opsForHash()------HashMap键值对操作
二、Spring 整合使用Spring Data Redis
①引入依赖
org.springframework.data spring-data-redis 2.1.5.RELEASE redis.clients jedis 2.9.1
②编写redis配置文件:redis.properties
#redis地址
redis.host=127.0.0.1
#redis端口
redis.port=6379
#redis密码,一般不需要
redis.password=""
#最大空闲时间
redis.maxIdle=400
#最大连接数
redis.maxTotal=6000
#最大等待时间
redis.maxWaitMillis=1000
#连接耗尽时是否阻塞,false报异常,true阻塞超时 默认:true
redis.blockWhenExhausted=true
#在获得链接的时候检查有效性,默认false
redis.testOnBorrow=true
#超时时间,默认:2000
redis.timeout=100000
③编写Spring配置文件并配置Redis:application.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd http://www.springframework.org/schema/aop
http://www.springframework.org/schema/aop/spring-aop.xsd">
<!--开启注解扫描-->
<context:annotation-config/>
<!--指定组件扫描范围-->
<context:component-scan base-package="com.my.spring"/>
<!--引入redis资源文件-->
<context:property-placeholder location="classpath*:redis.properties"/>
<!-- redis数据源 -->
<bean id="poolConfig" class="redis.clients.jedis.JedisPoolConfig">
<!-- 最大空闲数 -->
<property name="maxIdle" value="${redis.maxIdle}" />
<!-- 最大空连接数 -->
<property name="maxTotal" value="${redis.maxTotal}" />
<!-- 最大等待时间 -->
<property name="maxWaitMillis" value="${redis.maxWaitMillis}" />
<!-- 连接超时时是否阻塞,false时报异常,ture阻塞直到超时, 默认true -->
<property name="blockWhenExhausted" value="${redis.blockWhenExhausted}" />
<!-- 返回连接时,检测连接是否成功 -->
<property name="testOnBorrow" value="${redis.testOnBorrow}" />
</bean>
<!-- Spring-redis连接池管理工厂 -->
<bean id="jedisConnectionFactory" class="org.springframework.data.redis.connection.jedis.JedisConnectionFactory">
<!-- IP地址 -->
<property name="hostName" value="${redis.host}" />
<!-- 端口号 -->
<property name="port" value="${redis.port}" />
<!-- 超时时间 默认2000-->
<property name="timeout" value="${redis.timeout}" />
<!-- 连接池配置引用 -->
<property name="poolConfig" ref="poolConfig" />
<!-- usePool:是否使用连接池 -->
<property name="usePool" value="true"/>
</bean>
<!-- 注册RedisTemplate -->
<bean id="redisTemplate" class="org.springframework.data.redis.core.RedisTemplate">
<!--注入连接池管理工具-->
<property name="connectionFactory" ref="jedisConnectionFactory" />
<!--设置Redis的key序列化方式-->
<property name="keySerializer">
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<!--设置Redis的value序列化方式-->
<property name="valueSerializer">
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<!--设置Redis存入haseMap时的key序列化方式-->
<property name="hashKeySerializer">
<bean class="org.springframework.data.redis.serializer.StringRedisSerializer" />
</property>
<!--设置Redis存入haseMap时的value序列化方式-->
<property name="hashValueSerializer">
<bean class="org.springframework.data.redis.serializer.JdkSerializationRedisSerializer" />
</property>
<!--开启事务 -->
<property name="enableTransactionSupport" value="true"></property>
</bean>
<!--自定义redis工具类 -->
<bean id="redisHelper" class="com.my.spring.util.RedisHelper">
<property name="redisTemplate" ref="redisTemplate" />
</bean>
</beans>
④编写Redis工具类:RedisManager,注入RedisTemplate
@Data
public class RedisHelper {
//注入RedisTemplate
private RedisTemplate<String,Object> redisTemplate;
/**
* 设置过期时间
* @param key
* @param time
* @return
*/
public boolean expire(String key, long time) {
return this.redisTemplate.expire(key,time, TimeUnit.SECONDS);
}
/**
* 是否存在key
* @param key
* @return
*/
public Object hasKey(String key){
return this.redisTemplate.hasKey(key);
# **Java高频面试专题合集解析:**
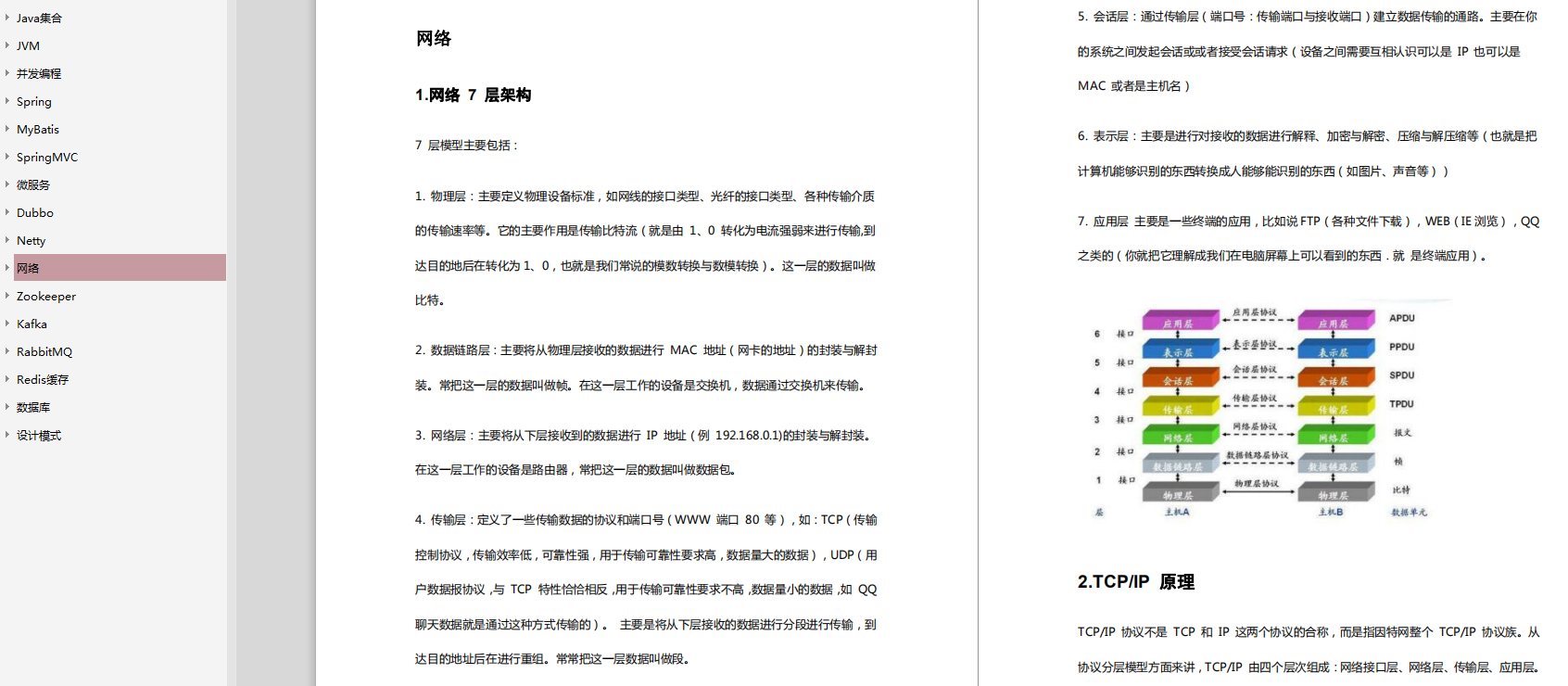
当然在这还有更多整理总结的Java进阶学习笔记和面试题未展示,其中囊括了**Dubbo、Redis、Netty、zookeeper、Spring cloud、分布式、高并发等架构资料和完整的Java架构学习进阶导图!**
**[CodeChina开源项目:【一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频】](
)**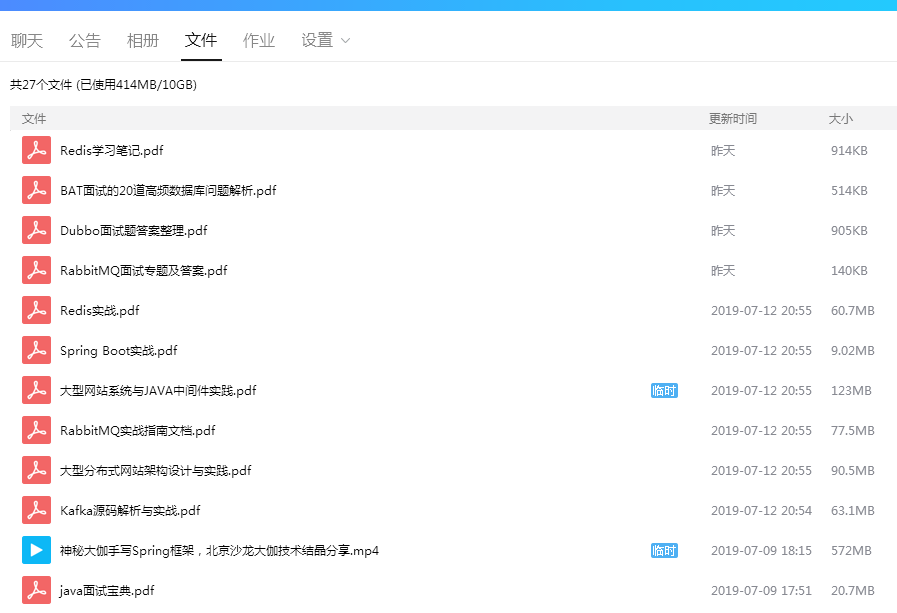
**更多Java架构进阶资料展示**
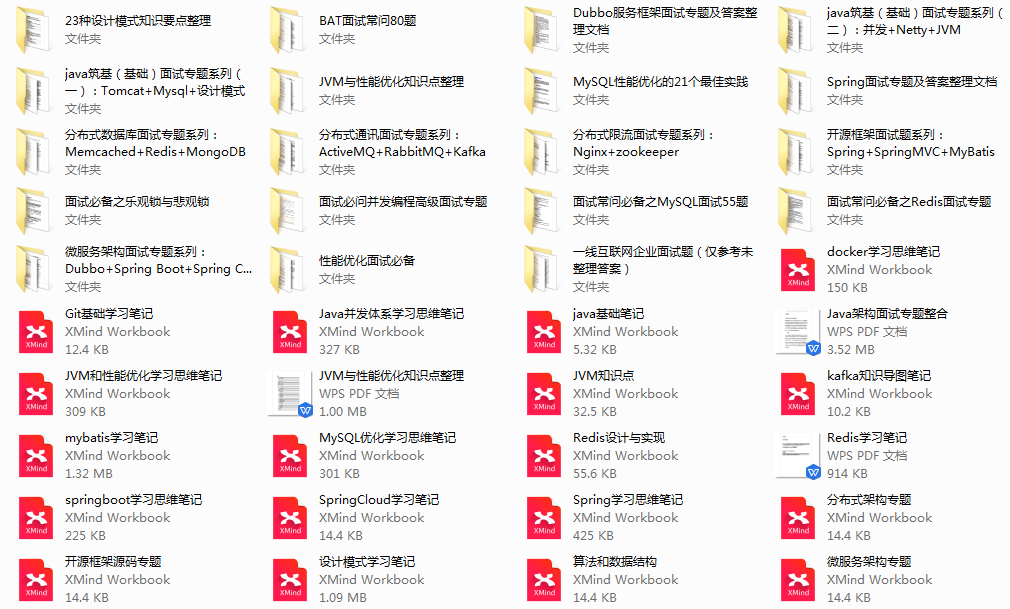
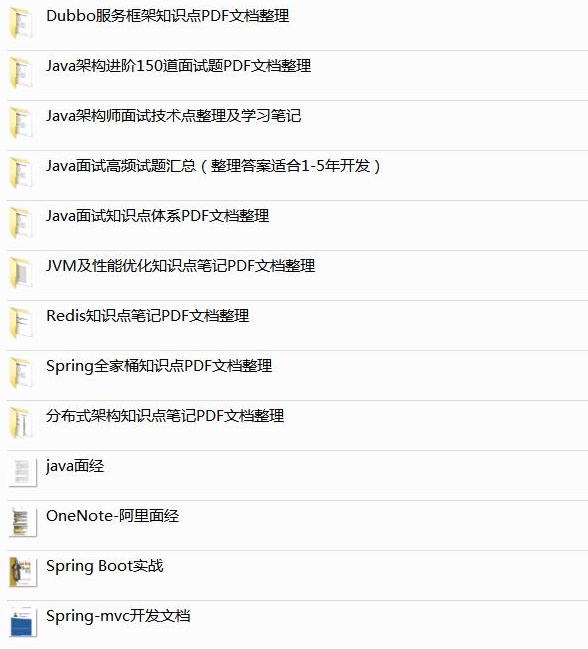
Netty、zookeeper、Spring cloud、分布式、高并发等架构资料和完整的Java架构学习进阶导图!**
**[CodeChina开源项目:【一线大厂Java面试题解析+核心总结学习笔记+最新讲解视频】](
)**[外链图片转存中...(img-rVXwmi0u-1631189236495)]
**更多Java架构进阶资料展示**
[外链图片转存中...(img-4JXToTRI-1631189236497)]
[外链图片转存中...(img-yAqI2w8N-1631189236500)]
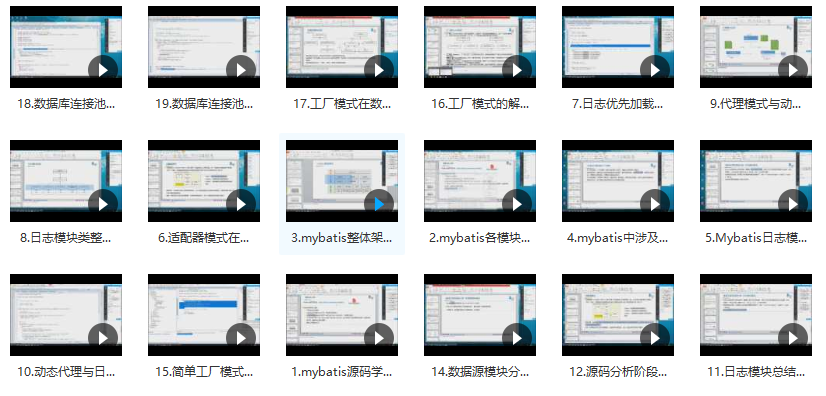