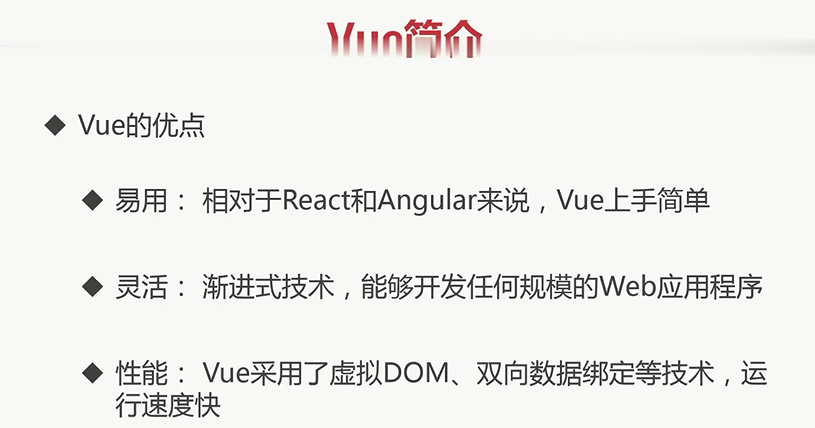
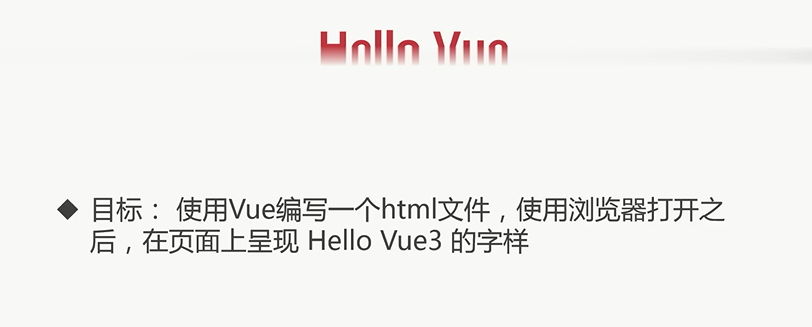
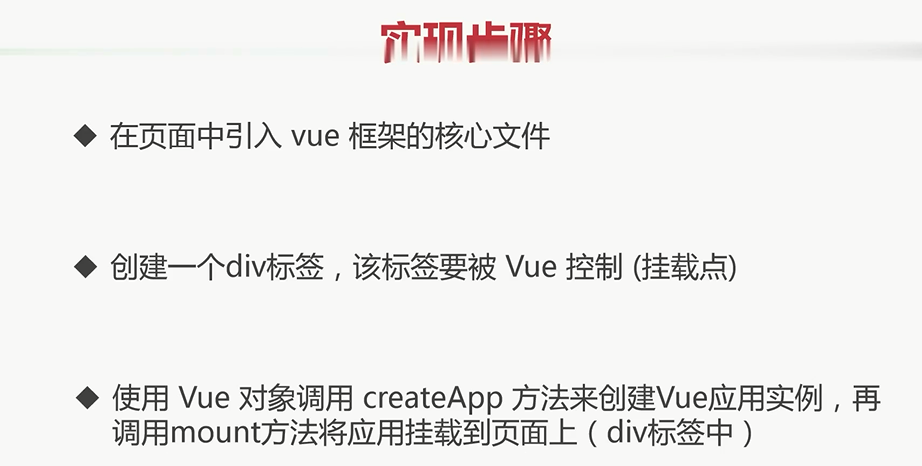
<html>
<head>
<!-- 第一步引入vue的核心文件 -->
<script src="./lib/vue.js"></script>
</head>
<body>
<!-- 第二步:在Body中设置一个标签 -->
<div id="app">
{{msg}}
</div>
<script>
// 第三步
Vue.createApp({
data(){
return{
msg:'Hello Vue3'
}
}
}).mount('#app')//这里是选择器
</script>
</body>
</html>
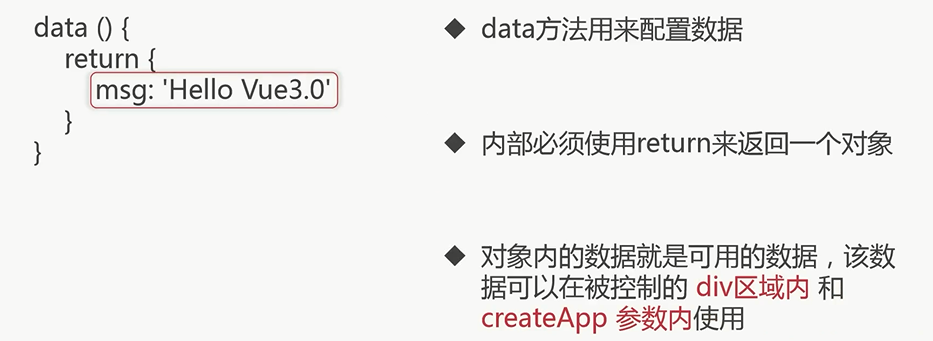
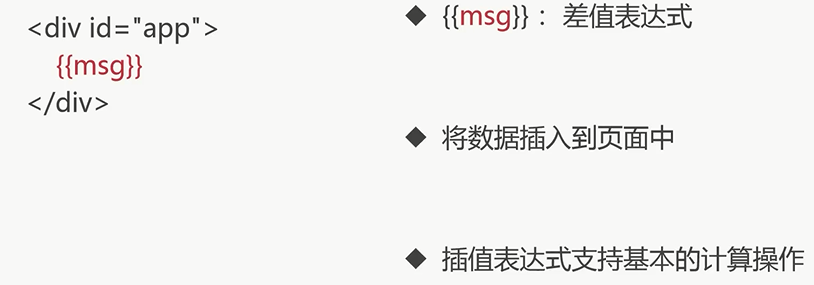
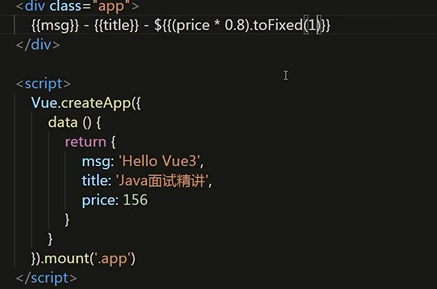

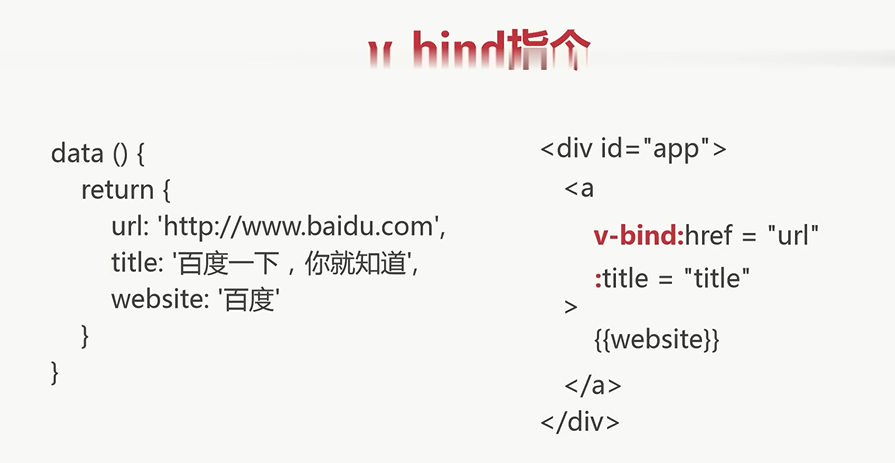
<body>
<div id="app">
<a v-bind:href="url" :title="title">{{website}}</a>
<img v-bind:src="src" alt="">
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
url:'http://www.baidu.com',
title:'百度一下',
website:'百度',
src:'./lib/1.jpg'
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
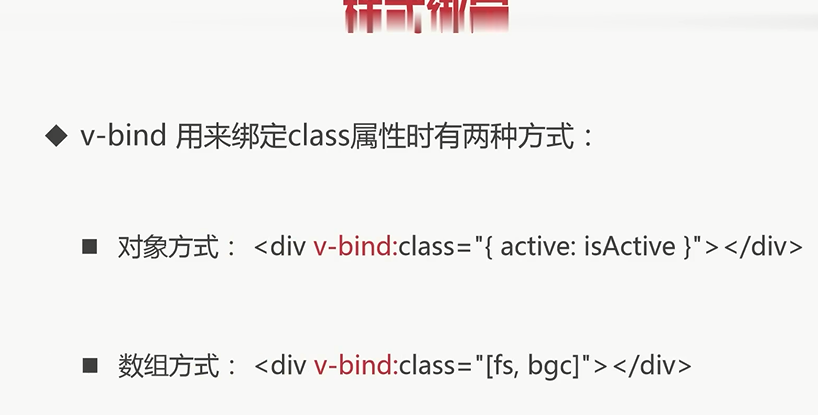
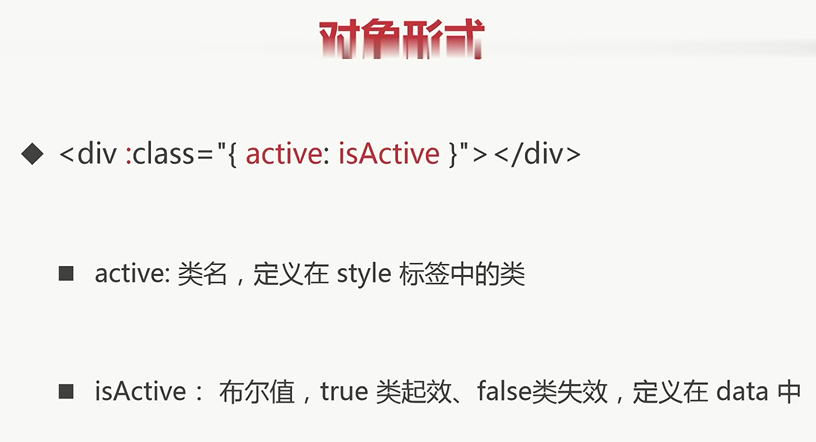
<style>
.active{
color: red;
border: 1px solid #33ee11;
}
.aaa{
background-color: orange;
}
</style>
</head>
<body>
<div id="app">
<p :class="{active:isActive,aaa:isAAA}">明月及是由,把酒问青天</p>
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
isActive:true,
isAAA:true
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
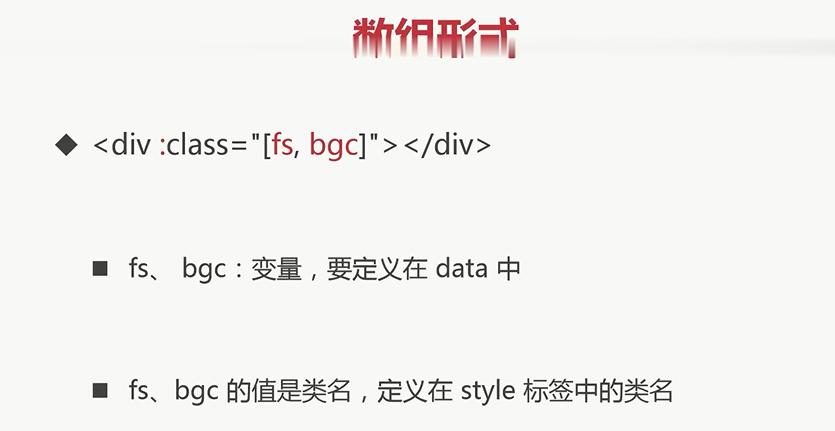
<style>
.active{
color: red;
border: 1px solid #33ee11;
}
.aaa{
background-color: orange;
}
</style>
</head>
<body>
<div id="app">
<h3 :class="[c1,c2]">test</h3>
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
c1:'active',
c2: 'aaa'
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
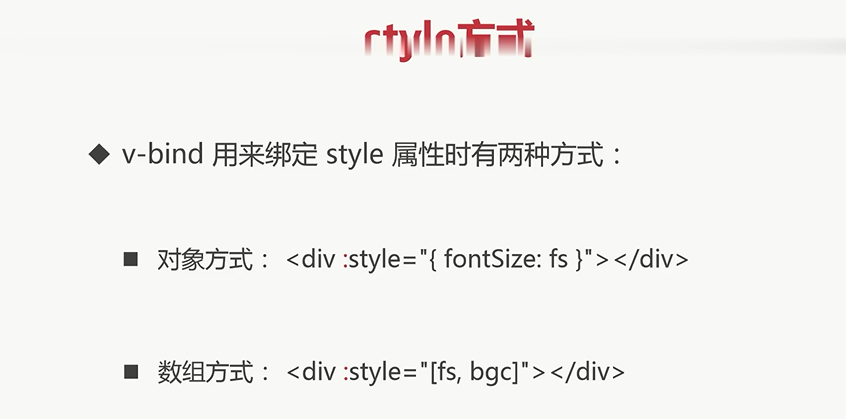
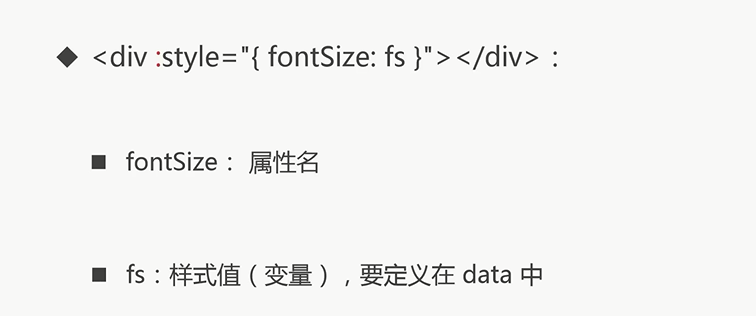
<body>
<div id="app">
<p :style="{fontSize:fs,backgroundColor:bgc}">明月几时有,把酒问青天</p>
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
方式一:变量形式
fs:'20px',
bgc:'orange'
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
<body>
<div id="app">
<p :style="{fontSize:objStyle.fs,backgroundColor:objStyle.bgc}">明月几时有,把酒问青天</p>
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
方式二:用一个对象的形式
objStyle:{
fs:'20px',
bgc:'orange'
}
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
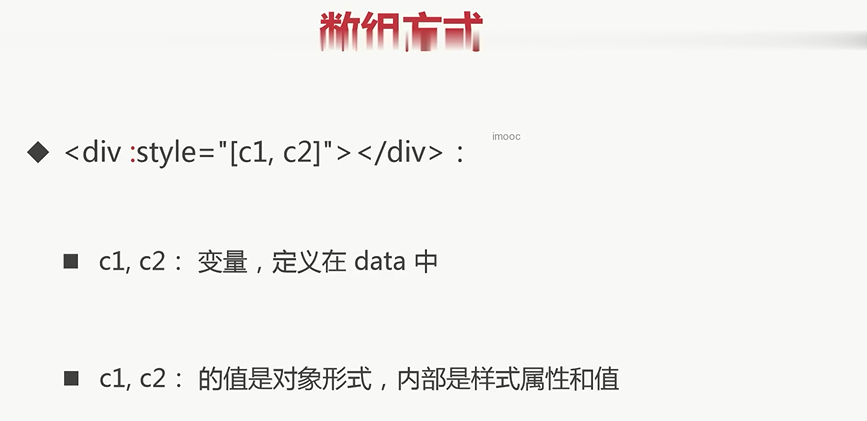
<body>
<div id="app">
<!-- 数组中必须是对象的形式 -->
<h3 :style="[a,b]">xxxxxxxx</h3>
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
objStyle:{
fs:'20px',
bgc:'orange'
},
a:{
fontSize:'30px',
color:'blue',
backgroundColor:'green'
},
b:{
border:'2px solid red'
}
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
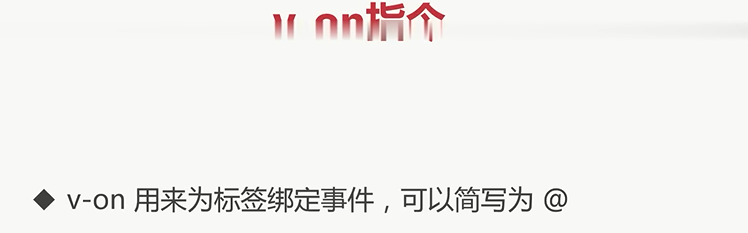
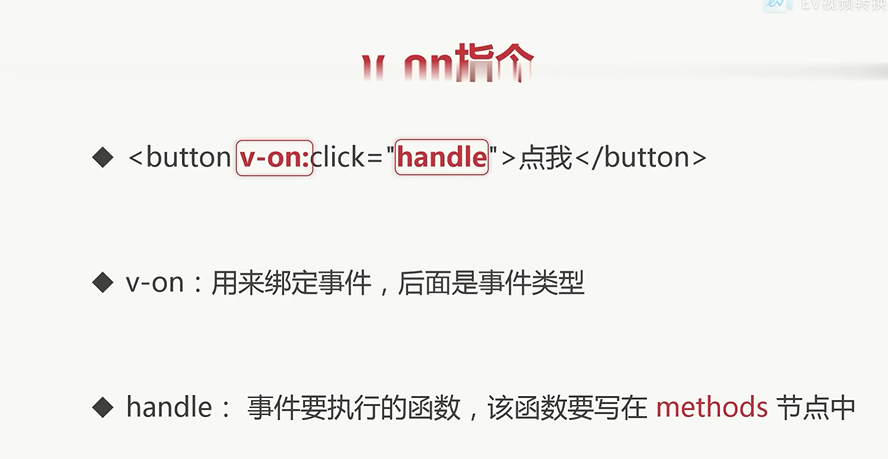
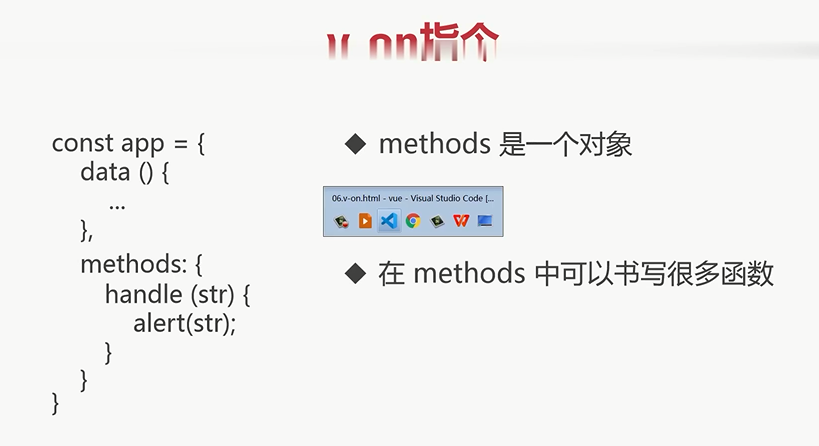
<head>
<style>
.status {
width: 100px;
height: 100px;
line-height: 100px;
text-align: center;
background-color: orange;
color: #fff;
}
</style>
</head>
<body>
<div id="app">
<!-- 第一个绑定事件是无参事件,第二个是有参事件 -->
<div :class="{status:isStatus}"
v-on:click="handle"
@mouseenter="enter('哈哈哈,我来了')">点我</div>
</div>
<script src="./lib/vue.js">点我</script>
<script>
// 目标:点击去除和添加样式
const app={
data(){
return{
isStatus:true
}
},
methods:{
// handle(){
// console.log("我被点了")
// },
enter(str){
console.log(str);
},
handle(){
// 注意调用data只需要用this即可
this.isStatus=!this.isStatus
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
切换图片案例:体会Vue封装了DOM,我们直接用Vue,不用再去处理DOM
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
#app .content {
width: 600px;
margin: 30px auto;
}
#app .content img {
width: 600px;
}
#app .content div {
display: flex;
justify-content: space-between;
}
</style>
</head>
<body>
<div id="app">
<div class="content">
<img :src="list[i]" alt="">
<div>
<button @click="prev">上一张</button>
<button @click="next">下一张</button>
</div>
</div>
</div>
<script src="./lib/vue.js"></script>
<script>
const app = {
data(){
//图片路径数组
return{
list:[
"./images/1.jpg",
"./images/2.jpg",
"./images/3.jpg",
"./images/4.jpg",
"./images/5.jpg"
],
//索引,对应图片数组的索引,0是初始
i:0
}
},
methods:{
next(){
this.i=++this.i==5?0:this.i;
},
prev(){
this.i=--this.i==-1?4:this.i;
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
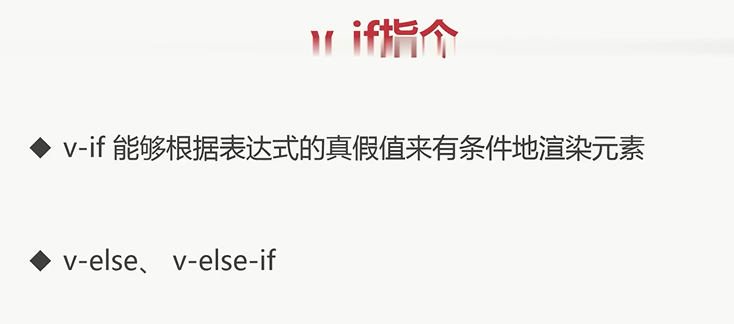
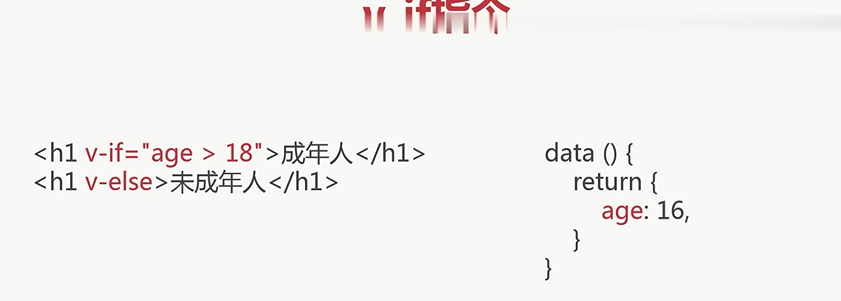
<body>
<div id="app">
//同类型写一块
<p v-if="age>18">成年人</p>
<p v-else>未成年</p>
<h3 v-if="score>70">优秀</h3>
<h3 v-else>良好</h3>
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
age:80,
score:20
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
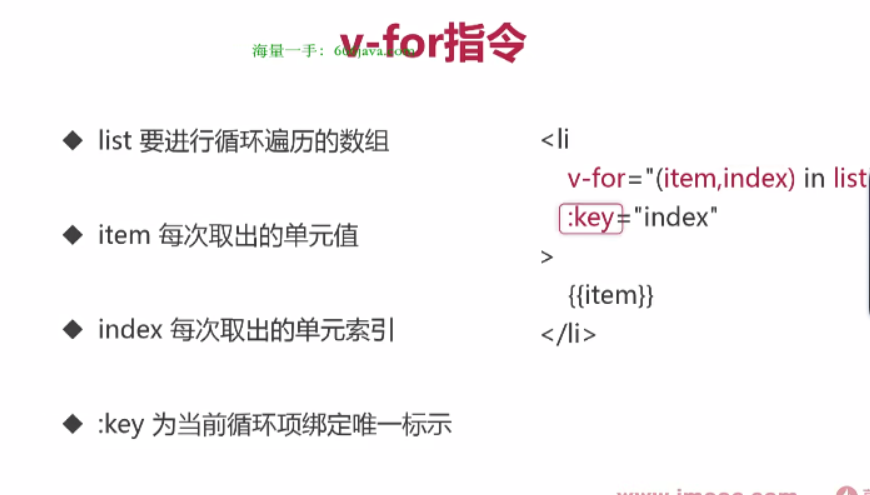
<body>
<div id="app">
<!-- for循环中是圆括号不是{}不要搞错,key要绑定唯一值 -->
<ur>
<li v-for="(item,index) in ary" :key="index">{{item}}</li>
</ur>
</div>
<script src="./lib/vue.js"></script>
<script>
const app={
data(){
return{
ary: ['xxx','2sss','ddd']
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
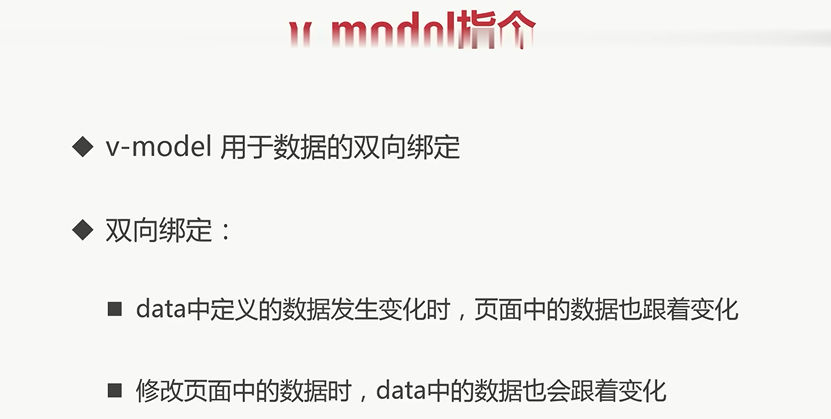
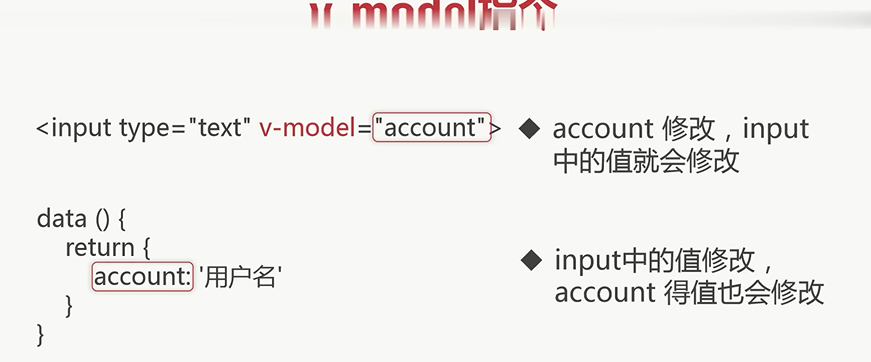
留言板实例:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
input {
width: 260px;
}
textarea {
width: 260px;
margin-top: 10px;
vertical-align: top;
}
ul {
padding: 0px;
list-style: none;
width: 300px;
border: 1px solid #ccc;
border-radius: 5px;
}
.msg {
box-sizing: border-box;
width: 300px;
padding: 10px;
border-bottom: 1px solid #ccc;
}
.close {
float: right;
cursor: pointer;
}
span:hover {
visibility: visible;
}
</style>
</head>
<body>
<div id="app">
姓名: <input type="text" v-model="name" /><br>
留言: <textarea cols="22" rows="10" v-model="content" ></textarea><br>
<button @click="send">发表</button>
<ul>
<li class="msg" v-for="(item, index) in list" :key="index">
{{item.name}} : {{item.content}}
</li>
</ul>
</div>
<script src="./lib/vue.js"></script>
<script>
const app = {
data () {
return {
list: [
{name:"后羿", content:"周日都让我射熄火了"},
{name:"鲁班", content:"智商250..."},
{name:"程咬金", content:"爱心之斧的正义冲击"},
{name:"孙悟空", content:"俺老孙来也"},
{name:"妲己", content:"妲己陪你玩哦"}
],
name: '',
content: ''
}
},
methods: {
send () {
//发表为空格或空的情况,trim是去掉空格
if (this.name.trim().length === 0 || this.content.trim().length === 0) {
alert('请正确输入姓名和留言')
return;
}
//数据不为空转成对象放入list
let tmp = {name: this.name, content: this.content};
this.list.push(tmp);
//放入完成后初始化网页中的输入框,双向绑定清空
this.name = '';
this.content = '';
}
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
</html>
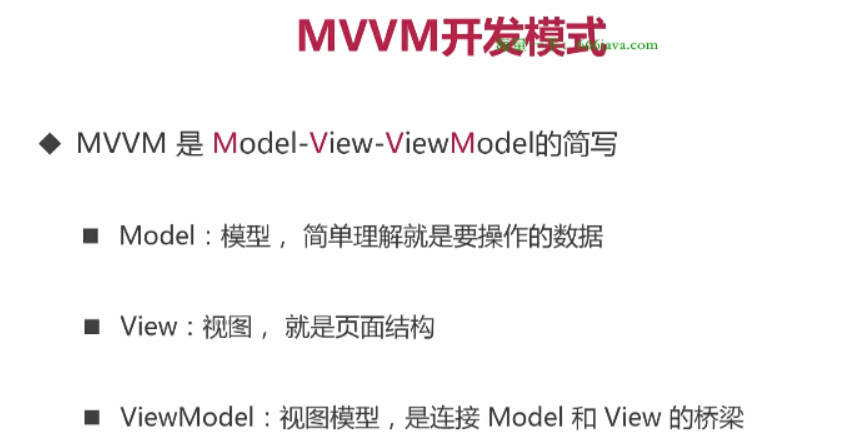

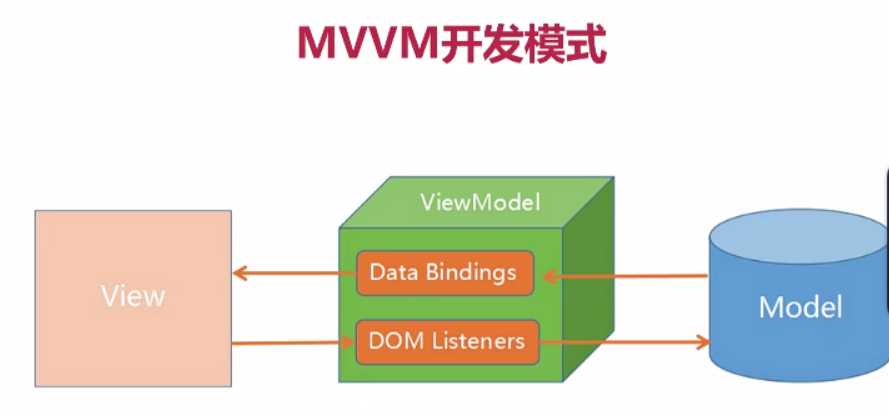
原来的时候数据都需要var定义并且用InnerHTML很麻烦,vue简化了这种操作
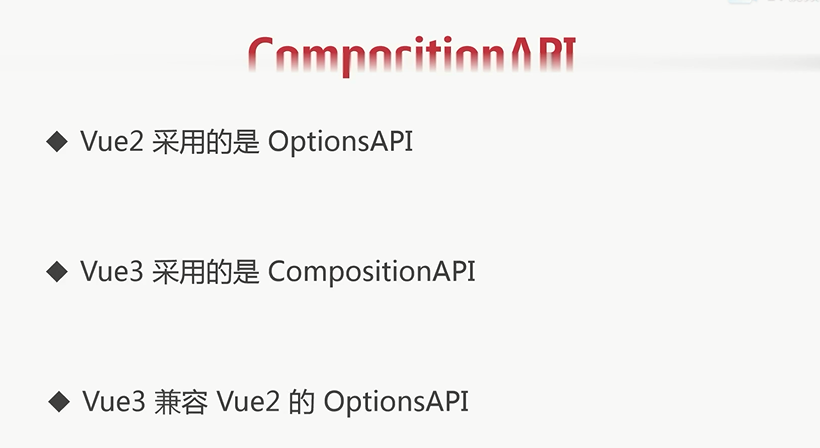
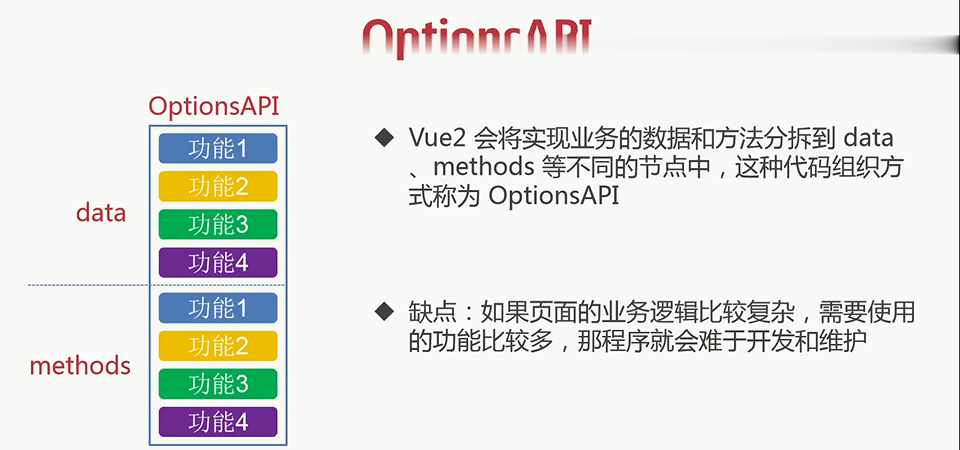
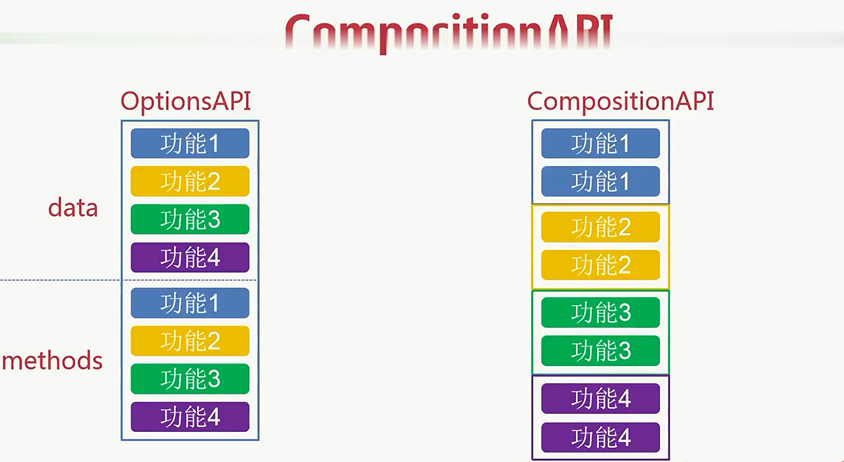
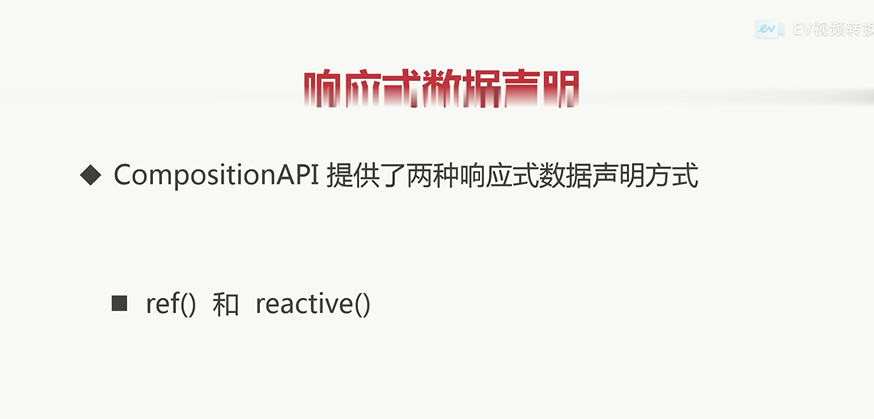
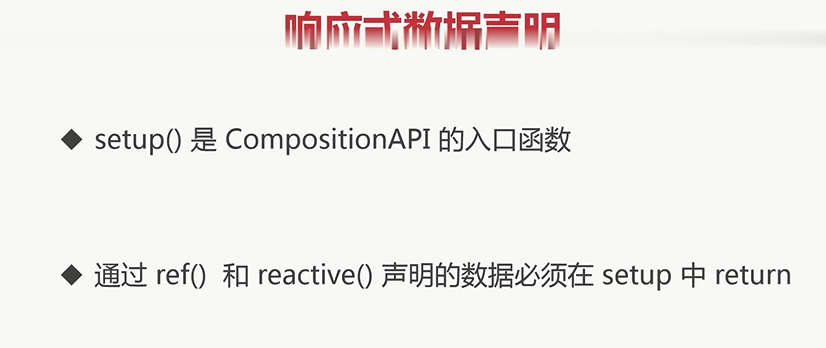
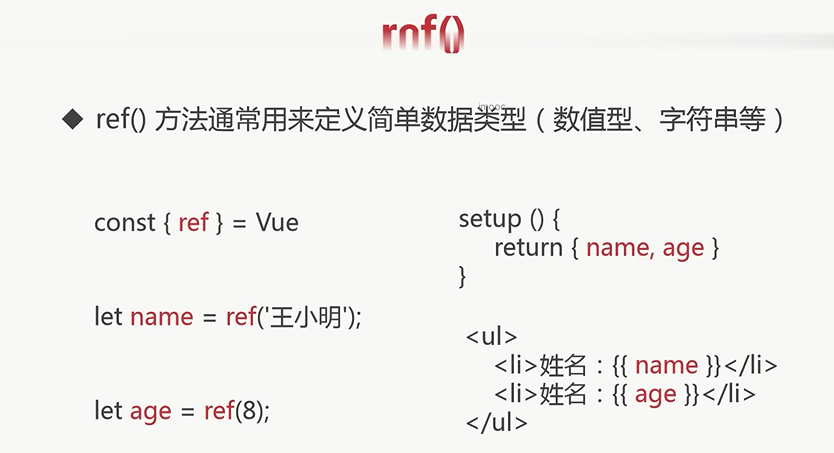
<body>
<div id="app">
<ul>
<li>{{name}} </li>
<li> {{age}}
<button @click="add">+</button>
</li>
</ul>
<input type="text" v-model="name"><br>
<input type="text" v-model="age">
</div>
<script src="./lib/vue.js"></script>
<script>
// 1.Vue对象中将ref方法解构出来
const { ref } = Vue;
2.//使用ref声明响应式数据
let name = ref('王小明');
let age = ref(8);
const add = () => {
age.value++;
}
const app = {
setup() {
return { name, age, add };
}
}
Vue.createApp(app).mount('#app');
</script>
</body>
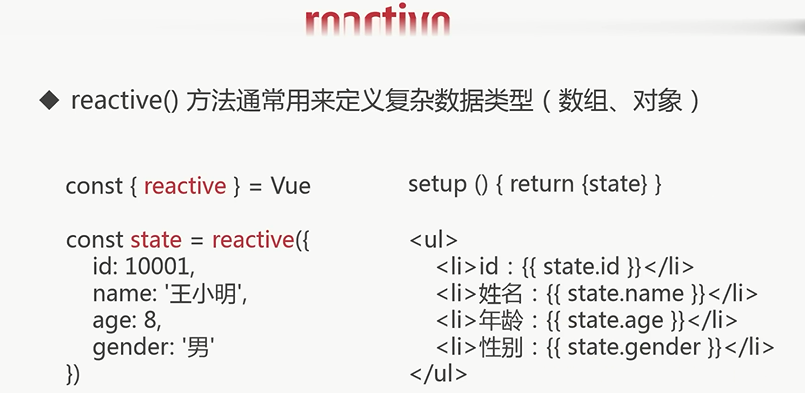
<body>
<div id="app">
<ul>
<!-- 数组 -->
<li v-for="item in list" :key="item.id">
{{item.id}} - {{item.name}} - {{item.age}}
</li>
<!-- 单个对象 -->
<li>
{{user.id}} - {{user.name}} - {{user.age}}
</li>
</ul>
<button @click="show">点我</button>
</div>
<script>
const { reactive } = Vue;
//对象数组的处理
const list = reactive([
{id: 1, name: 'zs', age: 8},
{id: 2, name: 'ls', age: 12},
{id: 3, name: 'ww', age: 10}
])
//对象的处理
const user = reactive({
id: 4,
name: 'zl',
age: 9
})
//方法的使用
const show = () => {
alert('哈哈哈');
}
//不管是定义了方法,还是对象还是数组都要return
const app = {
setup () {
return { list, user, show }
}
}
Vue.createApp(app).mount('#app');
</script>
</body>