/** Alias for [W300] */
@Stable
val Light = W300
/** The default font weight - alias for [W400] */
@Stable
val Normal = W400
/** Alias for [W500] */
@Stable
val Medium = W500
/** Alias for [W600] */
@Stable
val SemiBold = W600
/**
* A commonly used font weight that is heavier than normal - alias for [W700]
*/
@Stable
val Bold = W700
/** Alias for [W800] */
@Stable
val ExtraBold = W800
/** Alias for [W900] */
@Stable
val Black = W900
/** A list of all the font weights. */
internal val values: List<FontWeight> = listOf(
W100,
W200,
W300,
W400,
W500,
W600,
W700,
W800,
W900
)
看一下他们的显示效果:
@Composable
fun MyText() {
Column {
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.Thin,
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.ExtraLight
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.Light
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.Normal
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.Medium
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.SemiBold
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.Bold
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.ExtraBold
)
Text(
text = stringResource(R.string.my_text),
fontWeight = FontWeight.Black
)
}
}
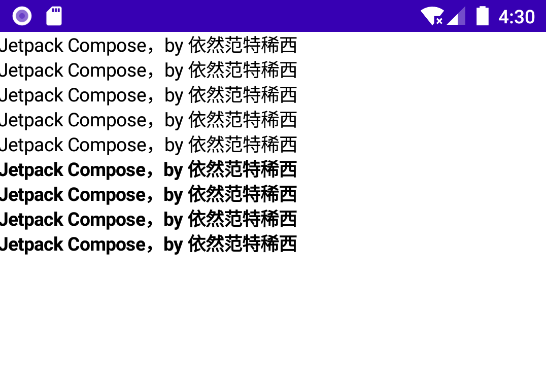
##### fontFamily 更改字体
`fontFamily`可以更改字体,FontFamily为我们默认内置了5种字体,`Default`、`SansSerif`、`Serif`、`Monospace`、`Cursive`。
@Composable
fun MyText() {
Column {
Text(
text = stringResource(R.string.my_text),
fontFamily = FontFamily.Default,
)
Text(
text = stringResource(R.string.my_text),
fontFamily = FontFamily.SansSerif
)
Text(
text = stringResource(R.string.my_text),
fontFamily = FontFamily.Serif
)
Text(
text = stringResource(R.string.my_text),
fontFamily = FontFamily.Monospace
)
Text(
text = stringResource(R.string.my_text),
fontFamily = FontFamily.Cursive
)
}
}
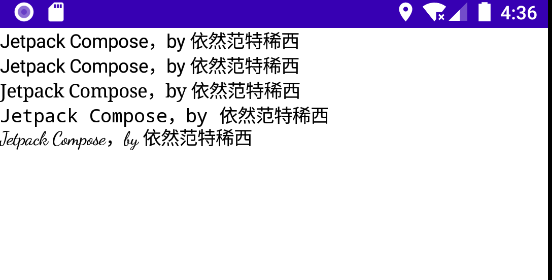
##### letterSpacing 更改文字间距
`letterSpacing`更改文字间距,更确切的说是更改字符间距,因为如果是英文的话,不会按单词来设置间距,而是按字母。
@Composable
fun MyText() {
Text(
text = stringResource(R.string.my_text),
letterSpacing = 10.sp,
)
}
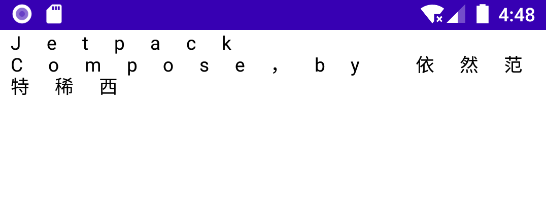
##### textDecoration 文字装饰器
`textDecoration`可以给文字装饰下划线和中划线。默认提供了三个值:
* `None`: 无装饰效果
* `LineThrough`: 添加中划线
* `Underline`: 添加下划线
@Composable
fun MyText() {
Column {
Text(
text = stringResource(R.string.my_text),
textDecoration = TextDe