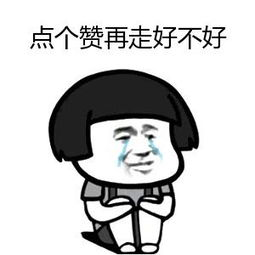
PyTorch | 创建 Tensor
1、从 numpy 创建 tensor
-
t
o
r
c
h
.
f
r
o
m
_
n
u
m
p
y
(
n
u
m
p
y
_
a
r
r
a
y
)
torch.from\_numpy(numpy\_array)
torch.from_numpy(numpy_array)
import numpy as np import torch from IPython.core.interactiveshell import InteractiveShell InteractiveShell.ast_node_interactivity = "all" a = np.array([2,3.3]) torch.from_numpy(a) o = np.ones(3) torch.from_numpy(o)
-
t
o
r
c
h
.
a
s
_
t
e
n
s
o
r
(
n
u
m
p
y
_
a
r
r
a
y
)
torch.as\_tensor(numpy\_array)
torch.as_tensor(numpy_array)
- 将
t
e
n
s
o
r
tensor
tensor 转换成
n
u
m
p
y
numpy
numpy
import numpy as np import torch x = torch.rand(3,3) n = x.numpy() print(type(n)) print(type(n) is np.ndarray)
2、从 List 创建 tensor
import torch
from IPython.core.interactiveshell import InteractiveShell
InteractiveShell.ast_node_interactivity = "all"
torch.tensor([1,2.3]) # 小写tensor接收指定数据
torch.Tensor(3) # 大写Tensor可以接收指定维度
torch.Tensor([1,2.3]) # 大写Tensor可以接收List数据,不建议使用
torch.tensor([[1,2.3],[4,5.6]])
torch.FloatTensor([1,2.3])# 不建议使用
3、创建指定值的 tensor
- 创建值全为 1 1 1 的 t e n s o r tensor tensor: t o r c h . o n e s ( d i m s ) torch.ones(dims) torch.ones(dims)
- 创建值全为 0 0 0 的 t e n s o r tensor tensor: t o r c h . z e r o s ( d i m s ) torch.zeros(dims) torch.zeros(dims)
- 创建值全为未初始化数据的 t e n s o r tensor tensor: t o r c h . e m p t y ( d i m s ) torch.empty(dims) torch.empty(dims)
- 创建 n n n 行 m m m 列对角矩阵: t o r c h . e y e ( n , m ) torch.eye(n,m) torch.eye(n,m)
- 创建
s
i
z
e
size
size 大小,值全为
v
a
l
u
e
value
value 的
t
e
n
s
o
r
tensor
tensor:
t
o
r
c
h
.
f
u
l
l
(
s
i
z
e
,
v
a
l
u
e
)
torch.full(size,value)
torch.full(size,value)
import torch from IPython.core.interactiveshell import InteractiveShell InteractiveShell.ast_node_interactivity = "all" torch.ones(3,4) # 创建值全为1的tensor torch.zeros(3,4) # 创建值全为0的tensor torch.empty(4,5) # 创建值全为未初始化数据的tensor torch.eye(4,4) # 创建4*4的对角矩阵 torch.full((3,4), 3.14) # 创建3*4值为3.14的tensor
4、从 tensor 创建 tensor(torch.**_like)
- 保留原始 t e n s o r tensor tensor 的形状,改变数据。
- 填充数据全为 1 1 1: t o r c h . o n e s _ l i k e ( x _ d a t a ) torch.ones\_like(x\_data) torch.ones_like(x_data)
- 填充数据全为 0 0 0: t o r c h . z e r o s _ l i k e ( x _ d a t a ) torch.zeros\_like(x\_data) torch.zeros_like(x_data)
- 填充未初始化数据: t o r c h . e m p t y _ l i k e ( x _ d a t a ) torch.empty\_like(x\_data) torch.empty_like(x_data)
- 0 − 1 0-1 0−1 随机填充数据: t o r c h . r a n d _ l i k e ( x _ d a t a ) torch.rand\_like(x\_data) torch.rand_like(x_data)
- 标准正态随机填充: t o r c h . r a n d n _ l i k e ( x _ d a t a ) torch.randn\_like(x\_data) torch.randn_like(x_data)
- 填充 [ l o w , h i g h ) [low, high) [low,high) 范围整数: t o r c h . r a n d i n t _ l i k e ( x _ d a t a , l o w , h i g h ) torch.randint\_like(x\_data, low, high) torch.randint_like(x_data,low,high)
- 改变数据类型,添加参数
d
t
y
p
e
:
t
o
r
c
h
.
r
a
n
d
_
l
i
k
e
(
x
_
d
a
t
a
,
d
t
y
p
e
=
f
l
o
a
t
)
dtype:torch.rand\_like(x\_data, dtype=float)
dtype:torch.rand_like(x_data,dtype=float)
import torch from IPython.core.interactiveshell import InteractiveShell InteractiveShell.ast_node_interactivity = "all" a = torch.randn(3,5) torch.ones_like(a) torch.empty_like(a) torch.rand_like(a) torch.randn_like(a) torch.randint_like(a,1,10) torch.rand_like(a,dtype=torch.float64)
5、生成未初始化数据
import torch
from IPython.core.interactiveshell import InteractiveShell
InteractiveShell.ast_node_interactivity = "all"
torch.empty(2,3)
torch.FloatTensor(3,4)
torch.IntTensor(3,4,5)
6、设置默认 Tensor
-
T
e
n
s
o
r
Tensor
Tensor 默认为
t
o
r
c
h
.
F
l
o
a
t
T
e
n
s
o
r
torch.FloatTensor
torch.FloatTensor,可以使用
set_default_tensor_type
方法修改默认 T e n s o r Tensor Tensor。import torch from IPython.core.interactiveshell import InteractiveShell InteractiveShell.ast_node_interactivity = "all" torch.Tensor(3,4).type() torch.set_default_tensor_type(torch.DoubleTensor) torch.Tensor(3,4).type()
7、随机初始化
-
t o r c h . r a n d ( d a t a ) : torch.rand(data): torch.rand(data):包含了从区间 [ 0 , 1 ) [0, 1) [0,1) 的均匀分布中抽取的一组随机数。
-
t o r c h . r a n d n ( 3 ) : torch.randn(3): torch.randn(3):包含了从标准正态分布(均值为 0 0 0,方差为 1 1 1,即高斯白噪声)中抽取的 3 3 3 个随机数。
-
t o r c h . r a n d i n t ( l o w , h i g h , s i z e ) : torch.randint(low,high,size): torch.randint(low,high,size):包含了从区间 [ l o w , h i g h ) [low, high) [low,high) 中抽取的一组随机数,形状由参数 s i z e size size 决定。
-
t o r c h . r a n d p e r m ( n ) : torch.randperm(n): torch.randperm(n):返回 n n n 个从 0 0 0 到 n − 1 n-1 n−1 的随机整数。
-
t o r c h . m a n u a l _ s e e d ( i n t ) : torch.manual\_seed(int): torch.manual_seed(int):
torch.manual_seed(int)
:为 C P U CPU CPU 设置种子用于生成随机数,以使得每次运行.py
文件结果是确定的,而不是每次随机函数生成的结果一样。torch.cuda.manual_seed(int)
:为当前 G P U GPU GPU 设置随机种子;如果使用多个 G P U GPU GPU,应该使用torch.cuda.manual_seed_all(int)
为所有的 G P U GPU GPU 设置种子。
python shuffle()方法 pytorch实现shuffle()功能 shuffle()方法将序列的所有元素随机排序 torch中没有random.shuffle()
使用torch.randperm(n)实现shuffle()功能示例:
import random
list = [20, 16, 10, 5]
print("排序前列表 : ", list)
random.shuffle(list)
print("排序后列表 : ", list)示例:
import torch
idx = torch.randperm(3)
a = torch.Tensor(4,2)
print(a)
print(idx,idx.type())
print(a[idx])
结果: 结果: -
t o r c h . n o r m a l ( A , B , s i z e ) : torch.normal(A,B,size): torch.normal(A,B,size):A表示均值,B表示标准差,形状由参数 s i z e size size 决定。
import torch from IPython.core.interactiveshell import InteractiveShell InteractiveShell.ast_node_interactivity = "all" torch.rand(3) # 返回一个张量,包含了从区间[0, 1)的均匀分布中抽取的一组随机数 torch.rand(3,3) torch.randn(3) # 返回一个张量,包含了从标准正态分布(均值为0,方差为1,即高斯白噪声)中抽取的一组随机数 torch.randn(3,3) torch.randint(1,10,[3,4]) # 返回一个张量,包含了从指定范围中抽取的一组随机数,形状由参数指定 torch.randperm(10) # 返回10个从0到9的随机整数
8、创建等量序列
- t o r c h . a r a n g e ( s t a r t , e n d , s t e p ) : torch.arange(start, end, step): torch.arange(start,end,step):返回以 s t a r t start start 为起点, e n d end end 为终点(不包含),以 s t e p step step 为步长的张量。
- t o r c h . l i n s p a c e ( s t a r t , e n d , s t e p s ) : torch.linspace(start, end, steps): torch.linspace(start,end,steps):返回包含在起点 s t a r t start start 和终点 e n d end end ,并在区间均匀分布的总计 s t e p s steps steps 个点。
-
t
o
r
c
h
.
l
o
g
s
p
a
c
e
(
s
t
a
r
t
,
e
n
d
,
s
t
e
p
s
)
:
torch.logspace(start, end, steps):
torch.logspace(start,end,steps):返回包含
1
0
s
t
a
r
t
10^{start}
10start 为起始值,
1
0
e
n
d
10^{end}
10end 终点,并在区间均匀分布的总计
s
t
e
p
s
steps
steps 个点。
import torch from IPython.core.interactiveshell import InteractiveShell InteractiveShell.ast_node_interactivity = "all" torch.arange(1,10,2) torch.linspace(1, 10, 7) torch.logspace(1,10,5)
9、判断是否为 tensor
-
t
o
r
c
h
.
i
s
_
t
e
n
s
o
r
(
o
b
j
)
:
torch.is\_tensor(obj):
torch.is_tensor(obj):如果
o
b
j
obj
obj 是一个
p
y
t
o
r
c
h
pytorch
pytorch 张量,则返回
T
r
u
e
True
True。
10、torch.tensor 与 torch.Tensor 的区别
- t o r c h . t e n s o r torch.tensor torch.tensor 是从数据中推断数据类型,而 t o r c h . T e n s o r torch.Tensor torch.Tensor 是 t o r c h . e m p t y torch.empty torch.empty(会随机产生垃圾数组,详见实例)和 t o r c h . t e n s o r torch.tensor torch.tensor 之间的一种混合。但是,当传入数据时, t o r c h . T e n s o r torch.Tensor torch.Tensor 使用全局默认 d t y p e ( F l o a t T e n s o r ) dtype(FloatTensor) dtype(FloatTensor);
- t o r c h . t e n s o r ( 1 ) torch.tensor(1) torch.tensor(1) 返回一个固定值 1 1 1,而 t o r c h . T e n s o r ( 1 ) torch.Tensor(1) torch.Tensor(1) 返回一个大小为 1 1 1 的张量,它是初始化的随机值。