文章目录
- I/O流
- 常用的文件操作
- 获取文件的相关信息
- 目录的操作和文件删除
- Java IO 流原理
- FileInputStream 介绍
- FileOutputStream 介绍文件字节输出流
- 编程完成图片/音乐 的拷贝
- FileReader (字符方式读)和 FileWriter(字符方式写) 介绍
- 节点流和处理流
- 模拟修饰器模式
- 处理流-BufferedReader 和 BufferedWriter
- 处理流-BufferedInputStream 和 BufferedOutputStream
- 对象流-ObjectInputStream 和 ObjectOutputStream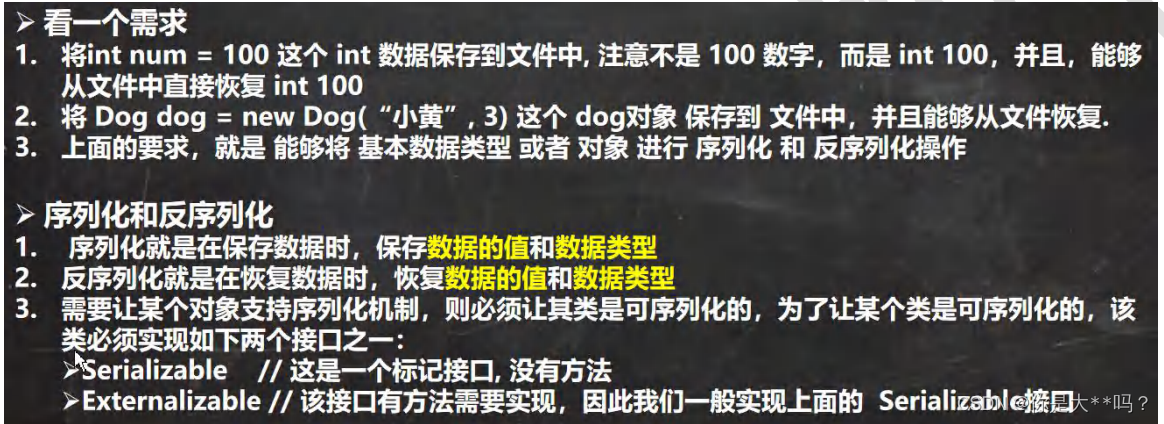
- 标准输入输出流 --standard
- 转换流-InputStreamReader 和 OutputStreamWriter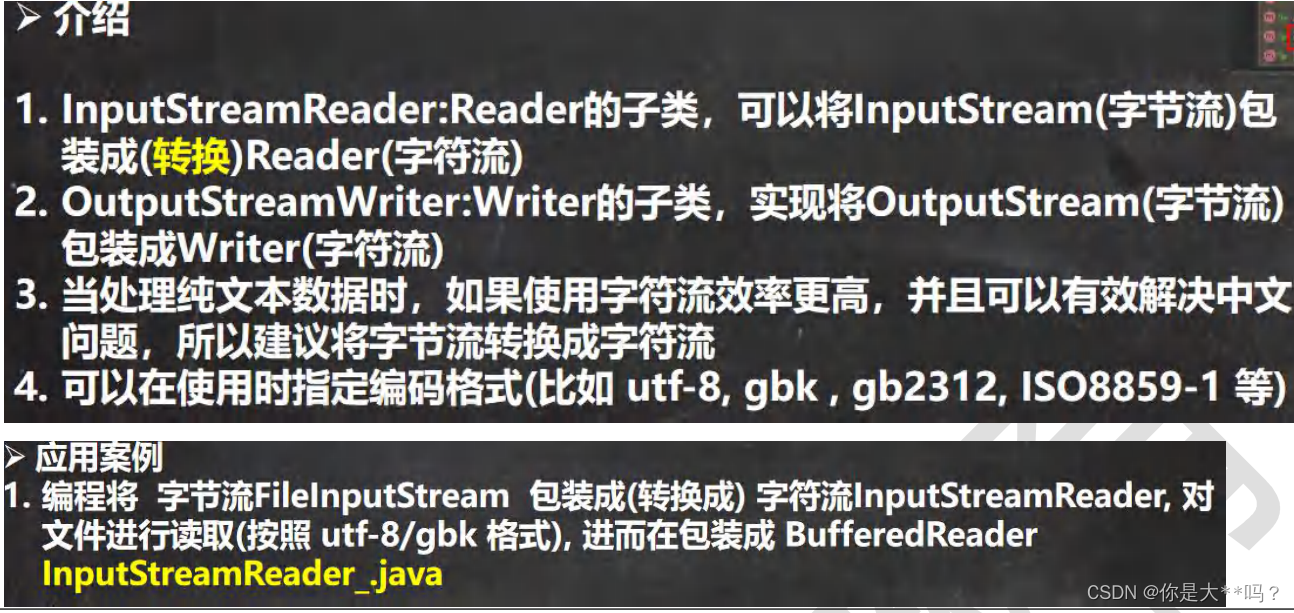
- 打印流-PrintStream 和 PrintWriter
- Properties 类
- 习题1
- 习题2
- 习题3
I/O流
常用的文件操作
package com.File_.FileBase;
import org.junit.jupiter.api.Test;
import java.io.File;
import java.io.IOException;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class file01 {
public static void main(String[] args) {
}
//三种创建文件的方式
//1.
public void method1(){
String s = new String("e:\\hello1.txt");
File filePath = new File(s);
//还要创建file文件现在只是在内存中有了一个file对象
try {
filePath.createNewFile();
System.out.println("文件创建成功...");
} catch (IOException e) {
e.printStackTrace();
}
}
@Test
//2.//(父目录文件对象,子路径)
public void method2(){
String s = new String("e:");
File filePath = new File(s);
String SonPath = new String("hello2.txt");
//(父目录文件对象,子路径)
File file = new File(filePath, SonPath);
//话还要创建file文件现在只是在内存中有了一个file对象
try {
file.createNewFile();
System.out.println("文件创建成功...");
} catch (IOException e) {
e.printStackTrace();
}
}
@Test
//3. //(父路径字符串,子路径字符串)
public void method3(){
String s = new String("e:");
String SonPath = new String("hello3.txt");
//(父路径字符串,子路径字符串)
File file = new File(s, SonPath);
//话还要创建file文件现在只是在内存中有了一个file对象
try {
file.createNewFile();
System.out.println("文件创建成功...");
} catch (IOException e) {
e.printStackTrace();
}
}
}
获取文件的相关信息
package com.File_.FileBase;
import java.io.File;
import java.io.IOException;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class file02 {
public static void main(String[] args) {
String s = new String("e:\\hi1.txt");
File file = new File(s);
try {
file.createNewFile();
System.out.println("文件创建成功...");
} catch (IOException e) {
e.printStackTrace();
}
//获取名字
System.out.println("文件名: "+file.getName());
//文件名: hi1.txt
//获取绝对路径
System.out.println("获取绝对路径:"+file.getAbsolutePath());
//获取绝对路径:e:\hi1.txt
//获得父类路径
System.out.println("父类路径:"+file.getParent());
//父类路径:e:\
//获得文件所占字节个数
System.out.println("该文件字节个数:"+file.length());
//该文件字节个数:10
//判断文件是否存在
System.out.println(file.exists());
//true
//判断是文件还是目录
System.out.println(file.isFile());
System.out.println(file.isDirectory());
// true
// false
}
}
目录的操作和文件删除
package com.File_.FileBase;
import java.io.File;
import java.io.IOException;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class file03 {
public static void main(String[] args) {
// File file = new File("e:\\hi2.txt");
// File file1 = new File("e:\\hi3.txt");
// File file2 = new File("e:\\hi4.txt");
// //创建三个文件
// try {
// file.createNewFile();
// file1.createNewFile();
// file2.createNewFile();
// } catch (IOException e) {
// e.printStackTrace();
// }
//
// System.out.println("删除第hi2个文件 "+file.delete());
// //删除第hi2个文件 true
//创建目录 创建一级目录mkdir 创建多级目录 mkdirs 删除delete
File file = new File("d:\\deom\\hi\\hello");
if(file.exists()){
System.out.println("文件目录存在,删除了");
file.delete();
}
else {
System.out.println("创建文件目录...");
// file.mkdir();创建一级目录
file.mkdirs();
System.out.println("创建成功");
}
}
}
Java IO 流原理
FileInputStream 介绍
请使用 FileInputStream 读取 hello.txt 文件, 并将文件内容显示到控制台
read()读一个字节返回来
read(byte[])读多个字节到byte数组
package com.File_.FileBase;
import org.junit.jupiter.api.Test;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class file04 {
public static void main(String[] args) {
}
/**
* 演示读取文件...
// * 单个字节的读取, 效率比较低
// * -> 使用 read(byte[] b)
// */
@Test
public void inputStream() {
//1.创建路径字符串
String filePath = new String("e:\\hello1.txt");
FileInputStream fileInputStream = null;
//以字节的方法来读出汉字占3个字节读出来会乱码
int obj = 0;
try {
//创建fileinputstream对象来读取文件内容
fileInputStream = new FileInputStream(filePath);
//调用read()方法返回一个字符的ASCII码 读到结束位置返回-1
while ((obj = (int) fileInputStream.read()) != -1) {
System.out.print((char)obj);//转为字符输出
//
}
} catch (IOException e) {
e.printStackTrace();
} finally {//必须关闭文件流
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* 演示读取文件...
// * 单个字节的读取, 效率比较低
// * -> 使用 read(byte[] b)
// */
@Test
public void inputStream1() {
//1.创建路径字符串
String filePath = new String("e:\\hello1.txt");
FileInputStream fileInputStream = null;
//以字节的方法来读出汉字占3个字节读出来会乱码
int Num = 0;
byte buf[]=new byte[8];//一次读八个字节
try {
//创建fileinputstream对象来读取文件内容
fileInputStream = new FileInputStream(filePath);
//调用read(byte[])方法返回获得字节个数 读到结束位置返回-1
while ((Num = fileInputStream.read(buf)) != -1) {
// new String(字节数组,起始下标,结束下标)
System.out.print(new String(buf,0,Num));
//
}
} catch (IOException e) {
e.printStackTrace();
} finally {//必须关闭文件流
try {
fileInputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
FileOutputStream 介绍文件字节输出流
要求: 请使用 FileOutputStream 在 a.txt 文件, 中写入 “hello, world” . [老师代码演示], 如果文件不存在, 会创建
文件(注意: 前提是目录已经存在.)
package com.File_.FileBase;
import org.junit.jupiter.api.Test;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class file05 {
public static void main(String[] args) {
}
/**
* 要求: 请使用 FileOutputStream 在 a.txt 文件, 中写入 “hello, world” . [老师代码演示], 如果文件不存在,
* 会创建文件(注意: 前提是目录已经存在.)
*/
@Test
public void OutputStream() {
//说明
//1. new FileOutputStream(filePath) 创建方式, 当写入内容是, 会覆盖原来的内容
//2. new FileOutputStream(filePath, true) 创建方式, 当写入内容是, 是追加到文件后面
//准备一个文件输出流
FileOutputStream fileOutputStream = null;
//1.创建一个新目录
File file = new File("e:\\output");
file.mkdir();//创建一级目录
try {
//创建了文件输出流若是该文件目录没有a.txt,系统会自动创建
fileOutputStream = new FileOutputStream("e:\\output\\a.txt",true);
//添加一个字节
fileOutputStream.write('A');
fileOutputStream.write('B');
写入字符串
String str="hello,world";
// fileOutputStream.write(str.getBytes());
// fileOutputStream.write(str.getBytes(),0,2);
//write方法里会自动追加
/*
public void write(byte b[], int off, int len) throws IOException {
writeBytes(b, off, len, append);
}
*/
} catch (IOException e) {
e.printStackTrace();
} finally {
try {//关闭文件
fileOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Write方法会自动追加添加的字符
编程完成图片/音乐 的拷贝
package com.File_.FileBase;
import java.io.*;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class file06 {
public static void main(String[] args) {
//完成 文件拷贝, 将d:\\AAA.jpg 拷贝 e:\\
// 暂存字节数组
byte buf[]=new byte[1024];
int readlen=0;
String filePathIn="d:\\AAA.jpg";
String filePathOut="e:\\AAAtemp.jpg";//目标路径,没有就自动创建
//创建一个输入流先把文件输入到内存
FileInputStream fileInputStream = null;
FileOutputStream fileOutputStream=null;
try {//创建一个输入对象
fileInputStream = new FileInputStream(filePathIn);
//创建一个输出流对象
fileOutputStream=new FileOutputStream(filePathOut);
//先输入到内存,利用while循环输入到字节数组暂存
//读1024个字节数据到buf返回读取的个数
while((readlen=fileInputStream.read(buf))!=-1){
//把读来的数据写到外设
fileOutputStream.write(buf,0,readlen);
}
System.out.println("读入数据完毕...");
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
fileInputStream.close();
fileOutputStream.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
FileReader (字符方式读)和 FileWriter(字符方式写) 介绍
FileReader 相关方法
FileWriter 常用方法
FileReader 和 FileWriter 应用案例
FileReader
package com.File_.FileBase;
import org.junit.jupiter.api.Test;
import java.io.FileReader;
import java.io.IOException;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class FileReader_ {
public static void main(String[] args) {
}
/**
* 字符的方式读story.txt文件的内容
*/
@Test
public void method1(){
//创建路径
String filePath="e:\\story.txt";
FileReader fileReader=null;
int ch=0;
try {
//创建读取的对象
fileReader=new FileReader(filePath);
//返回一个字节给ch
while ((ch=fileReader.read())!=-1){
System.out.print((char)ch);
/*
渡远荆门外,来从楚国游。
山随平野尽,江入大荒流。
*/
}
} catch (IOException e) {
e.printStackTrace();
}
finally {
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
@Test
public void method2(){
//创建路径
String filePath="e:\\story.txt";
FileReader fileReader=null;
char buf[]=new char[8];
int readCharNum=0;
System.out.println("Method2");
try {
//创建读取的对象
fileReader=new FileReader(filePath);
//读多个字符给buf数组 返回一个长度给readCharNum
while ((readCharNum=fileReader.read(buf))!=-1){
System.out.print(new String(buf,0,readCharNum));
/*
Method2
渡远荆门外,来从楚国游。
山随平野尽,江入大荒流。
*/
}
} catch (IOException e) {
e.printStackTrace();
}
finally {
try {
fileReader.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
FileWriter
package com.File_.FileBase;
import org.junit.jupiter.api.Test;
import java.io.FileWriter;
import java.io.IOException;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class Filewriter_ {
public static void main(String[] args) {
}
//使用 FileWriter 将 “风雨之后, 定见彩虹” 写入到 note.txt 文件中, 注意细节
@Test
public void Method1(){
String fileName="e:\\note.txt";
FileWriter fileWriter=null;
String Constent ="风雨之后,定见彩虹";
char chs[]={'a','a','x'};
try {
//创建输出流对象
fileWriter = new FileWriter(fileName,true);
// 3) write(int):写入单个字符
fileWriter.write('H');
// 4) write(char[]):写入指定数组
fileWriter.write(chs);
// 5) write(char[],off,len):写入指定数组的指定部分
fileWriter.write("hello".toCharArray(), 0, 3);
// 6) write(string) : 写入整个字符串
fileWriter.write(" 你好北京~");
fileWriter.write("风雨之后, 定见彩虹");
7) write(string,off,len):写入字符串的指定部分
fileWriter.write("上海天津", 0, 2);
//在数据量大的情况下, 可以使用循环操作.
char[] chars = Constent.toCharArray();//把字符串转换为字符数组
fileWriter.write(chars,0, chars.length);
System.out.println("写入成功...");
} catch (IOException e) {
e.printStackTrace();
} finally {
//关闭文件不关闭写入不了
try {
fileWriter.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
节点流和处理流
处理流的功能主要体现在以下两个方面:处理流的功能主要体现在以下两个方面:

模拟修饰器模式
package com.File_;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class simulation {
public static void main(String[] args) {
BufferReader_ bufferReader_ = new BufferReader_(new FileReader_());
bufferReader_.reader_.fileReader_();
}
}
class FileReader_ extends Reader_{
@Override
public void fileReader_() {
System.out.println("文件");
}
}
class StringReader_ extends Reader_{
@Override
public void stringReader_() {
System.out.println("字符串");
}
}
class BufferReader_ extends Reader_{
public Reader_ reader_=null;
public BufferReader_(Reader_ reader_) {
this.reader_ = reader_;
}
}
处理流-BufferedReader 和 BufferedWriter
package com.File_;
import java.io.*;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class BufferReader_ {
public static void main(String[] args) throws IOException {
String filePath="e:\\story.txt";
//把文件的读取方式放到BufferedReader
BufferedReader bufferReader = new BufferedReader(new FileReader(filePath));
String line;//按行读取
while((line=bufferReader.readLine())!=null){
System.out.println(line);
}
bufferReader.close();//关闭流
/*
public void close() throws IOException {
synchronized (lock) {
if (in == null)
return;
try {
in.close();//最终这还是关闭FileReader
} finally {
in = null;
cb = null;
}
}
}
*/
System.out.println("读取成功...");
}
}
BufferedWrite
public class BufferWrite_ {
public static void main(String[] args) throws IOException {
String filePath="e:\\YYY.txt";
//追加模式
BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(filePath,true));
bufferedWriter.write("hello world");
bufferedWriter.write("rnm");
bufferedWriter.close();//必须有close否则写入不进去,close这才真正实现写入功能
}
}
package com.File_;
import java.io.*;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class BufferedCopy_ {
public static void main(String[] args) throws IOException {
String filepath="e:\\note.txt";
String filepath1="e:\\note1.txt";
BufferedReader br =new BufferedReader(new FileReader(filepath));
BufferedWriter bw =new BufferedWriter(new FileWriter(filepath1));
String line;
//读出一行
while((line=br.readLine())!=null){
bw.write(line);//写入一行
//插入一个换行
bw.newLine();
}
System.out.println("拷贝完毕...");
br.close();
bw.close();
}
}
处理流-BufferedInputStream 和 BufferedOutputStream
package com.File_;
import java.io.*;
/**
* @author 你是大**吗?
* version 1.0
* 字节处理流
*/
@SuppressWarnings({"all"})
public class BufferedInputStream_ {
public static void main(String[] args) throws IOException {
String filepath="d:\\AAA.jpg";
String filepath1="d:\\AAA1.jpg";
BufferedInputStream bi = new BufferedInputStream(new FileInputStream(filepath));
BufferedOutputStream bo = new BufferedOutputStream(new FileOutputStream(filepath1));
byte by[]=new byte[1024];
int len=0;
//一次读出1024字节
while((len= bi.read(by))!=-1){
bo.write(by,0,len);
}
System.out.println("拷贝完毕...");
}
}
对象流-ObjectInputStream 和 ObjectOutputStream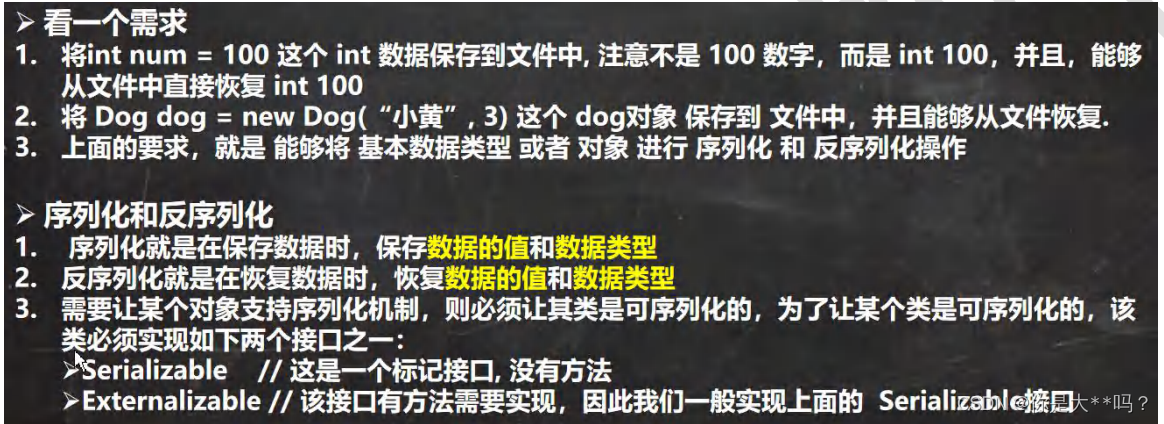
功能: 提供了对基本类型或对象类型的序列化和反序列化的方法
ObjectOutputStream 提供 序列化功能
ObjectInputStream 提供 反序列化功能
package com.File_;
import java.io.*;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class Test_ObjectIn {
public static void main(String[] args) throws IOException {
String filePath = "e:\\date.txt";
//ObjectOutputStream序列化文件到"date.dat"文件,底层还是用文件字节流
ObjectOutputStream oout = new ObjectOutputStream(new FileOutputStream(filePath));
oout.writeObject(new Dog());
oout.writeInt(100);//Integer实现了序列化接口
oout.writeBoolean(true);//Boolean实现了序列化接口
oout.close();
}
}
//实现了可序列化接口
class Dog implements Serializable {
String name = "大黄";
int age = 10;
}
package com.File_;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class Test_ObjectOut {
public static void main(String[] args) throws IOException, ClassNotFoundException {
//ObjectInputStream是反序列化
String filePath = "e:\\date.txt";
ObjectInputStream ooIn = new ObjectInputStream(new FileInputStream(filePath));
System.out.println(ooIn.readObject());//若是这个Dog不在同一个包要引用过来
System.out.println(ooIn.readInt());//一定要按循序读出来
System.out.println(ooIn.readBoolean());//一定要按循序读出来
ooIn.close();
System.out.println("完成反序列化...");
}
}
标准输入输出流 --standard
package com.File_;
import java.io.InputStream;
import java.io.PrintStream;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class standard {
public static void main(String[] args) {
//System.in--标准输入流
//public final static InputStream in = null;
//System.out--标准输出流
//System.out-- 标准输出流
//public final static PrintStream out = null;
PrintStream out=new PrintStream(System.out);
out.print("rnm,hello world");
}
}
转换流-InputStreamReader 和 OutputStreamWriter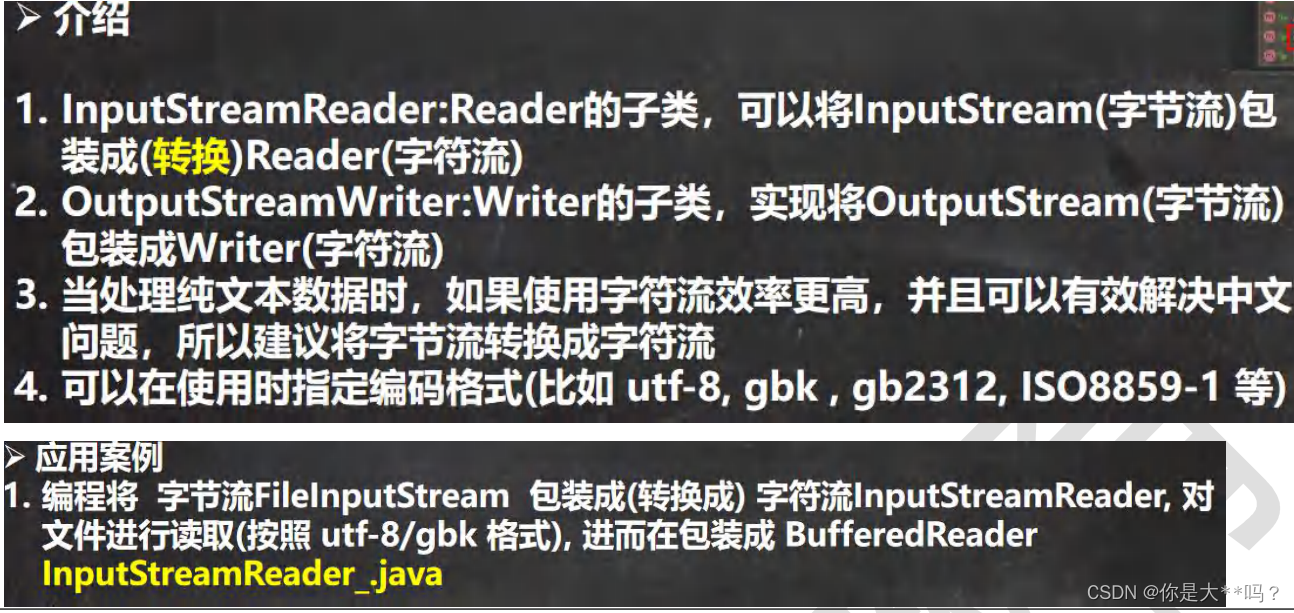
package com.File_;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class InputStreamReader_ {
public static void main(String[] args) throws IOException {
String filepath="e:\\Story.txt";
FileInputStream fileInputStream = new FileInputStream(filepath);
//将文件字节流转换为字符输入流
InputStreamReader inputStreamReader = new InputStreamReader(fileInputStream);
//将字符输入流包装为bufferedReader字符处理流
BufferedReader bufferedReader = new BufferedReader(inputStreamReader);
String line;
while((line=bufferedReader.readLine())!=null){
System.out.println(line);
}
bufferedReader.close();
}
}
package com.File_;
import java.io.BufferedWriter;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStreamWriter;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class OutPutStreamWrite_ {
public static void main(String[] args) throws IOException {
OutputStreamWriter gbk = new OutputStreamWriter(
new FileOutputStream("e:\\a.txt", true), "gbk");
BufferedWriter bufferedWriter = new BufferedWriter(gbk);
bufferedWriter.write("yyyyyyyy");
bufferedWriter.close();
}
}
打印流-PrintStream 和 PrintWriter
public class PrintWrite_ {
public static void main(String[] args) throws IOException {
//向屏幕输出
PrintWriter printWriter = new PrintWriter(System.out);
//向文件写入
// PrintWriter printWriter = new PrintWriter(new FileWriter("e:\\test1.txt",true));
printWriter.print("hello");
printWriter.close();//必须有关的动作否则不会输出
}
}
package com.File_;
import java.io.IOException;
import java.io.PrintStream;
/**
* @author 你是大**吗?
* version 1.0
* 按照字节写入
*/
@SuppressWarnings({"all"})
public class PrintStream_ {
public static void main(String[] args) throws IOException {
// PrintStream printStream = new PrintStream(System.out);
// printStream.print("10203123123");
// printStream.close();
PrintStream printStream = new PrintStream("e:\\BB.txt");
printStream.print("hwwwdw");
printStream.close();
}
}
Properties 类
基操
@SuppressWarnings({"all"})
public class Properties_ {
public static void main(String[] args) throws IOException {
Properties properties = new Properties();
//加载配置文件
properties.load(new FileReader("src\\com\\File_\\Mysql.Properties"));
//显示键值对
properties.list(System.out);
//根据键获得值
System.out.println( properties.getProperty("user"));
//root
}
}
package com.File_;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.util.Properties;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class Properties01 {
public static void main(String[] args) throws IOException {
Properties properties = new Properties();
//把键值对存到内存
properties.setProperty("user", "张三");//汉字会转为unicode码
properties.setProperty("pwd", "abc123");
properties.store(new FileOutputStream("src\\mysql.Properties"), "这是注解");
System.out.println("保存成功...");
//更新键值对
properties.setProperty("user","tom");
/*
#\u8FD9\u662F\u6CE8\u89E3 注解
#Wed May 25 15:47:35 CST 2022
user=\u5F20\u4E09
pwd=abc123
*/
}
}
习题1
package com.File_;
import java.io.*;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class Practical1 {
public static void main(String[] args) throws IOException {
//判断e盘下是否有文件夹mytemp 没有就创建
String filePath = "e:\\mytemp";
File file = new File(filePath);
if (!file.exists()) {
System.out.println("没有呢,我创建");
file.mkdir();
System.out.println("创建成功" + file.getName());
}
String filePath1 = "e:\\mytemp\\hello.txt";
File file1 = new File(filePath1);
if (!file1.exists()) {
System.out.println("没有,我创建");
file1.createNewFile();//创建文件
System.out.println("创建成功");
}
FileWriter fileWriter = new FileWriter(file1);
fileWriter.write("hello,world~");
fileWriter.close();
System.out.println("写入成功");
FileReader fileReader = new FileReader(file1);
BufferedReader bufferedReader = new BufferedReader(fileReader);
String line;
while ((line = bufferedReader.readLine()) != null) {
System.out.println(line);
}
bufferedReader.close();
}
}
习题2
package com.File_;
import java.io.*;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class Practical2 {
public static void main(String[] args) throws IOException {
//默认utf-8编码
//BufferedReader bufferedReader = new BufferedReader(new FileReader("e:\\story.txt"));
//文件字节输入流
FileInputStream fileReader = new FileInputStream("e:\\story.txt");
//gbk当前文件编码
InputStreamReader inputStreamReader = new InputStreamReader(fileReader, "gbk");
BufferedReader bufferedReader1 = new BufferedReader(inputStreamReader);
String line;
int num = 0;
while ((line = bufferedReader1.readLine()) != null) {
System.out.println((++num) + " " + line);
/**
* gbk编码
* 1 渡远荆门外,来从楚国游。
* 2 山随平野尽,江入大荒流。
* 3 hello king
*/
}
// while((line=bufferedReader.readLine())!=null){
// //System.out.println((++num)+" "+line);
// /**
// * 默认utf-8编码
// * 1 渡远荆门外,来从楚国游。
// * 2 山随平野尽,江入大荒流。
// * 3 hello king
// */
// System.out.println((++num)+" "+line);
//
// }
// bufferedReader.close();
bufferedReader1.close();
}
}
习题3
package com.File_;
import java.io.*;
import java.util.Properties;
/**
* @author 你是大**吗?
* version 1.0
*/
@SuppressWarnings({"all"})
public class Practical3 {
public static void main(String[] args) throws IOException, ClassNotFoundException {
//编写一个dog.Properties
Properties properties = new Properties();
//存到了内存
properties.setProperty("name","OOO");
properties.setProperty("age","10");
properties.setProperty("color","red");
properties.store(new FileOutputStream("dog.Properties"),"Dog~");
Dog_ dog_ = new Dog_();
//根据键获取值
dog_.setName(properties.getProperty("name"));
dog_.setAge(properties.getProperty("age"));
dog_.setColor(properties.getProperty("color"));
System.out.println(dog_);
//把dog对象系列化到dog.dat
//ObjectOutputStream就是就是专门搞序列化的
ObjectOutputStream objFile = new ObjectOutputStream(new FileOutputStream("e:\\dog.txt"));
objFile.writeObject(dog_);
//反序列化
ObjectInputStream objectIn = new ObjectInputStream(new FileInputStream("e:\\dog.txt"));
Object o = objectIn.readObject();
System.out.println(o.getClass()+" "+(Dog_)o);
}
}
class Dog_ implements Serializable {
public String name;
public String age;
public String color;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
public String getColor() {
return color;
}
public void setColor(String color) {
this.color = color;
}
@Override
public String toString() {
return "Dog_{" +
"name='" + name + '\'' +
", age='" + age + '\'' +
", color='" + color + '\'' +
'}';
}
}