一、认识原型
1、函数访问原型
- 每一个函数都有一个
原型
(是一个空间,或者是一个对象, 内部能存储一些东西) - 构造函数,
本质
上也是一个函数
, 所以他也有这个原型
- 原型内部都有一个
constructor
,这个属性表明当前这个原型是该函数的 - 函数访问原型:
函数.prototype
function Person(name, age) {
this.name = name;
this.age = age;
}
console.log(Person.prototype);
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.a = '我是后续通过 JS 代码添加到函数的原型内部的属性';
Person.prototype.sayHi = () => {
console.log('你好');
}
const p1 = new Person('QF001', 18);
const p2 = new Person('QF002', 28);
console.log(p1);
console.log(Person.prototype);
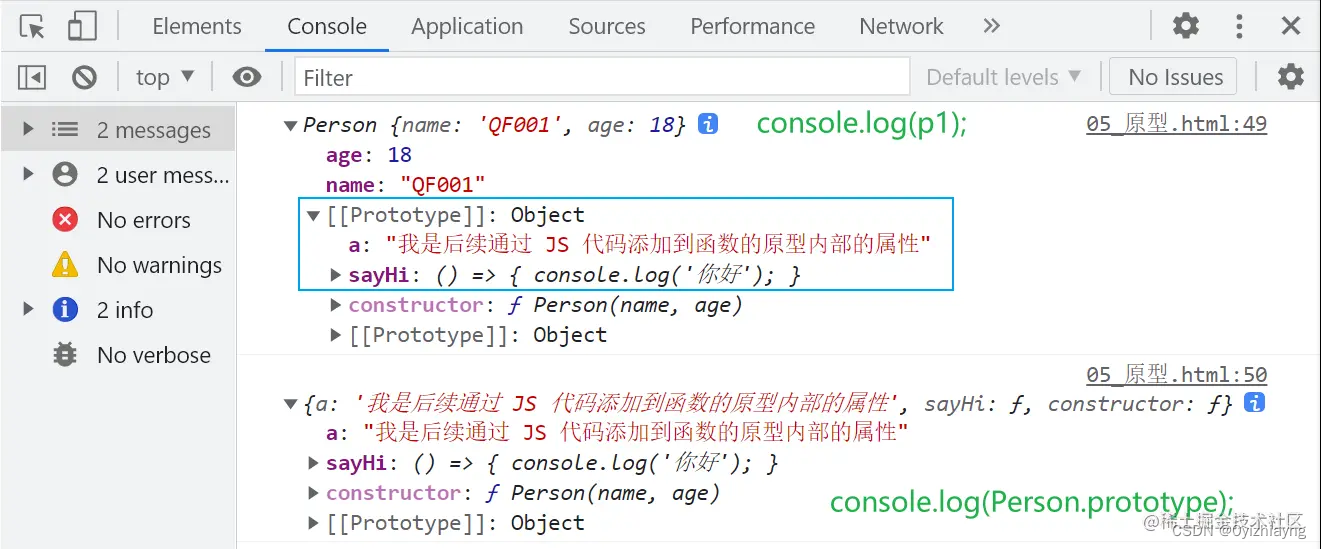
2、 对象访问原型
/**
* 1. 每一个对象都有一个 __proto__(两个下划线), 可以去访问到自己构造函数的原型
* 实例化对象, 本质上也是一个函数, 所以他可以访问到自己构造函数的原型
* 2. 对象访问原型: 对象.__proto__
*
* 3. 对象的访问规则, 现在当前作用域(对象内部)查找, 找到就使用
* 如果没找到, 则会顺着 __proto__ 向上查找
* 未完待续...
*
* 4. 构造函数函数体内, 通常写属性; 构造函数的原型内, 通常写方法(函数)
*
* 5. 构造函数的原型内部添加方法, 并不是为了给构造函数使用, 通常是为了给实例化对象使用
*/
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.a = '通过JS代码添加到函数的原型内部的属性'
Person.prototype.sayHi = () => {
console.log('你好');
}
const p1 = new Person('QF001', 18);
const p2 = new Person('QF002', 28);
p1.sayHi();
console.log(p1.a);
console.log(p1.__proto__);
console.log(p1.__proto__ === Person.prototype);
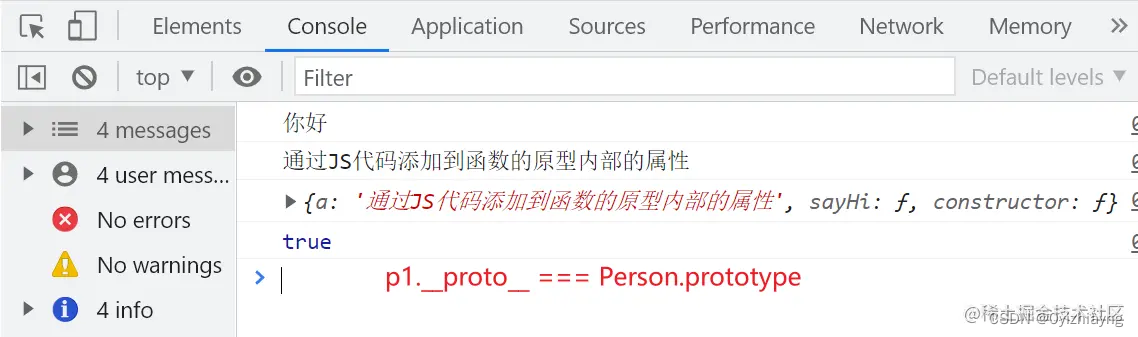
function Person(name, age) {
this.name = name;
this.age = age;
}
Person.prototype.a = '通过JS代码添加到函数的原型内部的属性'
Person.prototype.sayHi = () => {
console.log('你好');
}
const p1 = new Person('QF001', 18);
const p2 = new Person('QF002', 28);
console.log(p1.sayHi);
console.log(p2.sayHi);
console.log(p1.sayHi == p2.sayHi);
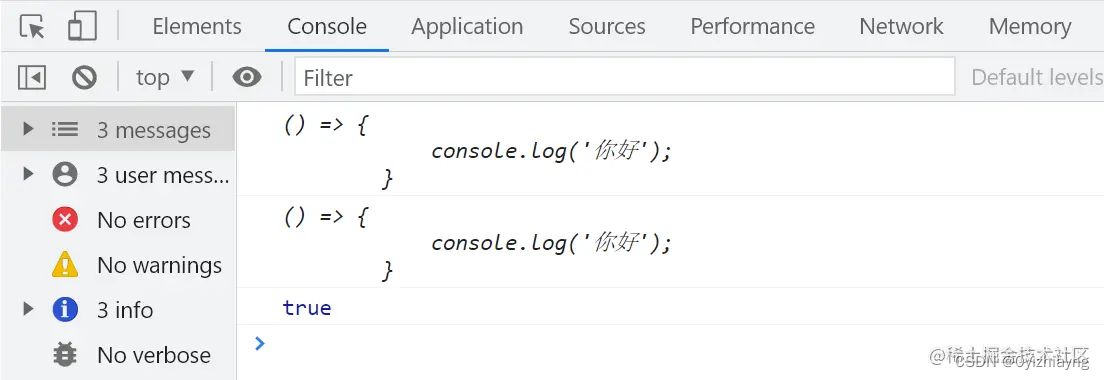
二、我们学的构造函数
1、构造函数
const arr = new Array();
const obj = new Object();
const reg = new RegExp();
const date = new Date();
console.log(arr);
console.log(obj);
console.log(reg);
console.log(date);
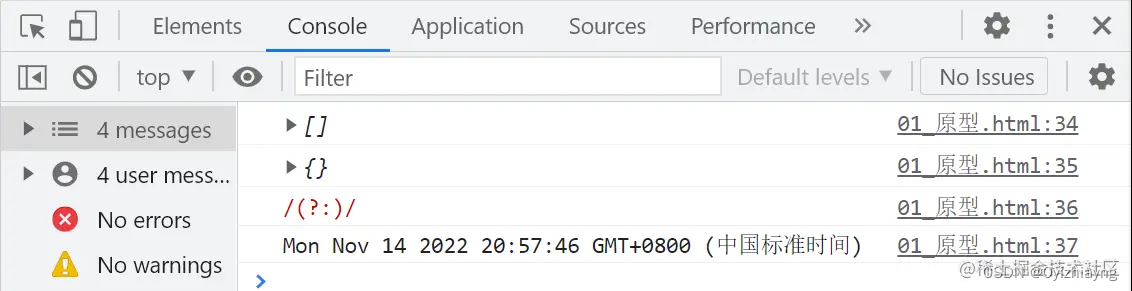
2、查构造函数的原型
const arr = new Array();
console.log(arr.__proto__);
console.log(Array.prototype);
console.log(Object.prototype);
/**
* 任何一个数组的构造函数 都是Array
* 任何一个对象的构造函数 都是Object
* 任何一个函数的构造函数 都是Function
*/
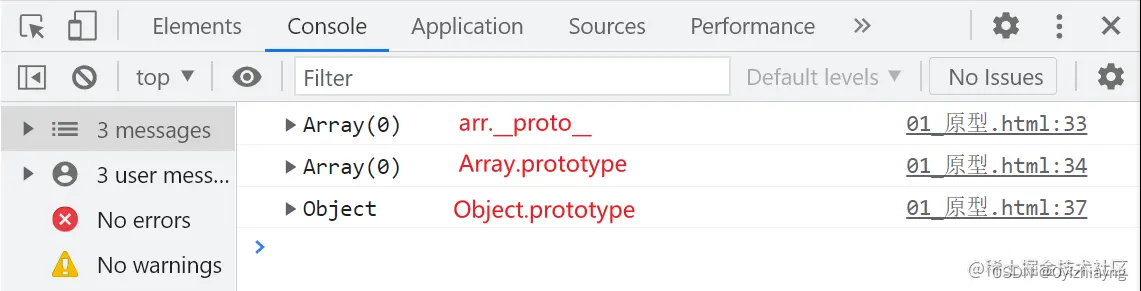
三、课堂案例
1、需求: 给数组扩展一个方法abc, 让他能够打印123
Array.prototype.abc = () => {
console.log(123);
}
const arr = new Array();
const arr1 = new Array();
arr.abc();
arr1.abc();
2、 需求: 给数组扩展一个,求最大值的方法
Array.prototype.getMax = function () {
let max = this[0];
for (let i = 1; i < this.length; i++) {
if (max < this[i]) {
max = this[i];
}
}
return max;
}
const arr1 = new Array(1, 2, 100, 5, 3, 4);
const max1 = arr1.getMax();
console.log(max1);
const arr2 = new Array(1, 2, 100, 5, 3, 4, 999);
const max2 = arr2.getMax();
console.log(max2);