迟了好久的补题,,现在真想把当时赛时的我拉出来捶一拳
排序大致按照题目难度。
C. 测量学
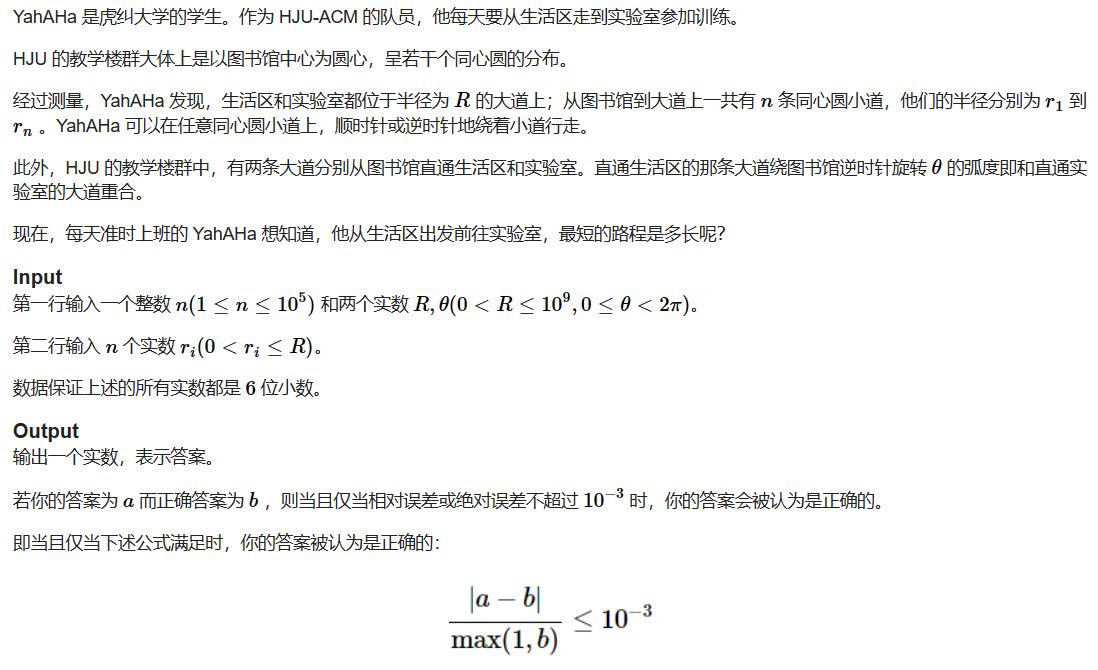
思路:直接循环遍历判断即可,注意角度要和2π取个最小值。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
const int N = 1e5 + 5;
const double PI = acos(-1);
int n;
double ang, r[N];;
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> n >> r[0] >> ang;
double ans = 1e18;
for(int i = 1; i <= n; i ++) {
std::cin >> r[i];
}
ang = std::min(ang, 2 * PI - ang);
for(int i = 0; i <= n; i ++) {
double res = (r[0] - r[i]) * 2 + r[i] * ang;
ans = std::min(ans, res);
}
std::cout << std::fixed << std::setprecision(6) << ans << '\n';
return 0;
}
A. 减肥计划
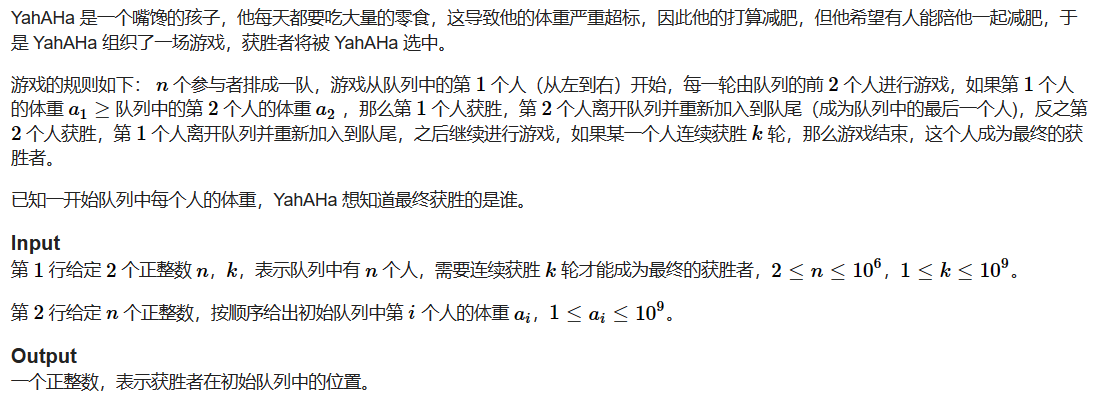
思路:由k的范围,容易想到k很大的时候情况:找到序列最靠前且最大的数输出即可。对于剩下的情况,k的范围较小,将原数组复制一遍,处理一个前缀最大的数组,遍历寻找一个位置,满足这个位置及之前的最大值是他自己,并且后面k -1位没有比它更大的。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
const int N = 2e6 + 5;
int n, k;
int a[N], pre[N];
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> n >> k;
int pos = -1, num = -1;
for(int i = 1; i <= n; i ++) {
std::cin >> a[i];
a[i + n] = a[i];
if(a[i] > num) {
num = a[i], pos = i;
}
}
if(k >= n - 1) {
std::cout << pos << '\n';
return 0;
}
for(int i = 1; i <= 2 * n; i ++) {
if(a[i] > pre[i - 1])
pre[i] = a[i];
else
pre[i] = pre[i - 1];
}
for(int i = 1; i <= 2 * n; i ++) {
if(pre[i] == a[i] && pre[k + i - 1] == a[i]) {
pos = i;
break;
}
}
std::cout << pos << '\n';
return 0;
}
E. 睡觉
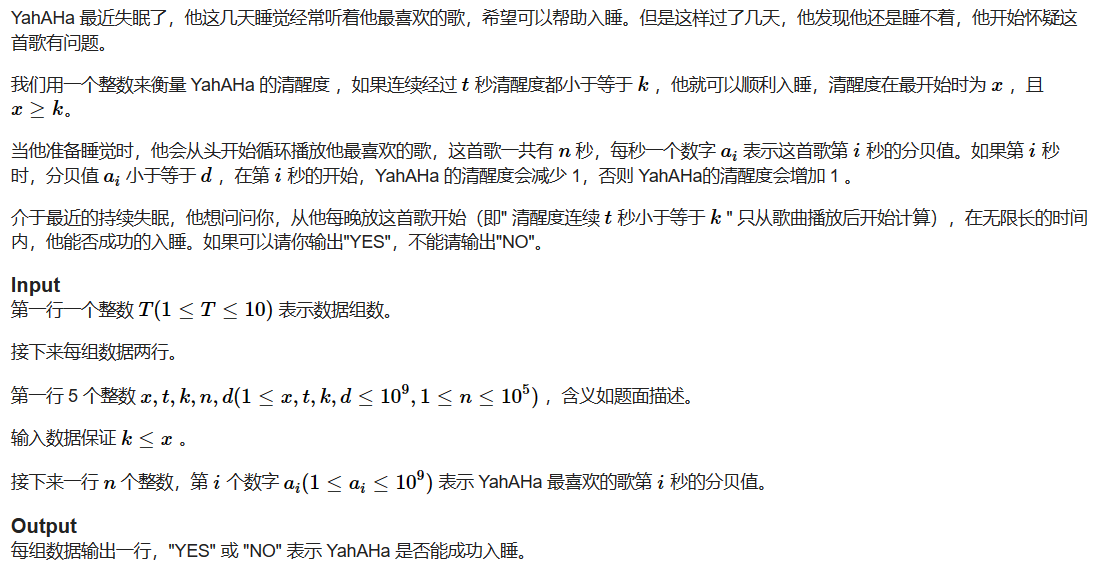
思路:主要是分情况讨论。我们经过一次循环,可以得到一个数字x1,由x1和原来的x的大小情况分类:
(1)x1小于x,说明每经过一首歌的影响,x都会减小,那么一定存在若干遍后,x小到满足条件;
(2)x1大于x,说明每经过一首歌的影响,x都会变大,那若存在满足情况的区间,那一定是在第一个区间最有可能满足条件,只需要在第一个区间内判断一下即可。
(3)x1等于x,这样可以想到原来x的性质,如果原来是大于k的,那情况和第二种情况类似,判断第一个区间即可;但是若是原来是等于k的,那极有可能第一段满足条件的区间和最后一段满足条件的区间的和是满足大于等于t的,所以要处理连续两个区间,当然要注意t的大小,如果t是大于等于n的,那必须两个个区间内的最大值要大于n才行。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
#define int long long
const int N = 2e6 + 5;
int T, x, t, k, n, d;
int a[N];
signed main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> T;
while(T --) {
std::cin >> x >> t >> k >> n >> d;
x -= k;
int original = x;
int cnt = 0, max = -1;;
for(int i = 1; i <= n; i ++) {
std::cin >> a[i];
if(a[i] <= d)
a[i] = -1;
else
a[i] = 1;
a[i + n] = a[i];
x += a[i];
if(x <= 0)
cnt ++;
else
cnt = 0;
max = std::max(max, cnt);
}
if(x < original) {
std::cout << "YES" << '\n';
continue;
}
if(x > original) {
std::cout << (max >= t ? "YES" : "NO") << '\n';
continue;
}
if(original > 0) {
std::cout << (max >= t ? "YES" : "NO") << '\n';
continue;
}
std::vector<int> vec;
x = original;
cnt = 0;
for(int i = 1; i <= n; i ++) {
x += a[i];
if(x <= 0) {
cnt ++;
}
else {
vec.push_back(cnt);
cnt = 0;
}
}
vec.push_back(cnt);
vec.push_back(vec[0] + vec[vec.size() - 1]);
max = *max_element(vec.begin(), vec.end());
t = std::min(t, n);
std::cout << (max >= t ? "YES" : "NO") << '\n';
}
return 0;
}
G. 排队打卡
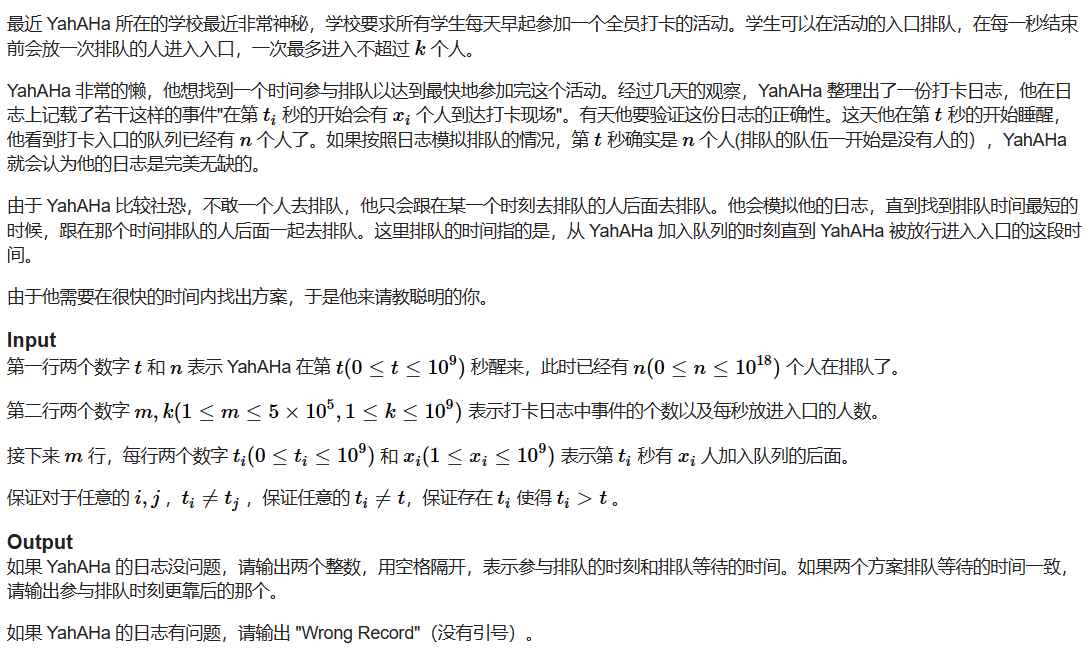
思路:按照题意模拟即可,注意给出的数据分段,不要搞错了边界。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
#define int long long
const int N = 5e5 + 5;
int t, n, m, k;
struct node {
int t, x;
} e[N];
bool cmp(node a, node b) {
if(a.t < b.t) return true;
else return false;
}
signed main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
int sum = 0, pos = -1;
std::cin >> t >> n >> m >> k;
for(int i = 1; i <= m; i ++) {
std::cin >> e[i].t >> e[i].x;
}
std::sort(e + 1, e + 1 + m, cmp);
for(int i = 1; i <= m; i ++) {
if(e[i].t > t && pos == -1)
pos = i - 1;
}
if(!pos) {
if(n) {
std::cout << "Wrong Record" << '\n';
return 0;
}
}
else {
sum = e[1].x;
for(int i = 2; i <= pos; i ++) {
sum -= std::min(sum, k * (e[i].t - e[i - 1].t));
sum += e[i].x;
}
sum -= std::min(sum, k * (t - e[pos].t));
if(sum != n) {
std::cout << "Wrong Record" << '\n';
return 0;
}
}
int last = t, cnt = 5e18, ans;
for(int i = pos + 1; i <= m; i ++) {
sum -= std::min(sum, k * (e[i].t - last));
sum += e[i].x;
last = e[i].t;
int res = (int)ceil((sum + 1) * 1.0 / (double)k);
if(res <= cnt) {
cnt = res;
ans = e[i].t;
}
}
std::cout << ans << ' ' << cnt << '\n';
return 0;
}
os:现在写来感觉这个题比E要简单,按照题意写就行了,也没啥需要思考的,为啥当时就没做出来呢
I. 宠物对战
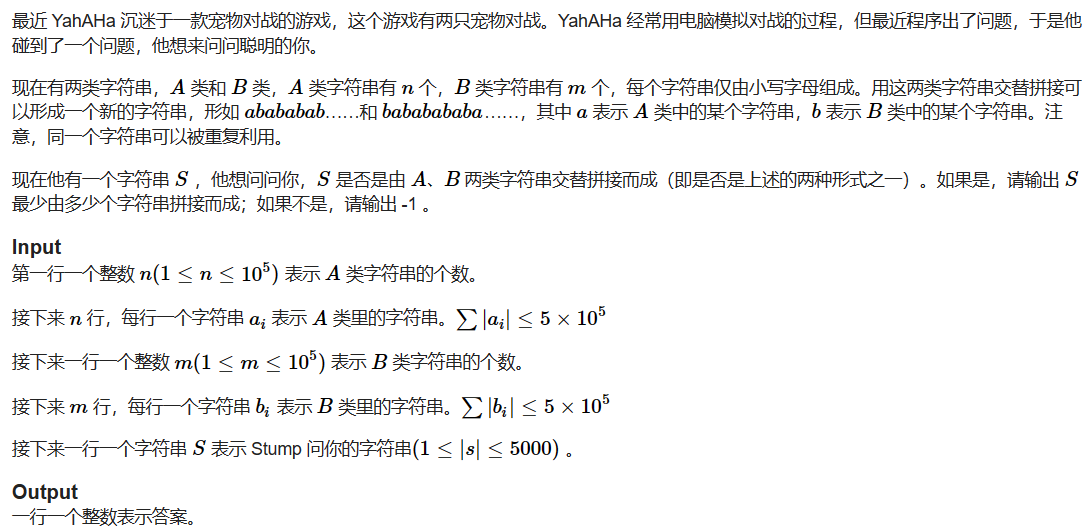
思路:其实看题目可以知道是一个比较典的dp问题。可以这样考虑,令f[i][0/1]表示处理到第i个字母,是由A中的字符串还是由B中的字符串组成的。可以考虑循环枚举最后到的位置,和这一整个字符串最后一个子字符串的开始位置,判断这个子字符串是否出自A或B组中,若是,则可以更新:
f[i][1/0] = min(f[i][1/0], f[j - 1][0/1] + 1);
而对于子字符串是否存在于A或者B组中,可以采用Trie树或者字符串哈希来处理,在这里我用的是字典树,但是在查询的时候加入了一点优化,如果直接查询的话会T飞欸。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
#define INF 0x3f3f3f3f
const int N = 5e5 + 5;
int n, m;
std::string a[N], b[N], s;
int f[N][2];
struct Trie {
int idx = 0;
int mp[N][26], cnt[N];
void insert(std::string s) {
int p = 0;
for(int i = 0; i < s.length(); i ++) {
int u = s[i] - 'a';
if(!mp[p][u]) mp[p][u] = ++ idx;
p = mp[p][u];
}
cnt[p] ++;
}
}A, B;
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> n;
for(int i = 1; i <= n; i ++) {
std::cin >> a[i];
A.insert(a[i]);
}
std::cin >> m;
for(int i = 1; i <= m; i ++) {
std::cin >> b[i];
B.insert(b[i]);
}
std::cin >> s;
int len = s.length();
s = ' ' + s;
memset(f, INF, sizeof(f));
f[0][1] = f[0][0] = 0;
for(int i = 0; i <= len; i ++) {
if(f[i][0] < INF) {
int p = 0;
for(int j = i + 1; j <= len; j ++) {
int u = s[j] - 'a';
if(A.mp[p][u]) {
p = A.mp[p][u];
if(A.cnt[p]) {
f[j][1] = std::min(f[j][1], f[i][0] + 1);
}
}
else break;
}
}
if(f[i][1] < INF) {
int p = 0;
for(int j = i + 1; j <= len; j ++) {
int u = s[j] - 'a';
if(B.mp[p][u]) {
p = B.mp[p][u];
if(B.cnt[p]) {
f[j][0] = std::min(f[j][0], f[i][1] + 1);
}
}
else break;
}
}
}
int ans = std::min(f[len][1], f[len][0]);
std::cout << (ans == INF ? -1 : ans) << '\n';
return 0;
}
os:学弟给的优化方法,tqlllllllll
H. 提瓦特之旅
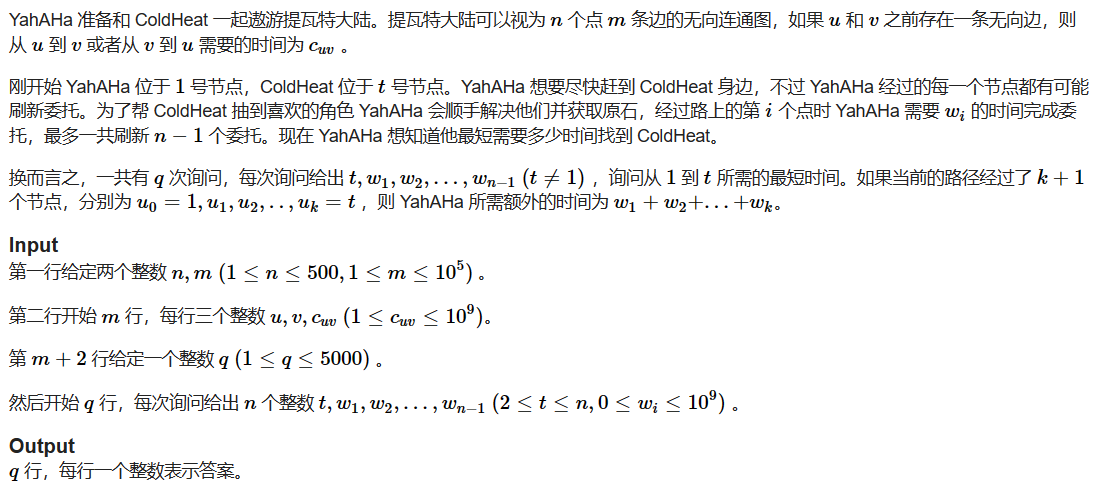
思路:如果说对于每个询问都跑一次最短路的话,那必然会t,想办法通过离线预处理部分东西来降低部分时间复杂度。所以可以通过预处理数组dis[i][j],表示经过i个点到达j的最短路径,这样在每次询问的时候就可以在O(n * q)的复杂度内得到答案。
AC Code:
#include <bits/stdc++.h>
typedef long long ll;
typedef std::pair<int, int> PII;
const int N = 505;
int n, m, q, x, t;
ll dis[N][N];
std::vector<PII> vec[N];
int main() {
std::ios::sync_with_stdio(false);
std::cin.tie(0);
std::cout.tie(0);
std::cin >> n >> m;
for(int i = 1; i <= m; i ++) {
int u, v, w;
std::cin >> u >> v >> w;
vec[u].push_back({v, w});
vec[v].push_back({u, w});
}
for(int i = 0; i <= n; i ++) {
for(int j = 0; j <= n; j ++) {
dis[i][j] = 3e18;
}
}
dis[0][1] = 0;
for(int i = 1; i <= n; i ++) {
for(int j = 1; j <= n; j ++) {
for(auto [u, w] : vec[j]) {
dis[i][j] = std::min(dis[i][j], dis[i - 1][u] + w);
}
}
}
std::cin >> q;
while(q --) {
std::cin >> t;
ll sum = 0, ans = 3e18;
for(int i = 1; i < n; i ++) {
std::cin >> x;
sum += x;
ans = std::min(ans, dis[i][t] + sum);
}
std::cout << ans << '\n';
}
return 0;
}
os:个人感觉这个题比前一个好写很多欸,但是可能就是怎样预处理卡住了吧QWQ
这次让我没想到的是和前几年难度差别这么大,感觉大概到了省赛难度吧,明年加油哇