MySQL数据库
SQL语言
- DQL语言: 查询
- DML语言: 插入、修改、删除语句
- DDL语言: 库和表的管理、数据类型、约束
- TCL语言:事物和事物处理
排序
select * from myemployees.employees order by salary desc;
select * from myemployees.employees order by salary asc;
select *
from myemployees.employees
where department_id>=90 order by hiredate asc
select *,salary*12*(1+ifnull(commission_pct,0)) 年薪
from myemployees.employees
order by salary*12*(1+ifnull(commission_pct,0)) desc
select *,salary*12*(1+ifnull(commission_pct,0)) 年薪
from myemployees.employees
order by 年薪 desc
select
length(last_name) 字节长度,
last_name,
salary
from myemployees.employees
order by length(last_name) desc;
select
employee_id,
first_name,
last_name,
salary
from myemployees.employees
order by salary asc,employee_id desc
常见函数
select length('john');
select length('张三丰hahaha');
show variables like '%char%'
select concat(last_name, '_', first_name)
from myemployees.employees
select upper('john');
select lower('joHn');
select concat(upper(first_name), '_', lower(last_name))
from myemployees.employees
select substr('李莫愁爱上了陆展元', 7) output;
select substr('李莫愁爱上了陆展元', 1, 3) output;
select concat(upper(substr(last_name, 1, 1)), '_', lower(substr(last_name, 2))) output
from myemployees.employees
select instr('杨不悔爱上了殷六侠', '殷六侠')
select length(trim(' 张翠山 ')) as Output;
select trim('a' from 'aaa张aaa翠山aaa') as output;
select trim('aa' from 'aaa张aaa翠山aaa') as output;
select lpad('殷素素', 10, '*') as output
select replace('张无忌爱上了周芷若', '周芷若', '赵敏');
select round(1.65);
select round(-1.65);
select ceil(1.52)
select ceil(-1.52)
select floor(-9.99)
select truncate(1.699999, 1)
select mod(-10, -3);
select now();
select current_date;
select current_time;
select year(now());
select year(hiredate)
from myemployees.employees;
select month(now());
select monthname(now())
select str_to_date('1998-3-2', '%Y-%c-%d') as output
select *
from myemployees.employees
where hiredate = '1992-4-3'
select *
from myemployees.employees
where hiredate = str_to_date('4-3 1992', '%c-%d %Y')
select date_format(now(), '%y年%m月%d日')
select last_name,
date_format(hiredate, '%m月/%d日 %y年') 入职日期
from myemployees.employees
where commission_pct is not null
select version();
select database();
select user();
select if(10 > 5, '大', '小');
select last_name,
commission_pct,
if(commission_pct is not null, '有奖金', '没奖金') judgement
from myemployees.employees
select salary 原始工资,
department_id,
case department_id
when 30 then salary * 1.1
when 40 then salary * 1.2
when 50 then salary * 1.3
else salary
end as 新工资
from myemployees.employees
order by 新工资 desc
select
salary,
case
when salary>20000 then 'A'
when salary>15000 then 'B'
when salary>10000 then 'C'
else 'D'
end
from myemployees.employees
select avg(salary), department_id
from myemployees.employees
group by department_id
select max(salary),
job_id
from myemployees.employees
group by job_id
select count(*), location_id
from myemployees.departments
group by location_id
select round(avg(salary), 2),
department_id
from myemployees.employees
where email like '%a%'
group by department_id
select max(salary),
manager_id
from myemployees.employees
where commission_pct is not null
group by manager_id
select count(*),
department_id
from myemployees.employees
group by department_id
having count(*) > 2
select a.co,
a.department_id
from (select count(*) co,
department_id
from myemployees.employees
group by department_id) a
where co > 2
select employee_id,
job_id,
max(salary)
from myemployees.employees
where commission_pct is not null
group by job_id
having max(salary) > 12000
select max(salary),
job_id
from myemployees.employees
where commission_pct is not null
group by job_id
select max(salary),
job_id
from myemployees.employees
where commission_pct is not null
group by job_id
having max(salary) > 12000
select manager_id,
min(salary)
from myemployees.employees
where manager_id > 102
group by manager_id
having min(salary) > 5000
select count(*),
length(last_name) len_name
from myemployees.employees
group by length(last_name)
having count(last_name) > 15
select count(*) c,
length(last_name) len_name
from myemployees.employees
group by len_name
having c > 15
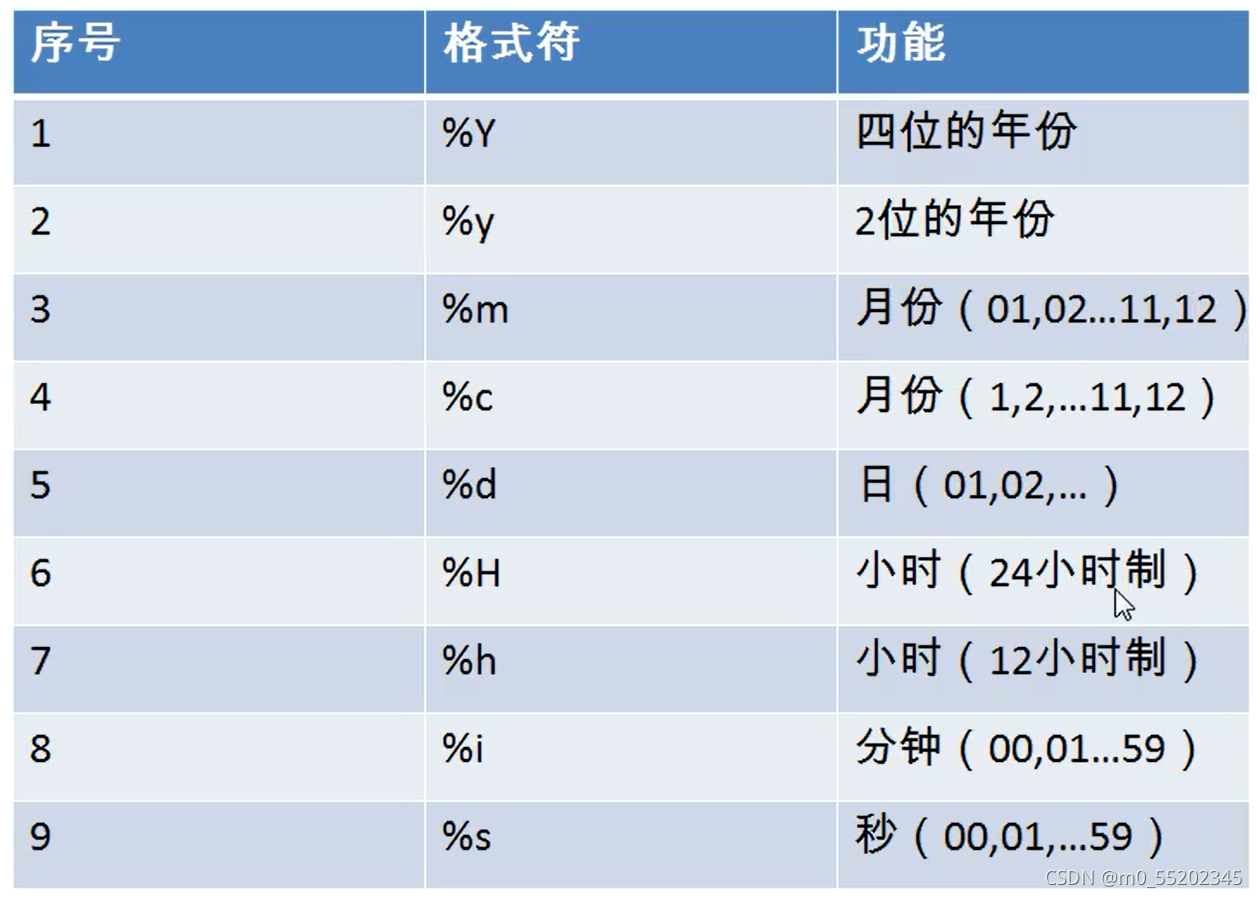