convert: true, //自动偏移坐标,偏移后的坐标为高德坐标,默认:true
showButton: false, //显示定位按钮,默认:true
showMarker: false, //定位成功后在定位到的位置显示点标记,默认:true
showCircle: false, //定位成功后用圆圈表示定位精度范围,默认:true
panToLocation: false, //定位成功后将定位到的位置作为地图中心点,默认:true
zoomToAccuracy:false //定位成功后调整地图视野范围使定位位置及精度范围视野内可见,默认:false
});
if(positionTimer) {
clearInterval(positionTimer)
}
positionTimer = setInterval(()=>{
geolocation.getCurrentPosition((status,result) =>{
if(status === 'complete') {
this.myLocation.longitude = result.position.lng
this.myLocation.latitude = result.position.lat
if(this.selfMarker) {
this.selfMarker.setPosition([this.myLocation.longitude, this.myLocation.latitude])
}
}
});
},1000)
});
}
}
// 获取当前城市adcode行政编码
this.cityInfo = await new Promise((resolve,reject)=>{
this.AMap.plugin('AMap.Geocoder', () => {
new this.AMap.Geocoder({ city: '',radius: 1000 }).getAddress([this.fromAppInfo.fromLongitude, this.fromAppInfo.fromLatitude],
(status, result) => {
if (status === 'complete' && result.regeocode) {
let address = result.regeocode.addressComponent;
address.adcode = adcodeDeal.dealAdCode(address.adcode)
// 直辖市去除
resolve(address)
}else{
resolve({adcode: null})
}
});
})
})
5. 绘制路线
// 规划绘制路线
drawRoute(planingRoutes) {
// 解析线路
const parseRouteToPath = (route) => {
let path = []
let continuityPath = []
for (let i = 0, l = route.steps.length; i < l; i++) {
let step = route.steps[i]
const arrayOfCoordinates = step.polyline.split(“;”).map(coord => coord.split(“,”));
path.push(arrayOfCoordinates.flat())
}
path = path.flat()
for(let i=0;i < path.length -1;i++) {
continuityPath.push([Number(path[i]),Number(path[i+1])])
i++
}
return continuityPath
}
planingRoutes.forEach(itemRoute=>{
this.routeLines.push(parseRouteToPath(itemRoute))
})
// 开始图标
this.startMarker = new this.AMap.Marker({
position: [this.routeLines[0][0][0],this.routeLines[0][0][1]],
// icon: 'https://webapi.amap.com/theme/v1.3/markers/n/start.png',
map: this.mapInstance,
content: this.$refs.startMarkerRef,
// offset: new this.AMap.Pixel(-80, -40),
anchor: new this.AMap.Pixel(0,0),
offset: new this.AMap.Pixel(-80,-50),
size: new this.AMap.Size(100, 100)
})
// 结束图标
this.endMarker = new this.AMap.Marker({
position: [this.routeLines[0][this.routeLines[0].length-1][0],this.routeLines[0][this.routeLines[0].length-1][1]],
// icon: 'https://webapi.amap.com/theme/v1.3/markers/n/end.png',
map: this.mapInstance,
content: this.$refs.endMarkerRef,
// offset: new this.AMap.Pixel(-80, -40),
anchor: new this.AMap.Pixel(0,0),
offset: new this.AMap.Pixel(-80,-50),
size: new this.AMap.Size(100, 100)
})
// 画线
this.routeLinesInstance = []
this.routeLines.forEach((path,index)=>{
let polyline = new this.AMap.Polyline({
path: path,
isOutline: true,
outlineColor: '#366d33',
borderWeight: index === 0 ? 2 : 1,
strokeWeight: index === 0 ? 5: 4,
showDir: true,
strokeOpacity: index === 0 ? 0.9 : 0.3,
strokeColor: index === 0 ? '#45ed45' : '#30aa30',
lineJoin: 'round',
extData: {
isSelect: index === 0,
index: index
}
})
polyline.hide()
this.routeLinesInstance.push(polyline)
this.mapInstance.add(polyline);
})
// 注册事件 实现点击切换线路
this.routeLinesInstance.forEach((polyline,index)=>{
polyline.on('click', (e) => {
polyline.setOptions({
borderWeight: 2,
strokeWeight: 5,
strokeOpacity: 0.9,
strokeColor: '#45ed45',
extData: {
isSelect: true,
index: index
}
})
let otherPath= this.routeLinesInstance.filter((item,ind) =>ind !== index)
otherPath.forEach(item=>{
item.setOptions({
borderWeight: 1,
strokeWeight: 4,
strokeOpacity: 0.3,
strokeColor: '#30aa30',
extData: {
isSelect: false,
index: item.getExtData().index,
}
})
})
// 变更路线
this.$eventBus.$emit('changeRouteLine')
// 调整视野达到最佳显示区域
this.mapInstance.setFitView([ this.startMarker, this.endMarker, ...this.routeLinesInstance ], false, [70,260,60,60])
})
})
this.routeLinesInstance[0].show()
this.startMarker.show()
this.endMarker.show()
},
### 3. uniapp端开发
1. 在manifest.json将这些定位权限开启,还要将key填写到App模块配置里的高德key里
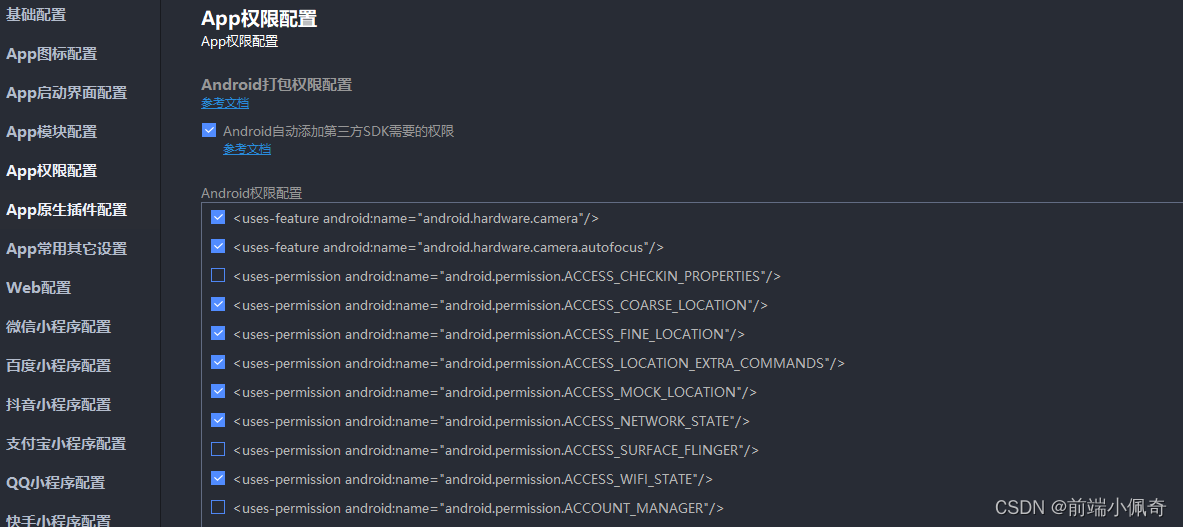
2. 在nvue中创建webview(nvue对webview层级问题和双向通信友好)
// webHeight = uni.getSystemInfoSync().windowHeight
### 3. uniapp与webview双向通信(微信小程序与app略有不同)
#### App中:app与webview双向通信
// app 向 web发送消息
-
通过src路径 拼接params参数传递
// app上发送
this.src = href + ‘?pageInfo=’ + encodeURIComponent(JSON.stringify(params))
// web上接收
const params = JSON.parse(this.$route.query.pageInfo) -
通过evalJs注册函数在web的windows上调用
// app向 web发送消息 (注意 需要将web在页面加载好了再调用,以防止报错,或加个延时再调用)
const params = JSON.stringify({id: ‘1234’,token: ‘xxxxxxx’})
this. r e f s . w e b v i e w R e f . e v a l J s ( ‘ s e n d M s g F r o m A p p ( refs.webviewRef.evalJs(`sendMsgFromApp( refs.webviewRef.evalJs(‘sendMsgFromApp({params})`)
// web上接收
window.sendMsgFromApp(params) {
console.log(params)
}
// web 向 app发消息
- 在web的index.html上引入这个uni插件(注意版本)
- 这个方法判断下插件是否注册挂载完成
document.addEventListener(‘UniAppJSBridgeReady’, () => {
// 挂载好了,可以使用插件中的方法了 - 向app发消息
uni.postMessage({ data: { message: ‘这是一段消息’ } })
// uni.navigateTo({url:‘xxx’}) // 直接跟uni方法一样
}) - app中webview上注册的方法 @onPostMessage=“getMessageFromWeb” 进行实时接收
#### 微信小程序中:微信小程序与webview双向通信
// 微信小程序 向 web发送消息
- 通过src路径 拼接params参数传递 ,这个方法每次调用都会让页面刷新(注意频率)
// 微信小程序 上发送
this.src = href + ‘?pageInfo=’ + encodeURIComponent(JSON.stringify(params))
// web上接收
const params = JSON.parse(this.$route.query.pageInfo)
-----> 注意不能使用evalJs调用…
// web 向 微信小程序发消息(由于微信小程序没法实时通过webview上onPostMessage方法调用,只能当页面摧毁或隐藏的时候才能调用,所以通过互转路由来获取web传递参数最好)
- 在web的index.html上引入这个微信的插件(注意版本)
- 这个方法判断下插件是否注册挂载完成
document.addEventListener(‘UniAppJSBridgeReady’, () => {
// 挂载好了,可以使用插件中的方法了 - 只能通过跳转url传参
uni.navigateTo({
自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。
深知大多数网络安全工程师,想要提升技能,往往是自己摸索成长,但自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!
因此收集整理了一份《2024年网络安全全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友。
既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上网络安全知识点,真正体系化!
由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新
如果你觉得这些内容对你有帮助,可以添加VX:vip204888 (备注网络安全获取)
本人从事网路安全工作12年,曾在2个大厂工作过,安全服务、售后服务、售前、攻防比赛、安全讲师、销售经理等职位都做过,对这个行业了解比较全面。
最近遍览了各种网络安全类的文章,内容参差不齐,其中不伐有大佬倾力教学,也有各种不良机构浑水摸鱼,在收到几条私信,发现大家对一套完整的系统的网络安全从学习路线到学习资料,甚至是工具有着不小的需求。
最后,我将这部分内容融会贯通成了一套282G的网络安全资料包,所有类目条理清晰,知识点层层递进,需要的小伙伴可以点击下方小卡片领取哦!下面就开始进入正题,如何从一个萌新一步一步进入网络安全行业。
学习路线图
其中最为瞩目也是最为基础的就是网络安全学习路线图,这里我给大家分享一份打磨了3个月,已经更新到4.0版本的网络安全学习路线图。
相比起繁琐的文字,还是生动的视频教程更加适合零基础的同学们学习,这里也是整理了一份与上述学习路线一一对应的网络安全视频教程。
网络安全工具箱
当然,当你入门之后,仅仅是视频教程已经不能满足你的需求了,你肯定需要学习各种工具的使用以及大量的实战项目,这里也分享一份我自己整理的网络安全入门工具以及使用教程和实战。
项目实战
最后就是项目实战,这里带来的是SRC资料&HW资料,毕竟实战是检验真理的唯一标准嘛~
面试题
归根结底,我们的最终目的都是为了就业,所以这份结合了多位朋友的亲身经验打磨的面试题合集你绝对不能错过!
一个人可以走的很快,但一群人才能走的更远。不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎扫码加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
*,毕竟实战是检验真理的唯一标准嘛~
面试题
归根结底,我们的最终目的都是为了就业,所以这份结合了多位朋友的亲身经验打磨的面试题合集你绝对不能错过!
一个人可以走的很快,但一群人才能走的更远。不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎扫码加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
[外链图片转存中…(img-7HKDpeut-1712926071801)]