根据输入的分数成绩列表,给排名前10派发奖学金。
(本笔记适合能熟练应用列表数据结构的 coder 翻阅)
-
Python 官网:https://www.python.org/
-
Free:大咖免费“圣经”教程《 python 完全自学教程》,不仅仅是基础那么简单……
地址:https://lqpybook.readthedocs.io/
自学并不是什么神秘的东西,一个人一辈子自学的时间总是比在学校学习的时间长,没有老师的时候总是比有老师的时候多。
—— 华罗庚

本文质量分:
CSDN质量分查询入口:http://www.csdn.net/qc
◆ 派发奖学金
1、题目描述
2、解题思路
由题目描述可知分数最高的前十名可分得奖学金(无关分数高低,只要排名前十就有奖学金)。所以只需倒序重排分数列表,依序把奖学金分发给前十名。第10以后,奖学金都是0。这有两种实现方式——
-
将奖学金从高到低放入列表:
[500, 500, 300, 300, 300, 300, 100, 100, 100, 100] -
其一:
用索引遍历成绩列表,索引不于9的按序(索引)打印其奖学金,其后奖学金都打印0。 -
其二:
把奖学金列表长度变得与学生成绩列表长度一致,不足追加0。索引遍历,依序打印成绩和奖学金。Python对于此“算法”,有zip()让遍历代码变得简洁优雅。
3、代码实现
3.1 “普适”算法(适宜任何高级语言)
用列表结构数据,索引输出。此种写法适用所有“语言”。
- 列表短长
def distribution(n, scores): # 列表短长普适写法。
''' 派发奖学金 '''
moneys = [500, 500, 300, 300, 300, 300, 100, 100, 100, 100] # 奖学金列表。
result = [] # 输出成绩奖学金对列表。
scores.sort(reverse=True) # 全班分数排名次。
for i in range(n):
money = moneys[i] if i < 10 else 0 # 前十名奖学金都为0。
result.append(f"{scores[i]} {money}")
return '\n'.join(result)
- 列表等长
def distribution(n, scores): # 列表等长普适写法。
''' 派发奖学金 '''
moneys = [500, 500, 300, 300, 300, 300, 100, 100, 100, 100] + [0]*(n-10) # 奖学金列表等长成绩列表。
result = [] # 输出成绩奖学金对列表。
scores.sort(reverse=True) # 全班分数排名次。
for i in range(n):
result.append(f"{scores[i]} {moneys[i]}")
return '\n'.join(result)
3.2 Python 专属zip() 写法代码
-
python 代码运行效果截屏图片
-
Python 专属zip()函数“算法”
def distribution(n, scores):
''' 派发奖学金 '''
money = [500, 300, 100] # 奖学金字等级列表。
num = (2, 4, 4) # 瓜分奖学金人数。
moneys = []
# 循环生成前10名奖学金列表。
for i,j in zip(money, num):
moneys += [i]*j
moneys += [0]*(n-10) # 全员奖学金。
scores.sort(reverse=True) # 全班分数排名次。
result = [f"{s} {m}" for s,m in zip(scores, moneys)] # 列表解析输出行字符串。
return '\n'.join(result) # 回车符拼接字符串输出字符列表。
- 如果直接写出奖学金列表,代码更“精简”
def distribution(n, scores): # Python 专属zip()“精简”写法。
''' 派发奖学金 '''
moneys = [500, 500, 300, 300, 300, 300, 100, 100, 100, 100] + [0]*(n-10) # 奖学金列表,与成绩列表等长。
scores.sort(reverse=True) # 全班分数排名次。
return '\n'.join([f"{s} {m}" for s,m in zip(scores, moneys)]) # 回车符拼接字符串输出字符列表。
4、完整源码
(源码较长,点此跳过源码)
#!/sur/bin/nve python
# coding: utf-8
def distribution(n, scores): # Python 专属zip()写法。
''' 派发奖学金 '''
money = [500, 300, 100] # 奖学金字等级列表。
num = (2, 4, 4) # 瓜分奖学金人数。
moneys = []
# 循环生成前10名奖学金列表。
for i,j in zip(money, num):
moneys += [i]*j
moneys += [0]*(n-10) # 全员奖学金。
scores.sort(reverse=True) # 全班分数排名次。
result = [f"{s} {m}" for s,m in zip(scores, moneys)] # 列表解析输出行字符串。
return '\n'.join(result) # 回车符拼接字符串输出字符列表。
def distribution6(n, scores): # Python 专属zip()“精简”写法。
''' 派发奖学金 '''
moneys = [500, 500, 300, 300, 300, 300, 100, 100, 100, 100] + [0]*(n-10) # 奖学金列表,与成绩列表等长。
scores.sort(reverse=True) # 全班分数排名次。
return '\n'.join([f"{s} {m}" for s,m in zip(scores, moneys)]) # 回车符拼接字符串输出字符列表。
def distribution6(n, scores): # 列表短长普适写法。
''' 派发奖学金 '''
moneys = [500, 500, 300, 300, 300, 300, 100, 100, 100, 100] # 奖学金列表。
result = [] # 输出成绩奖学金对列表。
scores.sort(reverse=True) # 全班分数排名次。
for i in range(n):
money = moneys[i] if i < 10 else 0 # 前十名奖学金都为0。
result.append(f"{scores[i]} {money}")
return '\n'.join(result)
def distribution6(n, scores): # 列表等长普适写法。
''' 派发奖学金 '''
moneys = [500, 500, 300, 300, 300, 300, 100, 100, 100, 100] + [0]*(n-10) # 奖学金列表等长成绩列表。
result = [] # 输出成绩奖学金对列表。
scores.sort(reverse=True) # 全班分数排名次。
for i in range(n):
result.append(f"{scores[i]} {moneys[i]}")
return '\n'.join(result)
if __name__ == '__main__':
in_s0 = '''12
100 98 90 91 89 78 81 88 82 80 85 93'''
except_out = '''100 500
98 500
93 300
91 300
90 300
89 300
88 100
85 100
82 100
81 100
80 0
78 0'''
in_s = in_s0.split('\n')
n = int(in_s[0])
scores = list(map(int, in_s[1].split()))
print(f"\n输入:\n{in_s0}\n预期输出:\n{except_out}\n\n实际输出:\n{distribution(n, scores)}")
上一篇: 字符串列表分类计算平均值(给定一字符串列表数据,按颜色分类计算价格平均值并写入列表)
下一篇:
我的HOT博:
本次共计收集 219 篇博文笔记信息,总阅读量 37.12w,平均阅读量 1694。已生成 25 篇阅读量不小于 3000 的博文笔记索引链接。数据采集于 2023-07-18 05:54:34 完成,用时 5 分 1.76 秒。
- 让QQ群昵称色变的神奇代码
( 56965 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122566500
点赞:24 踩 :0 收藏:81 打赏:0 评论:17
本篇博文笔记于 2022-01-18 19:15:08 首发,最晚于 2022-01-20 07:56:47 修改。 - ChatGPT国内镜像站初体验:聊天、Python代码生成等
( 54947 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/129035387
点赞:125 踩 :0 收藏:789 打赏:0 评论:75
本篇博文笔记于 2023-02-14 23:46:33 首发,最晚于 2023-07-03 05:50:55 修改。 - pandas 数据类型之 DataFrame
( 8875 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124525814
点赞:6 踩 :0 收藏:31 打赏:0 评论:0
本篇博文笔记于 2022-05-01 13:20:17 首发,最晚于 2022-05-08 08:46:13 修改。 - 个人信息提取(字符串)
( 6995 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124244618
点赞:1 踩 :0 收藏:12 打赏:0 评论:0
本篇博文笔记于 2022-04-18 11:07:12 首发,最晚于 2022-04-20 13:17:54 修改。 - 罗马数字转换器|罗马数字生成器
( 6741 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122592047
点赞:0 踩 :0 收藏:1 打赏:0 评论:0
本篇博文笔记于 2022-01-19 23:26:42 首发,最晚于 2022-01-21 18:37:46 修改。 - Python字符串居中显示
( 6644 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122163023
点赞:1 踩 :0 收藏:6 打赏:0 评论:1
本篇博文笔记于 2021-12-26 23:35:29 发布。 - Python列表(list)反序(降序)的7种实现方式
( 5755 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/128271700
点赞:5 踩 :0 收藏:18 打赏:0 评论:8
本篇博文笔记于 2022-12-11 23:54:15 首发,最晚于 2023-03-20 18:13:55 修改。 - 斐波那契数列的递归实现和for实现
( 5429 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122355295
点赞:4 踩 :0 收藏:2 打赏:0 评论:8
本篇博文笔记于 2022-01-06 23:27:40 发布。 - 练习:字符串统计(坑:f‘string‘报错)
( 4998 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121723096
点赞:0 踩 :0 收藏:1 打赏:0 评论:0
本篇博文笔记于 2021-12-04 22:54:29 发布。 - python清屏
( 4773 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/120762101
点赞:0 踩 :0 收藏:6 打赏:0 评论:0
本篇博文笔记于 2021-10-14 13:47:21 发布。 - 练习:尼姆游戏(聪明版/傻瓜式•人机对战)
( 4763 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121645399
点赞:14 踩 :0 收藏:42 打赏:0 评论:0
本篇博文笔记于 2021-11-30 23:43:17 发布。 - 回车符、换行符和回车换行符
( 4713 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/123109488
点赞:1 踩 :0 收藏:2 打赏:0 评论:0
本篇博文笔记于 2022-02-24 13:10:02 首发,最晚于 2022-02-25 20:07:40 修改。 - 练习:生成100个随机正整数
( 4109 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122558220
点赞:1 踩 :0 收藏:6 打赏:0 评论:0
本篇博文笔记于 2022-01-18 13:31:36 首发,最晚于 2022-01-20 07:58:12 修改。 - 密码强度检测器
( 4101 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121739694
点赞:1 踩 :0 收藏:4 打赏:0 评论:0
本篇博文笔记于 2021-12-06 09:08:25 首发,最晚于 2022-11-27 09:39:39 修改。 - 罗马数字转换器(用罗马数字构造元素的值取模实现)
( 3985 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122608526
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2022-01-20 19:38:12 首发,最晚于 2022-01-21 18:32:02 修改。 - 我的 Python.color() (Python 色彩打印控制)
( 3789 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/123194259
点赞:2 踩 :0 收藏:7 打赏:0 评论:0
本篇博文笔记于 2022-02-28 22:46:21 首发,最晚于 2022-03-03 10:30:03 修改。 - 练习:班里有人和我同生日难吗?(概率probability、蒙特卡洛随机模拟法)
( 3770 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124424935
点赞:1 踩 :0 收藏:4 打赏:0 评论:0
本篇博文笔记于 2022-04-26 12:46:25 首发,最晚于 2022-04-27 21:22:07 修改。 - 练习:仿真模拟福彩双色球——中500w巨奖到底有多难?跑跑代码就晓得了。
( 3475 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/125415626
点赞:3 踩 :0 收藏:4 打赏:0 评论:3
本篇博文笔记于 2022-06-22 19:54:20 首发,最晚于 2022-06-23 22:41:33 修改。 - random.sample()将在python 3.9x后续版本中被弃用
( 3338 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/120657230
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2021-10-08 18:35:09 发布。 - 聊天消息敏感词屏蔽系统(字符串替换 str.replace(str1, *) )
( 3309 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/124539589
点赞:4 踩 :0 收藏:2 打赏:0 评论:3
本篇博文笔记于 2022-05-02 13:02:39 首发,最晚于 2022-05-21 06:10:42 修改。 - Linux 脚本文件第一行的特殊注释符(井号和感叹号组合)的含义
( 3282 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/123087606
点赞:0 踩 :0 收藏:4 打赏:0 评论:3
本篇博文笔记于 2022-02-23 13:08:07 首发,最晚于 2022-04-04 23:52:38 修改。 - 练习:求列表(整数列表)平衡点
( 3148 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/121737612
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2021-12-05 23:28:10 发布。 - 练习:银行复利计算(用 for 循环解一道初中小题)
( 3043 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/123854548
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2022-03-30 20:06:37 首发,最晚于 2022-04-06 18:15:16 修改。 - 练习:小炼二维数组
( 3030 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/125175592
点赞:9 踩 :0 收藏:5 打赏:0 评论:9
本篇博文笔记于 2022-06-07 23:54:43 首发,最晚于 2022-06-08 00:31:49 修改。 - 练习:柱状图中最大矩形
( 3029 阅读)
博文地址:https://blog.csdn.net/m0_57158496/article/details/122032365
点赞:0 踩 :0 收藏:0 打赏:0 评论:0
本篇博文笔记于 2021-12-19 23:47:07 发布。
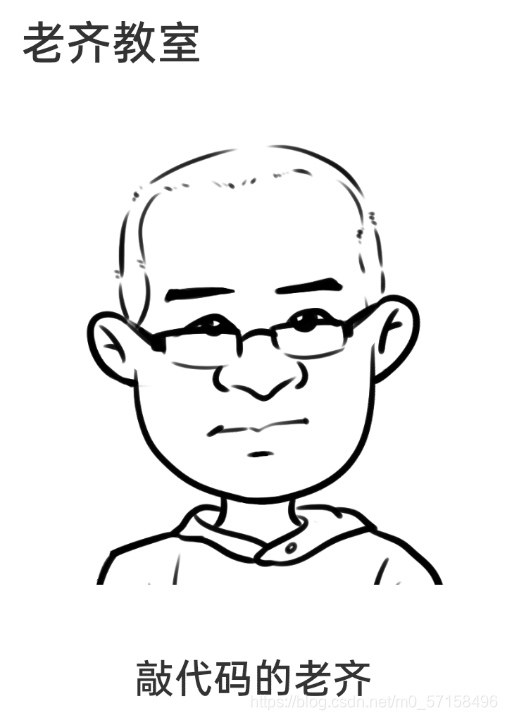
精品文章:
- 好文力荐:齐伟书稿 《python 完全自学教程》 Free连载(已完稿并集结成书,还有PDF版本百度网盘永久分享,点击跳转免费🆓下载。)
- OPP三大特性:封装中的property
- 通过内置对象理解python'
- 正则表达式
- python中“*”的作用
- Python 完全自学手册
- 海象运算符
- Python中的 `!=`与`is not`不同
- 学习编程的正确方法
来源:老齐教室
◆ Python 入门指南【Python 3.6.3】
好文力荐:
- 全栈领域优质创作者——[寒佬](还是国内某高校学生)博文“非技术文—关于英语和如何正确的提问”,“英语”和“会提问”是编程学习的两大利器。
- 【8大编程语言的适用领域】先别着急选语言学编程,先看它们能干嘛
- 靠谱程序员的好习惯
- 大佬帅地的优质好文“函数功能、结束条件、函数等价式”三大要素让您认清递归
CSDN实用技巧博文: