLACK);
frame.setVisible(true);
frame.setLayout(new GridLayout(2,1));
//4个面板
Panel p1 = new Panel(new BorderLayout());
Panel p2 = new Panel(new GridLayout(2,1));
Panel p3= new Panel(new BorderLayout());
Panel p4 = new Panel(new GridLayout(2,2));
//上面
p1.add(new Button("East-1"),BorderLayout.EAST);
p1.add(new Button("West-1"),BorderLayout.WEST);
p2.add(new Button("p2_btn_1"));
p2.add(new Button("p2_btn_2"));
p1.add(p2,BorderLayout.CENTER);
//下面
p3.add(new Button("East-2"),BorderLayout.EAST);
p3.add(new Button("West-2"),BorderLayout.WEST);
//中间四个
for (int i = 0; i < 4; i++) {
p4.add(new Button("for_"+i));
}
p3.add(p4,BorderLayout.CENTER);
frame.add(p1);
frame.add(p3);
}
}
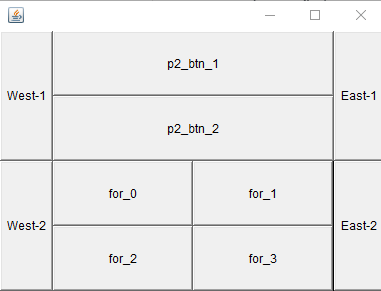
总结:
Frame是一个顶级窗口
Panel无法单独显示,必须添加到某个容器中
布局管理器
1、流式
2、东西南北中
3、表格
4、大小,定位,背景颜色,可见性,监听!
2、4事件监听
事件监听:当某个事情发生的时候,干什么?
package com.Zhang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestActionTwo {
public static void main(String[] args) {
//两个按钮,实现同一个监听
//开始 停止
Frame frame = new Frame("开始-停止");
Button button1 = new Button("start");
Button button2 = new Button("stop");
//可以显示的定义会返回的命令,如果不显示定义,则会走默认的值!
//可以多个按钮只写一个监听类
button2.setActionCommand("button2-stop");
Mymonitor mymonitor = new Mymonitor();
button1.addActionListener(mymonitor);
button2.addActionListener(mymonitor);
frame.add(button1,BorderLayout.NORTH);
frame.add(button2,BorderLayout.SOUTH);
frame.pack();
frame.setVisible(true);
}
}
class Mymonitor implements ActionListener{
@Override
public void actionPerformed(ActionEvent e) {
//e.getActionCommand()获得按钮的信息
System.out.println("按钮被点击了:msg=>"+ e.getActionCommand());
}
}
多个按钮共享一个事件。
2、5输入框TextField监听
class MyFrame extends Frame {
public MyFrame(){//构造器
TextField textField = new TextField();//写单行文本
add(textField);
//监听这个文本框输入的文字
MyActionListener2 myActionListener2 = new MyActionListener2();
//按下enter,就会触发这个输入框的事件
textField.addActionListener(myActionListener2);
//设置替换编码
textField.setEchoChar('*');
pack();
setVisible(true);
}
}
class MyActionListener2 implements ActionListener {
@Override
public void actionPerformed(ActionEvent e) {
TextField field=(TextField) e.getSource();//获得一些资源,返回的一个对象
System.out.println(field.getText());//获得输入框中的文本
field.setText("");//null 回车清空文本框
}
}
注:JAVA中的awt,swing的事件处理里面,e是指一个事件,用e.getSource()可以获得此事件,但getSource()返回的是Object类型(保持方法的通用性),所以对相应的组件产生的事件项进行强制转换,如:(TextField) e.getSource(),这样就可用TextField对象的方法了。
2、6 简易计算器,组合+内部类回顾复习
oop原则: 组合,大于继承!
class A extends B{
}
class A{
public B b;
简易计算器
package com.Zhang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
//简易计算器
public class TestCalc {
public static void main(String[] args) {
new Calculator();
}
}
//计算器类
class Calculator extends Frame {
public Calculator(){
//组件
//3个文本框
TextField num1 = new TextField(10);//字符数
TextField num2 = new TextField(10);//字符数
TextField num3 = new TextField(20);//字符数
//1个按钮
Button button = new Button("=");
button.addActionListener(new MyCalculatorListen(num1,num2,num3));
//1个标签
Label label = new Label("+");
//布局,流式
setLayout(new FlowLayout());
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
}
}
//监听器类
class MyCalculatorListen implements ActionListener{
//获取三个变量
private TextField num1,num2,num3;
//有参构造
public MyCalculatorListen(TextField num1, TextField num2,TextField num3){
this.num1=num1;
this.num2=num2;
this.num3=num3;
}
@Override
public void actionPerformed(ActionEvent e) {
//1、获得加数和被加数
int n1=Integer.parseInt(num1.getText());
int n2=Integer.parseInt(num2.getText());
//2、将这个值加法运算后放到第三个框
num3.setText(""+(n1+n2));//暴力拼接字符串
//num3.setText((n1+n2)+"");
//3、清除前两个框
num1.setText("");
num2.setText("");
}
}
注:Integer.parseInt()
完全改造为面向对象写法。
内部类:
更好的包装
package com.Zhang.lesson02;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class TestCalc {
public static void main(String[] args) {
new Calculator().loadFrame();//直接调用loadFrame方法
}
}
//计算器类
class Calculator extends Frame {
//属性
TextField num1,num2,num3;
//方法
public void loadFrame(){
//三个文本框
num1 = new TextField(10);//最多写多少字符
num2 = new TextField(10);
num3 = new TextField(20);//比前两个长
//一个按钮
Button button = new Button("=");
button.addActionListener(new MyCalculatorListener());
//一个标签 展示+
Label label = new Label("+");
//布局 流式
setLayout(new FlowLayout());
add(num1);
add(label);
add(num2);
add(button);
add(num3);
pack();
setVisible(true);
}
//监听器
//内部类最大的好处,就是可以畅通无阻的访问外部类
private class MyCalculatorListener implements ActionListener {
//在一个类中组合另一个类
Calculator calculator =null;
//没必要依次获取三个变量,直接把计算器类拿过来
@Override
public void actionPerformed(ActionEvent e) {
//获得加数和被加数
int i1 = Integer.parseInt(num1.getText());
int i2 = Integer.parseInt(num2.getText());
//相加后,放到第三个框
num3.setText(""+(i1+i2));
//清除前两个框
num1.setText("");
num2.setText("");
}
}
}
2、7 画笔
class MyPaint extends Frame {
public void LoadFrame(){
setBounds(200,200,600,400);
setVisible(true);
}
//画笔
@Override
public void paint(Graphics g) {
//画笔,需要有颜色,可以画画
g.setColor(Color.blue);
//g.drawOval(100,100,100,100);
g.fillOval(100,100,100,100);//实心的圆
g.setColor(Color.GREEN);
g.fillRect(150,200,200,200);
//养成习惯,画笔用完将他还原到最初的颜色(黑色)
}
}
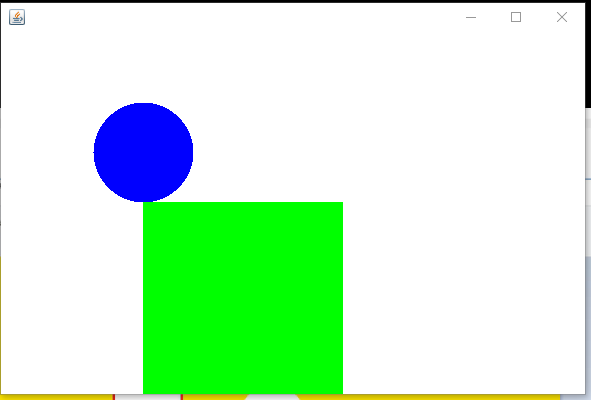
2、8鼠标监听
目的:想要实现鼠标画画!
package com.Zhang.lesson03;
import java.awt.*;
import java.awt.event.MouseAdapter;
import java.awt.event.MouseEvent;
import java.awt.event.MouseListener;
import java.util.ArrayList;
import java.util.Iterator;
//鼠标监听事件
public class TestMouseListener {
public static void main(String[] args) {
new MyFrame("画图");
}
}
//自己的类
class MyFrame extends Frame {
//画画需要画笔,需要监听鼠标当前的位置,需要集合来存储这个点
ArrayList points;
public MyFrame(String title){
super(title);
setBounds(200,200,400,300);//画板大小
//存鼠标点击的点
points=new ArrayList<>();
setVisible(true);
//鼠标监听器针对窗口。
this.addMouseListener(new MyMouseListener());
}
@Override
public void paint(Graphics g) {
//画画,监听鼠标的事件
Iterator iterator = points.iterator();
while(iterator.hasNext()){
Point point=(Point) iterator.next();
g.setColor(Color.BLUE);
g.fillOval(point.x,point.y,10,10);//位置,大小
}
}
//添加一个点到界面上面
public void addPaint(Point point){
points.add(point);
}
//适配器模式
private class MyMouseListener extends MouseAdapter{
//鼠标点击 按下 弹起 按住不放
@Override
public void mousePressed(MouseEvent e) {
MyFrame myFrame=(MyFrame) e.getSource();
//这里点击的时候,就会在界面上产生一个点!
//这个点就是鼠标的点
myFrame.addPaint(new Point(e.getX(),e.getY()));
//每次点击鼠标都需要重新画一遍
myFrame.repaint();//刷新
}
}
}
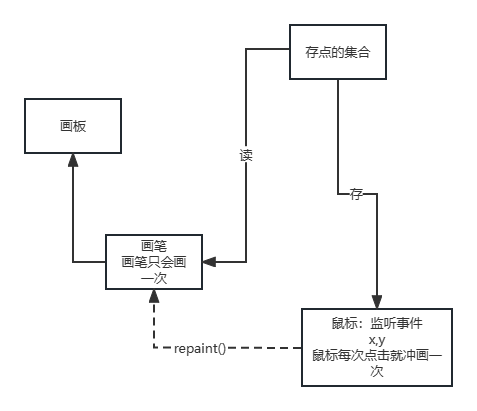
注:Java Iterator(迭代器)
iterator不是一个集合,它是一种用于访问集合的方法,可用于迭代ArrayList和HashSet等集合。
iterator:属于迭代输出,基本的操作原理:是不断的判断是否有下一个元素,有的话,则直接输出。
2、9窗口监听
package com.Zhang.lesson03;
import java.awt.*;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.awt.event.WindowListener;
public class TestWindow {
public static void main(String[] args) {
new WindowFrame();
}
}
class WindowFrame extends Frame {
public WindowFrame() {
setBackground(Color.BLUE);
setBounds(100, 100, 200, 200);
setVisible(true);
//addWindowListener(new MyWindowListener());
this.addWindowListener(
//匿名内部类
new WindowAdapter() {
//最常见的两个方法,激活和关闭
@Override
public void windowClosing(WindowEvent e) {//正在关闭中
System.out.println("windowClosing");
System.exit(0);
}
@Override
public void windowActivated(WindowEvent e) {
WindowFrame source=(WindowFrame) e.getSource();
source.setTitle("被激活了");
System.out.println("windowActivated");
}
});
}
}
2、10键盘监听
package com.Exercise.lesson03;
import java.awt.*;
import java.awt.event.KeyAdapter;
import java.awt.event.KeyEvent;
//键盘监听
public class TestKeyboardListener {
public static void main(String[] args) {
new KeyboardFrame();
}
}
class KeyboardFrame extends Frame {
public KeyboardFrame() {
setBounds(100,100,200,200);
setVisible(true);
this.addKeyListener(new KeyAdapter() {
//按下键盘,出发事件
@Override
public void keyPressed(KeyEvent e) {
//获得按下的键是哪一个,当前的码
int keycode=e.getKeyCode();
System.out.println(keycode);
//可以根据不同的操作,产生不同的结果
if(keycode==KeyEvent.VK_ENTER){//如果按下回车键,则输出回车
System.out.println("Enter");
}
}
});
}
}
3、Swing
3、1 窗口和面板
package com.Zhang.lesson04;
import javax.swing.*;
import java.awt.*;
public class JFrameDemo {
//init() 初始化
public void init(){
//顶级窗口
JFrame frame = new JFrame("这是一个JFrame窗口");
frame.setVisible(true);
frame.setBounds(100,100,200,200);
frame.setBackground(Color.BLUE);
//设置文字JLabel
JLabel label =new JLabel("欢迎来到狂神说java系列节目");
frame.add(label);
//容器实例化
//关闭事件
frame.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
//建立一个窗口
new JFrameDemo().init();
}
}
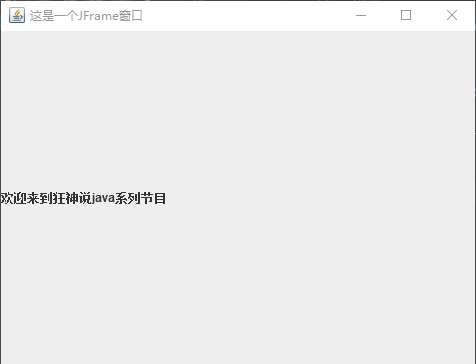
无颜色显示(直接设置没有颜色,需要一个容器)
标签居中
package com.Zhang.lesson04;
import javax.swing.*;
import java.awt.*;
public class JFrameDemo02 {
public static void main(String[] args) {
new MyJFrame2().init();
}
}
class MyJFrame2 extends JFrame {
public void init() {
this.setVisible(true);
this.setBounds(100,100,200,200);
JLabel label =new JLabel("欢迎来到狂神说java系列节目");
this.add(label);
//让文本标签居中
label.setHorizontalAlignment(SwingConstants.CENTER);//设置水平对齐
//获得一个容器
Container container = this.getContentPane();
container.setBackground(Color.green);
}
}
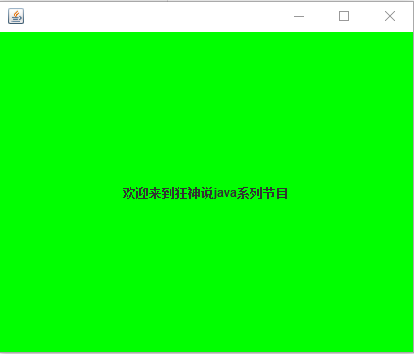
3、2 弹窗
JDialog,用来被弹出,默认有关闭事件。
package com.Zhang.lesson04;
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
//主窗口
public class DialogDemo extends JFrame {
public DialogDemo(){
this.setVisible(true);
this.setSize(700,500);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
//JFrame放东西需要一个容器
Container container = getContentPane();
//绝对布局,自己去决定放在什么位置
container.setLayout(null);
//按钮
JButton button = new JButton("欢迎来到王者荣耀");//创建
button.setBounds(30,30,200,50);
container.add(button);
//点击这个按钮的时候弹出一个弹窗
button.addActionListener(new ActionListener() {//匿名内部类 监听器
@Override
public void actionPerformed(ActionEvent e) {
//弹窗
new MyDialogDemo().setVisible(true);
}
});
}
public static void main(String[] args) {
new DialogDemo();
}
}
//弹窗的窗口
class MyDialogDemo extends JDialog{
public MyDialogDemo() {
this.setTitle("Dialog");//弹出的窗口的标签
this.setBounds(100,100,500,500);
JLabel jLabel = new JLabel("欢迎来到java狂神说");
this.add(jLabel);
jLabel.setHorizontalAlignment(SwingConstants.CENTER);//设置水平对齐
Container container = this.getContentPane();
container.setBackground(Color.green);
this.setVisible(true);
}
}
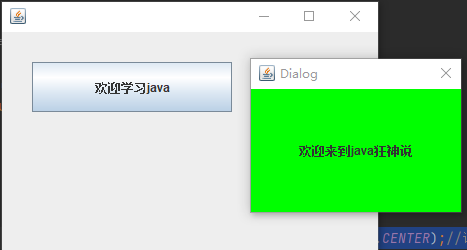
3、3标签
label
new JLabel("文字“);
图标icon
package com.Zhang.lesson04;
import javax.swing.*;
import java.awt.*;
//图标,需要实现类,Frame继承
public class IconDemo extends JFrame implements Icon {
//属性
private int width;
private int height;
public IconDemo(int width,int height) {
this.width=width;
this.height=height;
}
public IconDemo() {
}
public void init(){
//new一个图标类
IconDemo iconDemo = new IconDemo(15, 15);
//图标放在标签上,也可以放在按钮上!
JLabel label = new JLabel("icontest", iconDemo, SwingConstants.CENTER);
//JButton button = new JButton("iconbutton",iconDemo); container.add(label);
Container contentPane = getContentPane();//得到一个容器
contentPane.add(label);
this.setVisible(true);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);//关闭窗口
}
public static void main(String[] args) {
new IconDemo().init();//主函数,调用init()方法
}
//重写方法
@Override
public void paintIcon(Component c, Graphics g, int x, int y) {
g.fillOval(x,y,width,height);//画图标方法
}
@Override
public int getIconWidth() {
return this.width;
}
@Override
public int getIconHeight() {
return this.height;
}
}
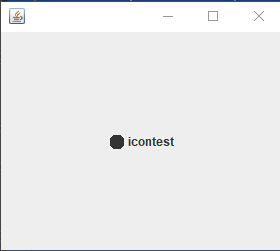
图片Icon
package com.Zhang.lesson04;
import javax.swing.*;
import java.awt.*;
import java.net.URL;
public class ImageIconDemo extends JFrame {
public ImageIconDemo() {//构造方法
//获取图片的地址
JLabel label = new JLabel("ImageIcon");
URL url = ImageIconDemo.class.getResource("R-C.jpg");//获取当前这个类同级下的资源
ImageIcon imageIcon = new ImageIcon(url);//命名不要冲突了
label.setIcon(imageIcon);
label.setHorizontalAlignment(SwingConstants.CENTER);
Container contentPane = getContentPane();
contentPane.add(label);
setVisible(true);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
setBounds(100,100,200,200);
}
public static void main(String[] args) {
new ImageIconDemo();
}
}
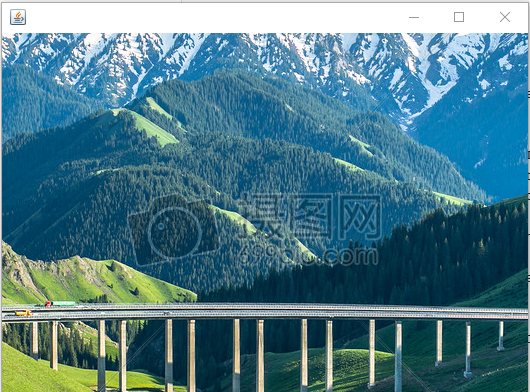
3、4 面板
package com.Zhang.lesson05;
import javax.swing.*;
import java.awt.*;
public class JPanelDemo extends JFrame {
public JPanelDemo() {
//所有的东西放在容器里面
Container contentPane = this.getContentPane();
contentPane.setLayout(new GridLayout(2,1,10,10));//后面的参数的意思,间距
JPanel Panel1 = new JPanel(new GridLayout(1,3));
JPanel Panel2 = new JPanel(new GridLayout(1,2));
JPanel Panel3 = new JPanel(new GridLayout(2,1));
JPanel Panel4 = new JPanel(new GridLayout(3,2));
Panel1.add(new Button("1"));
Panel1.add(new Button("1"));
Panel1.add(new Button("1"));
Panel2.add(new Button("2"));
Panel2.add(new Button("2"));
Panel3.add(new Button("3"));
Panel3.add(new Button("3"));
Panel4.add(new Button("4"));
Panel4.add(new Button("4"));
Panel4.add(new Button("4"));
Panel4.add(new Button("4"));
Panel4.add(new Button("4"));
Panel4.add(new Button("4"));
contentPane.add(Panel1);
contentPane.add(Panel2);
contentPane.add(Panel3);
contentPane.add(Panel4);
this.setVisible(true);
this.setSize(500,500);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new JPanelDemo();
}
}
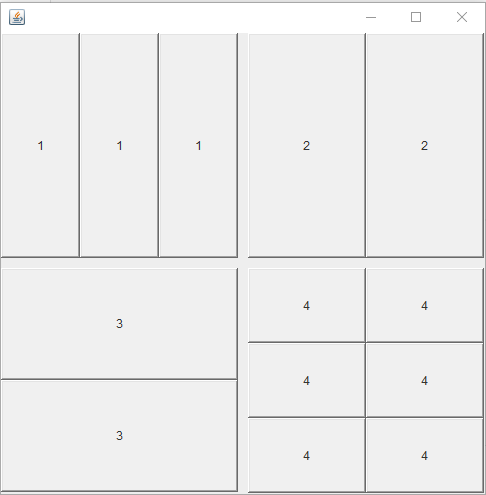
JScrollPanel
文本域
package com.Zhang.lesson05;
import javax.swing.*;
import java.awt.*;
public class JScrollDemo extends JFrame {
public JScrollDemo() {
//new一个容器
Container container = this.getContentPane();
//文本域
JTextArea textArea = new JTextArea(20, 50);
textArea.setText("欢迎学习java");
//Scroll面板
JScrollPane scrollPane = new JScrollPane(textArea);
container.add(scrollPane);
this.setVisible(true);
this.setBounds(100,100,300,150);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new JScrollDemo();
}
}
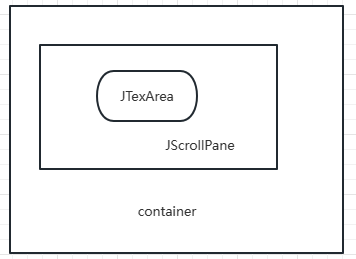
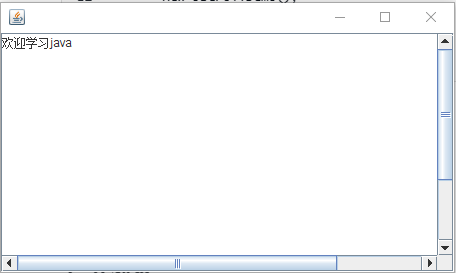
3、5按钮
图片按钮
package com.Zhang.lesson05;
import javax.swing.*;
import java.awt.*;
import java.net.URL;
public class JButtonDemo01 extends JFrame {
public JButtonDemo01(){
Container container = this.getContentPane();
//将一个图片变成一个图标
URL url = JButtonDemo01.class.getResource("R-C.jpg");
Icon icon = new ImageIcon(url);
//把这个图标放在按钮上
JButton button = new JButton();
button.setIcon(icon);
button.setToolTipText("图片按钮");
container.add(button);
this.setVisible(true);
this.setSize(500,300);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new JButtonDemo01();
}
}
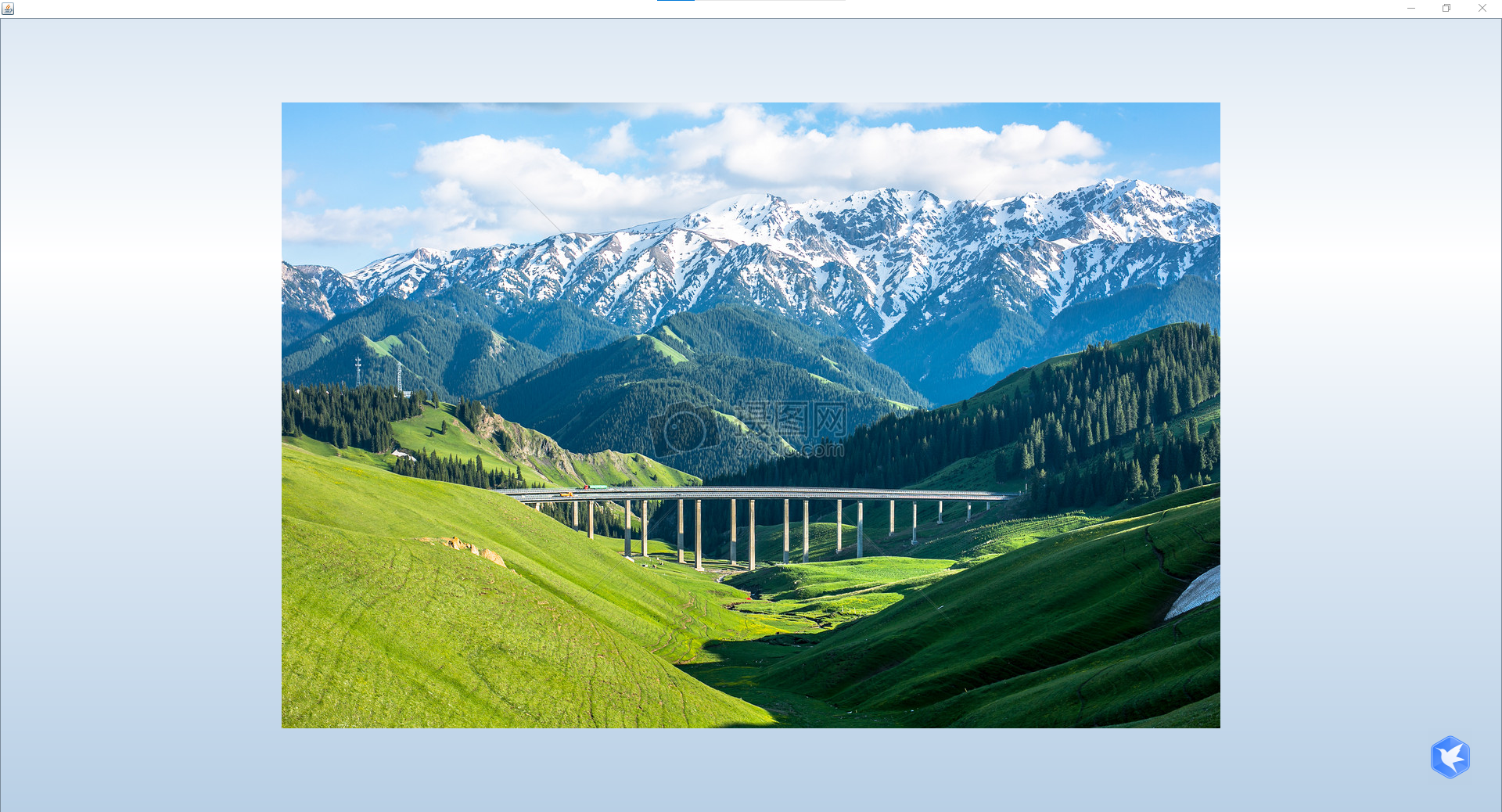
单选按钮
package com.Zhang.lesson05;
import javax.swing.*;
import java.awt.*;
import java.net.URL;
public class JButtonDemo02 extends JFrame{
public JButtonDemo02(){
Container container = this.getContentPane();
//将一个图片变成一个图标
URL url = JButtonDemo01.class.getResource("R-C.jpg");
Icon icon = new ImageIcon(url);
//单选框
JRadioButton radioButton01 = new JRadioButton("JRadioButton01");
JRadioButton radioButton02 = new JRadioButton("JRadioButton02");
JRadioButton radioButton03 = new JRadioButton("JRadioButton03");
//由于单选框只能选择一个,分组,一个组中只能选择一个
ButtonGroup group = new ButtonGroup();
group.add(radioButton01);
group.add(radioButton02);
group.add(radioButton03);
container.add(radioButton01,BorderLayout.CENTER);
container.add(radioButton02,BorderLayout.NORTH);
container.add(radioButton03,BorderLayout.SOUTH);
this.setVisible(true);
this.setSize(500,300);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new JButtonDemo02();
}
}
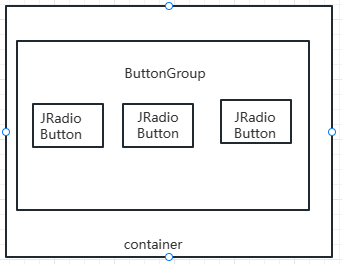
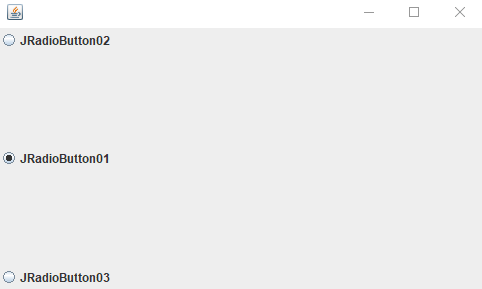
复选按钮
package com.Zhang.lesson05;
import javax.swing.*;
import java.awt.*;
import java.net.URL;
public class JButtonDemo03 extends JFrame{
public JButtonDemo03(){
Container container = this.getContentPane();
//将一个图片变成一个图标
URL url = JButtonDemo01.class.getResource("R-C.jpg");
Icon icon = new ImageIcon(url);
//多选框
JCheckBox checkBox01 = new JCheckBox("checkBox01");
JCheckBox checkBox02 = new JCheckBox("checkBox02");
container.add(checkBox01,BorderLayout.NORTH);
container.add(checkBox02,BorderLayout.SOUTH);
this.setVisible(true);
this.setSize(500,300);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new JButtonDemo03();
}
}
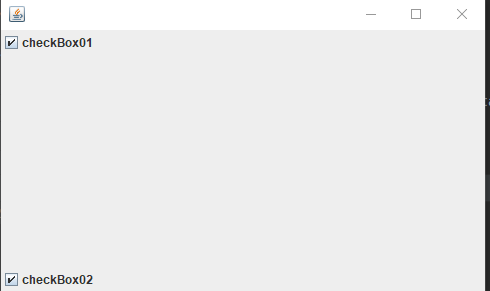
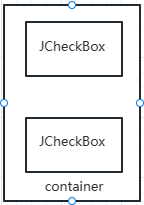
3、6 列表
下拉框
package com.Zhang.lesson06;
import javax.swing.*;
import java.awt.*;
public class TestComboboxDemo01 extends JFrame {
public TestComboboxDemo01() {
Container container = this.getContentPane();
JComboBox status = new JComboBox();
status.addItem(null);
status.addItem("正在上映");
status.addItem("已下架");
status.addItem("即将上映");
container.add(status);
this.setVisible(true);
this.setSize(500,350);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestComboboxDemo01();
}
}
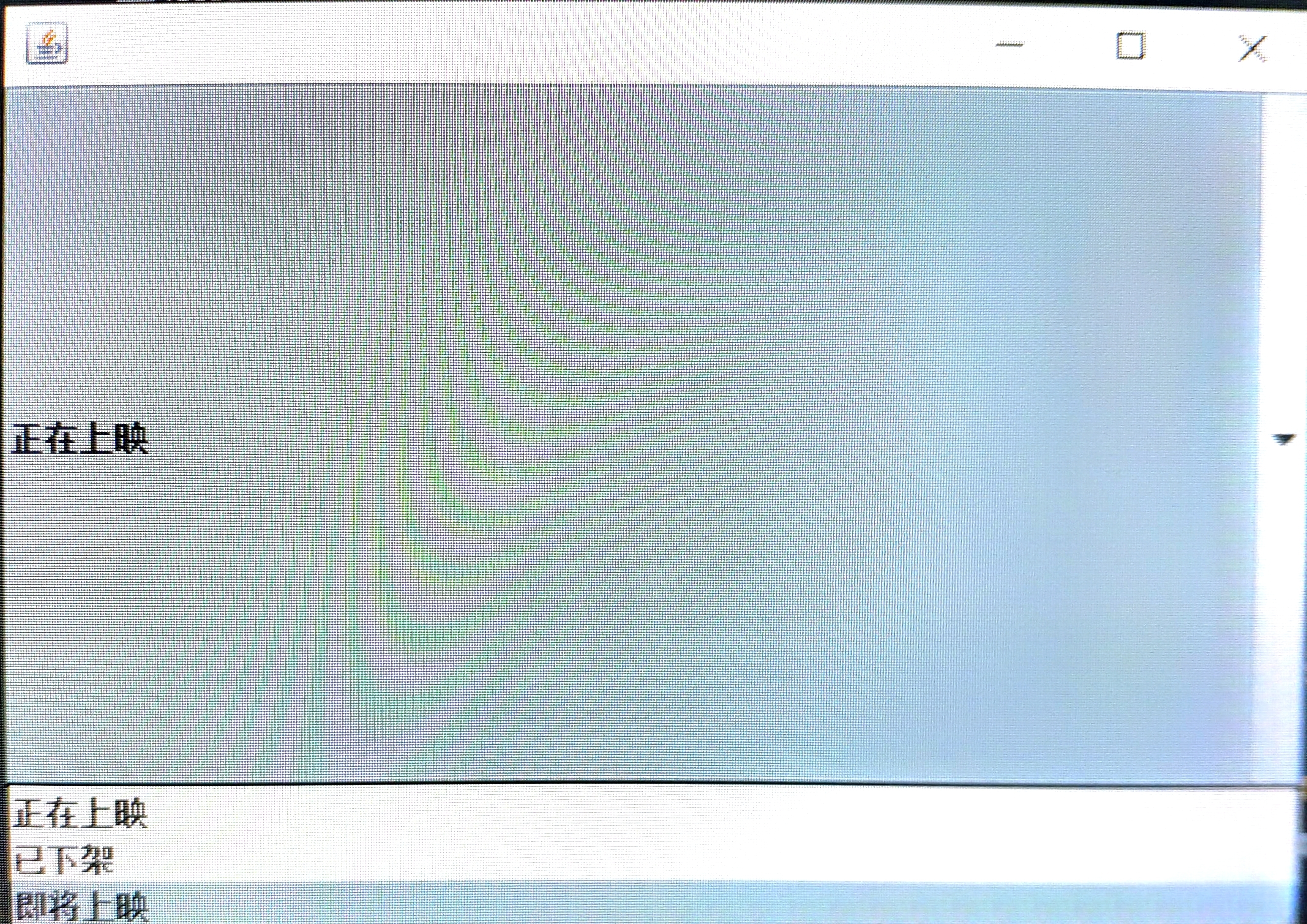
列表框
静态添加
public class TestComboBoxDemo02 extends JFrame {
public TestComboBoxDemo02(){
Container container = this.getContentPane();
//生成列表内容,静态添加
String[] contents={"1","2","3"};
JList list = new JList(contents);
container.add(list);
this.setVisible(true);
this.setSize(500,300);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestComboBoxDemo02();
}
}
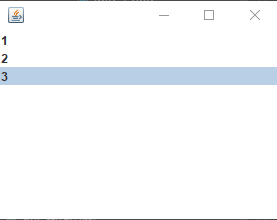
public class TestComboBoxDemo03 extends JFrame {
public TestComboBoxDemo03() {
Container container = this.getContentPane();
Vector contents = new Vector();//动态添加列表
JList jList = new JList(contents);
contents.add("张三");
contents.add("李四");
contents.add("王五");
container.add(jList);
this.setVisible(true);
this.setSize(500,300);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestComboBoxDemo03();
}
}
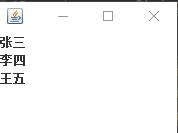
应用场景:
下拉框:选择地区,或者一些单个选项
列表:展示信息,一般是动态扩容。
3、7 文本框
文本框
package com.Zhang.lesson06;
import javax.swing.*;
import java.awt.*;
import java.util.Vector;
public class TestTextDemo01 extends JFrame{
public TestTextDemo01() {
Container container = this.getContentPane();
JTextField textField1 = new JTextField("hello");
JTextField textField2 = new JTextField("world",20);//最多放20个字符
container.add(textField1,BorderLayout.NORTH);
container.add(textField2,BorderLayout.SOUTH);
this.setVisible(true);
this.setSize(500,350);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestTextDemo01();
}
}
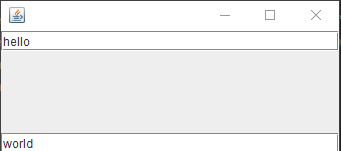
密码框
package com.Zhang.lesson06;
import javax.swing.*;
import java.awt.*;
public class TestTextDemo02 extends JFrame {
public TestTextDemo02() {
Container container = this.getContentPane();
JPasswordField passwordField = new JPasswordField();
passwordField.setEchoChar('*');
container.add(passwordField);
this.setVisible(true);
this.setSize(500,350);
this.setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
new TestTextDemo02();
}
}
随便输入,显示的文本是密码
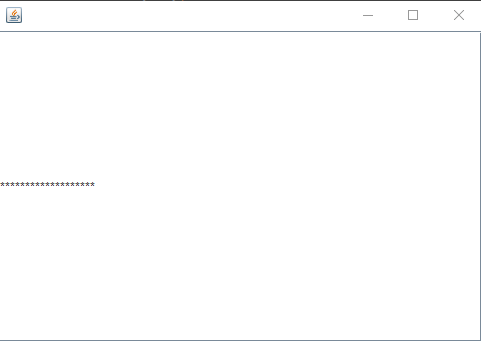
文本域
JTextArea textArea = new JTextArea(20, 50);
textArea.setText("欢迎学习java");