最后
Python崛起并且风靡,因为优点多、应用领域广、被大牛们认可。学习 Python 门槛很低,但它的晋级路线很多,通过它你能进入机器学习、数据挖掘、大数据,CS等更加高级的领域。Python可以做网络应用,可以做科学计算,数据分析,可以做网络爬虫,可以做机器学习、自然语言处理、可以写游戏、可以做桌面应用…Python可以做的很多,你需要学好基础,再选择明确的方向。这里给大家分享一份全套的 Python 学习资料,给那些想学习 Python 的小伙伴们一点帮助!
👉Python所有方向的学习路线👈
Python所有方向的技术点做的整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
👉Python必备开发工具👈
工欲善其事必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
👉Python全套学习视频👈
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
👉实战案例👈
学python就与学数学一样,是不能只看书不做题的,直接看步骤和答案会让人误以为自己全都掌握了,但是碰到生题的时候还是会一筹莫展。
因此在学习python的过程中一定要记得多动手写代码,教程只需要看一两遍即可。
👉大厂面试真题👈
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!
from nltk import download
from spellchecker import SpellChecker
def preprocess_text(text):
# 移除文本中的特殊字符和标点符号
processed_text = re.sub(r’[^\w\s]', ‘’, text)
# 将文本转换为小写
processed_text = processed_text.lower()
# 移除多余的空格
processed_text = re.sub(r'\s+', ' ', processed_text)
# 去除停用词
stop_words = set(stopwords.words('english'))
processed_text = ' '.join(word for word in processed_text.split() if word not in stop_words)
# 词形还原
download('averaged\_perceptron\_tagger')
download('wordnet')
lemmatizer = WordNetLemmatizer()
tokens = word_tokenize(processed_text)
tagged_tokens = pos_tag(tokens)
processed_text = ' '.join(lemmatizer.lemmatize(word, tag) for word, tag in tagged_tokens)
# 拼写纠正
spell = SpellChecker()
processed_text = ' '.join(spell.correction(word) for word in processed_text.split())
return processed_text
在这个修改后的函数中,我们首先导入了`nltk`库的`WordNetLemmatizer`、`word_tokenize`、`pos_tag`模块,以及`spellchecker`库的`SpellChecker`模块。
然后,在`preprocess_text`函数中,我们下载了`nltk`库的`averaged_perceptron_tagger`和`wordnet`资源,用于词形还原。
接下来,我们创建了一个词形还原器`lemmatizer`,使用`WordNetLemmatizer()`初始化。然后,我们使用`word_tokenize`函数将文本分词为单词列表,使用`pos_tag`函数为每个单词标记词性,然后使用列表推导式和词形还原器将每个单词还原为其原始形式,最后重新组合成一个经过词形还原的文本。
最后,我们创建了一个拼写纠正器`spell`,使用`SpellChecker()`初始化。然后,使用列表推导式和拼写纠正器对文本中的每个单词进行拼写纠正,并重新组合成一个经过拼写纠正的文本。
这样,预处理后的文本将进行词形还原和拼写纠正,以提高文本的质量和准确性。你可以根据自己的需求进一步修改这个函数,添加其他的预处理步骤,如实体识别、去除HTML标签等。
### 五、实体识别、去除HTML标签示例代码
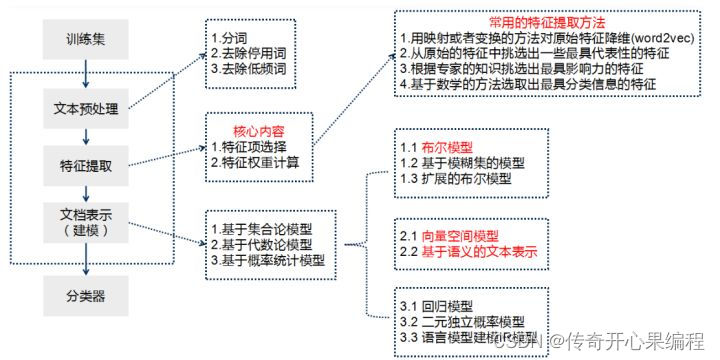要进行实体识别和去除HTML标签,我们可以使用`nltk`库中的`ne_chunk`和`BeautifulSoup`模块。下面是一个修改后的`preprocess_text`函数,包括实体识别和去除HTML标签的示例代码:
import re
import nltk
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
from nltk.tokenize import word_tokenize
from nltk import pos_tag
from nltk import ne_chunk
from nltk import download
from spellchecker import SpellChecker
from bs4 import BeautifulSoup
def preprocess_text(text):
# 去除HTML标签
processed_text = BeautifulSoup(text, “html.parser”).get_text()
# 移除文本中的特殊字符和标点符号
processed_text = re.sub(r'[^\w\s]', '', processed_text)
# 将文本转换为小写
processed_text = processed_text.lower()
# 移除多余的空格
processed_text = re.sub(r'\s+', ' ', processed_text)
# 去除停用词
stop_words = set(stopwords.words('english'))
processed_text = ' '.join(word for word in processed_text.split() if word not in stop_words)
# 词形还原
download('averaged\_perceptron\_tagger')
download('wordnet')
lemmatizer = WordNetLemmatizer()
tokens = word_tokenize(processed_text)
tagged_tokens = pos_tag(tokens)
processed_text = ' '.join(lemmatizer.lemmatize(word, tag) for word, tag in tagged_tokens)
# 拼写纠正
spell = SpellChecker()
processed_text = ' '.join(spell.correction(word) for word in processed_text.split())
# 实体识别
tagged_tokens = pos_tag(word_tokenize(processed_text))
processed_text = ' '.join(chunk.label() if hasattr(chunk, 'label') else chunk[0] for chunk in ne_chunk(tagged_tokens))
return processed_text
在这个修改后的函数中,我们首先导入了`nltk`库的`ne_chunk`模块,以及`BeautifulSoup`模块来处理HTML标签。
然后,在`preprocess_text`函数中,我们使用`BeautifulSoup`模块的`BeautifulSoup(text, "html.parser").get_text()`方法去除文本中的HTML标签。
接下来,我们继续之前的步骤,包括移除特殊字符和标点符号、转换为小写、移除多余的空格、去除停用词、词形还原和拼写纠正。
最后,我们使用`pos_tag`函数将文本中的单词标记词性,然后使用`ne_chunk`函数进行实体识别。我们使用列表推导式和条件判断语句,将识别出的实体标签保留下来,形成一个经过实体识别的文本。
这样,预处理后的文本将进行实体识别和去除HTML标签,以进一步提高文本的质量和准确性。你可以根据自己的需求进一步修改这个函数,添加其他的预处理步骤,如去除URL链接、处理缩写词等。
### 六、去除URL链接、处理缩写词示例代码
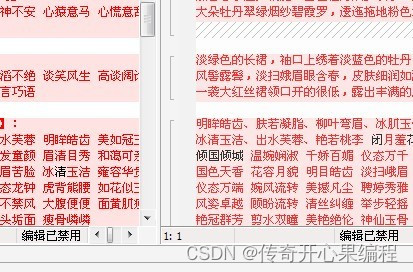要去除URL链接和处理缩写词,我们可以使用正则表达式来匹配和替换文本中的URL链接,以及使用一个缩写词词典来进行缩写词的替换。下面是一个修改后的`preprocess_text`函数,包括去除URL链接和处理缩写词的示例代码:
import re
import nltk
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
from nltk.tokenize import word_tokenize
from nltk import pos_tag
from nltk import ne_chunk
from nltk import download
from spellchecker import SpellChecker
from bs4 import BeautifulSoup
def preprocess_text(text):
# 去除HTML标签
processed_text = BeautifulSoup(text, “html.parser”).get_text()
# 去除URL链接
processed_text = re.sub(r'http\S+|www.\S+', '', processed_text)
# 移除文本中的特殊字符和标点符号
processed_text = re.sub(r'[^\w\s]', '', processed_text)
# 将文本转换为小写
processed_text = processed_text.lower()
# 移除多余的空格
processed_text = re.sub(r'\s+', ' ', processed_text)
# 去除停用词
stop_words = set(stopwords.words('english'))
processed_text = ' '.join(word for word in processed_text.split() if word not in stop_words)
# 处理缩写词
abbreviations = {
"can't": "cannot",
"won't": "will not",
"it's": "it is",
# 添加其他缩写词和对应的替换
}
processed_text = ' '.join(abbreviations.get(word, word) for word in processed_text.split())
# 词形还原
download('averaged\_perceptron\_tagger')
download('wordnet')
lemmatizer = WordNetLemmatizer()
tokens = word_tokenize(processed_text)
tagged_tokens = pos_tag(tokens)
processed_text = ' '.join(lemmatizer.lemmatize(word, tag) for word, tag in tagged_tokens)
# 拼写纠正
spell = SpellChecker()
processed_text = ' '.join(spell.correction(word) for word in processed_text.split())
# 实体识别
tagged_tokens = pos_tag(word_tokenize(processed_text))
processed_text = ' '.join(chunk.label() if hasattr(chunk, 'label') else chunk[0] for chunk in ne_chunk(tagged_tokens))
return processed_text
在这个修改后的函数中,我们首先导入了`re`模块,用于处理正则表达式匹配和替换。
然后,在`preprocess_text`函数中,我们使用`re.sub`函数和正则表达式`r'http\S+|www.\S+'`来匹配和替换文本中的URL链接。这样,我们可以将URL链接从文本中去除。
接下来,我们继续之前的步骤,包括去除HTML标签、移除特殊字符和标点符号、转换为小写、移除多余的空格、去除停用词、处理缩写词、词形还原和拼写纠正。
在处理缩写词时,我们创建了一个缩写词词典`abbreviations`,其中包含了一些常见的缩写词和对应的替换。你可以根据需要添加其他的缩写词和替换到这个词典中。
最后,我们使用`pos_tag`函数将文本中的单词标记词性,然后使用`ne_chunk`函数进行实体识别。我们使用列表推导式和条件判断语句,将识别出的实体标签保留下来,形成一个经过实体识别的文本。
这样,预处理后的文本将去除URL链接、处理缩写词,并进行其他的预处理步骤,以进一步提高文本的质量和准确性。你可以根据自己的需求进一步修改这个函数,添加其他的预处理步骤,如处理特定的符号、处理特定的文本模式等。
### 七、处理特定的符号、处理特定的文本模式示例代码
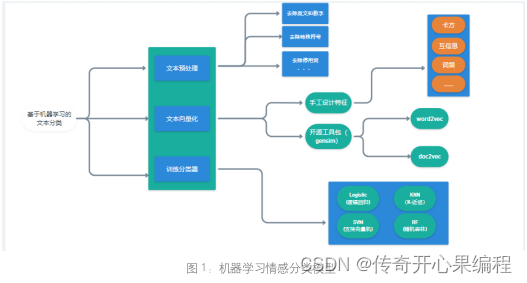根据你的需求,你可以进一步修改`preprocess_text`函数,添加其他的预处理步骤,如处理特定的符号、处理特定的文本模式等。下面是一个示例代码,包括处理特定符号和处理特定文本模式的预处理步骤:
import re
import nltk
from nltk.corpus import stopwords
from nltk.stem import WordNetLemmatizer
from nltk.tokenize import word_tokenize
from nltk import pos_tag
from nltk import ne_chunk
from nltk import download
from spellchecker import SpellChecker
from bs4 import BeautifulSoup
def preprocess_text(text):
# 去除HTML标签
processed_text = BeautifulSoup(text, “html.parser”).get_text()
# 去除URL链接
processed_text = re.sub(r'http\S+|www.\S+', '', processed_text)
# 移除文本中的特殊字符和标点符号
processed_text = re.sub(r'[^\w\s]', '', processed_text)
# 处理特定符号
processed_text = re.sub(r'\$', ' dollar ', processed_text)
processed_text = re.sub(r'%', ' percent ', processed_text)
# 将文本转换为小写
processed_text = processed_text.lower()
# 移除多余的空格
processed_text = re.sub(r'\s+', ' ', processed_text)
# 去除停用词
stop_words = set(stopwords.words('english'))
processed_text = ' '.join(word for word in processed_text.split() if word not in stop_words)
# 处理缩写词
abbreviations = {
"can't": "cannot",
"won't": "will not",
"it's": "it is",
# 添加其他缩写词和对应的替换
}
processed_text = ' '.join(abbreviations.get(word, word) for word in processed_text.split())
# 词形还原
download('averaged\_perceptron\_tagger')
download('wordnet')
lemmatizer = WordNetLemmatizer()
tokens = word_tokenize(processed_text)
tagged_tokens = pos_tag(tokens)
processed_text = ' '.join(lemmatizer.lemmatize(word, tag) for word, tag in tagged_tokens)
# 拼写纠正
spell = SpellChecker()
processed_text = ' '.join(spell.correction(word) for word in processed_text.split())
# 实体识别
tagged_tokens = pos_tag(word_tokenize(processed_text))
processed_text = ’ '.join(chunk.label() if hasattr(chunk, ‘label’) else chunk[0] for chunk in ne_chunk(tagged_tokens))
# 处理特定文本模式
# 示例:将日期格式统一为YYYY-MM-DD
processed_text = re.sub(r'\b(\d{1,2})[/-](\d{1,2})[/-](\d{2,4})\b', r'\3-\1-\2', processed_text)
# 示例:将电话号码格式统一为xxx-xxx-xxxx
processed_text = re.sub(r'\b(\d{3})[ -]?(\d{3})[ -]?(\d{4})\b', r'\1-\2-\3', processed_text)
return processed_text
在这个示例代码中,我们添加了两个处理特定文本模式的预处理步骤:
1. 将日期格式统一为YYYY-MM-DD:使用正则表达式`\b(\d{1,2})[/-](\d{1,2})[/-](\d{2,4})\b`匹配日期格式,并使用替换模式`\3-\1-\2`将日期格式统一为YYYY-MM-DD。
2. 将电话号码格式统一为xxx-xxx-xxxx:使用正则表达式`\b(\d{3})[ -]?(\d{3})[ -]?(\d{4})\b`匹配电话号码格式,并使用替换模式`\1-\2-\3`将电话号码格式统一为xxx-xxx-xxxx。
### 八、归纳总结
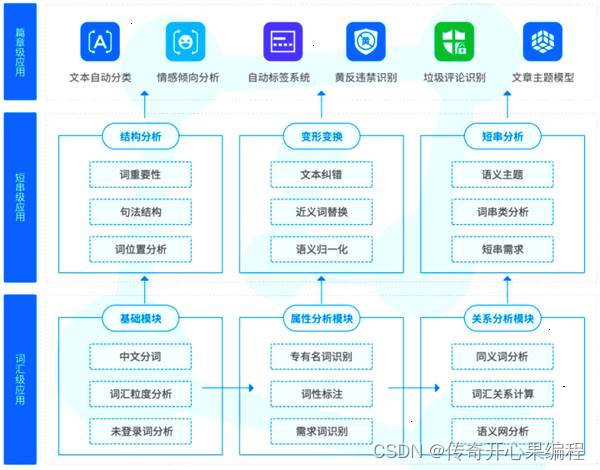下面是对`pyttsx3`中的`preprocess_text`函数进行归纳总结的知识点:
1. HTML标签去除:使用`BeautifulSoup`库去除文本中的HTML标签,以确保纯文本的输入。
2. URL链接去除:使用正则表达式`re.sub`函数去除文本中的URL链接。
3. 特殊字符和标点符号去除:使用正则表达式`re.sub`函数去除文本中的特殊字符和标点符号。
学好 Python 不论是就业还是做副业赚钱都不错,但要学会 Python 还是要有一个学习规划。最后大家分享一份全套的 Python 学习资料,给那些想学习 Python 的小伙伴们一点帮助!
### 一、Python所有方向的学习路线
Python所有方向路线就是把Python常用的技术点做整理,形成各个领域的知识点汇总,它的用处就在于,你可以按照上面的知识点去找对应的学习资源,保证自己学得较为全面。
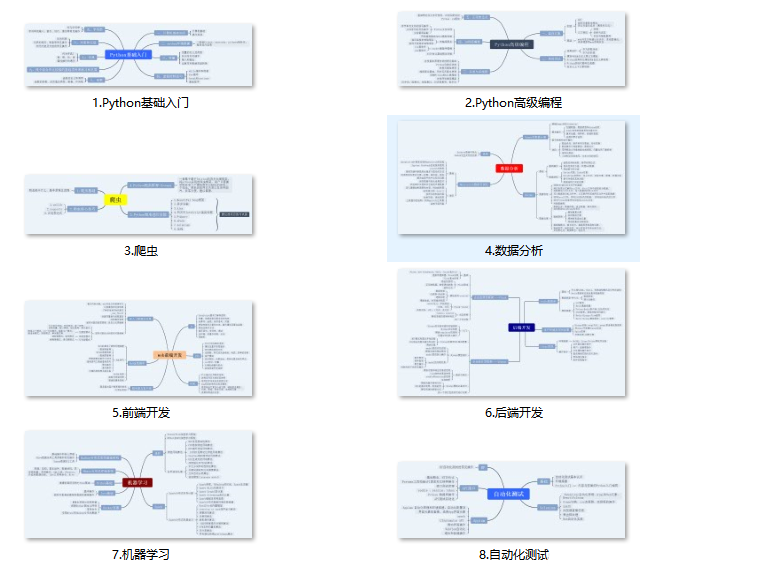
### 二、学习软件
工欲善其事必先利其器。学习Python常用的开发软件都在这里了,给大家节省了很多时间。
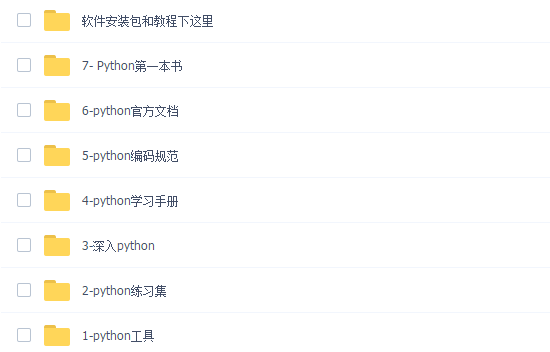
### 三、全套PDF电子书
书籍的好处就在于权威和体系健全,刚开始学习的时候你可以只看视频或者听某个人讲课,但等你学完之后,你觉得你掌握了,这时候建议还是得去看一下书籍,看权威技术书籍也是每个程序员必经之路。
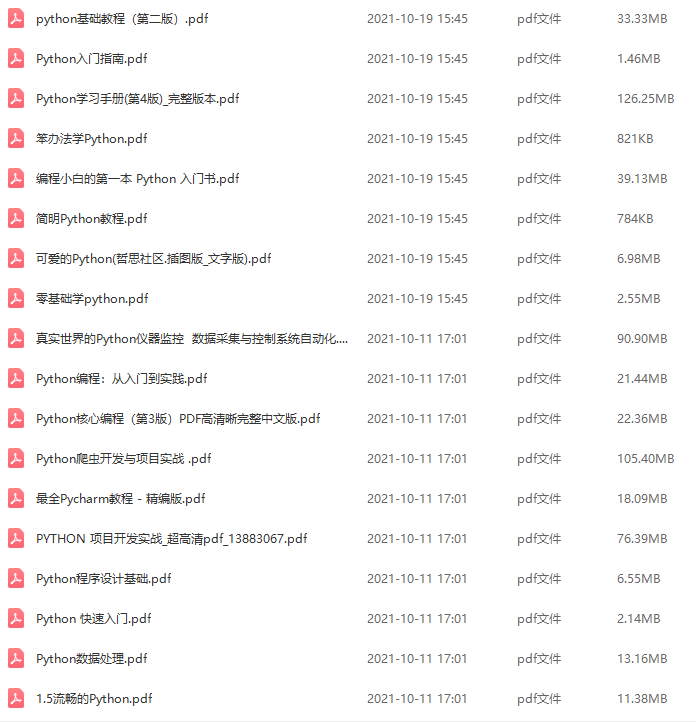
### 四、入门学习视频
我们在看视频学习的时候,不能光动眼动脑不动手,比较科学的学习方法是在理解之后运用它们,这时候练手项目就很适合了。
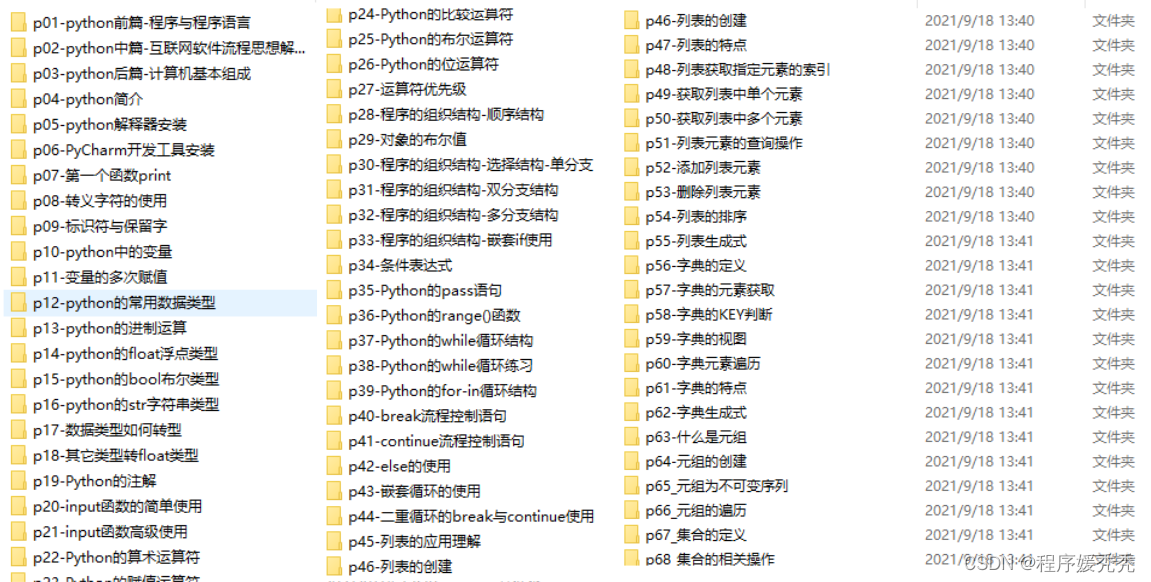
### 五、实战案例
光学理论是没用的,要学会跟着一起敲,要动手实操,才能将自己的所学运用到实际当中去,这时候可以搞点实战案例来学习。
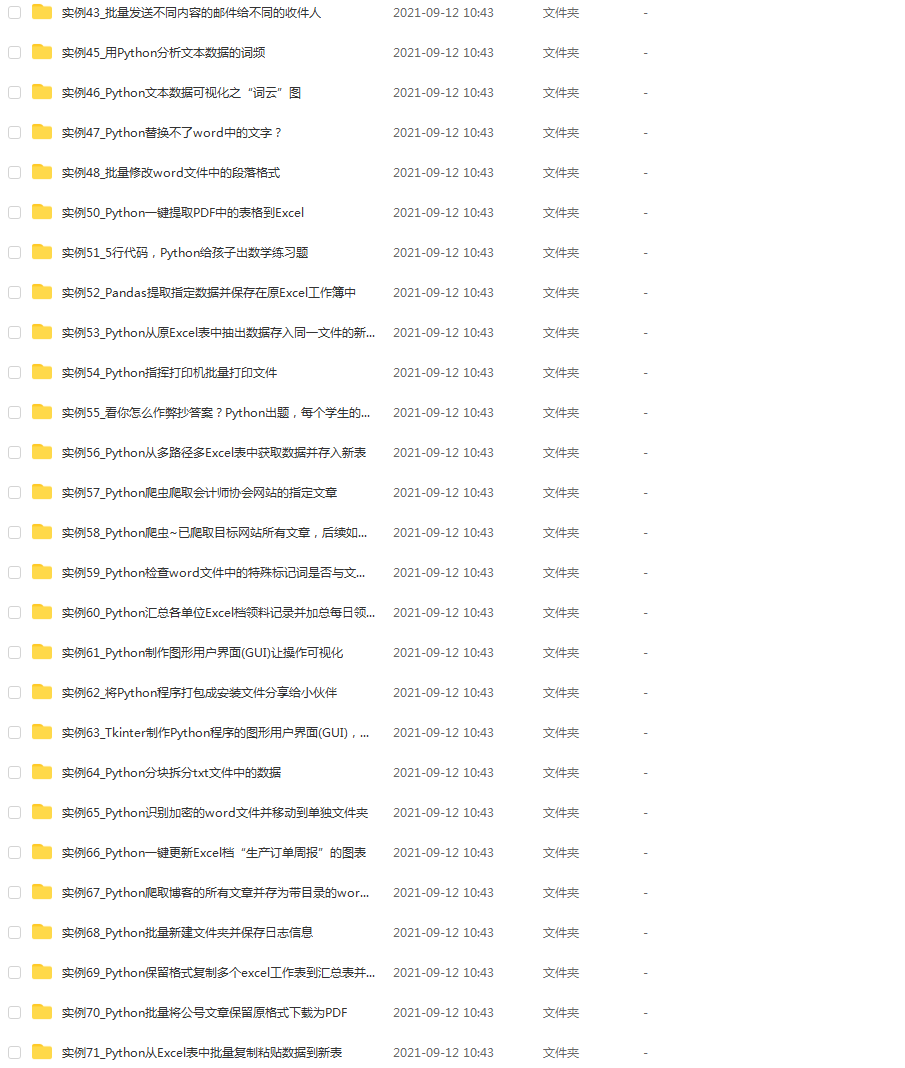
### 六、面试资料
我们学习Python必然是为了找到高薪的工作,下面这些面试题是来自阿里、腾讯、字节等一线互联网大厂最新的面试资料,并且有阿里大佬给出了权威的解答,刷完这一套面试资料相信大家都能找到满意的工作。
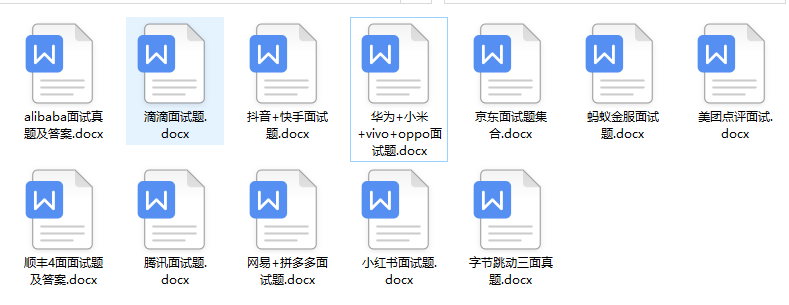
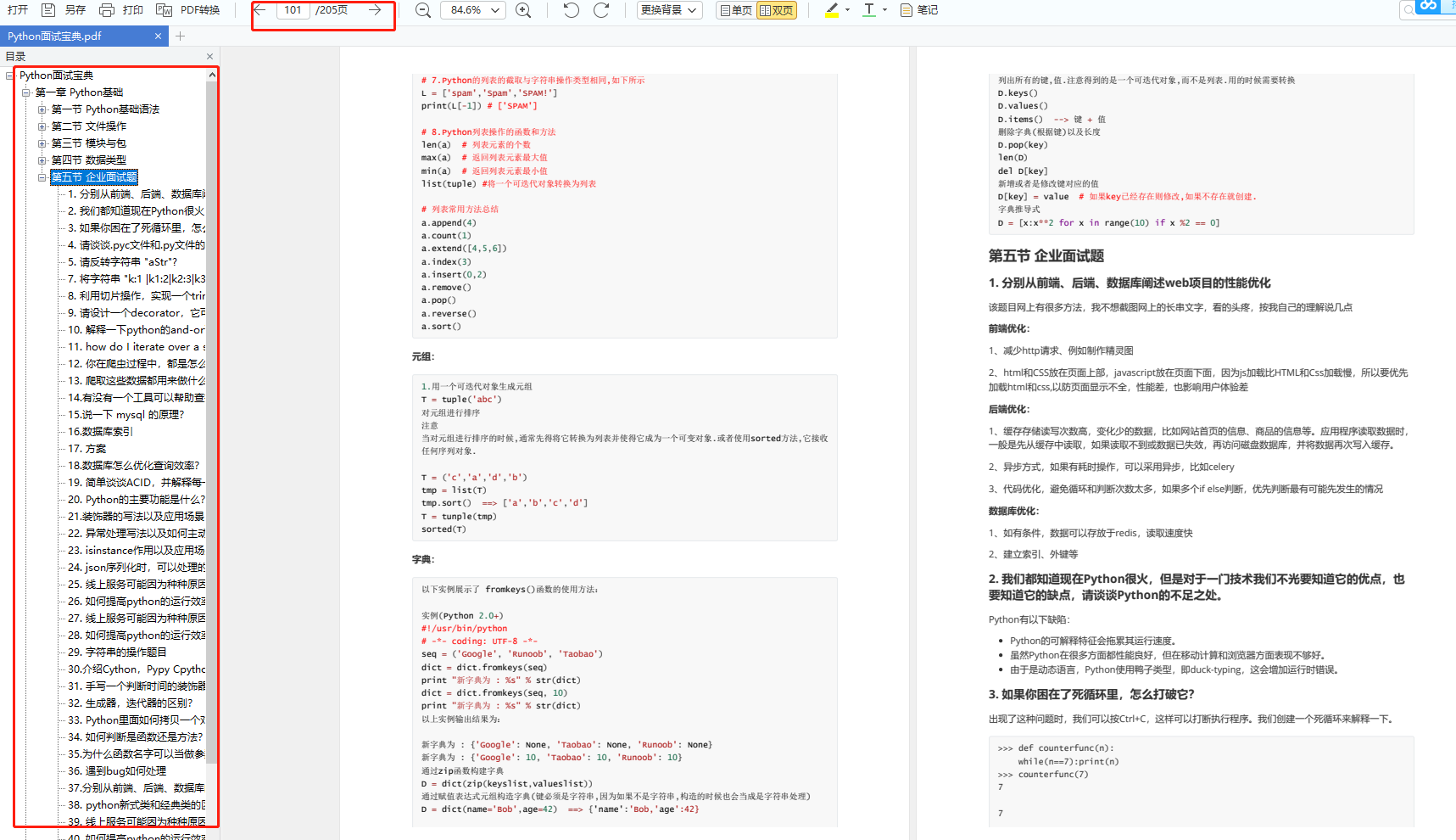
**网上学习资料一大堆,但如果学到的知识不成体系,遇到问题时只是浅尝辄止,不再深入研究,那么很难做到真正的技术提升。**
**[需要这份系统化学习资料的朋友,可以戳这里获取](https://bbs.csdn.net/forums/4304bb5a486d4c3ab8389e65ecb71ac0)**
**一个人可以走的很快,但一群人才能走的更远!不论你是正从事IT行业的老鸟或是对IT行业感兴趣的新人,都欢迎加入我们的的圈子(技术交流、学习资源、职场吐槽、大厂内推、面试辅导),让我们一起学习成长!**