Java 操作
1.pom 依赖
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>2.4.17</version>
</dependency>
2.resources 文件
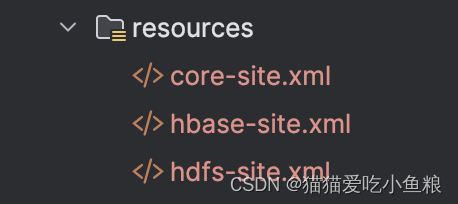
3.同步客户端代码示例
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.*;
import org.apache.hadoop.hbase.client.*;
import org.apache.hadoop.hbase.util.Bytes;
import java.io.IOException;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class HbaseUtilSyncTest {
public Connection getConnection(String zkHost
, String zkPort
, String scanTimeOut
, String threadMax
, String threadCore
, String rpcTimeout) throws IOException {
final Configuration configuration = HBaseConfiguration.create();
configuration.set("hbase.zookeeper.quorum", zkHost);
configuration.set("hbase.zookeeper.property.clientPort", zkPort);
configuration.set("hbase.client.scanner.timeout.period", scanTimeOut);
configuration.set("hbase.hconnection.threads.max", threadMax);
configuration.set("hbase.hconnection.threads.core", threadCore);
configuration.set("hbase.rpc.timeout", rpcTimeout);
return ConnectionFactory.createConnection(configuration);
}
//查询表是否存在,默认使用 default 命名空间,其他命名空间需要传入{namespace:tableName}
public Boolean isTableExist(Connection connection, String tableName) throws IOException {
final HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
return admin.tableExists(TableName.valueOf(tableName));
}
public void createTable(Connection connection, String tableName, String... columnFamilys) throws IOException {
final HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
if (columnFamilys.length <= 0) {
System.out.println("请先设置列簇信息");
} else if (admin.tableExists(TableName.valueOf(tableName))) {
System.out.println("表 " + tableName + " 已经存在。");
} else {
TableDescriptorBuilder builder = TableDescriptorBuilder.newBuilder(TableName.valueOf(tableName));
List<ColumnFamilyDescriptor> families = new ArrayList<>();
for (String columnFamily : columnFamilys) {
final ColumnFamilyDescriptorBuilder cfdBuilder = ColumnFamilyDescriptorBuilder.newBuilder(ColumnFamilyDescriptorBuilder.of(columnFamily));
final ColumnFamilyDescriptor cfd = cfdBuilder.setMaxVersions(10).setInMemory(true).setBlocksize(8 * 1024)
.setScope(HConstants.REPLICATION_SCOPE_LOCAL).build();
families.add(cfd);
}
final TableDescriptor tableDescriptor = builder.setColumnFamilies(families).build();
admin.createTable(tableDescriptor);
}
}
public void dropTable(Connection connection, String tableName) throws IOException {
final HBaseAdmin admin = (HBaseAdmin) connection.getAdmin();
if (admin.tableExists(TableName.valueOf(tableName))) {
admin.disableTable(TableName.valueOf(tableName));
admin.deleteTable(TableName.valueOf(tableName));
System.out.println("表 " + tableName + " 成功删除");
} else {
System.out.println("表 " + tableName + " 不存在");
}
}
public void addOneRowData(Connection connection
, String tableName
, String rowKey
, String columnFamily
, String column
, String value) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
//构建Put指令,rowKey 必传
Put put = new Put(Bytes.toBytes(rowKey));
//指定版本时间戳
put.addColumn(Bytes.toBytes(columnFamily), Bytes.toBytes(column), System.currentTimeMillis(), Bytes.toBytes(value));
// put.addColumn(Bytes.toBytes(columnFamily), Bytes.toBytes(column), Bytes.toBytes(value));
table.put(put);
//表操作之后 close,关闭线程
table.close();
}
public void addMultiRowData(Connection connection
, String tableName
, List<Put> data) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
table.put(data);
table.close();
}
public void deleteMultiRow(Connection connection, String tableName, String... rows) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
List<Delete> deletes = new ArrayList<>();
for (String row : rows) {
Delete delete = new Delete(Bytes.toBytes(row));
deletes.add(delete);
}
if (!deletes.isEmpty()) {
table.delete(deletes);
System.out.println("表数据删除成功");
}
table.close();
}
public void getRowData(Connection connection, String tableName, String row) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
// 按照 rowKey 查询
Get get = new Get(Bytes.toBytes(row));
final Result result = table.get(get);
if (result.isEmpty()) {
System.out.println(row + " 的 rowKey 在表中无数据");
} else {
for (Cell cell : result.rawCells()) {
System.out.println("rowKey:" + Bytes.toString(CellUtil.cloneRow(cell)));
System.out.println(" 列簇:" + Bytes.toString(CellUtil.cloneFamily(cell)));
System.out.println(" 列:" + Bytes.toString(CellUtil.cloneQualifier(cell)));
System.out.println(" 值:" + Bytes.toString(CellUtil.cloneValue(cell)));
System.out.println(" 版本时间戳:" + cell.getTimestamp());
System.out.println("==========================");
}
}
table.close();
}
public void scanRowData(Connection connection, String tableName, String startRow, String stopRow) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
Scan scan = new Scan();
if (null != startRow && !startRow.isEmpty()) {
scan.withStartRow(Bytes.toBytes(startRow));
}
if (null != stopRow && !stopRow.isEmpty()) {
//包含最后一行数据。 默认是左开右闭。
scan.withStopRow(Bytes.toBytes(stopRow), true);
}
ResultScanner scanner = table.getScanner(scan);
for (Result result : scanner) {
for (Cell cell : result.rawCells()) {
System.out.println("rowKey:" + Bytes.toString(CellUtil.cloneRow(cell)));
System.out.println(" 列簇:" + Bytes.toString(CellUtil.cloneFamily(cell)));
System.out.println(" 列:" + Bytes.toString(CellUtil.cloneQualifier(cell)));
System.out.println(" 值:" + Bytes.toString(CellUtil.cloneValue(cell)));
System.out.println(" 版本时间戳:" + cell.getTimestamp());
System.out.println("==========================");
}
System.out.println("-----------------------");
}
table.close();
}
public HashMap<String, Boolean> getBatchRowData(Connection connection, String tableName, List<String> row) throws IOException {
Table table = connection.getTable(TableName.valueOf(tableName));
ArrayList<Get> gets = new ArrayList<>();
for (String value : row) {
Get get = new Get(Bytes.toBytes(value));
gets.add(get);
}
Result[] results = table.get(gets);
HashMap<String, Boolean> res = new HashMap<>();
for (Result result : results) {
if (!result.isEmpty()) {
for (Cell cell : result.rawCells()) {
res.put(Bytes.toString(CellUtil.cloneRow(cell)), true);
}
}
}
table.close();
return res;
}
public static void main(String[] args) throws IOException {
HbaseUtilSyncTest baseDemo = new HbaseUtilSyncTest();
final Connection connection = baseDemo.getConnection("host"
, "port"
, "10000"
, "32"
, "8"
, "60000");
String tableName = "my_ns:Student";
String basicCF = "basicinfo";
String studyCF = "studyinfo";
//判断表是否存在
System.out.println(tableName + "=表是否存在=>" + baseDemo.isTableExist(connection, tableName));
//建表
baseDemo.createTable(connection, tableName, "basicinfo", "studyinfo");
//删除表
baseDemo.dropTable(connection, tableName);
//插入数据
System.out.println("插入一条数据");
baseDemo.addOneRowData(connection, tableName, "1001", basicCF, "age", "10");
baseDemo.addOneRowData(connection, tableName, "1001", basicCF, "name", "roy");
baseDemo.addOneRowData(connection, tableName, "1001", studyCF, "grade", "100");
baseDemo.addOneRowData(connection, tableName, "1002", basicCF, "age", "11");
baseDemo.addOneRowData(connection, tableName, "1002", basicCF, "name", "yula");
baseDemo.addOneRowData(connection, tableName, "1002", studyCF, "grade", "99");
System.out.println("插入批量数据");
ArrayList<Put> puts = new ArrayList<>();
puts.add(new Put(Bytes.toBytes("1003")).addColumn(Bytes.toBytes(basicCF), Bytes.toBytes("age"), Bytes.toBytes("12")));
puts.add(new Put(Bytes.toBytes("1003")).addColumn(Bytes.toBytes(basicCF), Bytes.toBytes("name"), Bytes.toBytes("sophia")));
puts.add(new Put(Bytes.toBytes("1003")).addColumn(Bytes.toBytes(studyCF), Bytes.toBytes("grade"), Bytes.toBytes("120")));
baseDemo.addMultiRowData(connection, tableName, puts);
//删除数据
baseDemo.deleteMultiRow(connection, tableName, "1001", "1003");
//根据 rowKey 查询数据
System.out.println("读取某一行信息");
baseDemo.getRowData(connection, tableName, "1004");
System.out.println("读取批量信息");
ArrayList<String> rows = new ArrayList<>();
rows.add("1001");
rows.add("1004");
HashMap<String, Boolean> batchRowData = baseDemo.getBatchRowData(connection, tableName, rows);
for (Map.Entry<String, Boolean> entry : batchRowData.entrySet()) {
System.out.println("key=" + entry.getKey() + ",value=" + entry.getValue());
}
connection.close();
}
}