Java实验3 员工管理系统
1.实验要求
- 进行一个企业员工基本信息的数据库设计,使用员工信息表、部门信息表两个数据表来存放数据。选择适当组件进行界面设计;编写事件代码:
员工信息包括:员工编号(主码),姓名,性别,出生年月,所在部门
所在部门信息表包括:部门代码(主码),部门名称
员工信息表可以提供以下功能:
1.员工基本信息的录入
2.员工基本信息的修改
3.员工基本信息的删除
4.员工基本信息的查询
5.员工基本信息的显示
用Java语言编写一个员工基本信息管理系统的图形用户界面,通过界面的按钮和文本框等操作来实现员工基本信息的录入、修改、删除、查询和显示,从而完成一个基本的员工信息管理系统用户界面。 - 在这里我们只编写员工类的信息表,以及具体功能。部门类只编写信息表菜单
2.具体创建过程
- 创建三个包:object包、operation包、staff包。
- object包中有DepartmentObject类和EmployeeObject类和Object类,分别实现部门和员工的信息表。并且DepartmentObject类和EmployeeObject类继承了Object类。
- operation包中AddOperation类、DelOperation类、DisplayOperation类、ExitOperation类、FindOperation类、ReworkOperation类,分别实现信息的录入、删除、显示、退出系统、查找和修改。
并且这些类的本质上都是类似的,所以都接口IOperation。 - staff包中Staff类和StaffList类,分别实现定义姓名、性别等成员变量和定义一个员工数组。
3.结果展示
- 录入信息
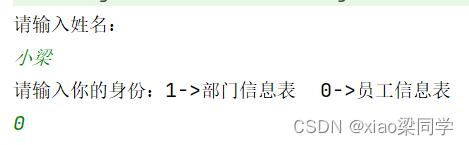
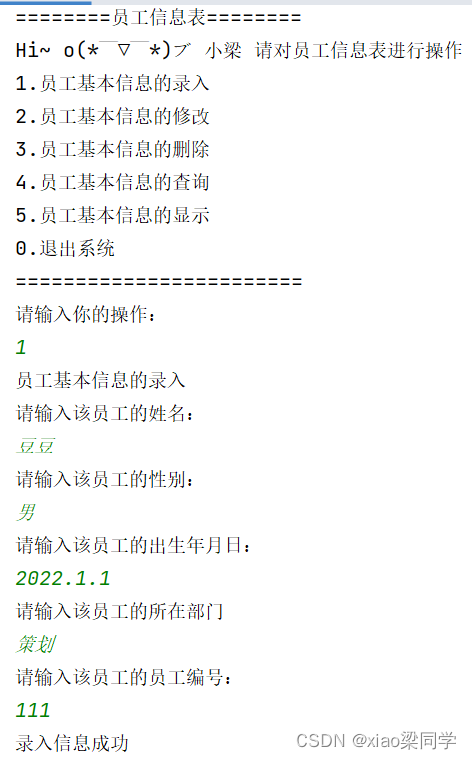
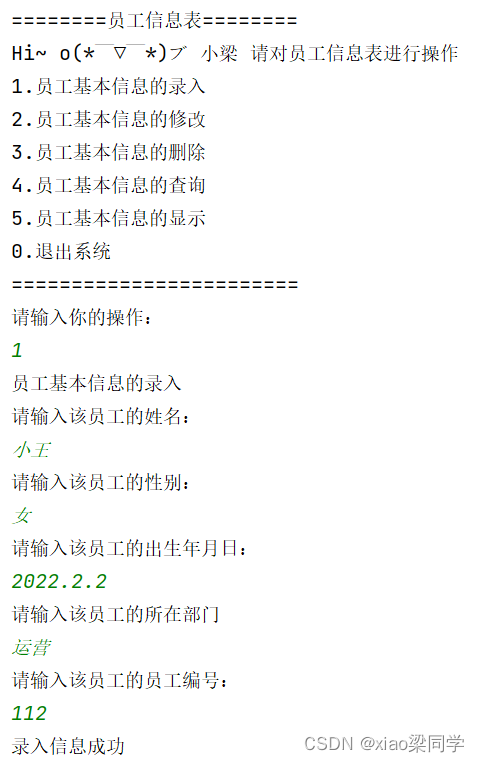
- 显示录入的信息
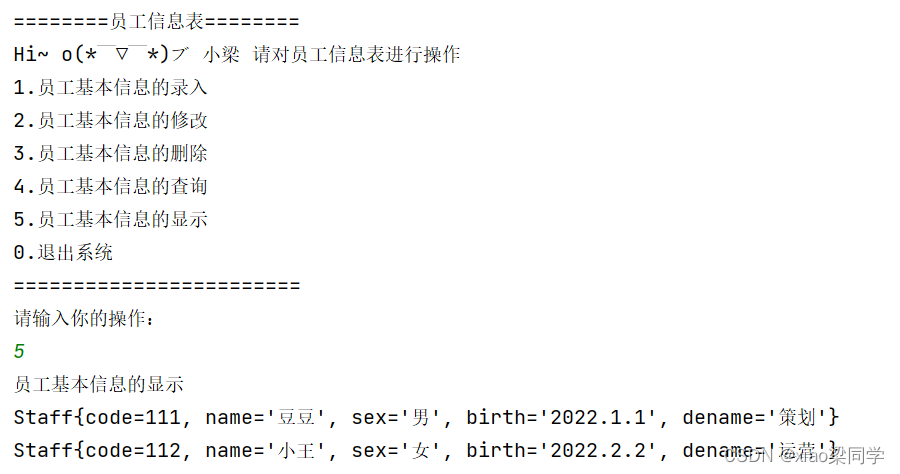
- 修改信息
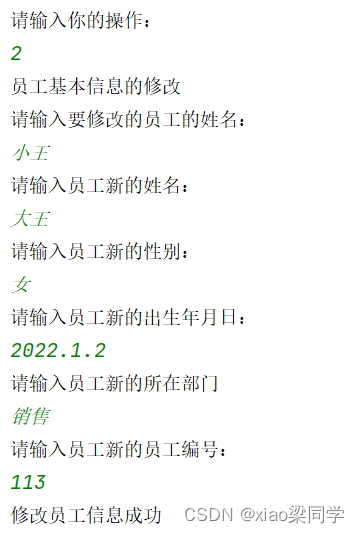
显示修改后的员工信息:
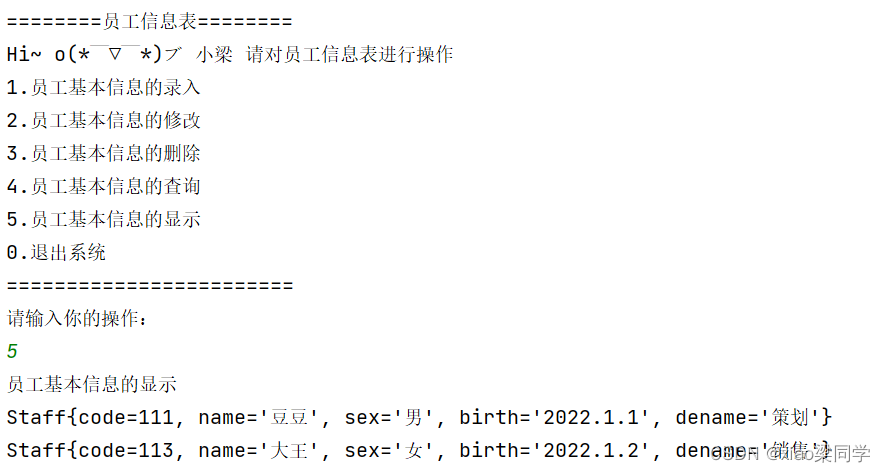
- 查询信息
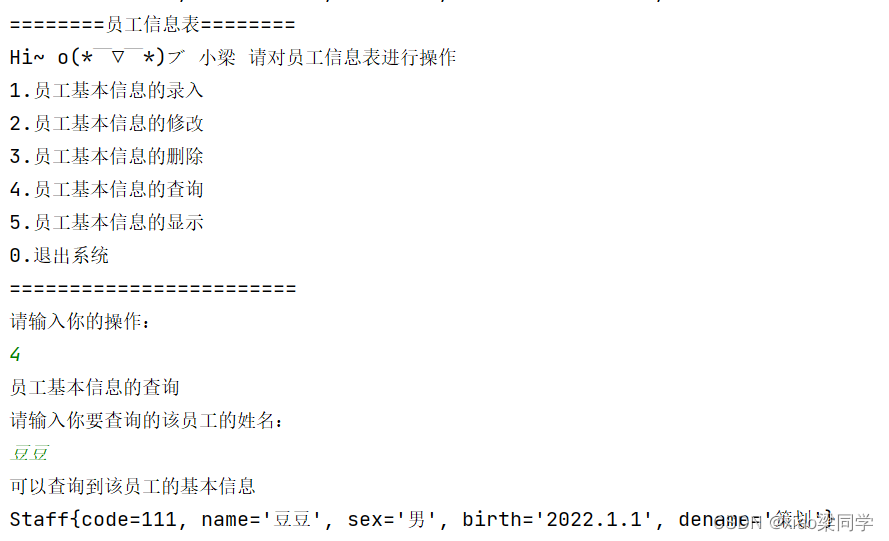
- 删除信息
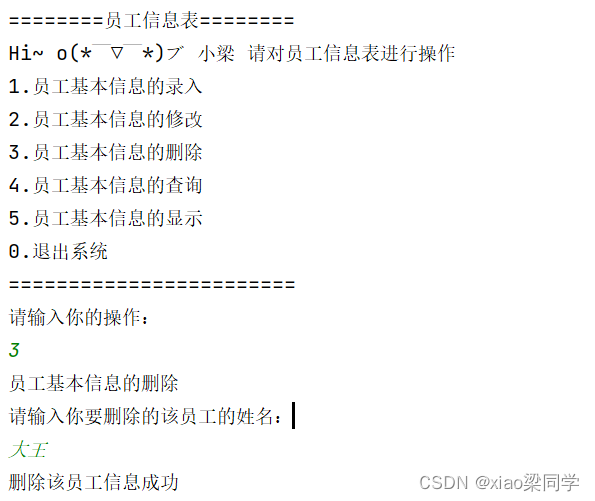
显示删除后的员工信息表:
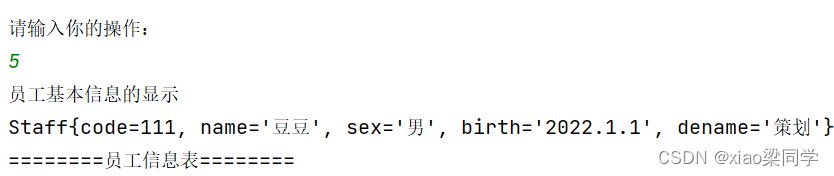
4.代码展示
import object.DepartmentObject;
import object.EmployeeObject;
import object.Object;
import staff.Staff;
import staff.StaffList;
import java.util.Scanner;
public class Main {
public static Object login(){
Scanner scan=new Scanner(System.in);
System.out.println("请输入姓名:");
String name=scan.nextLine();
System.out.println("请输入你的身份:1->部门信息表 0->员工信息表");
int choice =scan.nextInt();
if (choice==1){
return new DepartmentObject(name);
}else {
return new EmployeeObject(name);
}
}
public static void main(String[] args) {
StaffList staffList=new StaffList();
Object object=login();
while (true) {
int choice = object.menu();
object.doOperation(choice, staffList);
}
}
}
package staff;
public class Staff {
private int code;
private String name;
private String sex;
private String birth;
private String dename;
public Staff(int code, String name, String sex, String birth, String dename) {
this.code = code;
this.name = name;
this.sex = sex;
this.birth = birth;
this.dename = dename;
}
public int getCode() {
return code;
}
public void setCode(int code) {
this.code = code;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getBirth() {
return birth;
}
public void setBirth(String birth) {
this.birth = birth;
}
public String getDename() {
return dename;
}
public void setDename(String dename) {
this.dename = dename;
}
@Override
public String toString() {
return "Staff{" +
"code=" + code +
", name='" + name + '\'' +
", sex='" + sex +'\''+
", birth='" + birth +'\''+
", dename='" + dename + '\'' +
'}';
}
}
package staff;
public class StaffList {
public Staff[] staffList=new Staff[10];
private int Size;
public int getSize() {
return Size;
}
public void setSize(int size) {
this.Size = size;
}
public void setStaffList(int pos,Staff staff){
staffList[pos]=staff;
}
public Staff getStaff(int pos){
return staffList[pos];
}
}
package object;
import operation.*;
import java.util.Scanner;
public class DepartmentObject extends Object{
public DepartmentObject(String name) {
super(name);
this.iOperations=new IOperation[]{
new ExitOperation(),
new AddOperation(),
new ReworkOperation(),
new DelOperation(),
new FindOperation(),
new DisplayOperation()
};
}
public int menu(){
System.out.println("========部门信息表========");
System.out.println("Hi~ o(* ̄▽ ̄*)ブ "+this.name+" 请对部门信息表进行操作");
System.out.println("1.部门基本信息的录入");
System.out.println("2.部门基本信息的修改");
System.out.println("3.部门基本信息的删除");
System.out.println("4.部门基本信息的查询");
System.out.println("5.部门基本信息的显示");
System.out.println("0.退出系统");
System.out.println("=======================");
System.out.println("请输入你的操作:");
Scanner scanner=new Scanner(System.in);
int choice =scanner.nextInt();
return choice;
}
}
package object;
import operation.*;
import java.util.Scanner;
public class EmployeeObject extends Object{
public EmployeeObject(String name) {
super(name);
this.iOperations=new IOperation[]{
new ExitOperation(),
new AddOperation(),
new ReworkOperation(),
new DelOperation(),
new FindOperation(),
new DisplayOperation()
};
}
public int menu(){
System.out.println("========员工信息表========");
System.out.println("Hi~ o(* ̄▽ ̄*)ブ "+this.name+" 请对员工信息表进行操作");
System.out.println("1.员工基本信息的录入");
System.out.println("2.员工基本信息的修改");
System.out.println("3.员工基本信息的删除");
System.out.println("4.员工基本信息的查询");
System.out.println("5.员工基本信息的显示");
System.out.println("0.退出系统");
System.out.println("========================");
System.out.println("请输入你的操作:");
Scanner scanner=new Scanner(System.in);
int choice =scanner.nextInt();
return choice;
}
}
package object;
import operation.IOperation;
import staff.Staff;
import staff.StaffList;
public abstract class Object {
protected String name;
protected IOperation[] iOperations;
public Object(String name){
this.name=name;
}
public abstract int menu();
public void doOperation(int choice, StaffList staffList){
this.iOperations[choice].work(staffList);
}
}
package operation;
import staff.Staff;
import staff.StaffList;
public interface IOperation {
void work(StaffList staffList);
}
package operation;
import staff.Staff;
import staff.StaffList;
import java.util.Scanner;
public class AddOperation implements IOperation{
@Override
public void work(StaffList staffList) {
System.out.println("员工基本信息的录入");
Scanner scanner=new Scanner(System.in);
System.out.println("请输入该员工的姓名:");
String name=scanner.nextLine();
System.out.println("请输入该员工的性别:");
String sex=scanner.nextLine();
System.out.println("请输入该员工的出生年月日:");
String birth=scanner.nextLine();
System.out.println("请输入该员工的所在部门");
String dename=scanner.nextLine();
System.out.println("请输入该员工的员工编号:");
int code=scanner.nextInt();
Staff staff=new Staff(code,name,sex,birth,dename);
int currentSize=staffList.getSize();
staffList.setStaffList(currentSize,staff);
staffList.setSize(currentSize+1);
System.out.println("录入信息成功");
}
}
package operation;
import staff.Staff;
import staff.StaffList;
import java.util.Scanner;
public class DelOperation implements IOperation{
@Override
public void work(StaffList staffList) {
System.out.println("员工基本信息的删除");
Scanner scanner=new Scanner(System.in);
System.out.println("请输入你要删除的该员工的姓名:");
String name=scanner.nextLine();
int currentSize=staffList.getSize();
int index=-1;
for(int i=0;i<currentSize;i++){
Staff staff=staffList.getStaff(i);
if(staff.getName().equals(name)){
index=i;
break;
}
}
if(index==-1){
System.out.println("没有你要删除的员工信息");
return;
}
for(int i=index;i<currentSize-1;i++){
Staff staff=staffList.getStaff(i+1);
staffList.setStaffList(i,staff);
}
staffList.setSize(currentSize-1);
System.out.println("删除该员工信息成功");
}
}
package operation;
import staff.StaffList;
import staff.Staff;
import staff.StaffList;
public class DisplayOperation implements IOperation{
@Override
public void work(StaffList staffList) {
System.out.println("员工基本信息的显示");
int currentSize=staffList.getSize();
for(int i=0;i<currentSize;i++){
Staff staff=staffList.getStaff(i);
System.out.println(staff);
}
}
}
package operation;
import staff.Staff;
import staff.StaffList;
public class ExitOperation implements IOperation{
@Override
public void work(StaffList staffList) {
System.out.println("退出系统");
System.exit(0);
}
}
package operation;
import com.sun.glass.ui.Size;
import staff.Staff;
import staff.StaffList;
import java.util.Scanner;
public class FindOperation implements IOperation{
@Override
public void work(StaffList staffList) {
System.out.println("员工基本信息的查询");
Scanner scanner=new Scanner(System.in);
System.out.println("请输入你要查询的该员工的姓名:");
String name=scanner.nextLine();
int size=staffList.getSize();
for(int i = 0; i<size; i++){
Staff staff=staffList.getStaff(i);
if(staff.getName().equals(name)){
System.out.println("可以查询到该员工的基本信息");
System.out.println(staff);
return;
}
}
System.out.println("信息表中没有该员工的信息");
}
}
package operation;
import staff.Staff;
import staff.StaffList;
import java.util.Scanner;
public class ReworkOperation implements IOperation{
@Override
public void work(StaffList staffList) {
System.out.println("员工基本信息的修改");
Scanner scanner=new Scanner(System.in);
System.out.println("请输入要修改的员工的姓名:");
String name1=scanner.nextLine();
int currentSize=staffList.getSize();
int index=-1;
for(int i=0;i<currentSize;i++){
Staff staff=staffList.getStaff(i);
if(staff.getName().equals(name1)){
index=i;
break;
}
}
if(index==-1){
System.out.println("没有你要删除的员工信息");
}
else {
System.out.println("请输入员工新的姓名:");
String name=scanner.nextLine();
System.out.println("请输入员工新的性别:");
String sex=scanner.nextLine();
System.out.println("请输入员工新的出生年月日:");
String birth=scanner.nextLine();
System.out.println("请输入员工新的所在部门");
String dename=scanner.nextLine();
System.out.println("请输入员工新的员工编号:");
int code=scanner.nextInt();
Staff s=new Staff(code,name,sex,birth,dename);
s.setName(name);
s.setBirth(birth);
s.setDename(dename);
s.setSex(sex);
s.setCode(code);
staffList.setStaffList(index,s);
System.out.println("修改员工信息成功");
}
}
}