目录
1 什么是事务
事务是数据库操作最基本单元,逻辑上一组操作,要么都成功,如果有一个失败所有操
作都失败
2 事务的特性(ACID)
A(Atomicity)原子性:
一个事务必须被视为一个不可分割的最小工作单元,整个事务中的所有操作要么全部提交成功,要么全部失败回滚,对于一个事务来说,不可能只执行其中的一部分操作,这就是事务的原子性
C (Consistency) 一致性:
数据库总是从一个一致性的状态转换到另一个一致性的状态。
例如:转账过程系统崩了,A账户没有扣钱,B账户也没有多钱,因为没有提交事务
I (Isolation) 隔离性:
一个事务所做的修改操作在提交事务之前,对于其他事务来说是不可见的
例子:你们抓的人是周树人,和我鲁迅有什么关系
D (Durability)持久性:
一旦事务提交,则其所做的修改会永久保存到数据库
3 事务的隔离级别(Isolation))
(
1)事务有特性成为隔离性,多事务操作之间不会产生影响。不考虑隔离性产生很多问题
(2)有三个读问题:脏读、不可重复读、虚(幻)读
(3)脏读:一个未提交事务读取到另一个未提交事务的数据
(4)不可重复读:一个未提交事务读取到另一提交事务修改数据
(5)虚读:一个未提交事务读取到另一提交事务添加数据
(6)解决:通过设置事务隔离级别,解决读问题
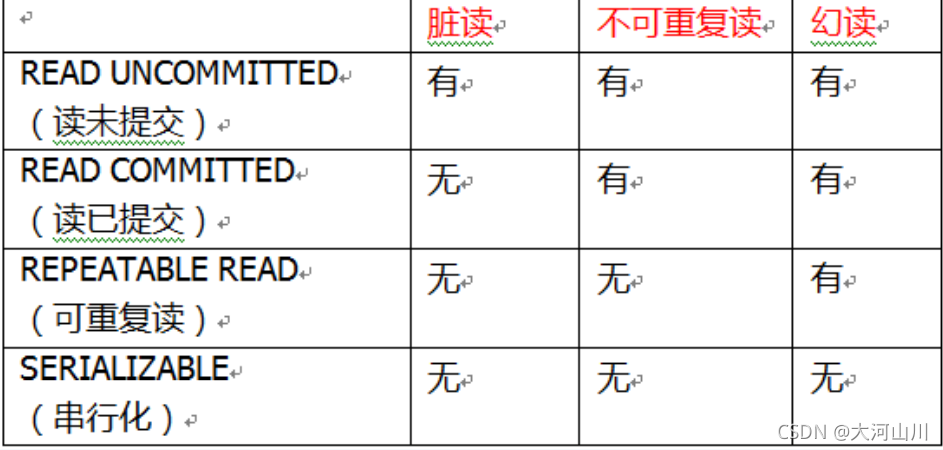
4 事务的传播行为(propagation)
前两个最常用
required:如果有事务在运行,当前的方法就在这个事务中运行,如果没有则创建新的事务
required_new:当前的方法必须启动新的事务运行,如果当前有事务,则挂起
5 案例
描述:模拟一个简单的转账功能,并使用事务
1 创建数据库表语句
CREATE TABLE `account` (
`username` varchar(255) NOT NULL,
`account` double(255,0) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8;
2 编写spring.xml配置文件
配置异常处理
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:util="http://www.springframework.org/schema/util"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx.xsd">
<!--开启注解扫描
1 如果扫描多个包,多个包使用逗号隔开
2 扫描包上层目录-->
<context:component-scan base-package="com.star"/>
<!--开启aop注解扫描-->
<aop:aspectj-autoproxy/>
<!--配置数据源-->
<bean id="dataSource" class="com.alibaba.druid.pool.DruidDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url" value="jdbc:mysql://localhost:3306/ssm"/>
<property name="username" value="root"/>
<property name="password" value="zjx666888"/>
</bean>
<!--配置jdbcTemplate-->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="dataSource"/>
</bean>
<!--创建事务管理器-->
<bean id="manager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<!--注入数据源-->
<property name="dataSource" ref="dataSource"/>
</bean>
<!--开启事务注解-->
<tx:annotation-driven transaction-manager="manager"></tx:annotation-driven>
</beans>
3 创建dao层
//创建dao接口
package com.star.dao;
public interface AccountDao {
public void transfer();
public void collect();
}
//创建dao实现类
package com.star.dao.impl;
import com.star.dao.AccountDao;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jdbc.core.JdbcTemplate;
import org.springframework.stereotype.Repository;
@Repository
public class AccountDaoImpl implements AccountDao {
@Autowired
private JdbcTemplate jdbcTemplate;
/**
* 转账
*/
@Override
public void transfer() {
String sql = "update Account set account = account - ? where username = ?";
jdbcTemplate.update(sql,100,"zjx");
}
/**
* 收款
*/
@Override
public void collect() {
String sql = "update Account set account = account + ? where username = ?";
jdbcTemplate.update(sql,100,"jwy");
}
}
4 创建service层
//创建service接口
package com.star.service;
public interface AccountService {
void transferAccounts();
}
//创建service接口实现类
package com.star.service.impl;
import com.star.dao.AccountDao;
import com.star.service.AccountService;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class AccountServiceImpl implements AccountService {
@Autowired
private AccountDao accountDao;
/**
* 转账
*/
@Override
@Transactional //开启注解 当服务发生异常时自动回滚
public void transferAccounts() {
accountDao.transfer(); //转账
int k = 10/0; //人为异常
accountDao.collect(); //收款
}
}
5 创建测试
@Test
public void testAccount(){
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("classpath:spring6transaction.xml");
AccountService accountService = applicationContext.getBean(AccountService.class);
accountService.transferAccounts();
}
6 测序结果
1 不开启注解扫描时
由此可见一个账户扣款了,一个账户未收到扣款,这样是万万不行滴。
2 开启注解测试
7 补充
在
service
类上面(或者
service
类里面方法上面)添加事务注解
(
1
)
@Transactional
,这个注解添加到类上面,也可以添加方法上面
(
2
)如果把这个注解添加类上面,这个类里面所有的方法都添加事务
(
3
)如果把这个注解添加方法上面,为这个方法添加事务
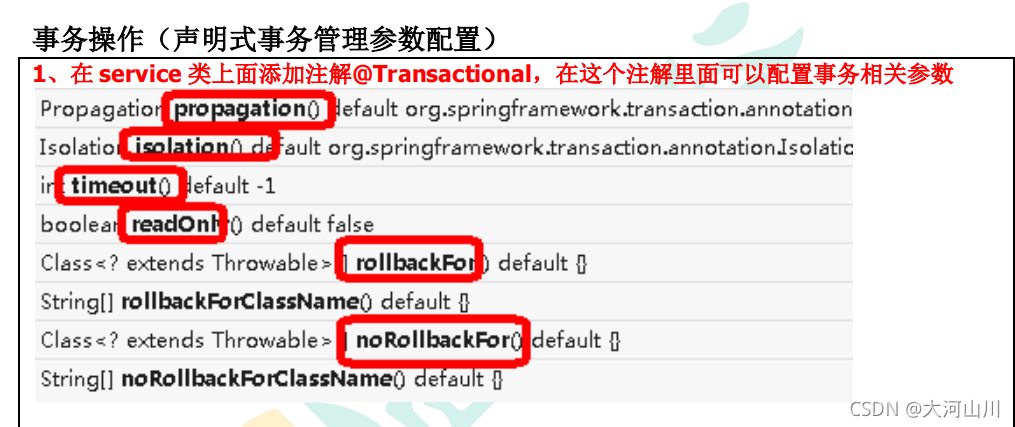