目录
一、概述
我们在编码过程中一定是要处理异常的,传统异常处理方式存在一些问题:
- 1. 项目中会有很多地方都要进行异常处理,编码麻烦,代码冗余,不易维护
- 2. 异常处理的方式不统一
- 3. 错误提示信息不统一,不友好
为了解决上述问题,我们在
P2P
项目中设计了一套好用的异常处理机制:
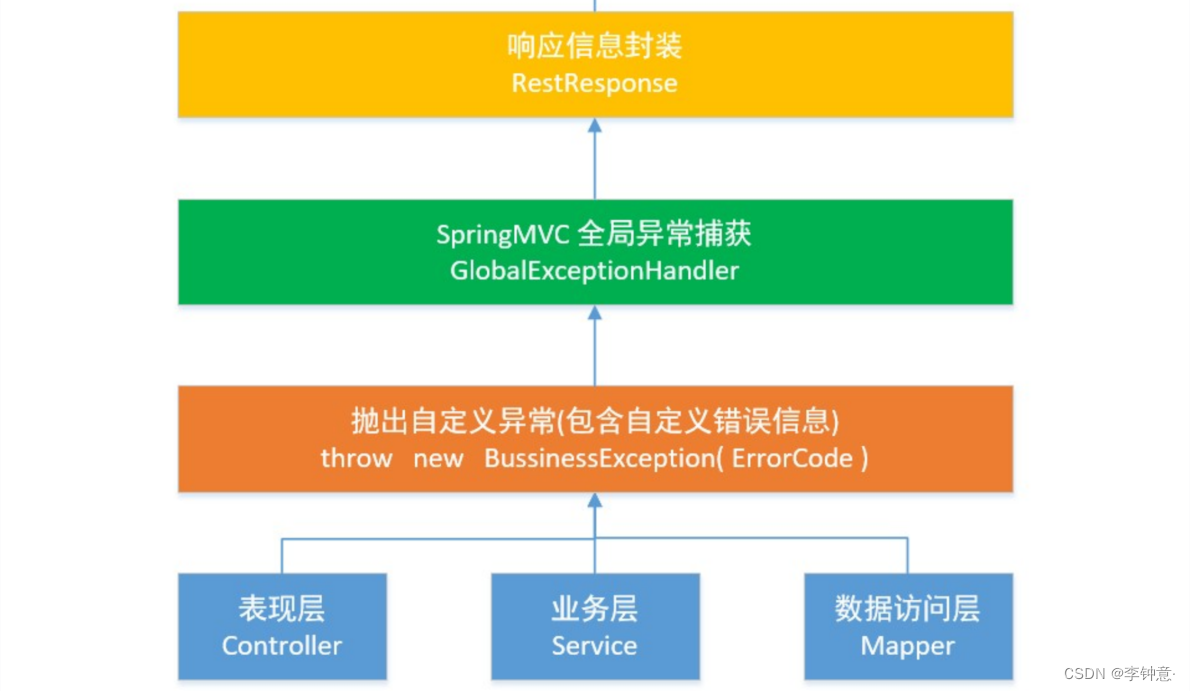
- 1. 自定义异常类,自定义错误代码和提示信息(统一且友好)
- 2. 各层只抛异常(建议在业务层),不做异常处理
- 3. 异常捕获和处理统一交给SpringMVC的全局异常捕获类
- 4. 响应给前端的错误提示信息进行统一封装
二. API设计
1、
自定义错误代码和提示信息 接口:ErrorCode.java
public interface ErrorCode {
int getCode();
String getDesc();
}
2、CommonErrorCode.java
提供通用错误编码和提示信息:
/**
* 异常编码 0成功、-1熔断、 -2 标准参数校验不通过 -3会话超时
* 前两位:服务标识
* 中间两位:模块标识
* 后两位:异常标识
*/
public enum CommonErrorCode implements ErrorCode {
公用异常编码 //
SUCCESS(0, "成功"),
FUSE(-1, "网关调用熔断"),
/**
* 传入参数与接口不匹配
*/
E_100101(100101,"传入参数与接口不匹配"),
/**
* 验证码错误
*/
E_100102(100102,"验证码错误"),
/**
* 验证码为空
*/
E_100103(100103,"验证码为空"),
/**
* 查询结果为空
*/
E_100104(100104,"查询结果为空"),
/**
* ID格式不正确或超出Long存储范围
*/
E_100105(100105,"ID格式不正确或超出Long存储范围"),
E_100106(100106,"请求失败"),
E_999990(999990,"调用微服务-交易中心 被熔断"),
E_999991(999991,"调用微服务-授权服务 被熔断"),
E_999992(999992,"调用微服务-用户服务 被熔断"),
E_999993(999993,"调用微服务-资源服务 被熔断"),
E_999994(999994,"调用微服务-同步服务 被熔断"),
E_999995(999995,"调用微服务-统一账户服务 被熔断"),
E_999996(999996,"调用微服务-存管代理服务 被熔断"),
/**
* 调用微服务-还款服务 被熔断
*/
E_999997(999997,"调用微服务-还款服务 被熔断"),
CUSTOM(999998,"自定义异常"),
/**
* 未知错误
*/
UNKOWN(999999,"未知错误");
private int code;
private String desc;
@Override
public int getCode() {
return code;
}
@Override
public String getDesc() {
return desc;
}
private CommonErrorCode(int code, String desc) {
this.code = code;
this.desc = desc;
}
public static CommonErrorCode setErrorCode(int code) {
for (CommonErrorCode errorCode : CommonErrorCode.values()) {
if (errorCode.getCode()==code) {
return errorCode;
}
}
return null;
}
}
3、各个微服务可以根据自身业务提供错误编码和提示信息类,例如:统一账户微服务的
AccountErrorCode.java
/**
* 异常编码 0成功、-1熔断、 -2 标准参数校验不通过 -3会话超时
* 前两位:服务标识
* 中间两位:模块标识
* 后两位:异常标识
* 统一账号服务异常编码 以13开始
*/
public enum AccountErrorCode implements ErrorCode {
E_130101(130101, "用户名已存在"),
E_130104(130104, "用户未注册"),
E_130105(130105, "用户名或密码错误"),
E_140141(140141,"注册失败"),
E_140151(140151,"获取短信验证码失败"),
E_140152(140152,"验证码错误"),
;
private int code;
private String desc;
@Override
public int getCode() {
return code;
}
@Override
public String getDesc() {
return desc;
}
private AccountErrorCode(int code, String desc) {
this.code = code;
this.desc = desc;
}
public static AccountErrorCode setErrorCode(int code) {
for (AccountErrorCode errorCode : AccountErrorCode.values()) {
if (errorCode.getCode()==code) {
return errorCode;
}
}
return null;
}
}
4、自定义异常类:BusinessException.java
//业务异常
public class BusinessException extends RuntimeException {
private static final long serialVersionUID = 5565760508056698922L;
private ErrorCode errorCode;
public BusinessException(ErrorCode errorCode) {
super();
this.errorCode = errorCode;
}
public BusinessException() {
super();
}
public BusinessException(String arg0, Throwable arg1, boolean arg2, boolean arg3) {
super(arg0, arg1, arg2, arg3);
}
public BusinessException(ErrorCode errorCode, String arg0, Throwable arg1, boolean arg2, boolean arg3) {
super(arg0, arg1, arg2, arg3);
this.errorCode = errorCode;
}
public BusinessException(String arg0, Throwable arg1) {
super(arg0, arg1);
}
public BusinessException(ErrorCode errorCode, String arg0, Throwable arg1) {
super(arg0, arg1);
this.errorCode = errorCode;
}
public BusinessException(String arg0) {
super(arg0);
}
public BusinessException(ErrorCode errorCode, String arg0) {
super(arg0);
this.errorCode = errorCode;
}
public BusinessException(Throwable arg0) {
super(arg0);
}
public BusinessException(ErrorCode errorCode, Throwable arg0) {
super(arg0);
this.errorCode = errorCode;
}
public ErrorCode getErrorCode() {
return errorCode;
}
public void setErrorCode(ErrorCode errorCode) {
this.errorCode = errorCode;
}
}
5、SpringMVC全局异常捕获类:GlobalExceptionHandler.java
@ControllerAdvice
@Slf4j
public class GlobalExceptionHandler {
private final static Logger LOGGER = LoggerFactory.getLogger(GlobalExceptionHandler.class);
@ExceptionHandler(value = Exception.class)
@ResponseBody
public RestResponse<Nullable> exceptionGet(HttpServletRequest req, HttpServletResponse response , Exception e) {
if (e instanceof BusinessException) {
BusinessException be = (BusinessException) e;
if(CommonErrorCode.CUSTOM.equals(be.getErrorCode())){
return new RestResponse<Nullable>(be.getErrorCode().getCode(), be.getMessage());
}else{
return new RestResponse<Nullable>(be.getErrorCode().getCode(), be.getErrorCode().getDesc());
}
}else if(e instanceof NoHandlerFoundException){
return new RestResponse<Nullable>(404, "找不到资源");
}else if(e instanceof HttpRequestMethodNotSupportedException){
return new RestResponse<Nullable>(405, "method 方法不支持");
}else if(e instanceof HttpMediaTypeNotSupportedException){
return new RestResponse<Nullable>(415, "不支持媒体类型");
}
log.error("【系统异常】" + e.getMessage());
return new RestResponse<Nullable>(CommonErrorCode.UNKOWN.getCode(), CommonErrorCode.UNKOWN.getDesc());
}
}