6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
6)创建对应的实体对象
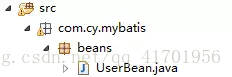
对应的java代码:
1 package com.cy.mybatis.beans;
2
3 import java.io.Serializable;
4
5 public class UserBean implements Serializable{
6
7 private static final long serialVersionUID = 1L;
8 private Integer id;
9 private String username;
10 private String password;
11 private Double account;
12
13 public UserBean() {
14 super();
15 }
16
17 public UserBean(String username, String password, Double account) {
18 super();
19 this.username = username;
20 this.password = password;
21 this.account = account;
22 }
23
24 public UserBean(Integer id, String username, String password, Double account) {
25 super();
26 this.id = id;
27 this.username = username;
28 this.password = password;
29 this.account = account;
30 }
31
32 public Integer getId() {
33 return id;
34 }
35
36 public void setId(Integer id) {
37 this.id = id;
38 }
39
40 public String getUsername() {
41 return username;
42 }
43
44 public void setUsername(String username) {
45 this.username = username;
46 }
47
48 public String getPassword() {
49 return password;
50 }
51
52 public void setPassword(String password) {
53 this.password = password;
54 }
55
56 public Double getAccount() {
57 return account;
58 }
59
60 public void setAccount(Double account) {
61 this.account = account;
62 }
63
64 @Override
65 public String toString() {
66 return “UserBean [id=” + id + “, username=” + username + “, password=”
67 + password + “, account=” + account + “]”;
68 }
69
70
71
72
73 }
7)创建方法接口UserMapper.java和定义操作t\_user表的sql映射文件UserMapper.xml
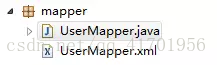
提供简单的增删改查数据信息。
1 package com.cy.mybatis.mapper;
2
3 import java.util.List;
4
5 import com.cy.mybatis.beans.UserBean;
6
7 public interface UserMapper {
8 /**
9 * 新增用戶
10 * @param user
11 * @return
12 * @throws Exception
13 */
14 public int insertUser(UserBean user) throws Exception;
15 /**
16 * 修改用戶
17 * @param user
18 * @param id
19 * @return
20 * @throws Exception
21 */
22 public int updateUser (UserBean user,int id) throws Exception;
23 /**
24 * 刪除用戶
25 * @param id
26 * @return
27 * @throws Exception
28 */
29 public int deleteUser(int id) throws Exception;
30 /**
31 * 根据id查询用户信息
32 * @param id
33 * @return
34 * @throws Exception
35 */
36 public UserBean selectUserById(int id) throws Exception;
37 /**
38 * 查询所有的用户信息
39 * @return
40 * @throws Exception
41 */
42 public List selectAllUser() throws Exception;
43 }
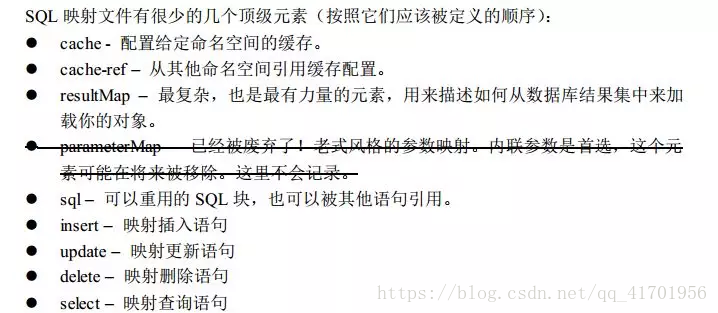
UserMapper.xml
1 <?xml version="1.0" encoding="UTF-8"?>
2
3
4
5
6
7
8
9
10
11
12 <!-- useGeneratedKeys:( 仅 对 insert 有 用 ) 这 会 告 诉 MyBatis 使 用 JDBC 的getGeneratedKeys
13 方法来取出由数据(比如:像 MySQL 和 SQLServer 这样的数据库管理系统的自动递增字段)内部生成的主键。默认值: false。 -->
14
15
16
17
18
19 insert into t_user (username,password,account) values (#{username},#{password},#{account})
20
21
22
23 update t_user set username=#{username},password=#{password},account=#{account} where id=#{id}
24
25
26
27 delete from t_user where id=#{id}
28
29
30
31 select * from t_user where id=#{id}
32
33
34
35 select * from t_user
36
37
38
39
这时需要为mybatis.cfg.xml里注册UserMapper.xml文件。
1 <?xml version="1.0" encoding="UTF-8"?>
2
3
4
5
6
7
8
9
10
11 <!-- 别名方式1,一个一个的配置 type中放置的是类的全路径,alias中放置的是类别名
12 -->
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39 <!-- 告知映射文件方式1,一个一个的配置
40 –>
41
42
43
44
8)需要建立一个工具类文件

1 package com.cy.mybatis.tools;
2
3 import java.io.Reader;
4
5 import org.apache.ibatis.io.Resources;
6 import org.apache.ibatis.session.SqlSession;
7 import org.apache.ibatis.session.SqlSessionFactory;
8 import org.apache.ibatis.session.SqlSessionFactoryBuilder;
9
10 public class DBTools {
11 public static SqlSessionFactory sessionFactory;
12
13 static{
14 try {
15 //使用MyBatis提供的Resources类加载mybatis的配置文件
16 Reader reader = Resources.getResourceAsReader(“mybatis.cfg.xml”);
17 //构建sqlSession的工厂
18 sessionFactory = new SqlSessionFactoryBuilder().build(reader);
19 } catch (Exception e) {
20 e.printStackTrace();
21 }
22
23 }
24 //创建能执行映射文件中sql的sqlSession
25 public static SqlSession getSession(){
26 return sessionFactory.openSession();
27 }
28
29 }
9)写个测试
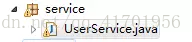
1 package com.cy.mybatis.service;
2
3 import java.util.List;
4
5 import org.apache.ibatis.session.SqlSession;
6
7 import com.cy.mybatis.beans.UserBean;
8 import com.cy.mybatis.tools.DBTools;
9 import com.cy.mybatis.mapper.UserMapper;
10
11 public class UserService {
12
13
14 15
16 public static void main(String[] args) {
17 insertUser();
18 // deleteUser();
19 // selectUserById();
20 // selectAllUser();
21 }
22
23
24 /**
25 * 新增用户
26 */
27 private static void insertUser() {
28 SqlSession session = DBTools.getSession();
29 UserMapper mapper = session.getMapper(UserMapper.class);
30 UserBean user = new UserBean(“懿”, “1314520”, 7000.0);
31 try {
32 mapper.insertUser(user);
33 System.out.println(user.toString());
34 session.commit();
35 } catch (Exception e) {
36 e.printStackTrace();
37 session.rollback();
38 }
39 }
40
41