el-carousel轮播图
轮播图循环,每一项再用循环
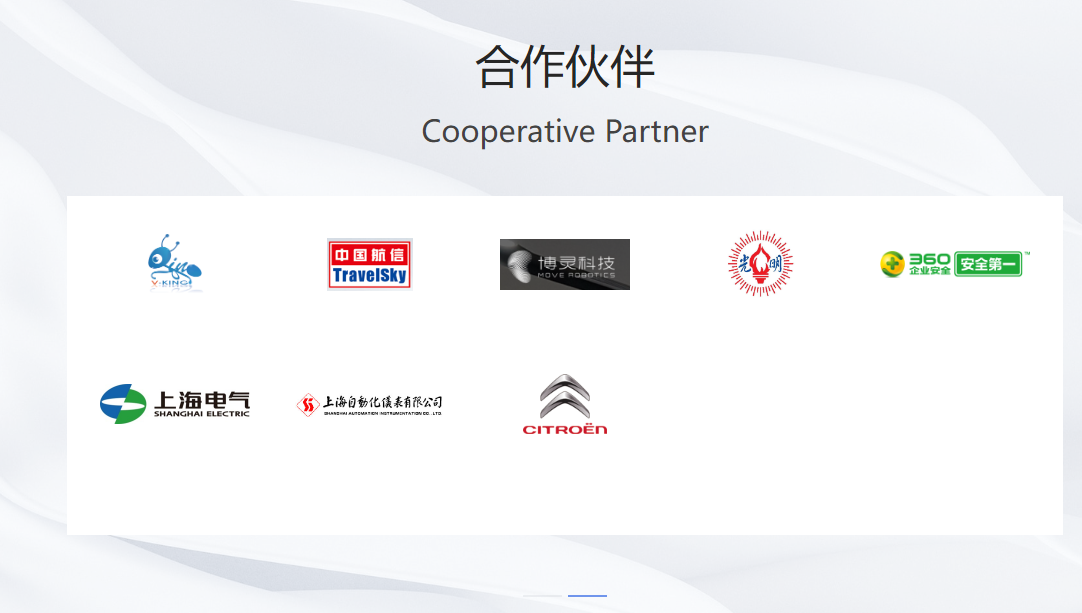
<el-carousel height="452px" :autoplay="false" indicator-position="outside">
<el-carousel-item v-for="(item, index) in cooperativePartnerData" :key="index">
<div class="content">
<div class="step-item" v-for="(tab, i) in item.list" :key="i">
<img :src="$getImageUrl(tab.img)" alt="" />
</div>
</div>
</el-carousel-item>
</el-carousel>
// 合作伙伴数据
const cooperativePartnerData = reactive([
{
list: [
{
img: "assets/img/partner01.png",
},
{
img: "assets/img/partner02.png",
},
{
img: "assets/img/partner03.png",
},
{
img: "assets/img/partner04.png",
},
{
img: "assets/img/partner05.png",
},
{
img: "assets/img/partner06.png",
},
{
img: "assets/img/partner07.png",
},
{
img: "assets/img/partner08.png",
},
{
img: "assets/img/partner09.png",
},
{
img: "assets/img/partner10.png",
},
{
img: "assets/img/partner11.png",
},
{
img: "assets/img/partner12.png",
},
{
img: "assets/img/partner13.png",
},
{
img: "assets/img/partner14.png",
},
{
img: "assets/img/partner15.png",
},
]
},
{
list: [
{
img: "assets/img/partner16.png",
},
{
img: "assets/img/partner17.png",
},
{
img: "assets/img/partner18.png",
},
{
img: "assets/img/partner19.png",
},
{
img: "assets/img/partner20.png",
},
{
img: "assets/img/partner21.png",
},
{
img: "assets/img/partner22.png",
},
{
img: "assets/img/partner23.png",
},]
}
]);
swiper轮播图
swiper例1
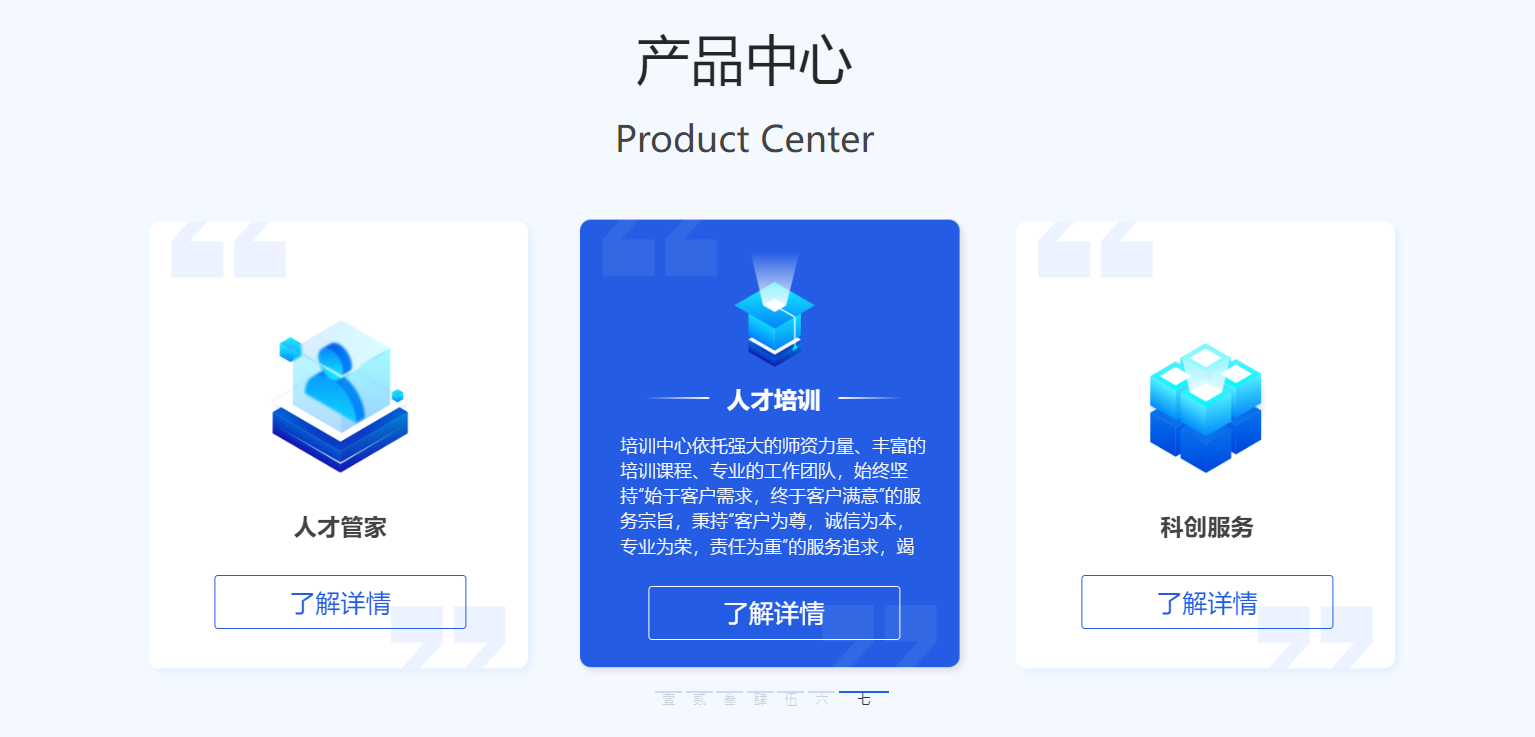
参考:
1.renderBullet(index, className)_Swiper参数选项
<swiper :loop="swiper_options.loop" :centeredSlides="swiper_options.centeredSlides" :slides-per-view="3"
:space-between="44" navigation :pagination="swiper_options.pagination" :autoplay="swiper_options.autoplay">
<swiper-slide v-for="item in productCenterData" :key="item.id" v-slot="{ isActive }">
<div :class="['swiperImg', isActive ? 'swiperImgActive' : '']">
<img :src="$getImageUrl(item.img)" alt="" />
</div>
<div :class="['swiperTitle', isActive ? 'swiperTitleActive' : '']">
<div class="titleLeft"></div>
<div class="titleCenter">{{ item.title }}</div>
<div class="titleRight"></div>
</div>
<div :class="['swiperContent', isActive ? 'swiperContentActive' : '']">
{{ item.content }}
</div>
<div :class="['swiperButton', isActive ? 'swiperButtonActive' : '']">
<button>了解详情</button>
</div>
</swiper-slide>
</swiper>
import SwiperCore, { Pagination, Autoplay, Navigation } from "swiper";
import { Swiper, SwiperSlide } from "swiper/vue";
import "swiper/swiper.scss";
import "swiper/components/pagination/pagination.scss";
import "swiper/components/navigation/navigation.scss";
import "swiper/swiper-bundle.css";
import { reactive, onMounted, ref } from "vue";
SwiperCore.use([Autoplay, Pagination, Navigation]);
// 产品中心数据
const productCenterData = reactive([
{
id: 1,
img: "assets/img/ic_scientific_innovation_service_normal.png",
title: "科创服务",
content:
"详细了解您的企业文化、公司背景、行业和产品特点,与您进行充分有效沟通,对所需招聘的职位进行讨论和分析",
},
{
id: 2,
img: "assets/img/ic_staff_care_normal.png",
title: "员工关怀",
content:
"提供候选人离/入职辅导,确保候选人入职,协助双方尽快磨合",
},
{
id: 3,
img: "assets/img/ic_consultation_and_planning_normal.png",
title: "规划与咨询",
content:
"对候选人信息分析、过滤,包括职位现状、沟通能力、离职动机、专业能力、薪酬水准等;筛选并面试",
},
{
id: 4,
img: "assets/img/ic_legal_advice_and_services_normal.png",
title: "法律咨询与服务",
content:
"因候选人自身原因而离职或没能通过保证期,将为您在保证期内免费推荐候选人",
},
{
id: 5,
img: "assets/img/ic_talent_recruitment_normal.png",
title: "人才招聘",
content:
"详细了解您的企业文化、公司背景、行业和产品特点,与您进行充分有效沟通,对所需招聘的职位进行讨论和分析",
},
{
id: 6,
img: "assets/img/ic_talent_butler_normal.png",
title: "人才管家",
content:
"提供候选人离/入职辅导,确保候选人入职,协助双方尽快磨合",
},
{
id: 7,
img: "assets/img/ic_talent_training_hover.png",
title: "人才培训",
content:
"培训中心依托强大的师资力量、丰富的培训课程、专业的工作团队,始终坚持“始于客户需求,终于客户满意”的服务宗旨,秉持“客户为尊,诚信为本,专业为荣,责任为重”的服务追求,竭诚为客户提供专业化、高效率的人才培训服务。",
},
]);
// swiper相关配置属性放在swiper_options这个变量里
let swiper_options = reactive({
autoplay: {
delay: 3000,
pauseOnMouseEnter: true, //鼠标移入时停止自动轮播
},
pagination: {
//将指示器设置为可点击
clickable: true,
//自定义指示器
renderBullet: function (index, className) {
console.log(index + '099999');
let text = ref()
switch (index) {
case 0: text = '壹'; break;
case 1: text = '贰'; break;
case 2: text = '叁'; break;
case 3: text = '肆'; break;
case 4: text = '伍'; break;
case 5: text = '六'; break;
case 6: text = '七'; break;
case 7: text = '八'; break;
}
return '<span class="' + className + '">' + text + '</span>';
},
},
centeredSlides: true,//让指示器对应的每一项居中
loop: true //轮播图中的每一项循环完成后,首尾相接,重新循环。
});
加了scoped会不生效
加了scoped会不生效
<style lang="scss">
// swiper分页器
.swiper-pagination {
.swiper-pagination-bullets {
bottom: -60px;
z-index: 9999;
}
.swiper-pagination-bullet {
width: 30px;
height: 2px;
background-color: #245de3;
}
.swiper-pagination-bullet-active {
width: 56px;
height: 2px;
background-color: #245de3;
}
}
// swiper左右箭头
.swiper-button-prev {
display: none;
}
.swiper-button-next {
display: none;
}
.swiper-slide-prev {
background: url("@/assets/img/bg_product_center_normal.png") no-repeat;
}
.swiper-slide-active {
background: url("@/assets/img/bg_product_center_hover.png") no-repeat !important;
width: 438px !important;
}
.swiper-slide-next {
background: url("@/assets/img/bg_product_center_normal.png") no-repeat;
}
.swiper-container-horizontal>.swiper-pagination-bullets .swiper-pagination-bullet {
margin: 0 4px 0 0 !important;
border-radius: 0 !important;
}
</style>
<style lang="scss" scoped>
.swiper-container {
width: 1400px;
height: 557px;
.swiperImg {
padding-top: 116px;
padding-bottom: 38px;
width: 172px;
margin: 0 auto;
img {
display: inline-block;
margin: 0 auto;
width: 172px;
height: 172px;
}
}
.swiperImgActive {
width: 128px;
padding-top: 40px !important;
padding-bottom: 16px !important;
margin: 0 auto;
img {
display: inline-block;
margin: 0 auto;
width: 128px;
height: 128px;
}
}
.swiperTitle {
margin-bottom: 36px;
.titleLeft {
display: none;
}
.titleCenter {
height: 37px;
font-size: 26px;
text-align: center;
font-weight: 600;
color: #434343;
line-height: 37px;
}
.titleRight {
display: none;
}
}
.swiperTitleActive {
display: flex;
align-items: center;
justify-content: center;
margin-bottom: 20px;
.titleLeft {
display: block;
width: 70px;
height: 1px;
border: 2px solid;
border-image: linear-gradient(270deg,
rgba(255, 255, 255, 1),
rgba(36, 93, 227, 0)) 2 2;
}
.titleCenter {
color: white;
margin: 0 20px;
}
.titleRight {
display: block;
width: 70px;
height: 1px;
border: 2px solid;
border-image: linear-gradient(270deg,
rgba(36, 93, 227, 0),
rgba(255, 255, 255, 1)) 2 2;
}
}
.swiperContent {
display: none;
}
.swiperContentActive {
display: block;
width: 342px;
height: 140px;
font-size: 20px;
margin: 0 auto;
font-weight: 300;
color: #ffffff;
margin-bottom: 30px;
overflow: hidden;
text-overflow: ellipsis;
}
.swiperButton {
text-align: center;
button {
cursor: pointer;
width: 280px;
height: 60px;
border-radius: 4px;
border: 1px solid #245de3;
background: none;
font-size: 28px;
text-align: center;
color: #245de3;
}
}
.swiperButtonActive {
button {
border: 1px solid #ffffff;
color: #ffffff;
}
}
}
</style>
swiper例2--自定义指示器
swiper的其他属性
:centeredSlides="true" //每页有三个部分时,指示器对应的div居中显示
:loop="true" //循环完之后从头开始循环,首尾相接
slides-per-view="3" //同时显示三个图片
:space-between="40" //三个图片之间的间距是40px
pagination: {
el: '.swiper-pagination',//分页器自动隐藏
clickable :true, //分页器可点击
},
watchOverflow:true,//当没有足够的slide切换时,例如只有1个slide(非loop),swiper会失效且隐藏导航。
grabCursor : true,//设置为true时,鼠标覆盖Swiper时指针会变成手掌形状,拖动时指针会变成抓手形状。
autoplay: {//启动自动切换,具体选项如下:
delay: 3000,//自动切换的时间间隔,单位ms
stopOnLastSlide: false,//如果设置为true,当切换到最后一个slide时停止自动切换。(loop模式下无效)。
disableOnInteraction: false,//用户操作swiper之后,是否禁止autoplay。默认为true:停止。
},
分页器和左右箭头不显示是import没有引入完全。
swiper版本不同其内容略微有差别。
部分样式如果加了scoped会不显示。
isActive时swiper自带的动态样式。