A. Divide and Conquer
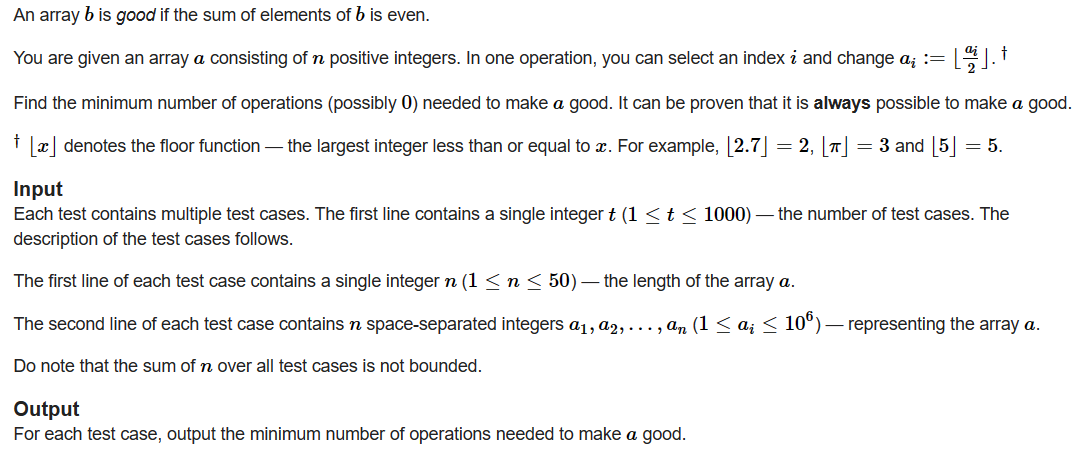
给出一个数组,每次操作可以选择一个位置,将它的数字除以2向下取整,问得到的数组之和是偶数时所需要的最少次数是多少。
思路:暴力判断每一位奇偶性改变时所需的最少操作数,如果当前的数组和不是偶数时,只需要改变其中一个数字的奇偶性即可。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
const int N = 2e5 + 5;
int t, n;
ll a[N];
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> t;
while(t --) {
std::cin >> n;
ll sum = 0;
for(int i = 1; i <= n; i ++) {
std::cin >> a[i];
sum += a[i];
}
if(sum % 2 == 0) {
std::cout << 0 << '\n';
continue;
}
int ans = 1e9;
for(int i = 1; i <= n; i ++) {
int res = a[i] % 2, cnt = 0;
while(a[i] % 2 == res) {
a[i] /= 2;
cnt ++;
if(!a[i]) break;
}
ans = std::min(ans, cnt);
}
std::cout << ans << '\n';
}
return 0;
}
B. Make Array Good
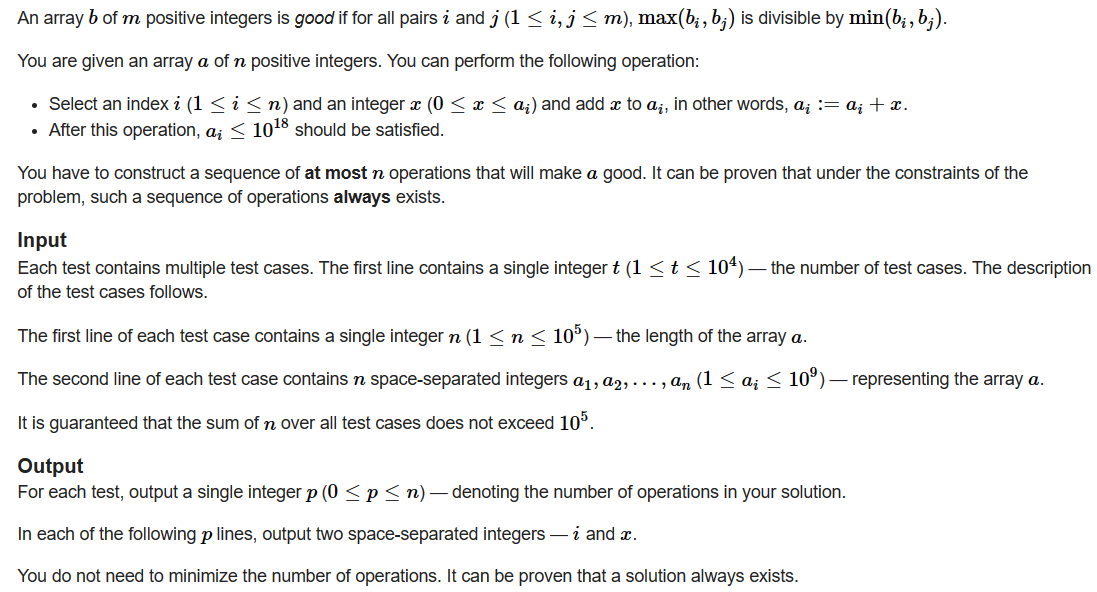
给出一个数组,每次操作可以选择一个数,将它加上一个小于该数的任意数字,构造这样的一系列操作,使得操作完成后任意两个数都有倍数关系。
思路:因为每次加上的数字都必须小于当前数字,而且必须每两个数都是倍数关系,则所有的数字都必须是某个数的倍数,所以取2,这样也可以保证每次加入的最小数都小于当前数字。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
typedef std::pair<int, ll> PII;
const int N = 2e5 + 5;
int t, n;
ll a[N];
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> t;
while(t --) {
std::cin >> n;
for(int i = 1; i <= n; i ++) {
std::cin >> a[i];
}
std::vector<PII> vec;
for(int i = 1; i <= n; i ++) {
ll num = 1;
while(num < a[i])
num <<= 1;
vec.push_back({i, num - a[i]});
}
std::cout << vec.size() << '\n';
for(auto [x, y] : vec)
std::cout << x << ' ' << y << '\n';
}
return 0;
}
C. Binary Strings are Fun
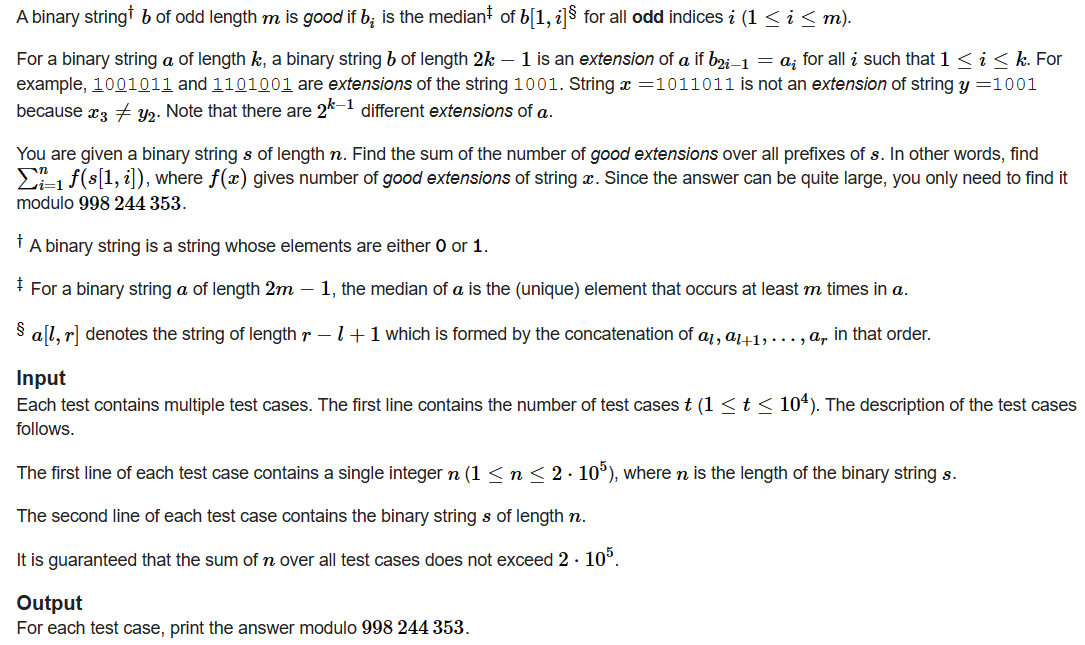
给出一个01串,求所有的前缀满足good extension有多少个。extension是对于01串,隔一个插入一个0或1构造的字符串,即构造得到的奇数位按顺序等于原字符串。good串是指对于01串中所有的奇数位都满足这一位的字符在前面出现过至少n/2次,n是当前奇数位的位置数。
思路:因为对于每个前缀都会满足条件,所以显然,如果当前位与前一位相同时,中间这一位插入0或1都可以,答案是前一位的答案*2;如果当前位和前一位不同,则中间只能插入与当前位相同的字符,答案为1,。顺序遍历答案累加即可。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
const int N = 2e5 + 5;
int t, n;
std::string s;
template<const int T>
struct ModInt {
const static int mod = T;
int x;
ModInt(int x = 0) : x(x % mod) {}
ModInt(ll x) : x(int(x % mod)) {}
int val() { return x; }
ModInt operator + (const ModInt &a) const { int x0 = x + a.x; return ModInt(x0 < mod ? x0 : x0 - mod); }
ModInt operator - (const ModInt &a) const { int x0 = x - a.x; return ModInt(x0 < 0 ? x0 + mod : x0); }
ModInt operator * (const ModInt &a) const { return ModInt(1LL * x * a.x % mod); }
ModInt operator / (const ModInt &a) const { return *this * a.inv(); }
void operator += (const ModInt &a) { x += a.x; if (x >= mod) x -= mod; }
void operator -= (const ModInt &a) { x -= a.x; if (x < 0) x += mod; }
void operator *= (const ModInt &a) { x = 1LL * x * a.x % mod; }
void operator /= (const ModInt &a) { *this = *this / a; }
friend std::ostream &operator<<(std::ostream &os, const ModInt &a) { return os << a.x;}
ModInt pow(int64_t n) const {
ModInt res(1), mul(x);
while(n){
if (n & 1) res *= mul;
mul *= mul;
n >>= 1;
}
return res;
}
ModInt inv() const {
int a = x, b = mod, u = 1, v = 0;
while (b) {
int t = a / b;
a -= t * b; std::swap(a, b);
u -= t * v; std::swap(u, v);
}
if (u < 0) u += mod;
return u;
}
};
typedef ModInt<998244353> mint;
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> t;
while(t --) {
std::cin >> n >> s;
s = ' ' + s;
mint ans = 0, cnt = 0;
for(int i = 1; i <= n; i ++) {
if(s[i] == s[i - 1]) {
cnt *= 2;
ans += cnt;
}
else {
cnt = 1;
ans += 1;
}
}
std::cout << ans << '\n';
}
return 0;
}
os:这个题题目不好懂
D. GCD Queries
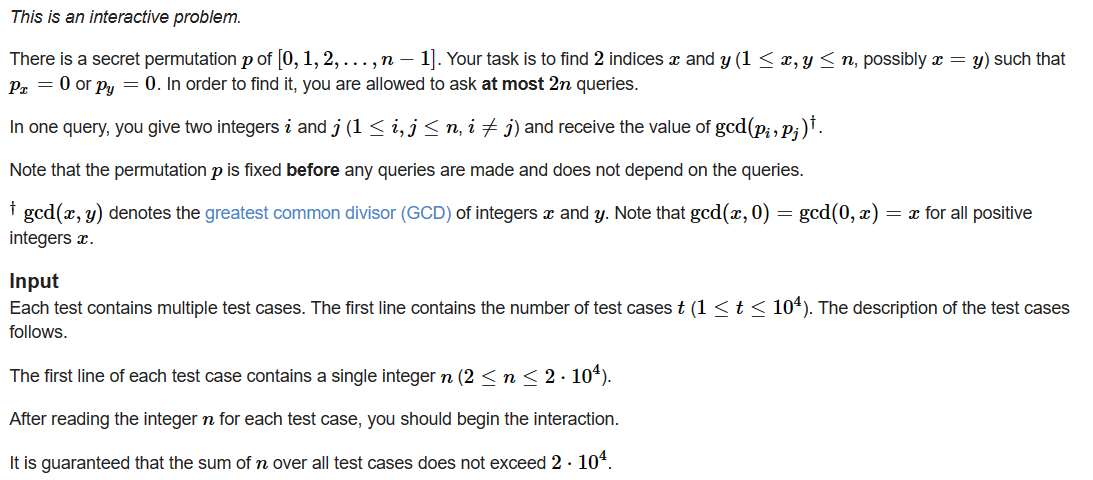
给出一个长度为n的0~n - 1的permutation,在2*n次询问内得到两个位置,其中一个是0。每次询问可以询问两个位置的数字的gcd。
思路:考虑在2*n次内删去n - 2个数,可以任意选择三个位置i,j,k,令x = gcd(i, j),y = gcd(j, k),根据x和y的大小关系删去数字。写法可以直接在队列里循环即可。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
const int N = 2e5 + 5;
int t, n;
int ask(int i, int j) {
std::cout << "? " << i << ' ' << j << '\n';
std::cout.flush();
int x;
std::cin >> x;
return x;
}
int main() {
// std::ios::sync_with_stdio(false);
// std::cin.tie(0);
// std::cout.tie(0);
std::cin >> t;
while(t --) {
std::cin >> n;
std::queue<int> q;
for(int i = 1; i <= n; i ++) {
q.push(i);
}
while(q.size() > 2) {
int i = q.front();
q.pop();
int j = q.front();
q.pop();
int k = q.front();
q.pop();
int x = ask(i, j), y = ask(j, k);
if(x == y) q.push(i), q.push(k);
else if(x > y) q.push(i), q.push(j);
else q.push(j), q.push(k);
}
int x = q.front();
q.pop();
int y = q.front();
q.pop();
std::cout << "! " << x << ' ' << y << '\n';
std::cout.flush();
std::cin >> x;
}
return 0;
}