是线性表,有先后顺序的线性表,但是限制操作数据的位置,限制只能在线性表的一端进行操作(插入和删除),其他任意位置都不允许操作,就把这种特殊的线性表叫做栈
特殊线性表:允许操作的一段叫做栈顶,不能操作的一端叫做栈底;没有数据叫做空栈
栈:就是一种只能在
一端进行存取
的线性表,遵循
先进后出
原则的数据结构,对于栈而言,只能操作栈顶元素,不能越过栈顶元素操作其他元素;如果操作完栈顶元素(插入、删除),则会有的栈顶元素栈的每次操作都是操作最后一个能操作的元素
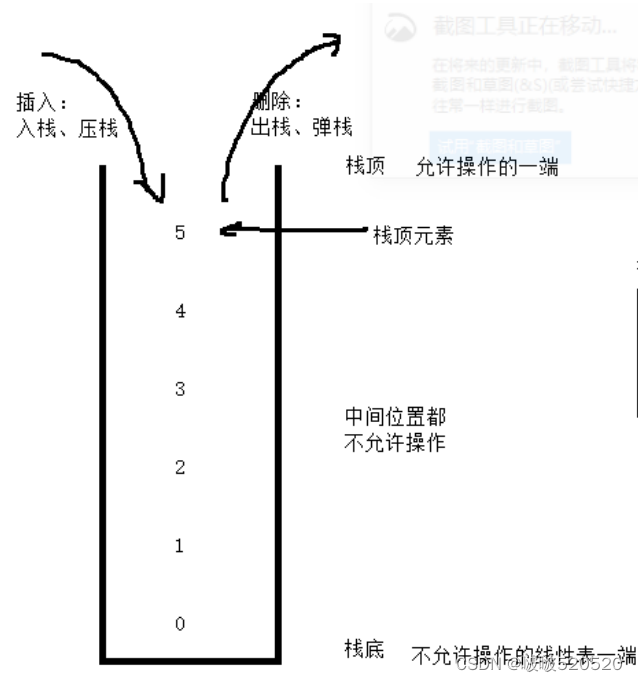
顺序栈:(顺序表,限制操作位置的顺序表)
逻辑结构:栈
o
存储结构:顺序存储
申请一段连续的空间来存储数据,限制只能在存储空间一端进行操作存取
顺序存储有两个端点(a0, an
)
,
选择不能操作的一端,栈顶就从栈底位置开始移动,栈顶指向最后一个元素的位置,当插入时,栈顶移动到最新(下一个)数据元素位置,当删除时,栈顶移动到最新 (上一个)数据元素位置
顺序栈:就是顺序表,规定栈顶为,最后一个元素位置,每次都只能操作顺序最后一个元素(删除最后一个元素、插入最后一个元素〉
链式栈:(链表,限制操作位置的链表)
逻辑结构:栈
存储结构:链式存储
每个元素申请空间存储地址来表示先后顺序,需要存储元素时才申请空间,有第一个元素以及最后
一个元素两端,限制只能在一端进行操作
头节点的next
存栈顶元素,每次都在头节点位置进行操作,头节点的
next
就是栈顶位置,每个栈顶元素存储上一个栈顶元素的地址;节点的next
为
NULL
就是栈底元素
链式楼:就是链表,规定栈顶为,头节点的next,每次操作都只能操作(插入、删除)第一个元素
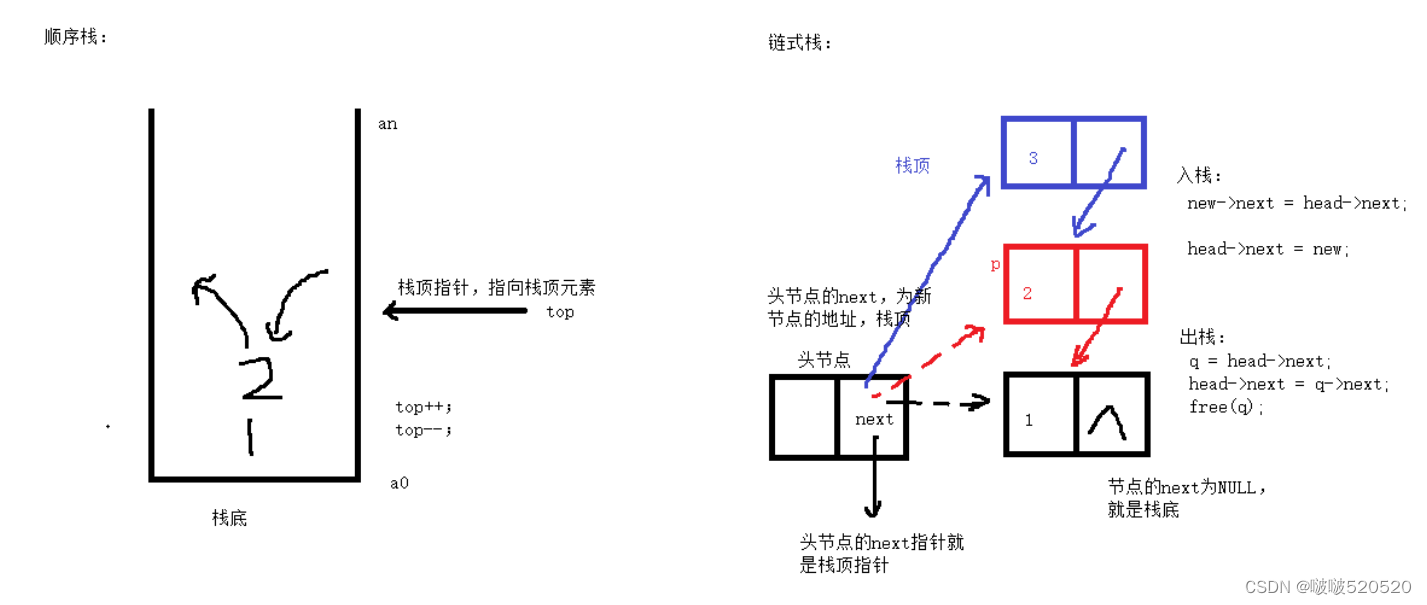
顺序栈例程:
#include <stdio.h>
#include <stdlib.h>
struct stack
{
int data[10];
int top;
};
struct stack * create_stack()
{
struct stack * Stack = malloc(sizeof(struct stack));
Stack->top = 0;
return Stack;
}
int full(struct stack * p)
{
if(p->top == 10)
{
printf("is full\n");
return 1;
}
return 0;
}
int empty(struct stack * p)
{
if(p->top == 0)
{
printf("is empty\n");
return 1;
}
return 0;
}
void push_stack(struct stack * p,int data) //输入
{
if(full(p))
{
return;
}
p->data[p->top] = data;
p->top++;
}
void pop_stack(struct stack * p) //删除
{
if(empty(p))
{
return ;
}
p->top--;
printf("pop data is %d\n",p->data[p->top]);
}
void show_top(struct stack *p)
{
printf("top data is %d\n",p->data[p->top - 1]);
}
void show_stack(struct stack *p)
{
int i = p->top - 1;
for(;i >= 0;i--)
{
printf("%d ",p->data[i]);
}
printf("\n");
}
int main()
{
struct stack * s = create_stack();
pop_stack(s);
push_stack(s,1);
push_stack(s,2);
push_stack(s,3);
show_stack(s);
push_stack(s,5);
return 0;
}
链表栈例程:
#include <stdio.h>
#include <stdlib.h>
//链表节点申明
struct node
{
int data;
struct node * next;
};
//空栈创建,头节点
struct node * create_stack()
{
struct node * head = malloc(sizeof(struct node));
head->next = NULL;//栈顶和栈底在同一个位置
return head;
}
int empty(struct node * head)
{
if(head->next == NULL)
{
printf("is empty\n");
return 1;
}
return 0;
}
void push_stack(struct node * head,int data) //插入
{
struct node * new = malloc(sizeof(struct node));
new->next = head->next;
head->next = new;
new->data = data;
}
void pop_stack(struct node * head) //删除
{
if(empty(head))
{
return ;
}
struct node * del = head->next;
head->next = del->next;
printf("%d\n",del->data);
free(del);
}
void show(struct node * head)
{
struct node * p = head;
while(p->next != NULL)
{
p = p->next;
printf("%d ",p->data);
}
printf("\n");
}
int main()
{
struct node * head = create_stack();
pop_stack(head);
push_stack(head,10);
push_stack(head,20);
show(head);
return 0;
}