头文件
#include<iostream>
using namespace std;
class Node
{
private:
int _val;
Node* _next;
Node* _pre;
public:
Node(int val = int())
:_val(val),_next(nullptr),_pre(nullptr)
{
}
Node(const Node& src)
:_val(src._val),_next(nullptr),_pre(nullptr)
{
}
Node& operator =(const Node &src)
{
if (&src == this)
{
return *this;
}
_val = src._val;
_next = src._next;
_pre = src._pre;
}
int get_val();
void set_val(int val);
Node* get_next();
void set_next(Node* next);
Node* get_pre();
void set_pre(Node* pre);
};
class List
{
public:
typedef Node LIST_NODE_TYPE;
List();
~List();
void push_back(int val);
void pop_back();
void push_front(int val);
void pop_front();
bool Is_empty();
void show()
{
if (Is_empty())
{
cout << "list is empty!" << endl;
}
LIST_NODE_TYPE* p = _head->get_next();
while (p!=NULL)
{
cout <<p->get_val()<< " ";
p = p->get_next();
}
}
private:
LIST_NODE_TYPE* _head;
LIST_NODE_TYPE* _tail;
};
函数实现
#include"homework2(链表).h"
int Node::get_val()
{
return _val;
}
void Node::set_val(int val)
{
_val = val;
}
Node* Node::get_next()
{
return _next;
}
void Node::set_next(Node* next)
{
_next = next;
}
Node* Node::get_pre()
{
return _pre;
}
void Node::set_pre(Node* pre)
{
_pre = pre;
}
List::List()
{
_head = new LIST_NODE_TYPE();
_tail = _head;
}
List::~List()
{
while (!Is_empty())
{
pop_back();
}
delete _head;
_head = _tail = NULL;
}
void List::push_back(int val)
{
LIST_NODE_TYPE* node = new LIST_NODE_TYPE(val);
if (Is_empty())
{
_head->set_next(node);
_head->set_pre(_tail);
_tail = node;
_tail->set_pre(_head);
return;
}
node->set_pre(_tail);
_tail->set_next(node);
_tail = node;
return;
}
void List::pop_back()
{
if (Is_empty())
{
cout << "list is empty" << endl;
return;
}
_tail = _tail->get_pre();
_tail->set_next(NULL);
}
void List::push_front(int val)
{
LIST_NODE_TYPE* node = new LIST_NODE_TYPE(val);
if (Is_empty())
{
push_back(val);
}
node->set_next(_head->get_next());
node->set_pre(_head);
_head->get_next()->set_pre(node);
_head->set_next(node);
return;
}
void List::pop_front()
{
if (Is_empty())
{
cout << "list is empty!" << endl;
return;
}
_head->set_next(_head->get_next()->get_next());
return;
}
bool List::Is_empty()
{
return _head == _tail;
}
测试:
int main()
{
List p1;
p1.push_back(10);
p1.push_front(20);
p1.pop_front();
for (int i = 0; i < 10; i++)
{
p1.push_front(i + 10);
}
p1.show();
}
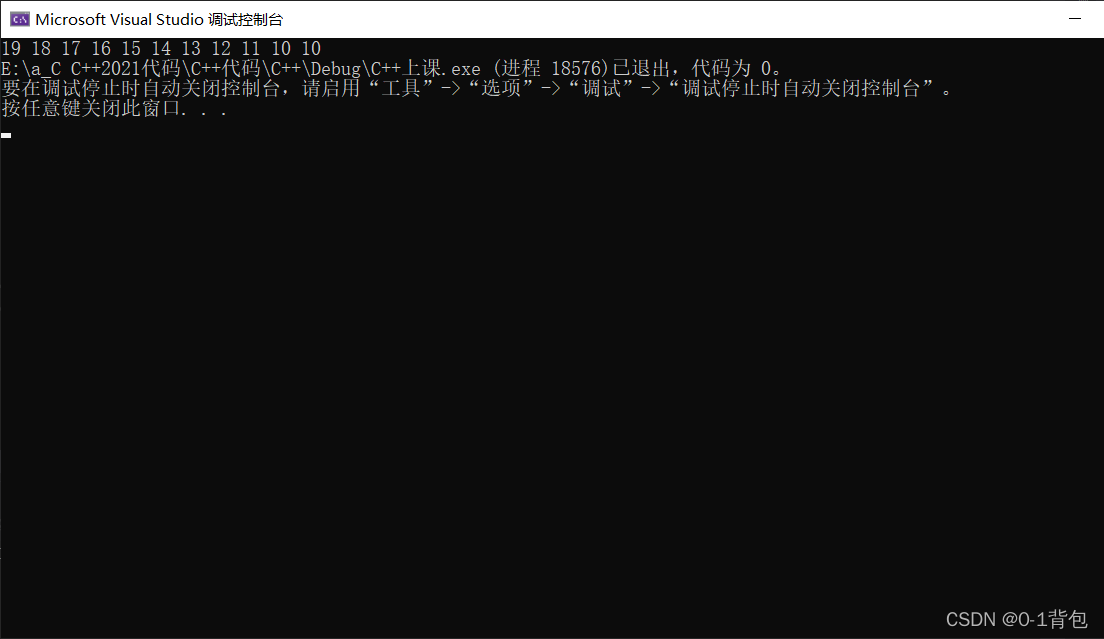