为了大家可以更清楚地看到题目,我特意去找了当时的比赛文档,希望大家可以有更好的体验。
试题A:组队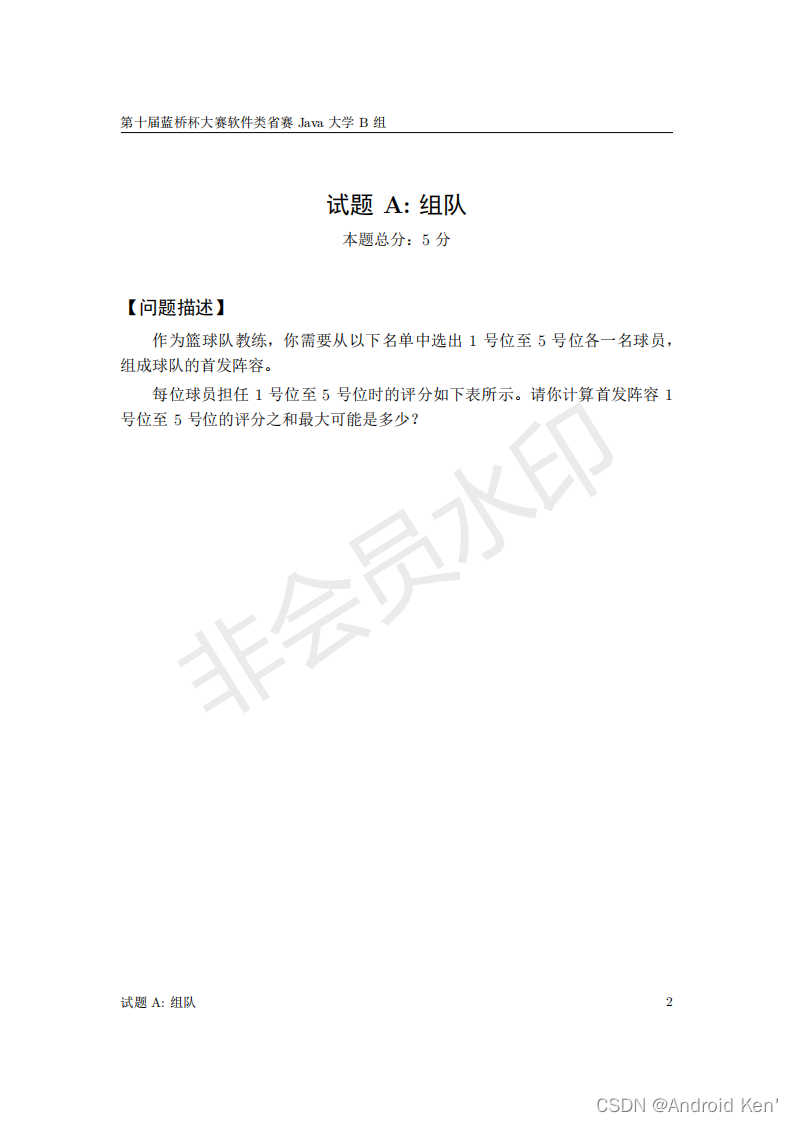
组队这一题是签到题目,很简单这里不在赘述。
答案:490(填空题,直接写答案即可)
试题B:不同字串
代码如下:
import java.util.*;
/*
第十届第二题:不同字串
*/
public class 不同字串{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
String s = sc.nextLine();
//0100110001010001
Set set = new HashSet();
int n = 1;
while (n <= s.length()){
for (int i = n;i <= s.length();i++){
String s1 = s.substring(i-n, i);
set.add(s1);
}
n++;
}
System.out.println(set.size());
}
}
(这里采用的是循环嵌套的方式,还有Hashset用来去重,相比来说比较简单一点)
答案: 100(填空题,直接写答案即可)
试题C:数列求值
代码如下:
public class 数列求值{
//第十届第三题:数列求值
public static void main(String[] args) {
long a = 1;
long b = 1;
long c = 1;
long result = 0;
for (int i = 4;i <=20190324;i++){
result = (a+b+c)%10000;
a = b;
b = c;
c = result;
}
System.out.println(result);
}
}
值得注意的是,这里的数字会特别大,即使是long类型也会越界,因为我们只需要最后四位数字,所以采用将每次相加的结果进行%10000即可,也就是保留最后我们所需要的四位数字
答案:4659(填空题,直接写答案即可)
试题D:数的分解
代码如下:
public class 数的分解{
//第十届第四题:数的分解
public static void main(String[] args) {
int count = 0;
for (int i = 1; i <= 2019; i++) {
String s1 = i + "";
if (s1.contains("2") || s1.contains("4")) continue;
for (int j = i + 1; j <= 2019; j++) {
String s2 = j + "";
if (s2.contains("2") || s2.contains("4")) continue;
for (int k = j + 1; k <= 2019; k++) {
String s3 = k + "";
if (s3.contains("2") || s3.contains("4")) continue;
if (i + j + k == 2019) {
count++;
}
}
}
}
System.out.println(count);
}
}
答案:40785 (填空题,直接写答案即可)
试题E:迷宫
代码如下:
import java.util.LinkedList;
import java.util.Queue;
//第十届第五题:迷宫
public class 迷宫{
public static void main(String[] args){
String s = "01010101001011001001010110010110100100001000101010"
+ "00001000100000101010010000100000001001100110100101"
+ "01111011010010001000001101001011100011000000010000"
+ "01000000001010100011010000101000001010101011001011"
+ "00011111000000101000010010100010100000101100000000"
+ "11001000110101000010101100011010011010101011110111"
+ "00011011010101001001001010000001000101001110000000"
+ "10100000101000100110101010111110011000010000111010"
+ "00111000001010100001100010000001000101001100001001"
+ "11000110100001110010001001010101010101010001101000"
+ "00010000100100000101001010101110100010101010000101"
+ "11100100101001001000010000010101010100100100010100"
+ "00000010000000101011001111010001100000101010100011"
+ "10101010011100001000011000010110011110110100001000"
+ "10101010100001101010100101000010100000111011101001"
+ "10000000101100010000101100101101001011100000000100"
+ "10101001000000010100100001000100000100011110101001"
+ "00101001010101101001010100011010101101110000110101"
+ "11001010000100001100000010100101000001000111000010"
+ "00001000110000110101101000000100101001001000011101"
+ "10100101000101000000001110110010110101101010100001"
+ "00101000010000110101010000100010001001000100010101"
+ "10100001000110010001000010101001010101011111010010"
+ "00000100101000000110010100101001000001000000000010"
+ "11010000001001110111001001000011101001011011101000"
+ "00000110100010001000100000001000011101000000110011"
+ "10101000101000100010001111100010101001010000001000"
+ "10000010100101001010110000000100101010001011101000"
+ "00111100001000010000000110111000000001000000001011"
+ "10000001100111010111010001000110111010101101111000";
int c = 50;
int r = 30;
char[][] map = new char[r][c];
for (int i = 0, k = 0; i < r; i++, k += 50) {
map[i] = s.substring(k, k + 50).toCharArray();
}
BFS(map, r, c);
}
public static void BFS(char[][] map, int r, int c) {
int[] x = { 1, 0, 0, -1 };
int[] y = { 0, -1, 1, 0 };
char dirName[] = { 'D', 'L', 'R', 'U' };
boolean[][] vis = new boolean[r][c];
Queue<Node> queue = new LinkedList<Node>();
Node node = new Node(0, 0, "");
vis[0][0] = true;
queue.offer(node);
while (!queue.isEmpty()) {
Node curNode = queue.poll();
if (curNode.x == r - 1 && curNode.y == c - 1) {
System.out.println(curNode.path);
System.out.println(curNode.path.length());
break;
}
for (int i = 0; i < 4; i++) {
int nextX = curNode.x + x[i];
int nextY = curNode.y + y[i];
if (nextX >= 0 && nextX < r && nextY >= 0 && nextY < c && vis[nextX][nextY] == false) {
if (map[nextX][nextY] == '0') {
queue.offer(new Node(nextX, nextY, curNode.path + dirName[i]));
vis[nextX][nextY] = true;
}
}
}
}
}
}
//节点类
class Node {
int x;
int y;
String path;
public Node(int x, int y, String path) {
super();
this.x = x;
this.y = y;
this.path = path;
}
}
这是典型的BFS例题,起点(0,0)就是这棵遍历树的根节点,四个方向就是每个节点的4个子节点,走到叶子结点只有两种情况:①堵路(拐回到走过的路或者四周没有通路),返回上一层;②走到终点。此时是按照步数最少和字典序优先得到的最优解。
答案:186
DDDDRRURRRRRRDRRRRDDDLDDRDDDDDDDDDDDDRDDRRRURRUURRDDDDRDRRRRRRDRRURRDDDRRRRUURUUUUUUULULLUUUURRRRUULLLUUUULLUUULUURRURRURURRRDDRRRRRDDRRDDLLLDDRRDDRDDLDDDLLDDLLLDLDDDLDDRRRRRRRRRDDDDDDRR
试题F:特别数的和
代码如下:
import java.util.Scanner;
public class 特别的数{
//第十届第六题:特别的数
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int n = sc.nextInt();
long count = 0;
for (int i = 1;i <= n;i++){
String s = i + "";
if (s.contains("2") || s.contains("0") || s.contains("1") || s.contains("9")){
count = count + i;
}
}
System.out.println(count);
}
}
本题目为编程题目,一定要注意数据的类型,防止精度出现问题,这道题目是签到题目,很简单,不再多说。
试题G:外卖店优先级
代码如下:
import java.util.Scanner;
public class 外卖店优先级{
//第十届第七题:外卖店优先级
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int N = scanner.nextInt(), M = scanner.nextInt(), T = scanner.nextInt();
// M份订单
int[][] orders = new int[M][2];
for(int i = 0; i < M; i++) {
orders[i][0] = scanner.nextInt();
orders[i][1] = scanner.nextInt();
}
// N家评分 优先级
int[] grades = new int[N];
int[] ranks = new int[N];
for(int i = 1; i <= T; i++){
// 标记此时刻是否有订单
int[] sign = new int[N];
for(int j = 0; j < M; j++){
if(orders[j][0] == i){
grades[orders[j][1]-1] += 2;
sign[orders[j][1]-1] = 1;
// 进入优先缓存
if(grades[orders[j][1]-1] > 5) ranks[orders[j][1]-1] = 1;
}
}
// 此时可没有订单的降一级
for(int k = 0; k < N; k++){
if(sign[k] != 1) grades[k]--;
// 清除优先缓存
if(grades[k] < 3) ranks[k] = 0;
}
}
// 计算优先缓存的店家
int count = 0;
for(int i = 0; i < N; i++) {
if(ranks[i] == 1) count++;
}
System.out.println(count);
scanner.close();
}
}
我个人认为本题主要是找到合适的存储数据的载体(即数组),有一些人可能会想用Hashmap或者Hashset进行存储,但是由于数据或者数据对会存在重复的问题,故不可以使用,这里最好还是使用最基本的数组进行操作,一步一步处理即可。
试题H:任务相关性分析
代码如下:
import java.util.*;
public class 人物相关性分析{
//第十届第八题:人物相关性分析
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int K = scanner.nextInt();
// 要接受一个回车
scanner.nextLine();
String str = scanner.nextLine();
// 正则表达式匹配" "或者".", ". "分割后是空字符串
String[] substr = str.split("\\s+|\\.");
// 获得长度
int[] length = new int[substr.length];
for(int i = 0; i < substr.length; i++)
length[i] = substr[i].length();
int count = 0;
int index1 = -1, index2 = -1;
// 匹配Alice->Bob
for(int i = 0; i < substr.length; i++){
if(substr[i].equals("Alice")){
index1 = i;
}
if(substr[i].equals("Bob") && index1 != -1){
index2 = i;
int tempLength = 1;
for(int j = index1 + 1; j < index2; j++)
tempLength += length[j] + 1;
if(tempLength <= K) count++;
index1 = index2 = -1;
}
}
index1 = index2 = -1;
// 匹配Bob->Alice
for(int i = 0; i < substr.length; i++){
if(substr[i].equals("Bob")){
index1 = i;
}
if(substr[i].equals("Alice") && index1 != -1){
index2 = i;
int tempLength = 1;
for(int j = index1 + 1; j < index2; j++)
tempLength += length[j] + 1;
if(tempLength <= K) count++;
index1 = index2 = -1;
}
}
System.out.println(count);
scanner.close();
}
}
试题I:后缀表达式
代码如下:
import java.util.Arrays;
import java.util.Scanner;
public class 后缀表达式{
//第十届第九题:后缀表达式
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int N = scanner.nextInt(), M = scanner.nextInt();
int[] numbers = new int[N+M+1];
int minus = 0;
for(int i = 0; i < N + M + 1; i++){
numbers[i] = scanner.nextInt();
// 负数个数
if(numbers[i] < 0) minus++;
}
// 从小到大排序
Arrays.sort(numbers);
int sum = 0, n = M + N;
// 只有加号
if(M == 0){
for(int i = 0; i <= n; i++)
sum += numbers[i];
}
// 只有减号
else if(N == 0){
// 全部是正数
if(numbers[0] >= 0){
sum = - numbers[0];
for(int i = 1; i <= n; i++)
sum += numbers[i];
}
// 全部是负数
else if(minus == n){
sum = numbers[n];
for(int i = 0; i < n; i++)
sum += numbers[i];
}
// 有正数有负数
else{
for(int i = 0; i <= n; i++)
sum += Math.abs(numbers[i]);
}
}
// 有加号有减号
else{
// 全部是正数
if(numbers[0] >= 0){
sum = - numbers[0];
for(int i = 1; i <= n; i++)
sum += numbers[i];
}
// 全部是负数 或 有正数有负数 M < minus
else if(minus == n || M < minus){
for(int i = 0; i <= n; i++){
if(i <= M+1) sum += Math.abs(numbers[i]);
else sum += numbers[i];
}
}
// M > minus
else{
for (int i = 0; i <= n; i++)
sum += Math.abs(numbers[i]);
}
}
System.out.println(sum);
scanner.close();
}
}
试题J:灵能传输
最后一题我就放个题目,懂得都懂。。。。。。。。
最后希望各位大佬能够给予一些意见,算法小新希望得到各位的指导,我觉得相比算法我还是更喜欢开发相关的,评论区留下你们的想法哦。