Collectors.counting 中内部做的调用reducing()进行数据汇总
public static <T> Collector<T, ?, Long> counting() {
return reducing(0L, e -> 1L, Long::sum);
}
public class CollectorsDemo {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 23));
studentList.add(new Student("李四", 20));
studentList.add(new Student("王五", 19));
studentList.add(new Student("小明", 21));
studentList.add(new Student("小红", 24));
//获取年龄最大的学生
Optional<Student> max = studentList.stream().collect(Collectors.maxBy(Comparator.comparing(Student::getAge)));
System.out.println(max);
//获取年龄最小的学生
Optional<Student> min = studentList.stream().collect(Collectors.minBy(Comparator.comparing(Student::getAge)));
System.out.println(min);
}
}
**
public class CollectorsDemo {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 23));
studentList.add(new Student("李四", 20));
studentList.add(new Student("王五", 19));
studentList.add(new Student("小明", 21));
studentList.add(new Student("小红", 24));
//法一:
Double collect = studentList.stream().collect(Collectors.averagingInt(Student::getAge));
System.out.println(collect);
//法二:提取年龄然后求平均值
OptionalDouble average = studentList.stream().mapToDouble(Student::getAge).average();
System.out.println(average);
}
}
**
public class CollectorsDemo {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 23));
studentList.add(new Student("李四", 20));
studentList.add(new Student("王五", 19));
studentList.add(new Student("小明", 21));
studentList.add(new Student("小红", 24));
IntSummaryStatistics collect = studentList.stream().collect(Collectors.summarizingInt(Student::getAge));
//总数
System.out.println(collect.getCount());
//求和
System.out.println(collect.getSum());
//最小数
System.out.println(collect.getMin());
//平均数
System.out.println(collect.getAverage());
//最大数
System.out.println(collect.getMax());
}
}
**
public class CollectorsDemo {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 23));
studentList.add(new Student("李四", 20));
studentList.add(new Student("王五", 19));
studentList.add(new Student("小明", 21));
studentList.add(new Student("小红", 24));
String collect = studentList.stream().map(Student::getName).collect(Collectors.joining(","));
System.out.println(collect);
}
}
内部通过StringBuilder来把每一个映射的值进行拼接
public static Collector<CharSequence, ?, String> joining(CharSequence delimiter,
CharSequence prefix,
CharSequence suffix) {
return new CollectorImpl<>(
() -> new StringJoiner(delimiter, prefix, suffix),
StringJoiner::add, StringJoiner::merge,
StringJoiner::toString, CH_NOID);
}
==================================================================
通过groupBy()实现对数据分组
**
根据年龄分组学生信息
public class CollectorsDemo {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 23));
studentList.add(new Student("李四", 23));
studentList.add(new Student("王五", 19));
studentList.add(new Student("小明", 19));
studentList.add(new Student("小红", 20));
Map<Integer, List<Student>> collect = studentList.stream().collect(Collectors.groupingBy(Student::getAge));
System.out.println(collect);
}
}
**
结果:
{19=[Student{id=null, name='王五', age=19}, Student{id=null, name='小明', age=19}], 20=[Student{id=null, name='小红', age=20}], 23=[Student{id=null, name='张三', age=23}, Student{id=null, name='李四', age=23}]}
Collectors.groupingBy提供了一个重载方法,其会在内层分组(第二个参数)结果,传递给外层分组(第一个参数)作为其继续分组的依据
按照年龄分组及是否及格分组
public class CollectorsDemo {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 23, true));
studentList.add(new Student("李四", 23, false));
studentList.add(new Student("王五", 19, true));
studentList.add(new Student("小明", 19, true));
studentList.add(new Student("小红", 20, true));
Map<Integer, Map<String, List<Student>>> collect = studentList.stream().collect(Collectors.groupingBy(Student::getAge, Collectors.groupingBy(item -> {
if (item.isPass()) {
return "pass";
} else {
return "no pass";
}
})));
System.out.println(collect);
}
}
运行结果:
{19={pass=[Student{id=null, name='王五', age=19, pass=true}, Student{id=null, name='小明', age=19, pass=true}]}, 20={pass=[Student{id=null, name='小红', age=20, pass=true}]}, 23={pass=[Student{id=null, name='张三', age=23, pass=true}], no pass=[Student{id=null, name='李四', age=23, pass=false}]}}
- 案例:根据年龄分组,然后统计总数
public class CollectorsDemo {
public static void main(String[] args) {
List<Student> studentList = new ArrayList<>();
studentList.add(new Student("张三", 23, true));
studentList.add(new Student("李四", 23, false));
studentList.add(new Student("王五", 19, true));
studentList.add(new Student("小明", 19, true));
studentList.add(new Student("小红", 20, true));
Map<Integer, Long> collect = studentList.stream().collect(Collectors.groupingBy(Student::getAge, Collectors.counting()));
System.out.println(collect);
}
}
## 一线互联网大厂Java核心面试题库
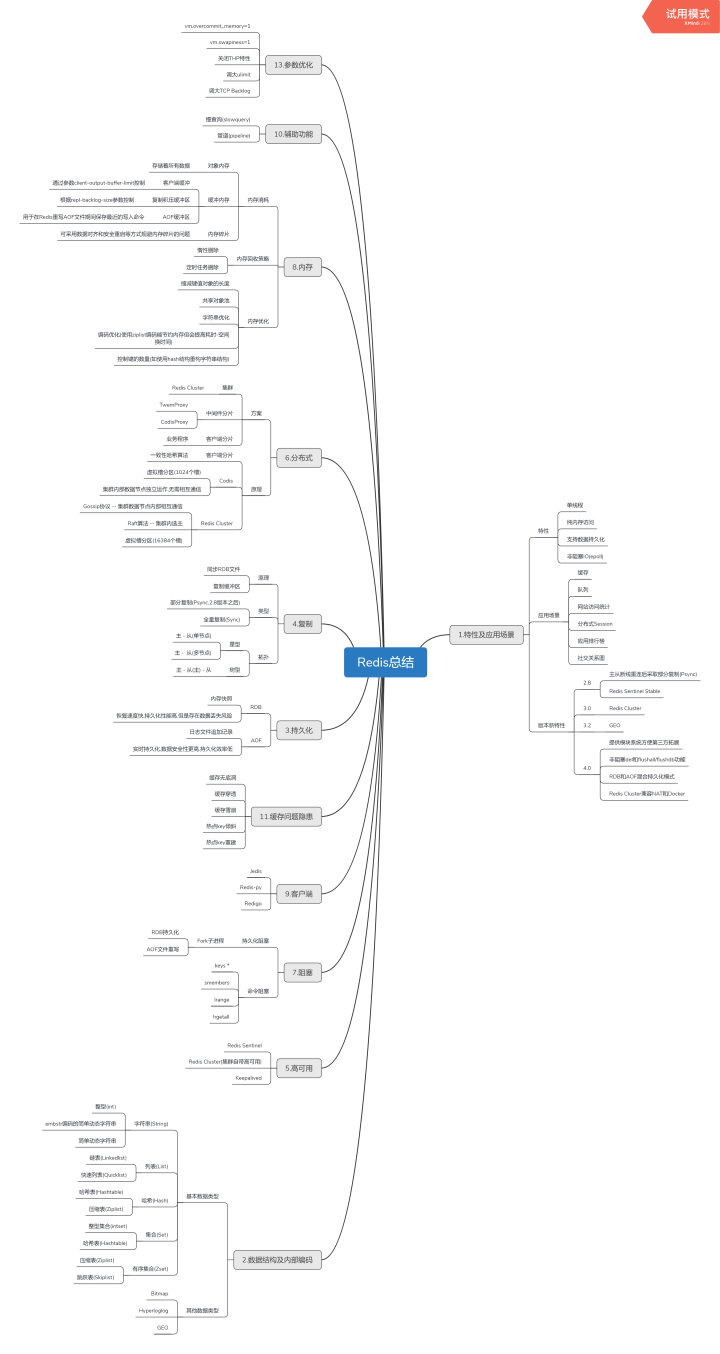
studentList.add(new Student("王五", 19, true));
studentList.add(new Student("小明", 19, true));
studentList.add(new Student("小红", 20, true));
Map<Integer, Long> collect = studentList.stream().collect(Collectors.groupingBy(Student::getAge, Collectors.counting()));
System.out.println(collect);
}
}
## 一线互联网大厂Java核心面试题库
[外链图片转存中...(img-kGsScAMG-1628146456791)]
正逢面试跳槽季,给大家整理了大厂问到的一些面试真题,由于文章长度限制,只给大家展示了部分题目,更多Java基础、异常、集合、并发编程、JVM、Spring全家桶、MyBatis、Redis、数据库、中间件MQ、Dubbo、Linux、Tomcat、ZooKeeper、Netty等等...已整理上传在**我的[腾讯文档【一线互联网大厂Java核心面试题库】点击即可领取](https://gitee.com/vip204888/java-p7)**,并会持续更新...感兴趣的朋友可以看看支持一波!