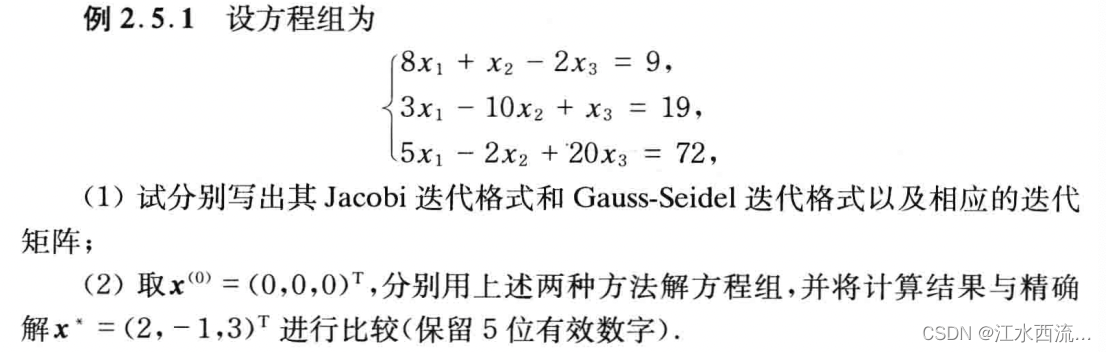
def jacobi(x1,x2,x3,count=1):
y1=-2*x2 - 3*x3 + 6
y2=-4/5*x1 - 6/5*x3 + 3.2
y3=-7/9*x1 - 8/9*x2 + 24/9
if abs(y1-x1)<0.00001 and abs(y2-x2)<0.00001 and abs(y3-x3)<0.00001:
print('最终的计算结果为%s、%s和%s' %(y1,y2,y3))
else:
if count<10:
print('第%s次迭代的计算结果为%s、%s和%s' %(count,y1,y2,y3))
x1,x2,x3,count = y1,y2,y3,count+1
return jacobi(x1,x2,x3,count)
def seidel(x1,x2,x3,count=1):
y1=(-1/8)*x2 + 1/4*x3 + 9/8
y2=3/10*y1 + 1/10*x3 - 19/10
y3=(-1/4)*y1 + 1/10*y2 + 3.6
if abs(y1-x1)<0.00001 and abs(y2-x2)<0.00001 and abs(y3-x3)<0.00001:
print('最终的计算结果为%s、%s和%s' %(y1,y2,y3))
else:
print('第%s次迭代的计算结果为%s、%s和%s' %(count,y1,y2,y3))
x1,x2,x3,count = y1,y2,y3,count+1
return seidel(x1,x2,x3,count)
def sor(x1,x2,x3,w,count=1):
y1=(1-w)*x1 + w*((-1/8)*x2 + 1/4*x3 + 9/8)
y2=(1-w)*x2 + w*(3/10*y1 + 1/10*x3 - 19/10)
y3=(1-w)*x3 + w*((-1/4)*y1 + 1/10*y2 + 3.6)
if abs(y1-x1)<0.00001 and abs(y2-x2)<0.00001 and abs(y3-x3)<0.00001:
print('最终的计算结果为%s、%s和%s' %(y1,y2,y3))
else:
print('第%s次迭代的计算结果为%s、%s和%s' %(count,y1,y2,y3))
x1,x2,x3,count = y1,y2,y3,count+1
return sor(x1,x2,x3,w,count)